Executor および ExecutorService API は、スレッドの実行を管理および制御するための重要なツールです。これらは java.util.concurrent パッケージの一部です。スレッドの作成、管理、同期の複雑さを抽象化することで、同時プログラミングのプロセスを簡素化します。
Executors は、java.util.concurrent パッケージのユーティリティ クラスで、さまざまな種類の ExecutorService インスタンスを作成および管理するためのファクトリ メソッドを提供します。これにより、スレッド プールの作成プロセスが簡素化され、さまざまな構成でエグゼキューター インスタンスを簡単に作成および管理できるようになります。
Executor API Java 1.5 以降で利用できるインターフェースです。 execute(実行可能なコマンド)メソッドを提供します。 これは基本インターフェイスであり、ExecutorService はこのインターフェイスを拡張します。指定されたコマンドは、将来、新しいスレッド、スレッド プールのスレッド、または同じスレッドによって実行され、void を返しません。
ExecutorService API Java 1.5 以降で利用できるインターフェースです。並行プログラミングでタスクの実行を制御するための複数の方法を提供します。実行可能なタスクと呼び出し可能なタスクの両方をサポートします。タスクのステータスとして Future を返します。以下に、最もよく使用される方法を示します。
submit() は、Callable タスクまたは Runnable タスクを受け入れ、Future 型の結果を返します。
invokeAny() は、実行するタスクのコレクションを受け取り、任意の 1 つのタスクが正常に実行された結果を返します。
invokeAll() は、実行するタスクのコレクションを受け取り、Future オブジェクト タイプのリストの形式ですべてのタスクの結果を返します。
shutdown() は、executor サービスをすぐに停止しませんが、新しいタスクを受け入れません。現在実行中のタスクがすべて終了すると、executor サービスがシャットダウンされます。
shutdownNow() は、Executor サービスを直ちに停止しようとしますが、実行中のすべてのタスクが同時に停止されることは保証されません。
awaitTermination(long timeout, TimeUnit単位) は、すべてのタスクが完了するか、タイムアウトが発生するか、現在のスレッドが中断されるか、いずれか先に起こるまでブロック/待機します。現在のスレッドはブロックされます。
ExecutorService の種類
- FixedThreadPool 指定された数のスレッドで固定サイズのスレッド プールを作成します。送信されたタスクは同時に実行されます。タスクがない場合、スレッドはタスクが到着するまでアイドル状態になります。スレッドがビジーの場合、タスクはキューに追加されます。
ExecutorService fixedThreadPool = Executors.newScheduledThreadPool(5); Future<string> submit = fixedThreadPool.submit(() -> { System.out.println("Task executed by " + Thread.currentThread().getName()); return Thread.currentThread().getName(); }); fixedThreadPool.shutdown(); </string>
- CachedThreadPool スレッドのプールを作成し、ワークロードに基づいてプールに必要なスレッドの数を自動的に調整します。スレッドが 60 秒以上アイドル状態の場合、スレッドは終了します。これは動的負荷に対してうまく機能します。スレッドはアイドル タイムアウト後に強制終了されるため、ここではリソースがより有効に活用されます。
ExecutorService fixedThreadPool = Executors.newCachedThreadPool(); Future<string> submit = fixedThreadPool.submit(() -> { System.out.println("Task executed by " + Thread.currentThread().getName()); return Thread.currentThread().getName(); }); fixedThreadPool.shutdown(); </string>
- SingleThreadExecutor 単一のスレッドを作成し、タスクを順番に実行します。ここでは並列処理は行われません。
ExecutorService fixedThreadPool = Executors.newSingleThreadExecutor(); Future<string> submit = fixedThreadPool.submit(() -> { System.out.println("Task executed by " + Thread.currentThread().getName()); return Thread.currentThread().getName(); }); fixedThreadPool.shutdown() </string>
- ScheduledThreadPool/ScheduledExecutor タスクを定期的な間隔で実行したり、一定の遅延後に実行したりする機能を持つスレッドまたは trhead プールを作成します。
ScheduledExecutorService scheduler = Executors.newSingleThreadScheduledExecutor(); // Single-threaded scheduler ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(5); // Multi-threaded scheduler
scheduler.schedule(task, 10, TimeUnit.SECONDS); // Schedule task to run after 10 seconds. scheduler.scheduleAtFixedRate(task, 5, 10, TimeUnit.SECONDS); //It schedules a task to run every 10 seconds with an initial delay of 5 seconds. scheduler.scheduleWithFixedDelay(task, 5, 10, TimeUnit.SECONDS); //It schedules a task to run with a fixed delay of 10 seconds between the end of one execution and the start of the next, with an initial delay of 5 seconds. scheduler.schedule(() -> scheduler.shutdown(), 20, TimeUnit.SECONDS); //It schedules a shutdown of the scheduler after 20 seconds to stop the example.
