基本原則
障害が発生するとすぐに検出して報告し、無効な状態がシステム全体に伝播するのを防ぎます。
1. 入力の検証
class UserRegistration { public function register(array $data): void { // Validate all inputs immediately $this->validateEmail($data['email']); $this->validatePassword($data['password']); $this->validateAge($data['age']); // Only proceed if all validations pass $this->createUser($data); } private function validateEmail(string $email): void { if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { throw new ValidationException('Invalid email format'); } if ($this->emailExists($email)) { throw new DuplicateEmailException('Email already registered'); } } }
目的:
- 無効なデータがシステムに入るのを防ぎます
- 複雑な操作の前に失敗することでリソースを節約します
- ユーザーに明確なエラー メッセージを提供します
- データの整合性を維持します
2. 設定のロード
class AppConfig { private array $config; public function __construct(string $configPath) { if (!file_exists($configPath)) { throw new ConfigurationException("Config file not found: $configPath"); } $config = parse_ini_file($configPath, true); if ($config === false) { throw new ConfigurationException("Invalid config file format"); } $this->validateRequiredSettings($config); $this->config = $config; } private function validateRequiredSettings(array $config): void { $required = ['database', 'api_key', 'environment']; foreach ($required as $key) { if (!isset($config[$key])) { throw new ConfigurationException("Missing required config: $key"); } } } }
目的:
- アプリケーションが有効な構成で起動することを保証します
- 設定不足による実行時エラーを防止します
- 構成の問題をすぐに可視化します
- 構成の問題のデバッグを簡素化します
3. リソースの初期化
class DatabaseConnection { private PDO $connection; public function __construct(array $config) { try { $this->validateDatabaseConfig($config); $this->connection = new PDO( $this->buildDsn($config), $config['username'], $config['password'], [PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION] ); } catch (PDOException $e) { throw new DatabaseConnectionException( "Failed to connect to database: " . $e->getMessage() ); } } private function validateDatabaseConfig(array $config): void { $required = ['host', 'port', 'database', 'username', 'password']; foreach ($required as $param) { if (!isset($config[$param])) { throw new DatabaseConfigException("Missing $param in database config"); } } } }
目的:
- リソースが適切に初期化されていることを確認します
- アプリケーションが無効なリソースで実行されるのを防ぎます
- 起動時にリソースの問題を可視化します
- 無効なリソースによる連鎖的な失敗を回避します
4. 外部サービスコール
class PaymentGateway { public function processPayment(Order $order): PaymentResult { // Validate API credentials if (!$this->validateApiCredentials()) { throw new ApiConfigurationException('Invalid API credentials'); } // Validate order before external call if (!$order->isValid()) { throw new InvalidOrderException('Invalid order state'); } try { $response = $this->apiClient->charge($order); if (!$response->isSuccessful()) { throw new PaymentFailedException($response->getError()); } return new PaymentResult($response); } catch (ApiException $e) { throw new PaymentProcessingException( "Payment processing failed: " . $e->getMessage() ); } } }
