導入
Spring ログイン アプリケーション は、Spring Boot を使用して構築された安全で堅牢なユーザー管理システムです。このプロジェクトでは、認証、認可、およびユーザー アカウント機能を実装するための最新のアプローチを示します。主な機能には、ユーザー登録、BCrypt による安全なパスワード処理、電子メールベースのパスワード リセット、JWT (JSON Web Token) 認証などがあります。拡張性とスケーラビリティを念頭に置いて設計されたこのアプリケーションは、ユーザー管理とロールベースのアクセス制御を必要とするプロジェクトの優れた基盤として機能します。
Spring Security、Spring Data JPA、JavaMailSender などの Spring の強力なツールを活用することで、このプロジェクトはセキュリティ、保守性、容易さのベスト プラクティスを保証します。統合の。小規模な Web アプリケーションを構築している場合でも、大規模なエンタープライズ システムを構築している場合でも、このプロジェクトは、ユーザー アカウントを安全に管理するための実用的でよく構造化された開始点を提供します。
構成
Pom.xml の依存関係
<dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-oauth2-resource-server</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-security</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-web</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-validation</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-mail</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-devtools</artifactid> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupid>org.postgresql</groupid> <artifactid>postgresql</artifactid> <scope>runtime</scope> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency> <dependency> <groupid>org.springframework.security</groupid> <artifactid>spring-security-test</artifactid> <scope>test</scope> </dependency> </dependencies>
ドッカー
PostgreSQL データベースを実行するには、docker-compose.yaml ファイルを作成します。
services: postgres: image: postgres:latest ports: - "5432:5432" environment: - POSTGRES_DB=database - POSTGRES_USER=admin - POSTGRES_PASSWORD=admin volumes: - postgres_data:/var/lib/postgresql/data volumes: postgres_data:
実行:
docker compose up -d
アプリケーションのプロパティ
spring.application.name=login_app spring.datasource.url=jdbc:postgresql://localhost:5432/database spring.datasource.username=admin spring.datasource.password=admin spring.mail.host=sandbox.smtp.mailtrap.io spring.mail.port=2525 spring.mail.properties.mail.smtp.auth=true spring.mail.properties.mail.smtp.starttls.enable=true spring.mail.properties.mail.smtp.starttls.required=true spring.mail.default-encoding=UTF-8 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true spring.config.import=classpath:env.properties jwt.public.key=classpath:public.key jwt.private.key=classpath:private.key
環境プロパティ
spring.mail.username=<get in your mailtrap account> spring.mail.password=<get in your mailtrap account> </get></get>
非対称キーを作成するにはどうすればよいですか?
非対称キーを生成する方法については、この投稿をご覧ください
プロジェクトの構造
login_app/ ├── .mvn/ # Maven folder (Maven configurations) ├── src/ │ ├── main/ │ │ ├── java/ │ │ │ └── dev/ │ │ │ └── mspilari/ │ │ │ └── login_app/ │ │ │ ├── configs/ # Security, authentication, and other configurations │ │ │ ├── domains/ # Main application domains │ │ │ │ ├── email/ # Email-related logic │ │ │ │ └── user/ # User-related logic │ │ │ ├── exceptions/ # Custom exceptions and error handling │ │ │ └── utils/ # Utilities and helpers │ │ └── resources/ # Resources (e.g., configuration files) │ └── test/ # Application tests ├── target/ # Build folder generated by Maven ├── .gitattributes # Git attributes configuration ├── .gitignore # Git ignore file ├── docker-compose.yaml # Docker Compose configuration ├── HELP.md # Project help documentation ├── mvnw # Maven Wrapper script for Linux ├── mvnw.cmd # Maven Wrapper script for Windows └── pom.xml # Maven configuration file
特徴
- 電子メールとパスワードの検証によるユーザー登録
- JWT認証によるログイン
- メールリンク配信によるパスワード回復
- 一時トークンを使用したリンクによるパスワードのリセット
- フィールドの検証とエラー処理
コード
設定ディレクトリ
BCryptPasswordConfig.java
package dev.mspilari.login_app.configs; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; @Configuration public class BCryptPasswordConfig { @Bean public BCryptPasswordEncoder bPasswordEncoder() { return new BCryptPasswordEncoder(); } }
コードの内訳
-
@構成
- このアノテーションは、クラスに Bean 定義が含まれていることを Spring に伝えます。
- @Configuration のアノテーションが付けられたクラスはアプリケーションの起動時に処理され、@Bean のアノテーションが付けられたメソッドは戻り値をマネージド Bean として Spring アプリケーション コンテキストに追加します。
-
@Bean
- bPasswordEncoder() メソッドの @Bean アノテーションは、このメソッドが Spring アプリケーション コンテキストに Bean として登録する必要があるオブジェクトを返すことを示します。
- これにより、BCryptPasswordEncoder オブジェクトをアプリケーション内の必要な場所に挿入できるようになります。
-
BCryptPasswordEncoder
- これは、パスワードをエンコードするために Spring Security によって提供されるユーティリティ クラスです。
- これは、パスワードをハッシュするための強力で安全な方法と考えられている BCrypt ハッシュ アルゴリズム を使用します。このアルゴリズムは、ハッシュ化する前にパスワードに自動的に「ソルト」を追加し、辞書攻撃やレインボー テーブル攻撃に対する耐性を高めます。
-
メソッド bPasswordEncoder()
- このメソッドが Spring フレームワークによって呼び出されると、BCryptPasswordEncoder の新しいインスタンスが作成され、アプリケーション コンテキストで使用できるようになります。
- アプリケーション内の他のクラスは、この Bean を自動配線してパスワードをエンコードまたは照合できます。
JwtConfig.java
<dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-oauth2-resource-server</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-security</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-web</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-validation</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-mail</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-devtools</artifactid> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupid>org.postgresql</groupid> <artifactid>postgresql</artifactid> <scope>runtime</scope> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency> <dependency> <groupid>org.springframework.security</groupid> <artifactid>spring-security-test</artifactid> <scope>test</scope> </dependency> </dependencies>
コードの内訳
1.クラスレベルのアノテーション
services: postgres: image: postgres:latest ports: - "5432:5432" environment: - POSTGRES_DB=database - POSTGRES_USER=admin - POSTGRES_PASSWORD=admin volumes: - postgres_data:/var/lib/postgresql/data volumes: postgres_data:
- これは、Bean (Spring 管理コンポーネント) が定義されている Spring 構成クラスであることを示します。
- ここで定義された Bean は、Spring アプリケーション コンテキストで依存関係の注入に使用できます。
2.構成からの RSA キーの挿入
docker compose up -d
- @Value は、アプリケーションのプロパティ ファイル (application.yml や application.properties など) から 公開キー と 秘密キー を挿入するために使用されます。
- これらのキーは次のようにプロパティに含まれることが想定されます。
