Angular コンポーネントは Angular アプリケーションの基盤であり、ユーザー インターフェイスのモジュール式の再利用可能な部分を構築する方法を提供します。このガイドでは、Angular コンポーネントの構造からベスト プラクティスまで、Angular コンポーネントの基本について説明します。 Angular を初めて使用する場合でも、復習したい場合でも、この記事は Angular のコンポーネントの基本を理解するのに役立ちます。
Angular コンポーネントとは何ですか?
Angular では、コンポーネントはユーザー インターフェイス (UI) の一部を制御するクラスです。ボタン、タブ、入力、フォーム、ドロワー (実際には UI のあらゆる部分) について考えてみましょう。各コンポーネントは自己完結型であり、以下で構成されます:
- HTML テンプレート: UI のレイアウトと構造を定義します。
- CSS スタイル: コンポーネントの外観とスタイルを設定します。
- TypeScript クラス: コンポーネントのロジックとデータが含まれます。
- メタデータ: Angular がコンポーネントを認識して使用するための構成の詳細を提供します。
コンポーネントは、それぞれがヘッダー、サイドバー、カードなどのページの特定の部分を表すことができるため、モジュラー アプリケーションの作成に不可欠です。
Angular コンポーネントの基本構造
Angular コンポーネントは @Component デコレーターを使用して定義され、必要なテンプレート、スタイル、セレクターで構成されます。基本的な例を次に示します:
import { Component } from '@angular/core'; @Component({ selector: 'app-example', templateUrl: './example.component.html', styleUrls: ['./example.component.css'] }) export class ExampleComponent { title: string = 'Hello, Angular!'; getTitle() { return this.title; } }
この例では:
- selector はコンポーネントを表す HTML タグです。
- templateUrl は、HTML テンプレート ファイルを指します。
- styleUrls はコンポーネントの CSS ファイルを参照します。
- ExampleComponent クラスは、コンポーネントのデータとロジックを保持します。
一般的なコンポーネントのフォルダー構造
Angular プロジェクトは通常、コンポーネントとその関連ファイルを 1 つのフォルダーに整理し、Angular CLI の使用時に自動的に作成されます。コンポーネントの一般的なフォルダー構造には次のものが含まれます:
- example.component.ts: TypeScript クラスを定義します。
- example.component.html: HTML テンプレートが含まれます。
- example.component.css: コンポーネントのスタイルを保持します。
- example.component.spec.ts: コンポーネントのテストが含まれます。
コンポーネントのライフサイクル
Angular コンポーネントには、開発者がさまざまな段階でアクションを実行できるようにするフックを備えたライフサイクルがあります。一般的に使用されるライフサイクル フックには次のものがあります。
- ngOnInit: コンポーネントが初期化された後に呼び出されます。
- ngOnChanges: データ バインドされたプロパティが変更されるとトリガーされます。
- ngOnDestroy: Angular がコンポーネントを破棄する直前に呼び出されます。
たとえば、ngOnInit の使用方法は次のとおりです。
import { Component } from '@angular/core'; @Component({ selector: 'app-example', templateUrl: './example.component.html', styleUrls: ['./example.component.css'] }) export class ExampleComponent { title: string = 'Hello, Angular!'; getTitle() { return this.title; } }
ライフサイクル フックは柔軟性を提供し、コンポーネントのライフサイクルの特定の段階でロジックを管理しやすくします。
コンポーネント間の通信
実際のアプリケーションでは、データを共有したりアクションをトリガーしたりするために、コンポーネントが相互に対話する必要があることがよくあります。 Angular は、コンポーネント通信のためのいくつかのメソッドを提供します。
1. @Input と @Output
- @Input: 親コンポーネントが子コンポーネントにデータを渡すことを許可します。
- @Output: 子コンポーネントがその親にイベントを送信できるようにします。
例:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-lifecycle', template: '<p>Lifecycle example</p>', }) export class LifecycleComponent implements OnInit { ngOnInit() { console.log('Component initialized!'); } }
// child.component.ts import { Component, Input, Output, EventEmitter } from '@angular/core'; @Component({ selector: 'app-child', template: `<button>Send Message</button>`, }) export class ChildComponent { @Input() childMessage: string; @Output() messageEvent = new EventEmitter<string>(); sendMessage() { this.messageEvent.emit('Message from child!'); } } </string>
2.サービスベースのコミュニケーション
コンポーネントが親子関係にない場合、Angular サービスはデータとロジックを共有する簡単な方法を提供します。サービスはデフォルトでシングルトンです。つまり、アプリ全体でインスタンスが 1 つだけ存在します。
<!-- parent.component.html --> <app-child></app-child>
さまざまなコンポーネントでのサービスの使用:
import { Injectable } from '@angular/core'; import { BehaviorSubject } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class SharedService { private messageSource = new BehaviorSubject<string>('Default Message'); currentMessage = this.messageSource.asObservable(); changeMessage(message: string) { this.messageSource.next(message); } } </string>
// component-one.ts import { Component } from '@angular/core'; import { SharedService } from '../shared.service'; @Component({ selector: 'app-component-one', template: `<button>Change Message</button>`, }) export class ComponentOne { constructor(private sharedService: SharedService) {} changeMessage() { this.sharedService.changeMessage('Hello from Component One'); } }
Angular コンポーネントのベスト プラクティス
- 単一の責任: 可読性と保守性を向上させるために、各コンポーネントが 1 つの責任を持つようにします。
- 機能モジュール: 関連するコンポーネントを機能モジュールに整理します。これにより、遅延読み込みが容易になります。
- 変更検出の最適化: パフォーマンスを向上させるために、頻繁に更新されないコンポーネントには OnPush 変更検出を使用します。
- 通信のためのサービスの使用を制限する: サービスはデータの共有に役立ちますが、サービスに過度に依存するとコードが密結合になる可能性があります。可能な限り、親子通信には @Input と @Output を使用してください。
- テンプレートの簡素化: テンプレートを可能な限り単純にし、複雑なロジックをコンポーネント クラスに移動します。
結論
Angular コンポーネントは、スケーラブルなモジュール型アプリケーションの構築の中核です。構造、ライフサイクル、通信方法を理解することで、理解しやすく、構築しやすい効率的で保守可能なアプリケーションを作成できます。
次の記事では、Angular コンポーネントのライフサイクルをさらに詳しく説明し、各フックとそれを使用してコンポーネントを効果的に管理する方法を検討します。 Angular の強力なライフサイクル機能をさらに詳しく見ていきますので、ご期待ください!
以上がAngular のコンポーネントを理解するための基本ガイドの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
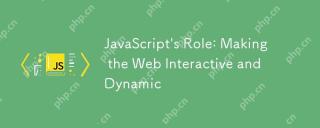
JavaScriptは、Webページのインタラクティブ性とダイナミズムを向上させるため、現代のWebサイトの中心にあります。 1)ページを更新せずにコンテンツを変更できます。2)Domapiを介してWebページを操作する、3)アニメーションやドラッグアンドドロップなどの複雑なインタラクティブ効果、4)ユーザーエクスペリエンスを改善するためのパフォーマンスとベストプラクティスを最適化します。
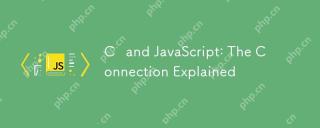
CおよびJavaScriptは、WebAssemblyを介して相互運用性を実現します。 1)CコードはWebAssemblyモジュールにコンパイルされ、JavaScript環境に導入され、コンピューティングパワーが強化されます。 2)ゲーム開発では、Cは物理エンジンとグラフィックスレンダリングを処理し、JavaScriptはゲームロジックとユーザーインターフェイスを担当します。
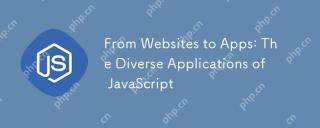
JavaScriptは、Webサイト、モバイルアプリケーション、デスクトップアプリケーション、サーバー側のプログラミングで広く使用されています。 1)Webサイト開発では、JavaScriptはHTMLおよびCSSと一緒にDOMを運用して、JQueryやReactなどのフレームワークをサポートします。 2)ReactNativeおよびIonicを通じて、JavaScriptはクロスプラットフォームモバイルアプリケーションを開発するために使用されます。 3)電子フレームワークにより、JavaScriptはデスクトップアプリケーションを構築できます。 4)node.jsを使用すると、JavaScriptがサーバー側で実行され、高い並行リクエストをサポートします。
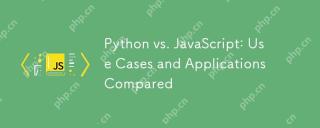
Pythonはデータサイエンスと自動化により適していますが、JavaScriptはフロントエンドとフルスタックの開発により適しています。 1. Pythonは、データ処理とモデリングのためにNumpyやPandasなどのライブラリを使用して、データサイエンスと機械学習でうまく機能します。 2。Pythonは、自動化とスクリプトにおいて簡潔で効率的です。 3. JavaScriptはフロントエンド開発に不可欠であり、動的なWebページと単一ページアプリケーションの構築に使用されます。 4. JavaScriptは、node.jsを通じてバックエンド開発において役割を果たし、フルスタック開発をサポートします。
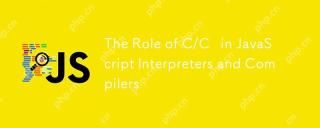
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。
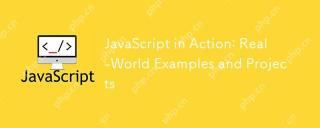
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
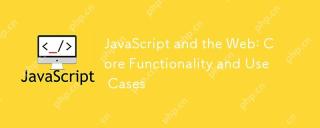
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
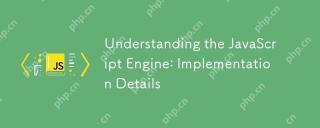
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール
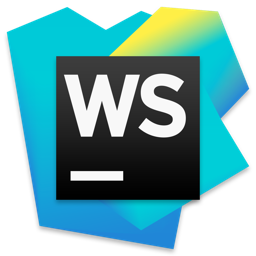
WebStorm Mac版
便利なJavaScript開発ツール

メモ帳++7.3.1
使いやすく無料のコードエディター

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、

ホットトピック









