「ああ、もう…私の状態はまためちゃくちゃです。」
React で状態を管理するときに、次のような問題に遭遇したことはありますか?
- useState と useReducer は便利ですが、コンポーネントの数が増えると状態の受け渡しが面倒になります。
- 複数のコンポーネント間で状態を共有するには、プロップドリルまたは useContext の導入に頼ることがよくあります。
- Redux のようなライブラリは強力ですが、学習には時間がかかります。
「状態を管理するもっと簡単な方法はないでしょうか?」
それが私が F-Box React を作成した理由です。
F-Box React を使用すると、状態管理のボイラープレートから解放され、コードをシンプルに保つことができます!
目次
- はじめに
- 基本的な例: カウンター アプリ
- RBox: React の外部で使用可能
- 複数のコンポーネント間で状態を共有する
- useReducer の代わりとして useRBox を使用する
- F-Box React の詳細と背景
- 結論
導入
F-Box React の使用方法を理解するために、具体的なコード例を見てみましょう。このセクションでは、例として単純なカウンター アプリを使用して useState と useRBox を比較します。
基本的な例: カウンター アプリ
通常の React 方法 (useState)
import { useState } from "react" function Counter() { const [count, setCount] = useState(0) return ( <div> <p>Count: {count}</p> <button onclick="{()"> setCount(count + 1)}>+1</button> </div> ) } export default Counter
この古典的なアプローチでは、useState を使用してカウントを管理します。
F-Box React の使用
import { useRBox, set } from "f-box-react" function Counter() { const [count, countBox] = useRBox(0) // Create an RBox with initial value 0 const setCount = set(countBox) // Get a convenient updater function for the RBox return ( <div> <p>Count: {count}</p> <button onclick="{()"> setCount(count + 1)}>+1</button> </div> ) } export default Counter
ここでは、useRBox を使用してカウンターを実装します。 useRBox は [value, RBox] ペアを返すため、useState.
とほぼ同じように使用できます。RBox: React の外部で使用可能
import { RBox } from "f-box-core" const numberBox = RBox.pack(0) // Subscribe to changes and log updates numberBox.subscribe((newValue) => { console.log(`Updated numberBox: ${newValue}`) }) // Change the value, which notifies subscribers reactively numberBox.setValue((prev) => prev + 1) // Updated numberBox: 1 numberBox.setValue((prev) => prev + 10) // Updated numberBox: 11
上で示したように、RBox は React に依存しないため、あらゆる TypeScript コードでリアクティブなデータ管理に使用できます。
複数のコンポーネント間での状態の共有
通常の React 方法 (useContext を使用)
import React, { createContext, useContext, useState } from "react" const CounterContext = createContext() function CounterProvider({ children }) { const [count, setCount] = useState(0) return ( <countercontext.provider value="{{" count setcount> {children} </countercontext.provider> ) } function CounterDisplay() { const { count } = useContext(CounterContext) return <p>Count: {count}</p> } function CounterButton() { const { setCount } = useContext(CounterContext) return <button onclick="{()"> setCount((prev) => prev + 1)}>+1</button> } function App() { return ( <counterprovider> <counterdisplay></counterdisplay> <counterbutton></counterbutton> </counterprovider> ) } export default App