spring.application.name=login_app spring.datasource.url=jdbc:postgresql://localhost:5432/database spring.datasource.username=admin spring.datasource.password=admin spring.mail.host=sandbox.smtp.mailtrap.io spring.mail.port=2525 spring.mail.properties.mail.smtp.auth=true spring.mail.properties.mail.smtp.starttls.enable=true spring.mail.properties.mail.smtp.starttls.required=true spring.mail.default-encoding=UTF-8 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true spring.config.import=classpath:env.properties jwt.public.key=classpath:public.key jwt.private.key=classpath:private.key
3. JWT エンコーダ Bean
spring.mail.username=<get in your mailtrap account> spring.mail.password=<get in your mailtrap account> </get></get>
- 目的: JWT トークンをエンコード (生成) するための Bean を作成します。
-
手順:
-
RSA キーを構築:
- RSAKey.Builder は、RSA 公開鍵/秘密鍵ペアの JWK (JSON Web Key) 表現を作成します。
-
JWK セットの作成:
- ImmutableJWKSet はキーをセットに保存します。このセットは、Nimbus JOSE ライブラリによってトークンに署名するために使用されます。
-
NimbusJwtEncoder:
- このエンコーダーは、ImmutableJWKSet を使用して、秘密キーを使用してトークンをエンコードし、署名します。
-
RSA キーを構築:
4. JWT デコーダ Bean
login_app/ ├── .mvn/ # Maven folder (Maven configurations) ├── src/ │ ├── main/ │ │ ├── java/ │ │ │ └── dev/ │ │ │ └── mspilari/ │ │ │ └── login_app/ │ │ │ ├── configs/ # Security, authentication, and other configurations │ │ │ ├── domains/ # Main application domains │ │ │ │ ├── email/ # Email-related logic │ │ │ │ └── user/ # User-related logic │ │ │ ├── exceptions/ # Custom exceptions and error handling │ │ │ └── utils/ # Utilities and helpers │ │ └── resources/ # Resources (e.g., configuration files) │ └── test/ # Application tests ├── target/ # Build folder generated by Maven ├── .gitattributes # Git attributes configuration ├── .gitignore # Git ignore file ├── docker-compose.yaml # Docker Compose configuration ├── HELP.md # Project help documentation ├── mvnw # Maven Wrapper script for Linux ├── mvnw.cmd # Maven Wrapper script for Windows └── pom.xml # Maven configuration file
- 目的: JWT トークンをデコードおよび検証するための Bean を作成します。
-
手順:
-
公開鍵の検証:
- NimbusJwtDecoder.withPublicKey() は RSA 公開キーを使用して構成されます。トークンの署名を検証します。
-
デコーダーのビルド:
- build() メソッドはデコーダー インスタンスを作成します。
-
公開鍵の検証:
JWT のエンコーディングとデコーディングの仕組み
-
JWT エンコーディング (トークン生成):
- JwtEncoder Bean は、署名付き JWT トークンを作成するために使用されます。通常、このトークンにはクレームとしてユーザー情報 (ユーザー名、ロールなど) が含まれており、RSA 秘密キーを使用して署名されます。
- 例:
package dev.mspilari.login_app.configs; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; @Configuration public class BCryptPasswordConfig { @Bean public BCryptPasswordEncoder bPasswordEncoder() { return new BCryptPasswordEncoder(); } }
-
JWT デコード (トークン検証):
- JwtDecoder Bean は、RSA 公開キーを使用してトークンをデコードおよび検証するために使用されます。これにより、トークンが次のように保証されます。
- サーバーによって発行されました (署名検証)。
- 改ざんされていません。
- 例:
package dev.mspilari.login_app.configs; import java.security.interfaces.RSAPrivateKey; import java.security.interfaces.RSAPublicKey; import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.oauth2.jwt.JwtDecoder; import org.springframework.security.oauth2.jwt.JwtEncoder; import org.springframework.security.oauth2.jwt.NimbusJwtDecoder; import org.springframework.security.oauth2.jwt.NimbusJwtEncoder; import com.nimbusds.jose.jwk.JWKSet; import com.nimbusds.jose.jwk.RSAKey; import com.nimbusds.jose.jwk.source.ImmutableJWKSet; @Configuration public class JwtConfig { @Value("${jwt.public.key}") private RSAPublicKey publicKey; @Value("${jwt.private.key}") private RSAPrivateKey privateKey; @Bean public JwtEncoder jwtEncoder() { var jwk = new RSAKey.Builder(this.publicKey).privateKey(this.privateKey).build(); var jwks = new ImmutableJWKSet(new JWKSet(jwk)); return new NimbusJwtEncoder(jwks); } @Bean public JwtDecoder jwtDecoder() { return NimbusJwtDecoder.withPublicKey(this.publicKey).build(); } }
