このコードは、2 次元点のセットで凸状の穴を見つけるアプローチを示します。このアプローチには、点群のビットマップの作成、ビットマップ内の各セルのデータ密度の計算、未使用領域のリストの作成 (map[][] = 0 または map[][]
アルゴリズムの C# 実装は次のとおりです。提供:
using System; using System.Collections.Generic; using System.Linq; using System.Drawing; namespace HoleFinder { class Program { // Define a cell structure for the bitmap public struct Cell { public double x0, x1, y0, y1; // Bounding box of points inside the cell public int count; // Number of points inside the cell } // Define a line structure for representing hole boundaries public struct Line { public double x0, y0, x1, y1; // Line edge points public int id; // Id of the hole to which the line belongs for segmentation/polygonization public int i0, i1, j0, j1; // Index in map[][] } // Compute the bounding box of the point cloud public static (double x0, double x1, double y0, double y1) ComputeBoundingBox(List<point> points) { double x0 = points[0].X; double x1 = points[0].X; double y0 = points[0].Y; double y1 = points[0].Y; foreach (var point in points) { if (point.X x1) x1 = point.X; if (point.Y y1) y1 = point.Y; } return (x0, x1, y0, y1); } // Create a bitmap of the point cloud public static int[,] CreateBitmap(List<point> points, (double x0, double x1, double y0, double y1) boundingBox, int N) { // Create a 2D array to represent the bitmap int[,] bitmap = new int[N, N]; // Compute the scale factors for converting point coordinates to bitmap indices double mx = N / (boundingBox.x1 - boundingBox.x0); double my = N / (boundingBox.y1 - boundingBox.y0); // Iterate over the points and increment the corresponding cells in the bitmap foreach (var point in points) { int i = (int)Math.Round((point.X - boundingBox.x0) * mx); int j = (int)Math.Round((point.Y - boundingBox.y0) * my); if (i >= 0 && i = 0 && j FindUnusedAreasHorizontalVertical(Cell[] map, int N, int treshold = 0) { List unusedAreas = new List(); // Scan horizontally for (int j = 0; j = 0) { unusedAreas.Add((i0, i1, j, j)); i0 = -1; i1 = -1; } } } if (i0 >= 0) unusedAreas.Add((i0, i1, j, j)); } // Scan vertically for (int i = 0; i = 0) { unusedAreas.Add((i, i, j0, j1)); j0 = -1; j1 = -1; } } } if (j0 >= 0) unusedAreas.Add((i, i, j0, j1)); } return unusedAreas; } // Segment the list of unused areas into groups of connected components public static List<list i0 int i1 j0 j1>> SegmentUnusedAreas(List unusedAreas) { // Initialize each unused area as a separate group List<list i0 int i1 j0 j1>> segments = new List<list i0 int i1 j0 j1>>(); foreach (var unusedArea in unusedAreas) { segments.Add(new List { unusedArea }); } // Iterate until no more segments can be