目的:
- 無効なデータによる不要な API 呼び出しを防止します
- 時間とリソースを節約します
- API の問題について即座にフィードバックを提供します
- 外部サービスとの対話中にシステムの信頼性を維持します
5. データ処理パイプライン
class DataProcessor { public function processBatch(array $records): array { $this->validateBatchSize($records); $results = []; foreach ($records as $index => $record) { try { $this->validateRecord($record); $results[] = $this->processRecord($record); } catch (ValidationException $e) { throw new BatchProcessingException( "Failed at record $index: " . $e->getMessage() ); } } return $results; } private function validateBatchSize(array $records): void { if (empty($records)) { throw new EmptyBatchException('Empty batch provided'); } if (count($records) > 1000) { throw new BatchSizeException('Batch size exceeds maximum limit'); } } }
目的:
- 処理全体を通じてデータの一貫性を確保します
- 無効なデータの部分的な処理を防止します
- データの問題を早期に可視化します
- 複雑なパイプラインでのエラー追跡を簡素化します
- 変換全体でデータの整合性を維持します
フェイルファストの利点
- エラーの早期検出
- よりクリーンなデバッグ
- 連鎖的な障害を防止します
- データの整合性を維持します
- システムの信頼性を向上させます
ベストプラクティス
- 強い型宣言を使用する
- 徹底した入力検証を実装する
- 特定の例外をスローする
- プロセスの早い段階で検証する
- 開発でアサーションを使用する
- 適切なエラー処理を実装する
- 失敗を適切にログに記録します
フェイルファストを使用する場合
- 入力検証
- 設定のロード中
- リソースの初期化
- 外部サービス呼び出し
- データ処理パイプライン
以上がフェイルファストの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
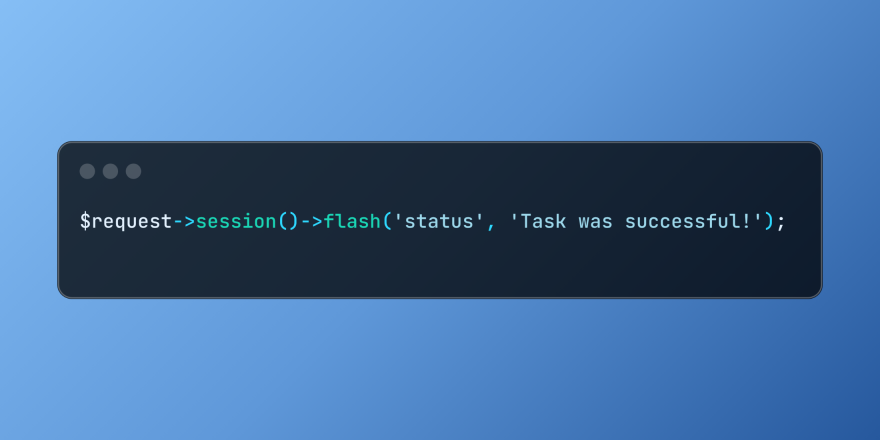
Laravelは、直感的なフラッシュメソッドを使用して、一時的なセッションデータの処理を簡素化します。これは、アプリケーション内に簡単なメッセージ、アラート、または通知を表示するのに最適です。 データは、デフォルトで次の要求のためにのみ持続します。 $リクエスト -
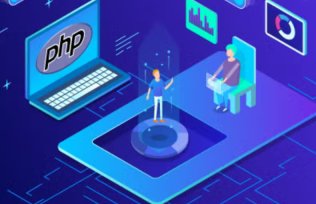
PHPクライアントURL(CURL)拡張機能は、開発者にとって強力なツールであり、リモートサーバーやREST APIとのシームレスな対話を可能にします。尊敬されるマルチプロトコルファイル転送ライブラリであるLibcurlを活用することにより、PHP Curlは効率的なexecuを促進します
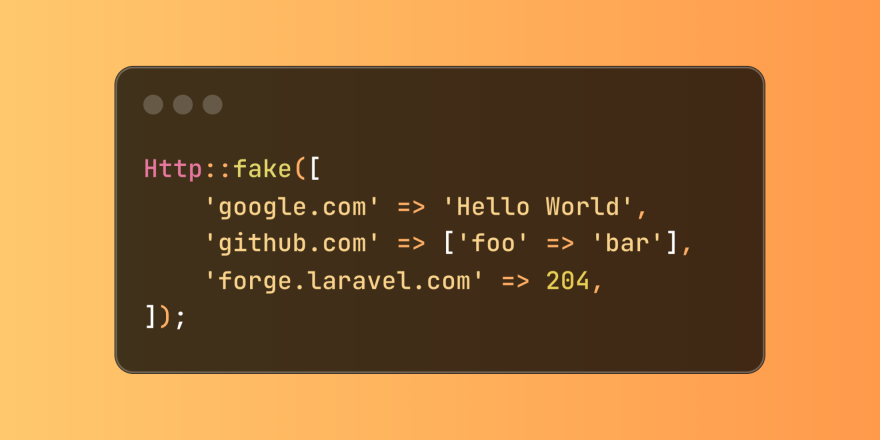
Laravelは簡潔なHTTP応答シミュレーション構文を提供し、HTTP相互作用テストを簡素化します。このアプローチは、テストシミュレーションをより直感的にしながら、コード冗長性を大幅に削減します。 基本的な実装は、さまざまな応答タイプのショートカットを提供します。 Illuminate \ support \ facades \ httpを使用します。 http :: fake([[ 'google.com' => 'hello world'、 'github.com' => ['foo' => 'bar']、 'forge.laravel.com' =>
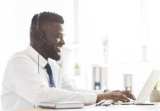
顧客の最も差し迫った問題にリアルタイムでインスタントソリューションを提供したいですか? ライブチャットを使用すると、顧客とのリアルタイムな会話を行い、すぐに問題を解決できます。それはあなたがあなたのカスタムにより速いサービスを提供することを可能にします
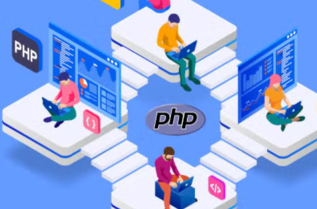
PHPロギングは、Webアプリケーションの監視とデバッグ、および重要なイベント、エラー、ランタイムの動作をキャプチャするために不可欠です。システムのパフォーマンスに関する貴重な洞察を提供し、問題の特定に役立ち、より速いトラブルシューティングをサポートします
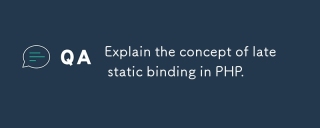
記事では、PHP 5.3で導入されたPHPの後期静的結合(LSB)について説明し、より柔軟な継承を求める静的メソッドコールのランタイム解像度を可能にします。 LSBの実用的なアプリケーションと潜在的なパフォーマ
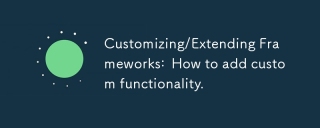
この記事では、フレームワークにカスタム機能を追加し、アーキテクチャの理解、拡張ポイントの識別、統合とデバッグのベストプラクティスに焦点を当てています。

記事では、入力検証、認証、定期的な更新など、脆弱性から保護するためのフレームワークの重要なセキュリティ機能について説明します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。
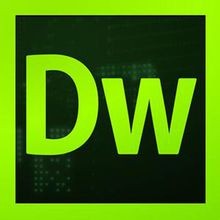
ドリームウィーバー CS6
ビジュアル Web 開発ツール

Safe Exam Browser
Safe Exam Browser は、オンライン試験を安全に受験するための安全なブラウザ環境です。このソフトウェアは、あらゆるコンピュータを安全なワークステーションに変えます。あらゆるユーティリティへのアクセスを制御し、学生が無許可のリソースを使用するのを防ぎます。

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール

ホットトピック