- JwtDecoder Bean は、RSA 公開キーを使用してトークンをデコードおよび検証するために使用されます。これにより、トークンが次のように保証されます。
SecurityConfig.java
<dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-oauth2-resource-server</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-security</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-web</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-validation</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-mail</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-devtools</artifactid> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupid>org.postgresql</groupid> <artifactid>postgresql</artifactid> <scope>runtime</scope> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency> <dependency> <groupid>org.springframework.security</groupid> <artifactid>spring-security-test</artifactid> <scope>test</scope> </dependency> </dependencies>
1.クラスレベルのアノテーション
services: postgres: image: postgres:latest ports: - "5432:5432" environment: - POSTGRES_DB=database - POSTGRES_USER=admin - POSTGRES_PASSWORD=admin volumes: - postgres_data:/var/lib/postgresql/data volumes: postgres_data:
- @Configuration: このクラスを Bean を定義する Spring 構成としてマークします。
- @EnableWebSecurity: Spring Security の Web セキュリティ機能を有効にします。
- @EnableMethodSecurity: @PreAuthorize や @Secured などのメソッドレベルのセキュリティ アノテーションをアクティブにします。これにより、ロール、権限、または条件に基づいてアプリケーション内の特定のメソッドへのアクセスを制御できます。
2. SecurityFilterChain Bean
docker compose up -d
- アプリケーションのセキュリティ フィルター チェーンを定義します。フィルター チェーンは、受信 HTTP リクエストに適用される一連のセキュリティ フィルターです。
3. CSRF保護
spring.application.name=login_app spring.datasource.url=jdbc:postgresql://localhost:5432/database spring.datasource.username=admin spring.datasource.password=admin spring.mail.host=sandbox.smtp.mailtrap.io spring.mail.port=2525 spring.mail.properties.mail.smtp.auth=true spring.mail.properties.mail.smtp.starttls.enable=true spring.mail.properties.mail.smtp.starttls.required=true spring.mail.default-encoding=UTF-8 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true spring.config.import=classpath:env.properties jwt.public.key=classpath:public.key jwt.private.key=classpath:private.key
-
CSRF (クロスサイト リクエスト フォージェリ) 保護が無効になっています。
- トークン (JWT など) はすでに不正なリクエストを防ぐ方法を提供しているため、CSRF 保護はステートレス API には不要であることがよくあります。
- これを無効にすると、この JWT ベースの API のセキュリティ構成が簡素化されます。
4.認可ルール
spring.mail.username=<get in your mailtrap account> spring.mail.password=<get in your mailtrap account> </get></get>
- どのエンドポイントに認証が必要かを構成します。
- すべてを許可:
- /user/register、/user/login、/user/redeem-password、/user/reset-password などのエンドポイントへの POST リクエストは誰でも公開されています (認証は必要ありません)。
- これらのエンドポイントは、ユーザー登録、ログイン、パスワードの回復/リセットに使用される可能性が高く、通常はログインせずにアクセスできます。
- 他のリクエストを認証する:
- 他のすべてのエンドポイント (anyRequest) には認証が必要です。