ExecutorService へのタスクの送信
タスクは、execute() メソッドと submit() メソッドを使用して ExecutorService に送信できます。 execute() メソッドは実行可能なタスクに使用されますが、submit() は実行可能なタスクと呼び出し可能なタスクの両方を処理できます。
executor.execute(new RunnableTask()); //fire-and-forgot executor.submit(new CallableTask()); //returns the status of task
ExecutorService をシャットダウンしています
ExecutorService をシャットダウンしてリソースを解放することが重要です。これは、shutdown() メソッドと shutdownNow() メソッドを使用して実行できます。
executor.shutdown(); // Initiates an orderly shutdown" executor.shutdownNow(); // Attempts to stop all actively executing tasks. executor.awaitTermination(long timeout, TimeUnit unit); //blocks the thread until all tasks are completed or timeout occurs or current thread is interrupted, whichever happens first. Returns `true `is tasks completed, otherwise `false`.
シャットダウンへの推奨アプローチ
executor.shutdown(); try { // Wait for tasks to complete or timeout if (!executor.awaitTermination(120, TimeUnit.SECONDS)) { // If the timeout occurs, force shutdown executor.shutdownNow(); } } catch (InterruptedException ex) { executor.shutdownNow(); Thread.currentThread().interrupt(); }
Runnable について
- Runnable はインターフェイスであり、スレッドによって実行できるタスクを表します。
- Threads または Executor サービスを使用して、Runnable タスクを実行できます。
- Runnable には run() メソッドがあり、データを返しません。
- チェック例外をスローできません。
Callable について
- 1.5で導入されました
- call() メソッドがあり、型 V を返します。
- これには throws Exception が含まれており、チェックされた例外をスローできます。
今後について
- タスクの将来の結果を表します。
- リクエストの処理が完了した後、結果は最終的に Future に表示されます。
- boolean isDone() リクエスト処理のステータスを返します。完了した場合は true、それ以外の場合は false。
- boolean cancel(boolean MayInterruptIfRunning) 送信されたタスクをキャンセルします。 MayInterruptIfRunning を false として渡すと、すでに開始されたタスクはキャンセルされません。
- boolean isCancelled() はタスクがキャンセルされたかどうかを返します。
- V get() はタスクの結果を返します。タスクが完了していない場合はスレッドをブロックします。
- V get(long timeout, TimeUnit Unit) 必要に応じて、計算が完了するまで最大でも指定された時間待機し、その結果を取得します。計算が完了していない場合、指定された時間が経過すると TimeoutException がスローされます。
コーディングと学習を楽しんでください !!!
ご質問がございましたら、コメントしてください。
以上がJava の Executor サービスの概要の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
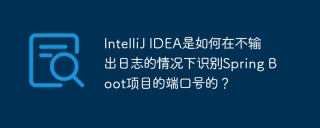
intellijideaultimatiateバージョンを使用してスプリングを開始します...
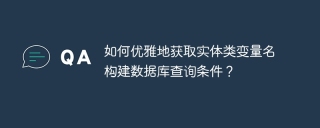
データベース操作にMyBatis-Plusまたはその他のORMフレームワークを使用する場合、エンティティクラスの属性名に基づいてクエリ条件を構築する必要があることがよくあります。あなたが毎回手動で...
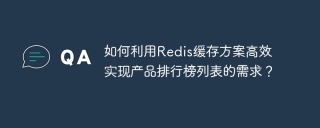
Redisキャッシュソリューションは、製品ランキングリストの要件をどのように実現しますか?開発プロセス中に、多くの場合、ランキングの要件に対処する必要があります。
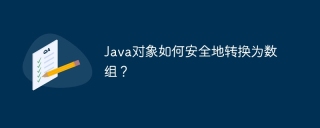
Javaオブジェクトと配列の変換:リスクの詳細な議論と鋳造タイプ変換の正しい方法多くのJava初心者は、オブジェクトのアレイへの変換に遭遇します...
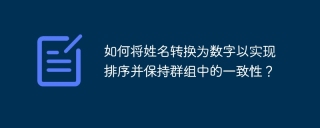
多くのアプリケーションシナリオでソートを実装するために名前を数値に変換するソリューションでは、ユーザーはグループ、特に1つでソートする必要がある場合があります...
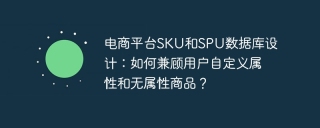
eコマースプラットフォーム上のSKUおよびSPUテーブルの設計の詳細な説明この記事では、eコマースプラットフォームでのSKUとSPUのデータベース設計の問題、特にユーザー定義の販売を扱う方法について説明します。
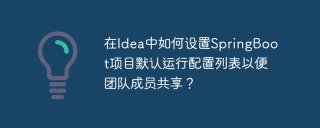
Intellijを使用して、Springboot Projectを設定する方法Default run configurationリスト...


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。
