リアクトロック
React-Rock は、React アプリケーションのグローバル状態を管理するための軽量パッケージです。ストアに行とメタデータを提供することでデータの処理を簡素化し、CRUD 操作などを実行するメソッドを提供します。 React コンポーネントと簡単に統合できるため、大規模なアプリケーションの複雑な状態を管理するための理想的なソリューションとなります。
インストール
React-Rock パッケージをインストールするには、プロジェクトで次のコマンドを実行します。
npm install react-rock
特徴
- グローバル ストア管理: グローバル ストア内の行とメタデータを管理します。
- CRUD 操作: 行に対して作成、読み取り、更新、削除の操作を実行します。
- メタ管理: メタデータを設定、取得、削除します。
- 最適化された再レンダリング: フリーズ オプションを使用してコンポーネントの再レンダリングを制御します。
- クラス コンポーネントのサポート: ストア データをクラス コンポーネントに統合するには StoreComponent を使用します。
基本的な例: ストアの作成とレコードの追加
新しいストアを作成してレコードを追加するには、createStore 関数を使用します。以下に例を示します:
import { createStore } from 'react-rock'; // Define RowType and MetaType type RowType = { name: string, age: number }; type MetaType = { totalRecords: number }; // Create a store const users = createStore<rowtype metatype>({ name: '', age: 0 }, { totalRecords: 0 }); // Add a new row to the store users.create({ name: 'John Doe', age: 30 }); </rowtype>
RowType の説明
行が作成されると、次のプロパティが含まれます:
type RowType<row> = Row & { _id: string; // Unique identifier for the row _index: number; // Index of the row in the store _observe: number; // Internal property to track changes } </row>
各行には、元のデータ (行) と、_id、_index、_observe などの追加のプロパティが含まれます。
メソッド
使用可能なすべてのメソッドとその説明をまとめた表を以下に示します。
Method | Description |
---|---|
create(row, freeze?) | Adds a new record to the store. Optionally, prevents re-rendering if freeze is true. |
createMany(rows, freeze?) | Adds multiple records to the store. Optionally, prevents re-rendering if freeze is true. |
update(row, where, freeze?) | Updates records based on the condition specified in where. |
updateAll(row, freeze?) | Updates all records in the store. Optionally, prevents re-rendering if freeze is true. |
delete(where, freeze?) | Deletes records based on the condition specified in where. |
move(oldIdx, newIdx, freeze?) | Moves a record from one index to another. |
clearAll(freeze?) | Clears all records from the store. Optionally, prevents re-rendering if freeze is true. |
getAll(args?) | Retrieves all rows from the store. |
find(where, args?) | Finds rows based on a condition specified in where. |
findFirst(where, freeze?) | Finds the first row that matches the condition in where. |
findById(_id, freeze?) | Finds a row by its _id. |
setMeta(key, value, freeze?) | Sets a value for a specific meta key. |
getMeta(key, freeze?) | Retrieves the value of a specific meta key. |
getAllMeta(freeze?) | Retrieves all meta data from the store. |
deleteMeta(key, freeze?) | Deletes a specific meta key. |
clearMeta(freeze?) | Clears all meta data from the store. |
findメソッドの例
find メソッドを使用すると、特定の条件に基づいてストア内の行を検索できます。
npm install react-rock
React コンポーネントでの再レンダリング
React-Rock はフリーズ メカニズムを提供することで再レンダリングを最適化します。ストアの更新が発生し、フリーズ オプションが有効になっている場合、find や findFirst などのメソッドを使用してストアにアクセスする React コンポーネントは自動的に再レンダリングされません。これにより、コンポーネントをいつ再レンダリングするかを制御できるようになり、大規模なアプリケーションのパフォーマンスが向上します。
WhereType
WhereType は、行をクエリするときに条件を指定するために使用されます。行をフィルタリングするためのクエリ構造を定義します。
クエリ値の種類
QueryValueType は、クエリの可能な条件を定義するために WhereType 内で使用されます。
Property | Description |
---|---|
contain | Finds values containing the specified string, number, or boolean. |
startWith | Finds values that start with the specified string or number. |
endWith | Finds values that end with the specified string or number. |
equalWith | Finds values that are exactly equal to the specified value. |
notEqualWith | Finds values that are not equal to the specified value. |
gt | Finds values greater than the specified number. |
lt | Finds values less than the specified number. |
gte | Finds values greater than or equal to the specified number. |
lte | Finds values less than or equal to the specified number. |
WhereType の例
import { createStore } from 'react-rock'; // Define RowType and MetaType type RowType = { name: string, age: number }; type MetaType = { totalRecords: number }; // Create a store const users = createStore<rowtype metatype>({ name: '', age: 0 }, { totalRecords: 0 }); // Add a new row to the store users.create({ name: 'John Doe', age: 30 }); </rowtype>