5. JWT 検証
login_app/ ├── .mvn/ # Maven folder (Maven configurations) ├── src/ │ ├── main/ │ │ ├── java/ │ │ │ └── dev/ │ │ │ └── mspilari/ │ │ │ └── login_app/ │ │ │ ├── configs/ # Security, authentication, and other configurations │ │ │ ├── domains/ # Main application domains │ │ │ │ ├── email/ # Email-related logic │ │ │ │ └── user/ # User-related logic │ │ │ ├── exceptions/ # Custom exceptions and error handling │ │ │ └── utils/ # Utilities and helpers │ │ └── resources/ # Resources (e.g., configuration files) │ └── test/ # Application tests ├── target/ # Build folder generated by Maven ├── .gitattributes # Git attributes configuration ├── .gitignore # Git ignore file ├── docker-compose.yaml # Docker Compose configuration ├── HELP.md # Project help documentation ├── mvnw # Maven Wrapper script for Linux ├── mvnw.cmd # Maven Wrapper script for Windows └── pom.xml # Maven configuration file
- JWT トークンを使用してリクエストを検証する OAuth 2.0 リソース サーバー としてアプリケーションを構成します。
-
JWT デコーダー:
- JwtDecoder Bean (JwtConfig によって提供される) は、セキュリティで保護されたエンドポイントへのリクエストの受信 JWT トークンを検証するために使用されます。
仕組み
- CSRF の無効化: これはステートレス JWT 認証に依存する API であるため、CSRF を無効にするのが一般的です。
-
認可ルール:
- 認証されていないユーザーは、明示的に許可されたエンドポイント (/user/register または /user/login など) にのみアクセスできます。
- その他のリクエストには有効な JWT トークンが必要です。
-
JWT 検証:
- Spring Security は、受信リクエストから Authorization ヘッダーを自動的に抽出します。
- ヘッダーに有効な JWT トークンが含まれている場合、リクエストは認証され、ユーザー コンテキストが確立されます。
- トークンが無効または欠落している場合、リクエストは拒否されます。
ドメインディレクトリ
電子メールディレクトリ
サービスディレクトリ
<dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-oauth2-resource-server</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-security</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-web</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-validation</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-mail</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-devtools</artifactid> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupid>org.postgresql</groupid> <artifactid>postgresql</artifactid> <scope>runtime</scope> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency> <dependency> <groupid>org.springframework.security</groupid> <artifactid>spring-security-test</artifactid> <scope>test</scope> </dependency> </dependencies>
ユーザーディレクトリ
コントローラのディレクトリ
services: postgres: image: postgres:latest ports: - "5432:5432" environment: - POSTGRES_DB=database - POSTGRES_USER=admin - POSTGRES_PASSWORD=admin volumes: - postgres_data:/var/lib/postgresql/data volumes: postgres_data:
DTOディレクトリ
UserDto.java
docker compose up -d
UserRedeemPasswordDto.java
spring.application.name=login_app spring.datasource.url=jdbc:postgresql://localhost:5432/database spring.datasource.username=admin spring.datasource.password=admin spring.mail.host=sandbox.smtp.mailtrap.io spring.mail.port=2525 spring.mail.properties.mail.smtp.auth=true spring.mail.properties.mail.smtp.starttls.enable=true spring.mail.properties.mail.smtp.starttls.required=true spring.mail.default-encoding=UTF-8 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true spring.config.import=classpath:env.properties jwt.public.key=classpath:public.key jwt.private.key=classpath:private.key
