Java バウンス ボール
問題:
フレームの端から跳ね返る複数のボールを画面上に描画する場合、2 番目のボールが最初のボールを上書きします。
与えられたコード:
提供されたコードは複数の跳ねるボールを描画しようとしますが、2 番目のボールが最初のボールを上書きします。
現在のアプローチでは、次の問題が存在します。
- 不透明なコンポーネントがそれぞれの上に配置されますその他。
- レイアウト マネージャーが指定されていないため、ボールの配置に影響します。
- ボールのサイズと位置の制御は効果的に処理されません。
- 速度
- ボールの更新は、 EDT。
- パネルを使用できるため、X/Y 値は必要ありません。
複数のスレッドのスケーラビリティの問題:
現在のアプローチでは、ボールごとに個別のスレッドを作成する必要があります。これにより、特にボールの数が増加した場合に、システム リソースに負担がかかる可能性があります。
代替アプローチ:
各ボールにコンポーネントを使用する代わりに、ボール用のコンテナを作成することを検討してください。そして、単純なアニメーション ループを使用して位置を更新し、再描画します。このアプローチはよりスケーラブルです。
実装:
上記の問題に対処する代替実装を次に示します。
public class AnimatedBalls { public static void main(String[] args) { new AnimatedBalls(); } public AnimatedBalls() { EventQueue.invokeLater(() -> { JFrame frame = new JFrame("Bouncing Balls"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(400, 400); frame.setVisible(true); // Create a container for the balls BallsPane ballsPane = new BallsPane(); frame.add(ballsPane); }); } public class BallsPane extends JPanel { private List<ball> balls; public BallsPane() { balls = new ArrayList(); for (int i = 0; i getWidth()) vx *= -1; if (y getHeight()) vy *= -1; // Update the ball's position x += vx; y += vy; } public void draw(Graphics g) { // Draw the ball as a filled circle g.setColor(Color.RED); g.fillOval(x - 5, y - 5, 10, 10); } } }</ball>
この代替実装では:
- 単一のアニメーション ループにより、すべてのアニメーション ループが更新されます。ボール。
- ボールは、単純なデータ構造 (コンポーネントではありません) で表されます。
- アニメーション ループは、フレームの端の境界を処理します。
- ランダム化は、変化に使用されます。ボールの開始速度と位置。
- update() メソッドは、それぞれの新しい位置を計算します。 ball.
- draw() メソッドは、ボールを塗りつぶされた円としてレンダリングします。
以上がJavaで複数の跳ねるボールを重複せずに効率的にアニメーション化するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
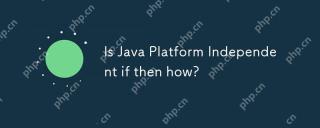
Javaは、Java Virtual Machines(JVMS)とBytecodeに依存している「Write and Averywherewherewherewherewherewherewhere」の哲学のために、プラットフォームに依存しません。 1)Javaコードは、JVMによって解釈されるか、地元でその場でコンパイルされたBytecodeにコンパイルされます。 2)ライブラリの依存関係、パフォーマンスの違い、環境構成に注意してください。 3)標準ライブラリを使用して、クロスプラットフォームのテストとバージョン管理がプラットフォームの独立性を確保するためのベストプラクティスです。
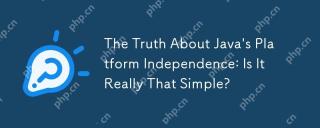
java'splatformindepenceisnotsimple; itinvolvescomplexities.1)jvmcompatibilitymustbeensuredacrosplatforms.2)nativeLibrariesandsystemCallSneedCarefulHandling.3)依存症の依存症の依存症と依存症の依存症と依存関係の増加 - プラットフォームのパフォーマンス
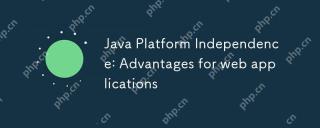
java'splatformentedentencebenefitswebapplicationsbyAllowingCodeTorunOnySystemwithajvm、simpledifyifieddeploymentandscaling.itenables:1)easydeploymentddifferentservers、2)Seamlessscalingacroscloudplatforms、および3)deminvermentementmentmentmentmentementtodeploymentpoce
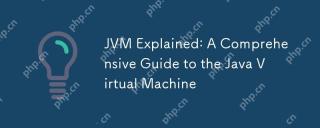
jvmistheruntimeenvironment forexecutingjavabytecode、Curivalforjavaの「writeonce、runanywhere」capability.itmanagesmemory、executessuressecurity、makingestessentionentionalforjavadevadedertionserstunterstanderforeffication devitivationdevation
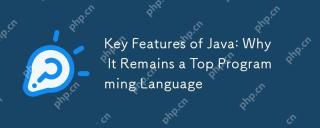
JavareMainsAtopChoiceFordevelopersDuetoitsPlatformEndepentence、Object-OrientedDesign、stryngting、automaticmemorymanagement、およびcomprehensivestandardlibrary.thesefeaturesmavaversatilatileandpowerful、sustableforawiderangeofplications、daspitesomech
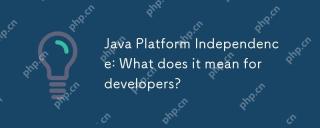
java'splatformentencemeansdeveloperscancancodecodeonceanddevicewithoutrocompilling.cancodecodecodecodecodecodecodecodecodecodecodecode compilling
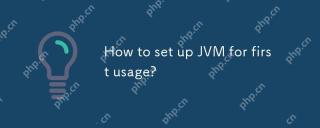
JVMをセットアップするには、次の手順に従う必要があります。1)JDKをダウンロードしてインストールする、2)環境変数を設定する、3)インストールの確認、4)IDEを設定する、5)ランナープログラムをテストします。 JVMのセットアップは、単に機能するだけでなく、メモリの割り当て、ガベージコレクション、パフォーマンスチューニング、エラー処理の最適化を行い、最適な動作を確保することも含まれます。
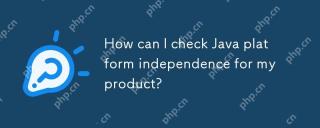
toensurejavaplatformindopendence、soflowthesesteps:1)compileandrunyourapplicationOnMultiplePlatformsusingDifferentosAndjvversions.2)utilizeci/cdpipelines


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

Safe Exam Browser
Safe Exam Browser は、オンライン試験を安全に受験するための安全なブラウザ環境です。このソフトウェアは、あらゆるコンピュータを安全なワークステーションに変えます。あらゆるユーティリティへのアクセスを制御し、学生が無許可のリソースを使用するのを防ぎます。

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。
