var、let、const の違い
1. var、let、const の概要
Feature | var | let | const |
---|---|---|---|
Scope | Function-scoped | Block-scoped | Block-scoped |
Re-declaration | Allowed within the same scope | Not allowed in the same scope | Not allowed in the same scope |
Re-assignment | Allowed | Allowed | Not allowed after initialization |
Initialization | Can be declared without initialization | Can be declared without initialization | Must be initialized at the time of declaration |
Hoisting | Hoisted but initialized to undefined | Hoisted but not initialized | Hoisted but not initialized |
var
Type | Function Scope | Block Scope |
---|---|---|
var | Variables are scoped to the enclosing function. | Does not support block scope. A var inside a block (if, for, etc.) leaks into the enclosing function or global scope. |
let / const | Not function-scoped. | Variables are confined to the block they are declared in. |
再宣言
if (true) { var x = 10; let y = 20; const z = 30; } console.log(x); // 10 (accessible because of function scope) console.log(y); // ReferenceError (block-scoped) console.log(z); // ReferenceError (block-scoped)
ホイスティング
Feature | var | let | const |
---|---|---|---|
Re-declaration | Allowed | Not allowed | Not allowed |
Re-assignment | Allowed | Allowed | Not allowed |
例:
if (true) { var x = 10; let y = 20; const z = 30; } console.log(x); // 10 (accessible because of function scope) console.log(y); // ReferenceError (block-scoped) console.log(z); // ReferenceError (block-scoped)
4.巻き上げ動作
Type | Hoisting Behavior |
---|---|
var | Hoisted to the top of the scope but initialized as undefined. |
let | Hoisted but not initialized. Accessing it before declaration causes a ReferenceError. |
const | Hoisted but not initialized. Must be initialized at the time of declaration. |
巻き上げ動作
// Re-declaration var a = 10; var a = 20; // Allowed let b = 30; // let b = 40; // SyntaxError: Identifier 'b' has already been declared const c = 50; // const c = 60; // SyntaxError: Identifier 'c' has already been declared // Re-assignment a = 15; // Allowed b = 35; // Allowed // c = 55; // TypeError: Assignment to constant variable
定数
Feature | let and const |
---|---|
Block Scope | Both are confined to the block in which they are declared. |
No Hoisting Initialization | Both are hoisted but cannot be accessed before initialization. |
Better Practice | Preferred over var for predictable scoping. |
5. let と const
の類似点Scenario | Recommended Keyword |
---|---|
Re-declare variables or use function scope | var (generally avoid unless necessary for legacy code). |
Variables that may change | let (e.g., counters, flags, intermediate calculations). |
Variables that should not change | const (e.g., configuration settings, fixed values). |
7.吊り上げ説明
ホイスティングとは何ですか?
ホイスティングは、コンパイル段階で宣言をスコープの先頭に移動する JavaScript のデフォルトの動作です。
- var: ホイストされ、未定義に初期化されます。
- let / const: ホイストされていますが、初期化されていません。これにより、ブロックの開始から宣言が検出されるまで、時間的デッド ゾーン (TDZ) が作成されます。
なぜホイスティングがこのように機能するのですか?
- コンパイルフェーズ: JavaScript はまずコードをスキャンして、変数と関数の宣言のためのメモリ空間を作成します。この段階では:
- var 変数は未定義に初期化されます。
- let 変数と const 変数は「ホイスト」されますが、初期化されないままになるため、TDZ となります。
- 関数宣言は完全にホイストされています。
- 実行フェーズ: JavaScript はコードを 1 行ずつ実行し始めます。変数と関数には、このフェーズ中に値が割り当てられます。
8.吊り上げの概要
Type | Hoisting | Access Before Declaration |
---|---|---|
var | Hoisted and initialized to undefined. | Allowed but value is undefined. |
let | Hoisted but not initialized. | Causes a ReferenceError. |
const | Hoisted but not initialized. | Causes a ReferenceError. |
例:
if (true) { var x = 10; let y = 20; const z = 30; } console.log(x); // 10 (accessible because of function scope) console.log(y); // ReferenceError (block-scoped) console.log(z); // ReferenceError (block-scoped)
結論
- 再代入の必要のない変数には、可能な限り const を使用してください。
- 同じスコープ内で再割り当てする必要がある変数には let を使用します。
- 従来のコードを使用する場合、または関数スコープの動作が必要な場合を除き、var を使用しないでください。
JavaScriptのデータ型
JavaScript には、プリミティブ タイプと 非プリミティブ (参照) タイプに分類されるさまざまなデータ型があります。以下に例と違いを示しながらそれぞれを説明します。
1. プリミティブデータ型
プリミティブ型は不変です。つまり、作成後に値を変更することはできません。これらはメモリに直接保存されます。
Data Type | Example | Description |
---|---|---|
String | "hello", 'world' | Represents a sequence of characters (text). Enclosed in single (''), double (""), or backticks (). |
Number | 42, 3.14, NaN | Represents both integers and floating-point numbers. Includes NaN (Not-a-Number) and Infinity. |
BigInt | 123n, 9007199254740991n | Used for numbers larger than Number.MAX_SAFE_INTEGER (2^53 - 1). Add n to create a BigInt. |
Boolean | true, false | Represents logical values, used in conditions to represent "yes/no" or "on/off". |
Undefined | undefined | Indicates a variable has been declared but not assigned a value. |
Null | null | Represents an intentional absence of value. Often used to reset or clear a variable. |
Symbol | Symbol('id') | Represents a unique identifier, mainly used as property keys for objects to avoid collisions. |
2. 非プリミティブ (参照) データ型
非プリミティブ型は変更可能であり、参照によって保存されます。これらは、データのコレクションまたはより複雑なエンティティを保存するために使用されます。
Data Type | Example | Description |
---|---|---|
Object | {name: 'John', age: 30} | A collection of key-value pairs. Keys are strings (or Symbols), and values can be any type. |
Array | [1, 2, 3, "apple"] | A list-like ordered collection of values. Access elements via index (e.g., array[0]). |
Function | function greet() {} | A reusable block of code that can be executed. Functions are first-class citizens in JavaScript. |
Date | new Date() | Represents date and time. Provides methods for manipulating dates and times. |
RegExp | /pattern/ | Represents regular expressions used for pattern matching and string searching. |
Map | new Map() | A collection of key-value pairs where keys can be of any type, unlike plain objects. |
Set | new Set([1, 2, 3]) | A collection of unique values, preventing duplicates. |
WeakMap | new WeakMap() | Similar to Map, but keys are weakly held, meaning they can be garbage-collected. |
WeakSet | new WeakSet() | Similar to Set, but holds objects weakly to prevent memory leaks. |
3. プリミティブ型と非プリミティブ型の主な違い
Aspect | Primitive Types | Non-Primitive Types |
---|---|---|
Mutability | Immutable: Values cannot be changed. | Mutable: Values can be modified. |
Storage | Stored directly in memory. | Stored as a reference to a memory location. |
Copy Behavior | Copied by value (creates a new value). | Copied by reference (points to the same object). |
Examples | string, number, boolean, etc. | object, array, function, etc. |
4. 特殊な場合
オペレーターのタイプ
- typeof null: JavaScript の歴史的なバグにより「オブジェクト」を返しますが、null はオブジェクトではありません。
- typeof NaN: 「非数値」を意味する場合でも、「数値」を返します。
- typeof function: オブジェクトのサブタイプである「function」を返します。
ダイナミックタイピング
JavaScript では、変数が実行時にさまざまな型の値を保持できるようになります:
if (true) { var x = 10; let y = 20; const z = 30; } console.log(x); // 10 (accessible because of function scope) console.log(y); // ReferenceError (block-scoped) console.log(z); // ReferenceError (block-scoped)
5. 各データ型の例
プリミティブ型
// Re-declaration var a = 10; var a = 20; // Allowed let b = 30; // let b = 40; // SyntaxError: Identifier 'b' has already been declared const c = 50; // const c = 60; // SyntaxError: Identifier 'c' has already been declared // Re-assignment a = 15; // Allowed b = 35; // Allowed // c = 55; // TypeError: Assignment to constant variable
非プリミティブ型
console.log(a); // undefined (hoisted) var a = 10; console.log(b); // ReferenceError (temporal dead zone) let b = 20; console.log(c); // ReferenceError (temporal dead zone) const c = 30;
6. typeof結果の概要
Expression | Result |
---|---|
typeof "hello" | "string" |
typeof 42 | "number" |
typeof 123n | "bigint" |
typeof true | "boolean" |
typeof undefined | "undefined" |
typeof null | "object" |
typeof Symbol() | "symbol" |
typeof {} | "object" |
typeof [] | "object" |
typeof function(){} | "function" |
結果
以上が必要なのは Javascript のチートシートのみです。の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
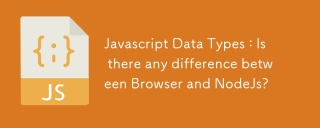
JavaScriptコアデータ型は、ブラウザとnode.jsで一貫していますが、余分なタイプとは異なる方法で処理されます。 1)グローバルオブジェクトはブラウザのウィンドウであり、node.jsのグローバルです2)バイナリデータの処理に使用されるNode.jsの一意のバッファオブジェクト。 3)パフォーマンスと時間の処理にも違いがあり、環境に従ってコードを調整する必要があります。
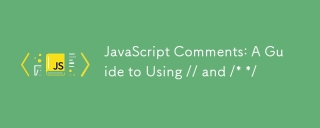
javascriptusestwotypesofcomments:シングルライン(//)およびマルチライン(//)
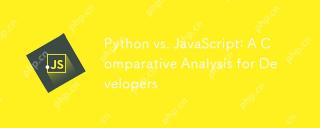
PythonとJavaScriptの主な違いは、タイプシステムとアプリケーションシナリオです。 1。Pythonは、科学的コンピューティングとデータ分析に適した動的タイプを使用します。 2。JavaScriptは弱いタイプを採用し、フロントエンドとフルスタックの開発で広く使用されています。この2つは、非同期プログラミングとパフォーマンスの最適化に独自の利点があり、選択する際にプロジェクトの要件に従って決定する必要があります。
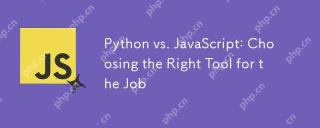
PythonまたはJavaScriptを選択するかどうかは、プロジェクトの種類によって異なります。1)データサイエンスおよび自動化タスクのPythonを選択します。 2)フロントエンドとフルスタック開発のためにJavaScriptを選択します。 Pythonは、データ処理と自動化における強力なライブラリに好まれていますが、JavaScriptはWebインタラクションとフルスタック開発の利点に不可欠です。
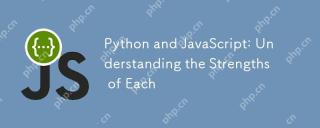
PythonとJavaScriptにはそれぞれ独自の利点があり、選択はプロジェクトのニーズと個人的な好みに依存します。 1. Pythonは、データサイエンスやバックエンド開発に適した簡潔な構文を備えた学習が簡単ですが、実行速度が遅くなっています。 2。JavaScriptはフロントエンド開発のいたるところにあり、強力な非同期プログラミング機能を備えています。 node.jsはフルスタックの開発に適していますが、構文は複雑でエラーが発生しやすい場合があります。
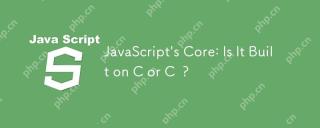
javascriptisnotbuiltoncorc;それは、解釈されていることを解釈しました。
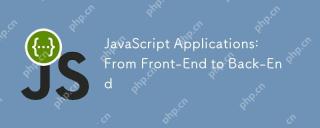
JavaScriptは、フロントエンドおよびバックエンド開発に使用できます。フロントエンドは、DOM操作を介してユーザーエクスペリエンスを強化し、バックエンドはnode.jsを介してサーバータスクを処理することを処理します。 1.フロントエンドの例:Webページテキストのコンテンツを変更します。 2。バックエンドの例:node.jsサーバーを作成します。
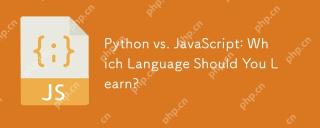
PythonまたはJavaScriptの選択は、キャリア開発、学習曲線、エコシステムに基づいている必要があります。1)キャリア開発:Pythonはデータサイエンスとバックエンド開発に適していますが、JavaScriptはフロントエンドおよびフルスタック開発に適しています。 2)学習曲線:Python構文は簡潔で初心者に適しています。 JavaScriptの構文は柔軟です。 3)エコシステム:Pythonには豊富な科学コンピューティングライブラリがあり、JavaScriptには強力なフロントエンドフレームワークがあります。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
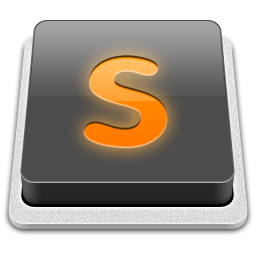
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
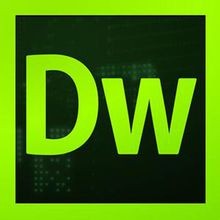
ドリームウィーバー CS6
ビジュアル Web 開発ツール