UserResetPasswordDto.java
spring.mail.username=<get in your mailtrap account> spring.mail.password=<get in your mailtrap account> </get></get>
エンティティディレクトリ
UserEntity.java
login_app/ ├── .mvn/ # Maven folder (Maven configurations) ├── src/ │ ├── main/ │ │ ├── java/ │ │ │ └── dev/ │ │ │ └── mspilari/ │ │ │ └── login_app/ │ │ │ ├── configs/ # Security, authentication, and other configurations │ │ │ ├── domains/ # Main application domains │ │ │ │ ├── email/ # Email-related logic │ │ │ │ └── user/ # User-related logic │ │ │ ├── exceptions/ # Custom exceptions and error handling │ │ │ └── utils/ # Utilities and helpers │ │ └── resources/ # Resources (e.g., configuration files) │ └── test/ # Application tests ├── target/ # Build folder generated by Maven ├── .gitattributes # Git attributes configuration ├── .gitignore # Git ignore file ├── docker-compose.yaml # Docker Compose configuration ├── HELP.md # Project help documentation ├── mvnw # Maven Wrapper script for Linux ├── mvnw.cmd # Maven Wrapper script for Windows └── pom.xml # Maven configuration file
列挙型ディレクトリ
Role.java
package dev.mspilari.login_app.configs; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; @Configuration public class BCryptPasswordConfig { @Bean public BCryptPasswordEncoder bPasswordEncoder() { return new BCryptPasswordEncoder(); } }
リポジトリディレクトリ
UserRepository.java
package dev.mspilari.login_app.configs; import java.security.interfaces.RSAPrivateKey; import java.security.interfaces.RSAPublicKey; import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.oauth2.jwt.JwtDecoder; import org.springframework.security.oauth2.jwt.JwtEncoder; import org.springframework.security.oauth2.jwt.NimbusJwtDecoder; import org.springframework.security.oauth2.jwt.NimbusJwtEncoder; import com.nimbusds.jose.jwk.JWKSet; import com.nimbusds.jose.jwk.RSAKey; import com.nimbusds.jose.jwk.source.ImmutableJWKSet; @Configuration public class JwtConfig { @Value("${jwt.public.key}") private RSAPublicKey publicKey; @Value("${jwt.private.key}") private RSAPrivateKey privateKey; @Bean public JwtEncoder jwtEncoder() { var jwk = new RSAKey.Builder(this.publicKey).privateKey(this.privateKey).build(); var jwks = new ImmutableJWKSet(new JWKSet(jwk)); return new NimbusJwtEncoder(jwks); } @Bean public JwtDecoder jwtDecoder() { return NimbusJwtDecoder.withPublicKey(this.publicKey).build(); } }
サービスディレクトリ
UserService.java
@Configuration
例外ディレクトリ
GlobalException.java
@Value("${jwt.public.key}") private RSAPublicKey publicKey; @Value("${jwt.private.key}") private RSAPrivateKey privateKey;
ユーティリティディレクトリ
JwtActions.java
jwt.public.key=<your-public-key> jwt.private.key=<your-private-key> </your-private-key></your-public-key>
結論
このプロジェクトでは、Spring Boot を使用して安全で機能が豊富なユーザー認証システムを実装することに成功しました。このアプリケーションには、ユーザー登録、ログイン、JWT ベースの認証などのコア機能に加えて、パスワード回復システムも組み込まれています。ユーザーは電子メールのリンクを通じてパスワードをリセットできるため、スムーズで安全な回復プロセスが保証されます。
