Python のデコレータ メカニズムと最新の型ヒント機能を組み合わせることで、テストの自動化が大幅に向上します。 Python の柔軟性と typing
モジュールのタイプ セーフを活用したこの強力な組み合わせにより、より保守しやすく、読みやすく、堅牢なテスト スイートが実現します。この記事では、テスト自動化フレームワーク内での応用に焦点を当てながら、高度なテクニックについて説明します。
typing
モジュールの拡張機能の活用
typing
モジュールは大幅な改善を受けました:
-
PEP 585: 標準コレクションのジェネリック型のネイティブ サポートにより、共通型の
typing
モジュールへの依存が最小限に抑えられます。 -
PEP 604:
|
演算子は Union 型の注釈を簡素化します。 -
PEP 647:
TypeAlias
は型別名の定義を明確にします。 - PEP 649: 注釈評価の遅延により、大規模プロジェクトの起動が高速化されます。
型指定されたパラメータ化されたデコレータの構築
これらの更新された入力機能を使用してデコレーターを作成する方法は次のとおりです。
from typing import Protocol, TypeVar, Generic, Callable, Any from functools import wraps # TypeVar for generic typing T = TypeVar('T') # Protocol for defining function structure class TestProtocol(Protocol): def __call__(self, *args: Any, **kwargs: Any) -> Any: ... def generic_decorator(param: str) -> Callable[[Callable[..., T]], Callable[..., T]]: """ Generic decorator for functions returning type T. Args: param: A string parameter. Returns: A callable wrapping the original function. """ def decorator(func: Callable[..., T]) -> Callable[..., T]: @wraps(func) # Preserves original function metadata def wrapper(*args: Any, **kwargs: Any) -> T: print(f"Decorator with param: {param}") return func(*args, **kwargs) return wrapper return decorator @generic_decorator("test_param") def test_function(x: int) -> int: """Returns input multiplied by 2.""" return x * 2
このデコレータは Protocol
を使用してテスト関数の構造を定義し、テスト フレームワークでのさまざまな関数シグネチャの柔軟性を高めます。
テスト自動化へのデコレータの適用
これらのデコレーターがテストの自動化をどのように強化するかを調べてみましょう:
1. Literal
from typing import Literal, Callable, Any import sys def run_only_on(platform: Literal["linux", "darwin", "win32"]) -> Callable: """ Runs a test only on the specified platform. Args: platform: Target platform. Returns: A callable wrapping the test function. """ def decorator(func: Callable) -> Callable: @wraps(func) def wrapper(*args: Any, **kwargs: Any) -> Any: if sys.platform == platform: return func(*args, **kwargs) print(f"Skipping test on platform: {sys.platform}") return None return wrapper return decorator @run_only_on("linux") def test_linux_feature() -> None: """Linux-specific test.""" pass
Literal
は、型チェッカーが有効な platform
値を認識することを保証し、どのテストがどのプラットフォームで実行されるかを明確にし、クロスプラットフォーム テストにとって重要です。
2.スレッド化を使用したタイムアウト デコレータ
from typing import Callable, Any, Optional import threading import time from concurrent.futures import ThreadPoolExecutor, TimeoutError def timeout(seconds: int) -> Callable: """ Enforces a timeout on test functions. Args: seconds: Maximum execution time. Returns: A callable wrapping the function with timeout logic. """ def decorator(func: Callable) -> Callable: @wraps(func) def wrapper(*args: Any, **kwargs: Any) -> Optional[Any]: with ThreadPoolExecutor(max_workers=1) as executor: future = executor.submit(func, *args, **kwargs) try: return future.result(timeout=seconds) except TimeoutError: print(f"Function {func.__name__} timed out after {seconds} seconds") return None return wrapper return decorator @timeout(5) def test_long_running_operation() -> None: """Test that times out if it takes too long.""" time.sleep(10) # Triggers timeout
これは、テストの実行時間を制御する際に不可欠な、信頼性の高いタイムアウト機能のためにスレッドを使用します。
3. Union タイプを使用した再試行メカニズム