このメソッドは useContext を使用して状態を共有しますが、コードが冗長になる傾向があります。
F-Box React の使用
import { RBox } from "f-box-core" import { useRBox } from "f-box-react" // Define a global RBox const counterBox = RBox.pack(0) function CounterDisplay() { const [count] = useRBox(counterBox) return <p>Count: {count}</p> } function CounterButton() { return ( <button onclick="{()"> counterBox.setValue((prev) => prev + 1)}>+1</button> ) } function App() { return ( <div> <counterdisplay></counterdisplay> <counterbutton></counterbutton> </div> ) } export default App
ここでは、グローバル RBox を定義し、各コンポーネントで useRBox を使用して状態を共有します。これにより、useContext やプロバイダーが不要になり、コードが単純になります。
useReducer の代替として useRBox を使用する
通常の React 方法 (useReducer を使用)
import { useReducer } from "react" type State = { name: string age: number } type Action = | { type: "incremented_age" } | { type: "changed_name"; nextName: string } function reducer(state: State, action: Action): State { switch (action.type) { case "incremented_age": { return { name: state.name, age: state.age + 1, } } case "changed_name": { return { name: action.nextName, age: state.age, } } } } const initialState = { name: "Taylor", age: 42 } export default function Form() { const [state, dispatch] = useReducer(reducer, initialState) function handleButtonClick() { dispatch({ type: "incremented_age" }) } function handleInputChange(e: React.ChangeEvent<htmlinputelement>) { dispatch({ type: "changed_name", nextName: e.target.value, }) } return ( <input value="{state.name}" onchange="{handleInputChange}"> <button onclick="{handleButtonClick}">Increment age</button> <p> Hello, {state.name}. You are {state.age}. </p> > ) } </htmlinputelement>
F-Box React の使用
import { useRBox, set } from "f-box-react" function useUserState(_name: string, _age: number) { const [name, nameBox] = useRBox(_name) const [age, ageBox] = useRBox(_age) return { user: { name, age }, changeName(e: React.ChangeEvent<htmlinputelement>) { set(nameBox)(e.target.value) }, incrementAge() { ageBox.setValue((prev) => prev + 1) }, } } export default function Form() { const { user, changeName, incrementAge } = useUserState("Taylor", 42) return ( <input value="{user.name}" onchange="{changeName}"> <button onclick="{incrementAge}">Increment age</button> <p> Hello, {user.name}. You are {user.age}. </p> > ) } </htmlinputelement>
useRBox を使用すると、リデューサーやアクション タイプを定義せずに状態を管理できるため、コードが簡素化されます。
F-Box Reactの詳細と背景
これまで、コード例を通して F-Box React の基本的な使い方を紹介してきました。次に、次の詳細情報について説明します:
- 背景: F-Box React はなぜ作成されたのですか?
- コアコンセプト (RBox と useRBox の詳細)
- インストールとセットアップ手順