引数の種類
ArgsType は、特定の行の選択や行のスキップなど、クエリの動作をカスタマイズするためのオプションを定義します。
Property | Description |
---|---|
getRow | Custom function to process rows before returning them. |
skip | Number of rows to skip. |
take | Number of rows to return. |
freeze | If true, prevents re-rendering when accessing the data. |
クラスコンポーネントを使用した例
クラスコンポーネントでストアを使用するには、StoreComponent クラスを拡張します。
npm install react-rock
CRUDの例
import { createStore } from 'react-rock'; // Define RowType and MetaType type RowType = { name: string, age: number }; type MetaType = { totalRecords: number }; // Create a store const users = createStore<rowtype metatype>({ name: '', age: 0 }, { totalRecords: 0 }); // Add a new row to the store users.create({ name: 'John Doe', age: 30 }); </rowtype>
find と Query の例
type RowType<row> = Row & { _id: string; // Unique identifier for the row _index: number; // Index of the row in the store _observe: number; // Internal property to track changes } </row>
複数のコンポーネントでのストアの使用例
React-Rock を使用すると、複数のコンポーネント間で同じストアを共有し、アプリ全体で一貫した状態を確保できます。
const foundUsers = users.find({ name: { equalWith: 'John Doe' } }); console.log(foundUsers);
種類の説明
- RowType: _id、_index、および _observe とユーザー定義のデータ フィールドを持つレコードを表します。
- ArgsType: スキップ、取得、カスタム行処理などの柔軟な行クエリのオプションを定義します。
- WhereType: contains、equalWith などのフィールド、および gt、lt などの範囲クエリを使用して、レコードをクエリするための条件を表します。
- QueryValueType: フィールド値に基づいて行をフィルター処理するために許可される条件タイプを指定します。
ライセンス
このパッケージは MIT ライセンスに基づいてライセンスされています。
このドキュメントでは、react-rock パッケージを効果的に使用する方法の簡潔な概要を提供します。
?貢献する
貢献は大歓迎です!投稿ガイドラインをご確認ください。
?ライセンス
このプロジェクトは MIT ライセンスに基づいてライセンスされています。
以上がリアクトロックの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
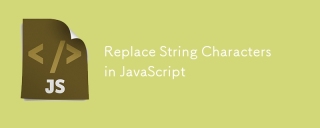
JavaScript文字列置換法とFAQの詳細な説明 この記事では、javaScriptの文字列文字を置き換える2つの方法について説明します:内部JavaScriptコードとWebページの内部HTML。 JavaScriptコード内の文字列を交換します 最も直接的な方法は、置換()メソッドを使用することです。 str = str.replace( "find"、 "置換"); この方法は、最初の一致のみを置き換えます。すべての一致を置き換えるには、正規表現を使用して、グローバルフラグGを追加します。 str = str.replace(/fi
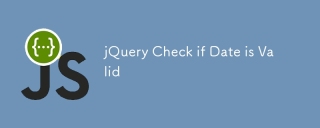
単純なJavaScript関数は、日付が有効かどうかを確認するために使用されます。 関数isvaliddate(s){ var bits = s.split( '/'); var d = new Date(bits [2] '/' bits [1] '/'ビット[0]); return !!(d &&(d.getmonth()1)== bits [1] && d.getdate()== number(bits [0])); } //テスト var
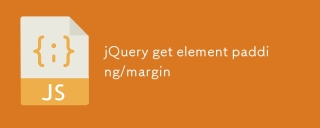
この記事では、jQueryを使用して、DOM要素の内側のマージン値とマージン値、特に外側の縁と要素の内側の縁の特定の位置を取得して設定する方法について説明します。 CSSを使用して要素の内側と外側の縁を設定することは可能ですが、正確な値を取得するのは難しい場合があります。 // 設定 $( "div.header")。css( "margin"、 "10px"); $( "div.header")。css( "padding"、 "10px"); このコードはそうだと思うかもしれません
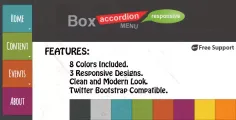
この記事では、10個の例外的なjQueryタブとアコーディオンについて説明します。 タブとアコーディオンの重要な違いは、コンテンツパネルの表示方法と非表示にあります。これらの10の例を掘り下げましょう。 関連記事:10 jQueryタブプラグイン
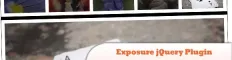
ウェブサイトのダイナミズムと視覚的な魅力を高めるために、10の例外的なjQueryプラグインを発見してください!このキュレーションされたコレクションは、画像アニメーションからインタラクティブなギャラリーまで、多様な機能を提供します。これらの強力なツールを探りましょう。 関連投稿: 1
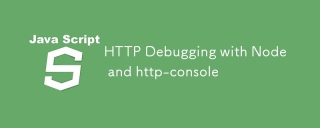
HTTP-Consoleは、HTTPコマンドを実行するためのコマンドラインインターフェイスを提供するノードモジュールです。 Webサーバー、Web Servに対して作成されているかどうかに関係なく、HTTPリクエストで何が起こっているかをデバッグして正確に確認するのに最適です
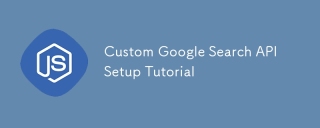
このチュートリアルでは、カスタムGoogle検索APIをブログまたはWebサイトに統合する方法を示し、標準のWordPressテーマ検索関数よりも洗練された検索エクスペリエンスを提供します。 驚くほど簡単です!検索をyに制限することができます
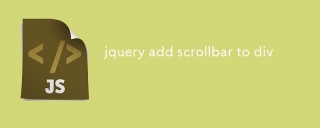
次のjQueryコードスニペットを使用して、Divコンテンツがコンテナ要素領域を超えたときにスクロールバーを追加できます。 (デモンストレーションはありません、それを直接firebugにコピーしてください) // d =ドキュメント // w =ウィンドウ // $ = jQuery var contentarea = $(this)、 wintop = contentarea.scrolltop()、 docheight = $(d).height()、 winheight = $(w).height()、 divheight = $( '#c


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

SublimeText3 中国語版
中国語版、とても使いやすい
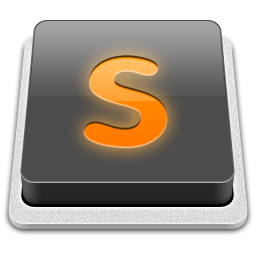
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。
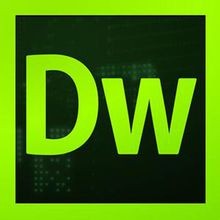
ドリームウィーバー CS6
ビジュアル Web 開発ツール

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
