Zkapps (ゼロ知識アプリケーション) は、ゼロ知識証明、具体的には zk-Snarks [ゼロ知識の簡潔な非対話型知識引数] を利用した mina プロトコル スマート コントラクトです。zkapps は snapps [スマート非対話型知識引数] に置き換えられました。アプリケーション]。 ZkApp スマート コントラクトは、o1js (TypeScript ライブラリ) を使用して作成されます。 zkApps はユーザーの Web ブラウザでクライアント側を実行し、小さな有効性証明のみを公開し、その後、Mina ノードによって検証されます。 Zkapp はスマート コントラクトと UI で構成されており、これについては次のセクションで詳しく説明します。
応用
個人データを介さずにユーザーの年齢を検証する年齢認証に関する zkapp を作成しました。
zkapp-cli npm パッケージのインストールに進み、zk 証明ビルド プロセスの一部として証明者機能と検証者機能を続行するためのテンプレートを実際に作成しました
実装
以下は検証カスタムロジックを追加する実装です。これは、プルーフ生成中に使用される zk-SNARK の回路ロジックを定義します。実際の証明者関数は o1js ライブラリによって管理され、zkApp メソッドがプライベート入力を使用してオフチェーンで実行されるときに呼び出されます。
import { Field, SmartContract, state, State, method } from 'o1js'; /** * Private Age Verification Contract * The contract will verify if the user's age is greater than or equal to the threshold age. * The contract uses zero-knowledge proofs to keep the user's age private. */ export class AgeVerification extends SmartContract { // State variable to store the verification result (valid or invalid) @state(Field) valid = State<field>(); // Method to initialize the state init() { super.init(); this.valid.set(Field(0)); // Default is invalid } // Method to verify the age @method async verifyAge(age: Field, threshold: Field) { // Compute age - threshold const difference = age.sub(threshold); // Use circuit-compatible logic to check if the difference is non-negative const isValid = difference.equals(Field(0)).or(difference.greaterThanOrEqual(Field(0))) ? Field(1) : Field(0); // Set the validity of the verification result this.valid.set(isValid); } } </field>
以下のスクリプトは、AgeVerification zkApp と対話するテスト スイートです。 txn.prove() 中に証明者ロジックを呼び出し、更新された状態をチェックすることで zkApp の動作を検証します。
実際の証明者関数は基礎となる zkApp メソッド (verifyAge) にあり、txn.prove() はテスト中に証明を生成するメカニズムです。
入力をテストするために、テスト スクリプトを次のように編集しました。
import { AccountUpdate, Field, Mina, PrivateKey, PublicKey } from 'o1js'; import { AgeVerification } from './AgeVerification'; // Import the correct contract let proofsEnabled = false; describe('AgeVerification', () => { let deployerAccount: Mina.TestPublicKey, deployerKey: PrivateKey, senderAccount: Mina.TestPublicKey, senderKey: PrivateKey, zkAppAddress: PublicKey, zkAppPrivateKey: PrivateKey, zkApp: AgeVerification; // Update to use AgeVerification instead of Add beforeAll(async () => { if (proofsEnabled) await AgeVerification.compile(); // Update compile for AgeVerification }); beforeEach(async () => { const Local = await Mina.LocalBlockchain({ proofsEnabled }); Mina.setActiveInstance(Local); [deployerAccount, senderAccount] = Local.testAccounts; let feePayer = Local.testAccounts[0].key; deployerKey = deployerAccount.key; senderKey = senderAccount.key; zkAppPrivateKey = PrivateKey.random(); zkAppAddress = zkAppPrivateKey.toPublicKey(); zkApp = new AgeVerification(zkAppAddress); // Instantiate AgeVerification contract }); async function localDeploy() { const txn = await Mina.transaction(deployerAccount, async () => { AccountUpdate.fundNewAccount(deployerAccount); await zkApp.deploy(); }); await txn.prove(); // this tx needs .sign(), because `deploy()` adds an account update that requires signature authorization await txn.sign([deployerKey, zkAppPrivateKey]).send(); } it('generates and deploys the `AgeVerification` smart contract', async () => { await localDeploy(); const valid = zkApp.valid.get(); // Access the 'valid' state variable expect(valid).toEqual(Field(0)); // Initially, the