電子メールベースのパスワード回復を容易にするために、Spring Email を安全で効率的な電子メール テスト サービスである Mailtrap と統合しました。これにより、アプリケーションは、電子メールが安全に送信され、制御された環境でテストされることを保証しながら、一時トークンを含むパスワード リセット リンクを送信できるようになります。この設定では、開発やテスト中に実際のユーザーを潜在的な問題にさらすことなく、パスワード回復などの機密性の高いワークフローを処理する方法を示します。
安全な認証方法、堅牢なパスワード管理、シームレスな電子メール統合の組み合わせにより、このアプリケーションは最新の Web システムにとって信頼できる基盤となります。開発者は、これらのプラクティスを特定の要件に合わせて調整し、スケーラビリティとユーザーの信頼の両方を確保できます。 Spring Security や Mailtrap などのベスト プラクティスとツールを活用することで、安全でユーザー重視のアプリケーションを簡単に構築する方法を実証しました。
?参照
- スプリングセキュリティ
- メールトラップ
- 春のメール
?プロジェクトリポジトリ
- Github 上のプロジェクト リポジトリ
?私に話して
- リンクトイン
- Github
- ポートフォリオ
以上がJWT トークンと電子メール リセット パスワードを使用したログイン システムの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
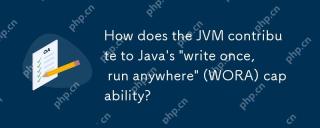
JVMは、バイトコード解釈、プラットフォームに依存しないAPI、動的クラスの負荷を介してJavaのWORA機能を実装します。 2。標準API抽象オペレーティングシステムの違い。 3.クラスは、実行時に動的にロードされ、一貫性を確保します。
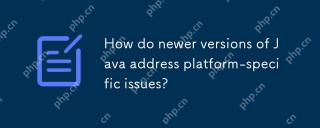
Javaの最新バージョンは、JVMの最適化、標準的なライブラリの改善、サードパーティライブラリサポートを通じて、プラットフォーム固有の問題を効果的に解決します。 1)Java11のZGCなどのJVM最適化により、ガベージコレクションのパフォーマンスが向上します。 2)Java9のモジュールシステムなどの標準的なライブラリの改善は、プラットフォーム関連の問題を削減します。 3)サードパーティライブラリは、OpenCVなどのプラットフォーム最適化バージョンを提供します。
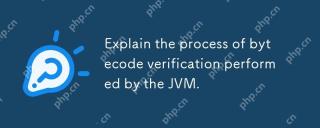
JVMのバイトコード検証プロセスには、4つの重要な手順が含まれます。1)クラスファイル形式が仕様に準拠しているかどうかを確認し、2)バイトコード命令の有効性と正確性を確認し、3)データフロー分析を実行してタイプの安全性を確保し、検証の完全性とパフォーマンスのバランスをとる。これらの手順を通じて、JVMは、安全で正しいバイトコードのみが実行されることを保証し、それによりプログラムの完全性とセキュリティを保護します。
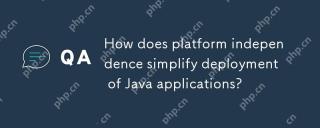
java'splatformendencealLowsApplicationStorunOperatingSystemwithajvm.1)singlecodebase:writeandcompileonceforallplatforms.2)easyUpdates:updatebytecodeforsimultaneousdeployment.3)テストの実験効果:scalbortffortfforduniverbehaviol.4)
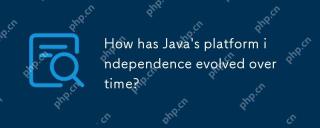
Javaのプラットフォームの独立性は、JVM、JITコンピレーション、標準化、ジェネリック、ラムダ式、Projectpanamaなどのテクノロジーを通じて継続的に強化されています。 1990年代以来、Javaは基本的なJVMから高性能モダンJVMに進化し、さまざまなプラットフォームでのコードの一貫性と効率を確保しています。
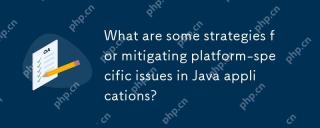
Javaはプラットフォーム固有の問題をどのように軽減しますか? Javaは、JVMおよび標準ライブラリを通じてプラットフォームに依存します。 1)bytecodeとjvmを使用して、オペレーティングシステムの違いを抽象化します。 2)標準のライブラリは、パスクラス処理ファイルパス、CHARSETクラス処理文字エンコードなど、クロスプラットフォームAPIを提供します。 3)最適化とデバッグのために、実際のプロジェクトで構成ファイルとマルチプラットフォームテストを使用します。
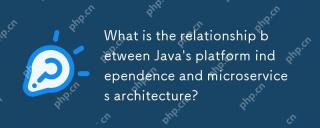
java'splatformentencentenhancesmicroservicesecturectureby byofferingdeploymentflexability、一貫性、スケーラビリティ、およびポート可能性。1)展開の展開の展開は、AllosmicRoserviThajvm.2)deploymentflexibility lowsmicroserviceSjvm.2)一貫性のあるAcrossServicessimplisimpligiessdevelisementand
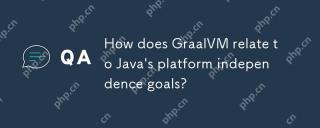
Graalvmは、Javaのプラットフォームの独立性を3つの方法で強化します。1。言語間の相互運用性、Javaが他の言語とシームレスに相互運用できるようにします。 2。独立したランタイム環境、graalvmnativeimageを介してJavaプログラムをローカル実行可能ファイルにコンパイルします。 3.パフォーマンスの最適化、Graalコンパイラは、Javaプログラムのパフォーマンスと一貫性を改善するための効率的なマシンコードを生成します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
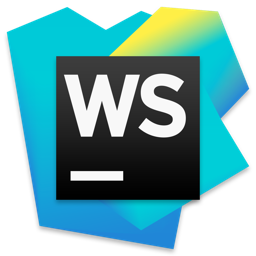
WebStorm Mac版
便利なJavaScript開発ツール

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!
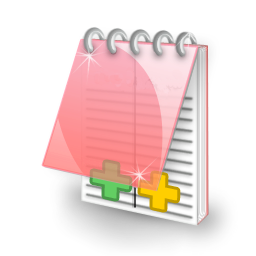
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません
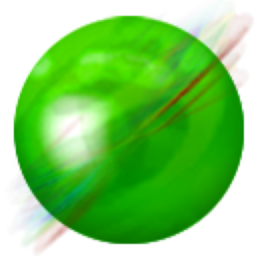
ZendStudio 13.5.1 Mac
強力な PHP 統合開発環境

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

ホットトピック