joined bool joined = true; while (joined) { joined = false; // Check if any two segments intersect or are adjacent for (int i = 0; i = unusedArea2.i0 && unusedArea1.j0 = unusedArea2.j0) { intersects = true; break; } } if (intersects) break; } // Check for adjacency bool adjacent = false; if (!intersects) { foreach (var unusedArea1 in segments[i]) { foreach (var unusedArea2 in segments[j]) { if (unusedArea1.i0 == unusedArea2.i0 && unusedArea1.i1 == unusedArea2.i1 && ((unusedArea1.j1 == unusedArea2.j0 && Math.Abs(unusedArea1.j0 - unusedArea2.j1) == 1) || (unusedArea1.j0 == unusedArea2.j1 && Math.Abs(unusedArea1.j1 - unusedArea2.j0) == 1))) { adjacent = true; break; } if (unusedArea1.j0 == unusedArea2.j0 && unusedArea1.j1 == unusedArea2.j1</list></list></list></point></point>
以上がC# を使用して 2D 点群内の凸穴を識別して多角形化するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
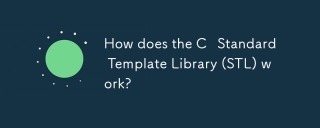
この記事では、C標準テンプレートライブラリ(STL)について説明し、そのコアコンポーネント(コンテナ、イテレーター、アルゴリズム、およびファンクター)に焦点を当てています。 これらが一般的なプログラミングを有効にし、コード効率を向上させ、読みやすさを改善する方法を詳述しています。
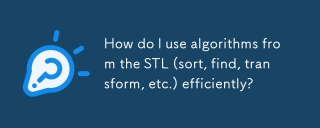
この記事では、cの効率的なSTLアルゴリズムの使用について詳しく説明しています。 データ構造の選択(ベクトル対リスト)、アルゴリズムの複雑さ分析(STD :: STD :: STD :: PARTIAL_SORTなど)、イテレーターの使用、および並列実行を強調しています。 のような一般的な落とし穴
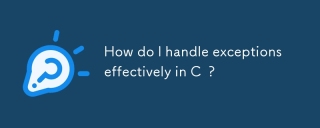
この記事では、Cでの効果的な例外処理、トライ、キャッチ、スローメカニックをカバーしています。 RAIIなどのベストプラクティス、不必要なキャッチブロックを避け、ログの例外をロギングすることを強調しています。 この記事では、パフォーマンスについても説明しています
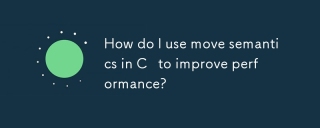
この記事では、不必要なコピーを回避することにより、パフォーマンスを向上させるために、CのMove Semanticsを使用することについて説明します。 STD :: MOVEを使用して、移動コンストラクターと割り当てオペレーターの実装をカバーし、効果的なAPPLの重要なシナリオと落とし穴を識別します
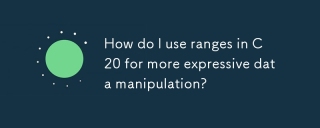
C 20の範囲は、表現力、複合性、効率を伴うデータ操作を強化します。複雑な変換を簡素化し、既存のコードベースに統合して、パフォーマンスと保守性を向上させます。
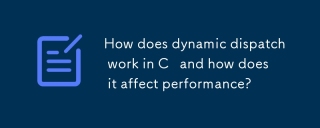
この記事では、Cでの動的発送、そのパフォーマンスコスト、および最適化戦略について説明します。動的ディスパッチがパフォーマンスに影響を与え、静的ディスパッチと比較するシナリオを強調し、パフォーマンスとパフォーマンスのトレードオフを強調します
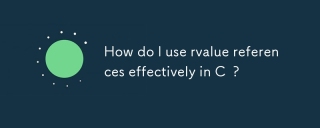
記事では、移動セマンティクス、完璧な転送、リソース管理のためのcでのr値参照の効果的な使用について説明し、ベストプラクティスとパフォーマンスの改善を強調しています。(159文字)
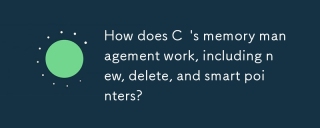
Cメモリ管理は、新しい、削除、およびスマートポインターを使用します。この記事では、マニュアルと自動化された管理と、スマートポインターがメモリリークを防ぐ方法について説明します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール
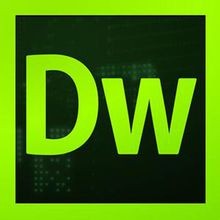
ドリームウィーバー CS6
ビジュアル Web 開発ツール

メモ帳++7.3.1
使いやすく無料のコードエディター

Safe Exam Browser
Safe Exam Browser は、オンライン試験を安全に受験するための安全なブラウザ環境です。このソフトウェアは、あらゆるコンピュータを安全なワークステーションに変えます。あらゆるユーティリティへのアクセスを制御し、学生が無許可のリソースを使用するのを防ぎます。

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!
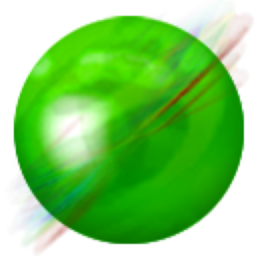
ZendStudio 13.5.1 Mac
強力な PHP 統合開発環境

ホットトピック