contract should set 'valid' to Field(0) }); it('correctly verifies the age in the `AgeVerification` smart contract', async () => { await localDeploy(); const age = Field(25); // Example age value const threshold = Field(18); // Example threshold value // Call the verifyAge method const txn = await Mina.transaction(senderAccount, async () => { await zkApp.verifyAge(age, threshold); // Use the verifyAge method }); await txn.prove(); await txn.sign([senderKey]).send(); const valid = zkApp.valid.get(); // Check the validity state after verification expect(valid).toEqual(Field(1)); // Expected to be valid if age >= threshold }); });
以下はテスト結果です
interact.ts ファイルに証明者メカニズムを追加しました。これは基本的に zk-SNARK 証明を生成し、mina ブロックチェーンでのトランザクションに移行するときに証明を送信します。 interact.ts スクリプトが証明を生成する間、検証はトランザクションの処理時に Min ブロックチェーンによって実行されます。これは、証明者が検証者 (Mina ネットワーク) がチェックする証明を生成するという zk-SNARK システムの重要な側面です。
import fs from 'fs/promises'; import { Mina, NetworkId, PrivateKey, Field } from 'o1js'; import { AgeVerification } from './AgeVerification'; // check command line arg let deployAlias = process.argv[2]; if (!deployAlias) throw Error(`Missing <deployalias> argument. Usage: node build/src/interact.js <deployalias> `); Error.stackTraceLimit = 1000; const DEFAULT_NETWORK_ID = 'testnet'; // parse config and private key from file type Config = { deployAliases: Record; }; let configJson: Config = JSON.parse(await fs.readFile('config.json', 'utf8')); let config = configJson.deployAliases[deployAlias]; let feepayerKeysBase58: { privateKey: string; publicKey: string } = JSON.parse( await fs.readFile(config.feepayerKeyPath, 'utf8') ); let zkAppKeysBase58: { privateKey: string; publicKey: string } = JSON.parse( await fs.readFile(config.keyPath, 'utf8') ); let feepayerKey = PrivateKey.fromBase58(feepayerKeysBase58.privateKey); let zkAppKey = PrivateKey.fromBase58(zkAppKeysBase58.privateKey); // set up Mina instance and contract we interact with const Network = Mina.Network({ // We need to default to the testnet networkId if none is specified for this deploy alias in config.json // This is to ensure the backward compatibility. networkId: (config.networkId ?? DEFAULT_NETWORK_ID) as NetworkId, mina: config.url, }); const fee = Number(config.fee) * 1e9; // in nanomina (1 billion = 1.0 mina) Mina.setActiveInstance(Network); let feepayerAddress = feepayerKey.toPublicKey(); let zkAppAddress = zkAppKey.toPublicKey(); let zkApp = new AgeVerification(zkAppAddress); let age = Field(25); // Example age let threshold = Field(18); // Example threshold age // compile the contract to create prover keys console.log('compile the contract...'); await AgeVerification.compile(); try { // call verifyAge() and send transaction console.log('build transaction and create proof...'); let tx = await Mina.transaction( { sender: feepayerAddress, fee }, async () => { await zkApp.verifyAge(age, threshold); // Replacing update() with verifyAge } ); await tx.prove(); console.log('send transaction...'); const sentTx = await tx.sign([feepayerKey]).send(); if (sentTx.status === 'pending') { console.log( '\nSuccess! Age verification transaction sent.\n' + '\nYour smart contract state will be updated' + `\nas soon as the transaction is included in a block:` + `\n${getTxnUrl(config.url, sentTx.hash)}` ); } } catch (err) { console.log(err); } function getTxnUrl(graphQlUrl: string, txnHash: string | undefined) { const hostName = new URL(graphQlUrl).hostname; const txnBroadcastServiceName = hostName .split('.') .filter((item) => item === 'minascan')?.[0]; const networkName = graphQlUrl .split('/') .filter((item) => item === 'mainnet' || item === 'devnet')?.[0]; if (txnBroadcastServiceName && networkName) { return `https://minascan.io/${networkName}/tx/${txnHash}?type=zk-tx`; } return `Transaction hash: ${txnHash}`; } </deployalias></deployalias>