これらの点は、F-Box React をより深く理解するために重要です。
背景: F-Box React はなぜ作成されたのですか?
元々、私は純粋に関数型プログラミング用の汎用ライブラリとして F-Box (f-box-core) を開発しました。 F-Box は、データ変換、副作用、非同期計算を簡素化するために、Box、Maybe、Either、Task などの抽象化を提供します。
F-Box 内に、RBox という名前のリアクティブ コンテナーが導入されました。 RBox は値の変化を監視し、事後対応的な状態管理を可能にします。
RBox を作成した後、「このリアクティブ ボックスを React に統合したらどうなるでしょうか? React アプリケーションの状態管理を簡素化できるかもしれません。」と考えました。この考えに基づいて、私は F-Box React (f-box-react) を開発しました。これは、React コンポーネント内で RBox を簡単に使用できるようにするフックのコレクションです。
その結果、F-Box React は驚くほどユーザーフレンドリーであることが判明し、シンプルかつ柔軟な方法で React の状態を管理するための強力なツールを提供しました。
中心となる概念
F-Box React の主要な要素は次のとおりです:
RBox
リアクティブな状態管理を可能にするコンテナ。 React とは独立して状態の変化を監視および管理できます。RBox を使用
React コンポーネント内で RBox を簡単に使用するためのカスタム フック。 useState に似た直感的な API を提供し、リアクティブな値を取得および更新できます。
これらの要素は次のことを意味します:
使用感あり状態
状態の処理は useState と同様に直感的です。複数のコンポーネント間で状態を簡単に共有します
複数のコンポーネント間で状態を簡単に共有できます。RBox は React 外でも使用できます
Reactに依存しないため、React以外の環境でも利用可能です
これにより、状態管理が非常に簡単になります。
インストールとセットアップの手順
F-Box React をプロジェクトに統合するには、npm または Yarn を使用して次のコマンドを実行します。 F-Box React は f-box-core に依存しているため、両方を同時にインストールする必要があります:
import { useState } from "react" function Counter() { const [count, setCount] = useState(0) return ( <div> <p>Count: {count}</p> <button onclick="{()"> setCount(count + 1)}>+1</button> </div> ) } export default Counter
インストール後、前の例で示したように useRBox などのフックをインポートして使用できます。
import { useRBox, set } from "f-box-react" function Counter() { const [count, countBox] = useRBox(0) // Create an RBox with initial value 0 const setCount = set(countBox) // Get a convenient updater function for the RBox return ( <div> <p>Count: {count}</p> <button onclick="{()"> setCount(count + 1)}>+1</button> </div> ) } export default Counter
また、RBox などの必須コンテナーを提供する f-box-core がインストールされていることを確認してください。
import { RBox } from "f-box-core" const numberBox = RBox.pack(0) // Subscribe to changes and log updates numberBox.subscribe((newValue) => { console.log(`Updated numberBox: ${newValue}`) }) // Change the value, which notifies subscribers reactively numberBox.setValue((prev) => prev + 1) // Updated numberBox: 1 numberBox.setValue((prev) => prev + 10) // Updated numberBox: 11
このセットアップにより、F-Box React を使用して状態を管理できるようになります。
結論
F-Box React を使用すると、React での状態管理が大幅に簡素化されます。
useState のような直感的
useRBox に初期値を渡すだけで、すぐに使用を開始できます。RBox は React の外部で動作します
Reactに依存しないため、サーバーサイドや他の環境でもご利用いただけます。簡単な状態共有
グローバル RBox を定義し、必要なときに useRBox を使用して、複数のコンポーネント間で状態を共有します。これにより、useContext または Redux を使用した複雑な設定が不要になります。
より簡単に状態を管理する方法をお探しの場合は、F-Box React を試してみてください!
- npm
- GitHub