from typing import Callable, Any, Union, Type, Tuple, Optional import time def retry_on_exception( exceptions: Union[Type[Exception], Tuple[Type[Exception], ...]], attempts: int = 3, delay: float = 1.0 ) -> Callable: """ Retries a function on specified exceptions. Args: exceptions: Exception type(s) to catch. attempts: Maximum retry attempts. delay: Delay between attempts. Returns: A callable wrapping the function with retry logic. """ def decorator(func: Callable) -> Callable: @wraps(func) def wrapper(*args: Any, **kwargs: Any) -> Any: last_exception: Optional[Exception] = None for attempt in range(attempts): try: return func(*args, **kwargs) except exceptions as e: last_exception = e print(f"Attempt {attempt + 1} failed with {type(e).__name__}: {str(e)}") time.sleep(delay) if last_exception: raise last_exception return wrapper return decorator @retry_on_exception(Exception, attempts=5) def test_network_connection() -> None: """Test network connection with retry logic.""" pass
この洗練されたバージョンでは、包括的な型ヒント、堅牢な例外処理、構成可能な再試行遅延が使用されます。 Union
タイプを使用すると、例外タイプを柔軟に指定できます。
結論
Python の高度な型指定機能をデコレーターに統合すると、型安全性とコードの可読性の両方が向上し、テスト自動化フレームワークが大幅に強化されます。 明示的な型定義により、適切なエラー処理とパフォーマンス制約を備えた正しい条件下でテストが実行されることが保証されます。これにより、より堅牢で保守性が高く、効率的なテストが可能になり、特に大規模な分散テスト環境、またはマルチプラットフォームのテスト環境で価値があります。
以上がテスト自動化における Python の型付きパラメーター化デコレーターの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
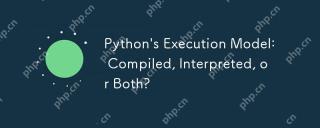
pythonisbothcompiledinterted.whenyourunapythonscript、itisfirstcompiledintobytecode、これはdenepythonvirtualmachine(pvm).thishybridapproaChallowsforplatform-platform-denodent-codebutcututicut。
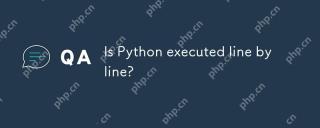
Pythonは厳密に行ごとの実行ではありませんが、最適化され、インタープレーターメカニズムに基づいて条件付き実行です。インタープリターは、コードをPVMによって実行されるBytecodeに変換し、定数式または最適化ループを事前促進する場合があります。これらのメカニズムを理解することで、コードを最適化し、効率を向上させることができます。
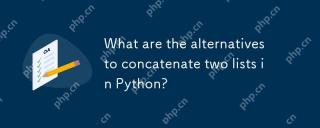
Pythonに2つのリストを接続する多くの方法があります。1。オペレーターを使用しますが、これはシンプルですが、大きなリストでは非効率的です。 2。効率的ですが、元のリストを変更する拡張メソッドを使用します。 3。=演算子を使用します。これは効率的で読み取り可能です。 4。itertools.chain関数を使用します。これはメモリ効率が高いが、追加のインポートが必要です。 5。リストの解析を使用します。これはエレガントですが、複雑すぎる場合があります。選択方法は、コードのコンテキストと要件に基づいている必要があります。
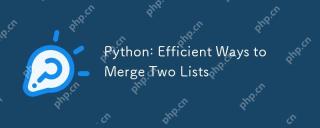
Pythonリストをマージするには多くの方法があります。1。オペレーターを使用します。オペレーターは、シンプルですが、大きなリストではメモリ効率的ではありません。 2。効率的ですが、元のリストを変更する拡張メソッドを使用します。 3. Itertools.chainを使用します。これは、大規模なデータセットに適しています。 4.使用 *オペレーター、1つのコードで小規模から中型のリストをマージします。 5. numpy.concatenateを使用します。これは、パフォーマンス要件の高い大規模なデータセットとシナリオに適しています。 6.小さなリストに適したが、非効率的な追加方法を使用します。メソッドを選択するときは、リストのサイズとアプリケーションのシナリオを考慮する必要があります。
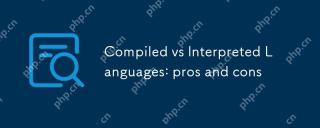
compiledlanguagesOfferspeedandsecurity、foredlanguagesprovideeaseofuseandportability.1)compiledlanguageslikec arefasterandsecurebuthavelOnderdevelopmentsplat dependency.2)
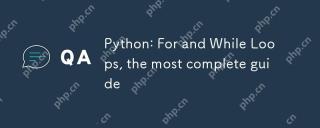
Pythonでは、forループは反復可能なオブジェクトを通過するために使用され、条件が満たされたときに操作を繰り返し実行するためにしばらくループが使用されます。 1)ループの例:リストを通過し、要素を印刷します。 2)ループの例:正しいと推測するまで、数値ゲームを推測します。マスタリングサイクルの原則と最適化手法は、コードの効率と信頼性を向上させることができます。
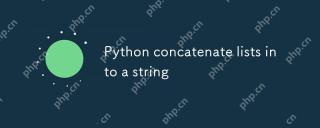
リストを文字列に連結するには、PythonのJoin()メソッドを使用して最良の選択です。 1)join()メソッドを使用して、 '' .join(my_list)などのリスト要素を文字列に連結します。 2)数字を含むリストの場合、連結する前にマップ(str、数字)を文字列に変換します。 3) '、'などの複雑なフォーマットに発電機式を使用できます。 4)混合データ型を処理するときは、MAP(STR、Mixed_List)を使用して、すべての要素を文字列に変換できるようにします。 5)大規模なリストには、 '' .join(lage_li)を使用します
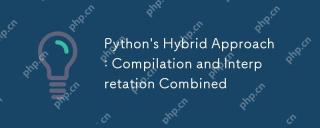
pythonusesahybridapproach、コンコイリティレーショントビテコードと解釈を組み合わせて、コードコンピレッドフォームと非依存性bytecode.2)


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
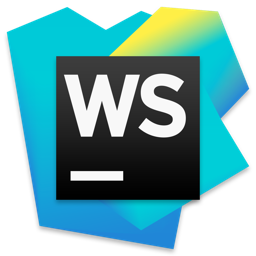
WebStorm Mac版
便利なJavaScript開発ツール

SublimeText3 中国語版
中国語版、とても使いやすい