年齢としきい値として 25 歳と 18 歳を入力しました。
npm run test を実行することでテストが正常に完了したため。 zk config
を使用して devnet へのデプロイを進めました。ここで次の入力を提供しました:
エイリアスのデプロイ: test
ネットワークの種類: テストネット
URL: https://api.minascan.io/node/devnet/v1/graphql
料金支払者: 新しい料金支払者キー
トランザクション: 0.1
URL はここから取得できます:
その後、デプロイすると次の応答が返されました。
コントラクトは次の開発ネットにデプロイされます
デプロイ後、RPC URL を指定して単純な HTML、CSS、JS を選択し、コントラクト アドレスをデプロイした UI を進めました。これが最終的な UI です。
これで、スマート コントラクトと UI を統合した後の zkapp の作成は完了です。 AgeVerification zkApp のユーザー インターフェイス (UI) を構築した後、フロントエンドとスマート コントラクトの統合により、ユーザーはゼロ知識証明システムとシームレスに対話できるようになります。 UI は、zk-SNARK を通じてプライバシーを維持しながら、ユーザーの年齢としきい値データの契約への提出を容易にします。これにより、ユーザーは実際の値を明らかにすることなく自分の年齢を確認でき、機密性が保たれます。バックエンドは証明者機能を利用して証明を生成し、Mina ブロックチェーンがそれを効率的に検証します。このエンドツーエンドのソリューションは、Mina の zk-SNARK ベースのアーキテクチャが提供するプライバシーとスケーラビリティの機能を最大限に活用しながら、安全でユーザーフレンドリーなエクスペリエンスを保証します。
以上がmina プロトコルの探索: zk アプリケーションの実用的な使用例の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
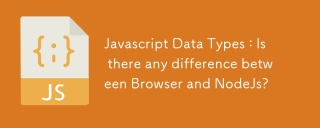
JavaScriptコアデータ型は、ブラウザとnode.jsで一貫していますが、余分なタイプとは異なる方法で処理されます。 1)グローバルオブジェクトはブラウザのウィンドウであり、node.jsのグローバルです2)バイナリデータの処理に使用されるNode.jsの一意のバッファオブジェクト。 3)パフォーマンスと時間の処理にも違いがあり、環境に従ってコードを調整する必要があります。
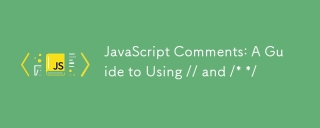
javascriptusestwotypesofcomments:シングルライン(//)およびマルチライン(//)
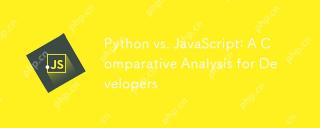
PythonとJavaScriptの主な違いは、タイプシステムとアプリケーションシナリオです。 1。Pythonは、科学的コンピューティングとデータ分析に適した動的タイプを使用します。 2。JavaScriptは弱いタイプを採用し、フロントエンドとフルスタックの開発で広く使用されています。この2つは、非同期プログラミングとパフォーマンスの最適化に独自の利点があり、選択する際にプロジェクトの要件に従って決定する必要があります。
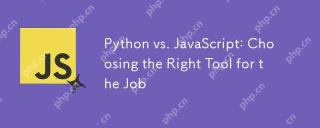
PythonまたはJavaScriptを選択するかどうかは、プロジェクトの種類によって異なります。1)データサイエンスおよび自動化タスクのPythonを選択します。 2)フロントエンドとフルスタック開発のためにJavaScriptを選択します。 Pythonは、データ処理と自動化における強力なライブラリに好まれていますが、JavaScriptはWebインタラクションとフルスタック開発の利点に不可欠です。
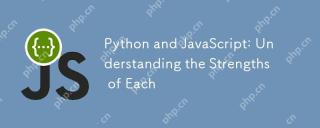
PythonとJavaScriptにはそれぞれ独自の利点があり、選択はプロジェクトのニーズと個人的な好みに依存します。 1. Pythonは、データサイエンスやバックエンド開発に適した簡潔な構文を備えた学習が簡単ですが、実行速度が遅くなっています。 2。JavaScriptはフロントエンド開発のいたるところにあり、強力な非同期プログラミング機能を備えています。 node.jsはフルスタックの開発に適していますが、構文は複雑でエラーが発生しやすい場合があります。
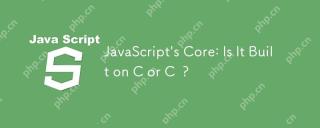
javascriptisnotbuiltoncorc;それは、解釈されていることを解釈しました。
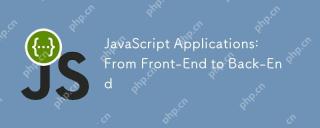
JavaScriptは、フロントエンドおよびバックエンド開発に使用できます。フロントエンドは、DOM操作を介してユーザーエクスペリエンスを強化し、バックエンドはnode.jsを介してサーバータスクを処理することを処理します。 1.フロントエンドの例:Webページテキストのコンテンツを変更します。 2。バックエンドの例:node.jsサーバーを作成します。
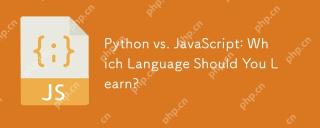
PythonまたはJavaScriptの選択は、キャリア開発、学習曲線、エコシステムに基づいている必要があります。1)キャリア開発:Pythonはデータサイエンスとバックエンド開発に適していますが、JavaScriptはフロントエンドおよびフルスタック開発に適しています。 2)学習曲線:Python構文は簡潔で初心者に適しています。 JavaScriptの構文は柔軟です。 3)エコシステム:Pythonには豊富な科学コンピューティングライブラリがあり、JavaScriptには強力なフロントエンドフレームワークがあります。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
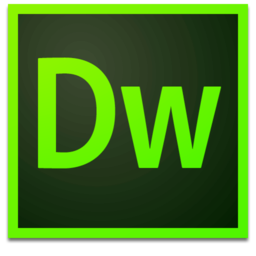
Dreamweaver Mac版
ビジュアル Web 開発ツール
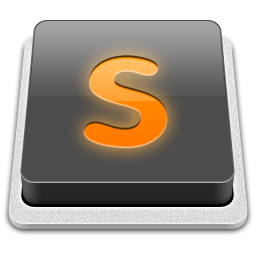
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)
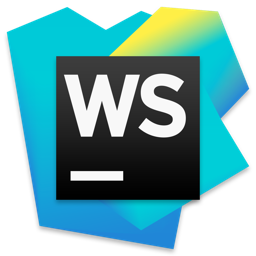
WebStorm Mac版
便利なJavaScript開発ツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