ここでは F-Box React の基本的な使い方と便利さを紹介しましたが、F-Box にはさらに多くの機能があります。非同期操作、エラー処理、より複雑なシナリオを処理できます。
詳細については、F-Box のドキュメントを参照してください。
F-Box React によって、React と TypeScript の開発がより楽しく、より簡単になることを願っています!
以上がReact での状態管理の簡素化: F-Box React の概要の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
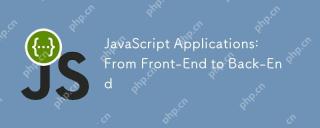
JavaScriptは、フロントエンドおよびバックエンド開発に使用できます。フロントエンドは、DOM操作を介してユーザーエクスペリエンスを強化し、バックエンドはnode.jsを介してサーバータスクを処理することを処理します。 1.フロントエンドの例:Webページテキストのコンテンツを変更します。 2。バックエンドの例:node.jsサーバーを作成します。
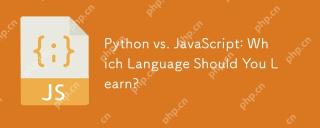
PythonまたはJavaScriptの選択は、キャリア開発、学習曲線、エコシステムに基づいている必要があります。1)キャリア開発:Pythonはデータサイエンスとバックエンド開発に適していますが、JavaScriptはフロントエンドおよびフルスタック開発に適しています。 2)学習曲線:Python構文は簡潔で初心者に適しています。 JavaScriptの構文は柔軟です。 3)エコシステム:Pythonには豊富な科学コンピューティングライブラリがあり、JavaScriptには強力なフロントエンドフレームワークがあります。
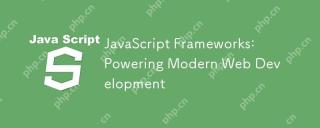
JavaScriptフレームワークのパワーは、開発を簡素化し、ユーザーエクスペリエンスとアプリケーションのパフォーマンスを向上させることにあります。フレームワークを選択するときは、次のことを検討してください。1。プロジェクトのサイズと複雑さ、2。チームエクスペリエンス、3。エコシステムとコミュニティサポート。
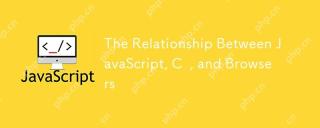
はじめに私はあなたがそれを奇妙に思うかもしれないことを知っています、JavaScript、C、およびブラウザは正確に何をしなければなりませんか?彼らは無関係であるように見えますが、実際、彼らは現代のウェブ開発において非常に重要な役割を果たしています。今日は、これら3つの間の密接なつながりについて説明します。この記事を通して、JavaScriptがブラウザでどのように実行されるか、ブラウザエンジンでのCの役割、およびそれらが協力してWebページのレンダリングと相互作用を駆動する方法を学びます。私たちは皆、JavaScriptとブラウザの関係を知っています。 JavaScriptは、フロントエンド開発のコア言語です。ブラウザで直接実行され、Webページが鮮明で興味深いものになります。なぜJavascrを疑問に思ったことがありますか
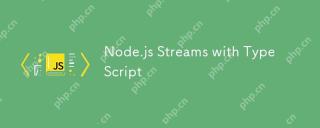
node.jsは、主にストリームのおかげで、効率的なI/Oで優れています。 ストリームはデータを段階的に処理し、メモリの過負荷を回避します。大きなファイル、ネットワークタスク、リアルタイムアプリケーションの場合。ストリームとTypeScriptのタイプの安全性を組み合わせることで、パワーが作成されます
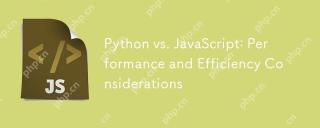
PythonとJavaScriptのパフォーマンスと効率の違いは、主に以下に反映されています。1)解釈された言語として、Pythonはゆっくりと実行されますが、開発効率が高く、迅速なプロトタイプ開発に適しています。 2)JavaScriptはブラウザ内の単一のスレッドに限定されていますが、マルチスレッドおよび非同期I/Oを使用してnode.jsのパフォーマンスを改善でき、両方とも実際のプロジェクトで利点があります。
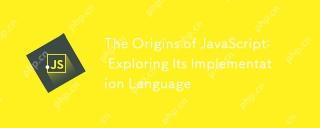
JavaScriptは1995年に発信され、Brandon Ikeによって作成され、言語をCに実現しました。 2。JavaScriptのメモリ管理とパフォーマンスの最適化は、C言語に依存しています。 3. C言語のクロスプラットフォーム機能は、さまざまなオペレーティングシステムでJavaScriptを効率的に実行するのに役立ちます。
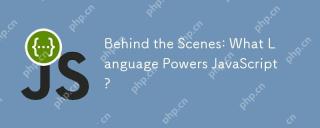
JavaScriptはブラウザとnode.js環境で実行され、JavaScriptエンジンに依存してコードを解析および実行します。 1)解析段階で抽象的構文ツリー(AST)を生成します。 2)ASTをコンパイル段階のバイトコードまたはマシンコードに変換します。 3)実行段階でコンパイルされたコードを実行します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
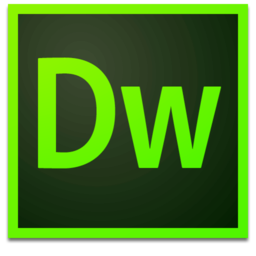
Dreamweaver Mac版
ビジュアル Web 開発ツール
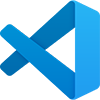
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター
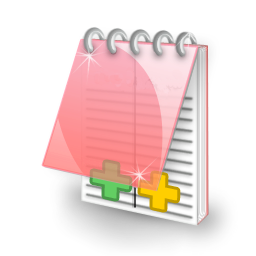
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター
