Python 3 で Web からファイルをダウンロードする
JAR ファイルをダウンロードするために JAD ファイルから URL を抽出すると、URL が文字列として保存されているためにエラーが発生しますタイプ。この問題を解決するために、Python 3 で文字列 URL を持つファイルをダウンロードする方法を検討します。
Web ページのコンテンツの取得:
Web ページのコンテンツを取得するには変数の場合、urllib.request.urlopen を使用して応答を読み取ることができます:
<code class="python">import urllib.request url = 'http://example.com/' response = urllib.request.urlopen(url) data = response.read() # bytes object text = data.decode('utf-8') # str object</code>
ファイルのダウンロードと保存:
簡単にファイルをダウンロードして保存するには、urllib .request.urlretrieve が最適です:
<code class="python">import urllib.request # Download and save file from url to file_name urllib.request.urlretrieve(url, file_name)</code>
または、ローカル パスと応答ヘッダーを取得できます:
<code class="python">file_name, headers = urllib.request.urlretrieve(url)</code>
urlopen と shutil.copyfileobj を使用した最適な解決策:
推奨されるアプローチは、urllib.request.urlopen を利用してファイルのような HTTP 応答オブジェクトを取得し、shutil.copyfileobj:
<code class="python">import urllib.request import shutil # Download and save file from url to file_name with urllib.request.urlopen(url) as response, open(file_name, 'wb') as out_file: shutil.copyfileobj(response, out_file)</code>
小さいファイルの代替アプローチ:
小さいファイルの場合、ダウンロード全体をバイト オブジェクトに保存してファイルに書き込むことができます:<code class="python">import urllib.request # Download and save file from url to file_name with urllib.request.urlopen(url) as response, open(file_name, 'wb') as out_file: data = response.read() # bytes object out_file.write(data)</code>
圧縮データをその場で抽出する:
HTTP サーバーがランダム ファイル アクセスをサポートしている場合は、圧縮データをその場で抽出することもできます:以上がPython 3 を使用して Web からファイルをダウンロードするにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
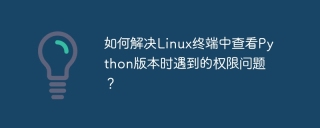
LinuxターミナルでPythonバージョンを表示する際の許可の問題の解決策PythonターミナルでPythonバージョンを表示しようとするとき、Pythonを入力してください...
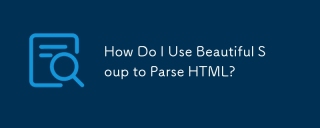
この記事では、Pythonライブラリである美しいスープを使用してHTMLを解析する方法について説明します。 find()、find_all()、select()、およびget_text()などの一般的な方法は、データ抽出、多様なHTML構造とエラーの処理、および代替案(SEL
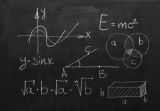
Pythonの統計モジュールは、強力なデータ統計分析機能を提供して、生物統計やビジネス分析などのデータの全体的な特性を迅速に理解できるようにします。データポイントを1つずつ見る代わりに、平均や分散などの統計を見て、無視される可能性のある元のデータの傾向と機能を発見し、大きなデータセットをより簡単かつ効果的に比較してください。 このチュートリアルでは、平均を計算し、データセットの分散の程度を測定する方法を説明します。特に明記しない限り、このモジュールのすべての関数は、単に平均を合計するのではなく、平均()関数の計算をサポートします。 浮動小数点数も使用できます。 ランダムをインポートします インポート統計 fractiから
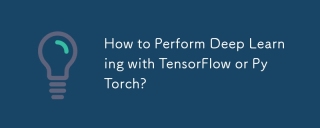
この記事では、深い学習のためにTensorflowとPytorchを比較しています。 関連する手順、データの準備、モデルの構築、トレーニング、評価、展開について詳しく説明しています。 特に計算グラップに関して、フレームワーク間の重要な違い
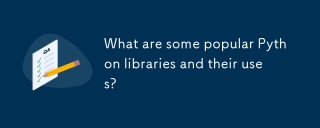
この記事では、numpy、pandas、matplotlib、scikit-learn、tensorflow、django、flask、and requestsなどの人気のあるPythonライブラリについて説明し、科学的コンピューティング、データ分析、視覚化、機械学習、Web開発、Hの使用について説明します。
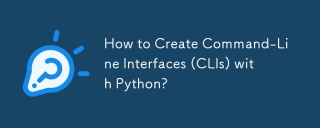
この記事では、コマンドラインインターフェイス(CLI)の構築に関するPython開発者をガイドします。 Typer、Click、Argparseなどのライブラリを使用して、入力/出力の処理を強調し、CLIの使いやすさを改善するためのユーザーフレンドリーな設計パターンを促進することを詳述しています。

PythonのPandasライブラリを使用する場合、異なる構造を持つ2つのデータフレーム間で列全体をコピーする方法は一般的な問題です。 2つのデータがあるとします...
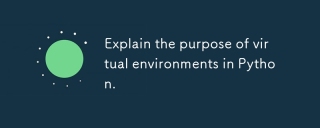
この記事では、Pythonにおける仮想環境の役割について説明し、プロジェクトの依存関係の管理と競合の回避に焦点を当てています。プロジェクト管理の改善と依存関係の問題を減らすための作成、アクティベーション、およびメリットを詳しく説明しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール
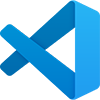
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。
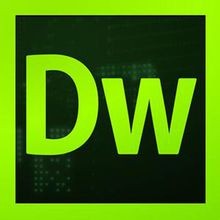
ドリームウィーバー CS6
ビジュアル Web 開発ツール
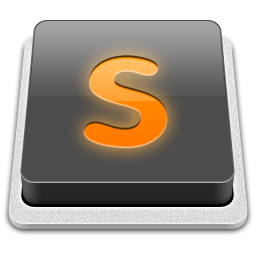
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック



