こんにちは。Chroma DB は、GenAI アプリケーションの操作に役立つベクトル データベースです。この記事では、MySQL の同様の関係を調べることで、Chroma DB でクエリを実行する方法を検討します。
スキーマ
SQL とは異なり、独自のスキーマを定義することはできません。 Chroma では、それぞれ独自の目的を持つ固定列が得られます:
import chromadb #setiing up the client client = chromadb.Client() collection = client.create_collection(name="name") collection.add( documents = ["str1","str2","str3",...] ids = [1,2,3,....] metadatas=[{"chapter": "3", "verse": "16"},{"chapter":"3", "verse":"5"}, ..] embeddings = [[1,2,3], [3,4,5], [5,6,7]] )
ID: 一意の ID です。 SQL とは異なり、自動インクリメントがないので、自分で指定する必要があることに注意してください
Documents: 埋め込みの生成に使用されるテキスト データを挿入するために使用されます。テキストを指定すると、埋め込みが自動的に作成されます。または、埋め込みを直接指定してテキストを別の場所に保存することもできます。
埋め込み: これらは類似性検索を実行するために使用されるため、私の意見では、データベースの最も重要な部分です。
メタデータ: これは、追加のコンテキストのためにデータベースに追加する可能性のある追加データを関連付けるために使用されます。
コレクションの基本が明確になったので、CRUD 操作に進み、データベースにクエリを実行する方法を見てみましょう。
CRUD操作
注: コレクションは Chroma のテーブルのようなものです
コレクションを作成するには、create_collection() を使用し、必要に応じて操作を実行できますが、コレクションがすでに作成されており、再度参照する必要がある場合は、get_collection() を使用する必要があります。そうしないと、エラーが発生します。
Create Table tablename
#Create a collection collection = client.create_collection(name="name") #If a collection is already made and you need to use it again the use collection = client.get_collection(name="name")
Insert into tablename Values(... , ..., ...)
collection.add( ids = [1] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] )
挿入されたデータを更新するか、データを削除するには、次のコマンドを使用できます
collection.update( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # If the id does not exist update will do nothing. to add data if id does not exist use collection.upsert( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # To delete data use delete and refrence the document or id or the feild collection.delete( documents = ["some text"] ) # Or you can delete from a bunch of ids using where that will apply filter on metadata collection.delete( ids=["id1", "id2", "id3",...], where={"chapter": "20"} )
クエリ
次に、特定のクエリがどのように見えるかを見ていきます
Select * from tablename Select * from tablename limit value Select Documents, Metadata from tablename
collection.get() collection.get(limit = val) collection.get(include = ["documents","metadata"])
get() は、より高度なクエリのために大規模なテーブル セットをフェッチするためにありますが、クエリ メソッドを使用する必要があります
Select A,B from table limit val
collection.query( n_results = val #limit includes = [A,B] )
これで、データをフィルタリングする 3 つの方法が考えられます: 類似性検索 (ベクター データベースが主に使用される用途)、メタデータ フィルタ、ドキュメント フィルタ
類似性検索
テキストまたは埋め込みに基づいて検索し、最も類似した出力を取得できます
collection.query(query_texts=["string"]) collection.query(query_embeddings=[[1,2,3]])
ChromaDB では、where および where_document パラメーターを使用して、クエリ中に結果を フィルター します。これらのフィルターを使用すると、メタデータまたは特定のドキュメント コンテンツに基づいて類似性検索を絞り込むことができます。
メタデータによるフィルター
where パラメーターを使用すると、関連するメタデータに基づいてドキュメントをフィルターできます。メタデータは通常、ドキュメントの挿入時に指定するキーと値のペアの辞書です。
カテゴリ、作成者、日付などのメタデータでドキュメントをフィルタリングします。
import chromadb #setiing up the client client = chromadb.Client() collection = client.create_collection(name="name") collection.add( documents = ["str1","str2","str3",...] ids = [1,2,3,....] metadatas=[{"chapter": "3", "verse": "16"},{"chapter":"3", "verse":"5"}, ..] embeddings = [[1,2,3], [3,4,5], [5,6,7]] )
Create Table tablename
ドキュメントの内容でフィルタリングする
where_document パラメーターを使用すると、ドキュメントの内容に基づいて直接フィルター処理できます。
特定のキーワードを含むドキュメントのみを取得します。
#Create a collection collection = client.create_collection(name="name") #If a collection is already made and you need to use it again the use collection = client.get_collection(name="name")
重要なメモ:
- $contains、$startsWith、$endsWith などの演算子を使用します。
- $contains: 部分文字列を含むドキュメントと一致します。
- $startsWith: 部分文字列で始まるドキュメントと一致します。
- $endsWith: 部分文字列で終わるドキュメントと一致します。
-
例:
Insert into tablename Values(... , ..., ...)
一般的な使用例:
次のように 3 つのフィルターをすべて組み合わせることができます:
-
特定のカテゴリ内を検索:
collection.add( ids = [1] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] )
-
特定の用語を含むドキュメントを検索:
collection.update( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # If the id does not exist update will do nothing. to add data if id does not exist use collection.upsert( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # To delete data use delete and refrence the document or id or the feild collection.delete( documents = ["some text"] ) # Or you can delete from a bunch of ids using where that will apply filter on metadata collection.delete( ids=["id1", "id2", "id3",...], where={"chapter": "20"} )
-
メタデータとドキュメント コンテンツ フィルターを組み合わせる:
Select * from tablename Select * from tablename limit value Select Documents, Metadata from tablename
これらのフィルターにより類似性検索の精度が向上し、ChromaDB が対象を絞ったドキュメント検索のための強力なツールになります。
結論
独自のプログラムを作成しようとするときに、このドキュメントにはまだ多くの要望が残されていると感じたので、この記事を書きました。これがお役に立てば幸いです!
読んでいただきありがとうございます。この記事が気に入っていただけましたら、ぜひ「いいね!」してシェアしてください。また、あなたがソフトウェア アーキテクチャに慣れておらず、さらに詳しく知りたい場合は、私が個人的にあなたと協力し、ソフトウェア アーキテクチャと設計の原則についてすべてを教えるための小さなグループを提供するグループ ベースのコホートを開始します。ご興味がございましたら、以下のフォームにご記入ください。 https://forms.gle/SUAxrzRyvbnV8uCGA
以上がSQLマインドのためのChromaDBの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
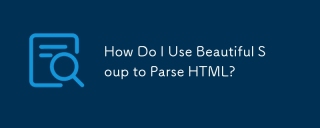
この記事では、Pythonライブラリである美しいスープを使用してHTMLを解析する方法について説明します。 find()、find_all()、select()、およびget_text()などの一般的な方法は、データ抽出、多様なHTML構造とエラーの処理、および代替案(SEL
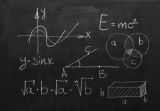
Pythonの統計モジュールは、強力なデータ統計分析機能を提供して、生物統計やビジネス分析などのデータの全体的な特性を迅速に理解できるようにします。データポイントを1つずつ見る代わりに、平均や分散などの統計を見て、無視される可能性のある元のデータの傾向と機能を発見し、大きなデータセットをより簡単かつ効果的に比較してください。 このチュートリアルでは、平均を計算し、データセットの分散の程度を測定する方法を説明します。特に明記しない限り、このモジュールのすべての関数は、単に平均を合計するのではなく、平均()関数の計算をサポートします。 浮動小数点数も使用できます。 ランダムをインポートします インポート統計 fractiから
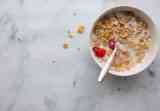
Pythonオブジェクトのシリアル化と脱介入は、非自明のプログラムの重要な側面です。 Pythonファイルに何かを保存すると、構成ファイルを読み取る場合、またはHTTPリクエストに応答する場合、オブジェクトシリアル化と脱滑り化を行います。 ある意味では、シリアル化と脱派化は、世界で最も退屈なものです。これらすべての形式とプロトコルを気にするのは誰ですか? Pythonオブジェクトを維持またはストリーミングし、後で完全に取得したいと考えています。 これは、概念レベルで世界を見るのに最適な方法です。ただし、実用的なレベルでは、選択したシリアル化スキーム、形式、またはプロトコルは、プログラムの速度、セキュリティ、メンテナンスの自由、およびその他の側面を決定する場合があります。
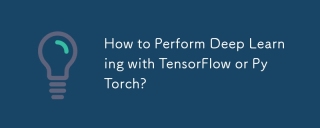
この記事では、深い学習のためにTensorflowとPytorchを比較しています。 関連する手順、データの準備、モデルの構築、トレーニング、評価、展開について詳しく説明しています。 特に計算グラップに関して、フレームワーク間の重要な違い
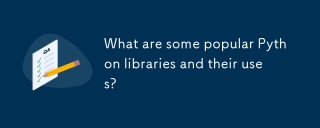
この記事では、numpy、pandas、matplotlib、scikit-learn、tensorflow、django、flask、and requestsなどの人気のあるPythonライブラリについて説明し、科学的コンピューティング、データ分析、視覚化、機械学習、Web開発、Hの使用について説明します。
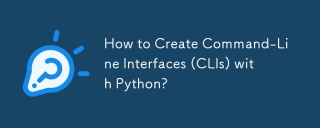
この記事では、コマンドラインインターフェイス(CLI)の構築に関するPython開発者をガイドします。 Typer、Click、Argparseなどのライブラリを使用して、入力/出力の処理を強調し、CLIの使いやすさを改善するためのユーザーフレンドリーな設計パターンを促進することを詳述しています。
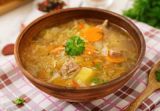
このチュートリアルは、単純なツリーナビゲーションを超えたDOM操作に焦点を当てた、美しいスープの以前の紹介に基づいています。 HTML構造を変更するための効率的な検索方法と技術を探ります。 1つの一般的なDOM検索方法はExです
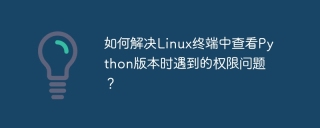
LinuxターミナルでPythonバージョンを表示する際の許可の問題の解決策PythonターミナルでPythonバージョンを表示しようとするとき、Pythonを入力してください...


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
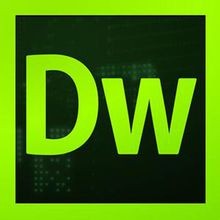
ドリームウィーバー CS6
ビジュアル Web 開発ツール
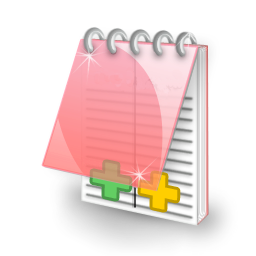
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません
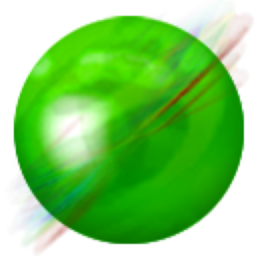
ZendStudio 13.5.1 Mac
強力な PHP 統合開発環境

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

ホットトピック









