Foreach ループでのラムダ式の失敗
デリゲートのリストを初期化する次のコードを考えます。各デリゲートは、特定の種類と挨拶を指定してメソッド SayGreetingToType を呼び出します。
<code class="csharp">public class MyClass { public delegate string PrintHelloType(string greeting); public void Execute() { Type[] types = new Type[] { typeof(string), typeof(float), typeof(int) }; List<printhellotype> helloMethods = new List<printhellotype>(); foreach (var type in types) { // Initialize the lambda expression with the captured variable 'type' var sayHello = new PrintHelloType(greeting => SayGreetingToType(type, greeting)); helloMethods.Add(sayHello); } // Call the delegates with the greeting "Hi" foreach (var helloMethod in helloMethods) { Console.WriteLine(helloMethod("Hi")); } } public string SayGreetingToType(Type type, string greetingText) { return greetingText + " " + type.Name; } }</printhellotype></printhellotype></code>
このコードを実行すると、次の結果が表示されると予想されます。
Hi String Hi Single Hi Int32
ただし、クロージャーの動作により、types 配列の最後の型 Int32 はすべてのラムダによってキャプチャされます。表現。その結果、すべてのデリゲートが同じ型で SayGreetingToType を呼び出し、次のような予期しない出力が発生します。
Hi Int32 Hi Int32 Hi Int32
解決策
この問題を解決するには、次のことが必要です。変数自体ではなくラムダ式内のループ変数の値をキャプチャするには:
<code class="csharp">public class MyClass { public delegate string PrintHelloType(string greeting); public void Execute() { Type[] types = new Type[] { typeof(string), typeof(float), typeof(int) }; List<printhellotype> helloMethods = new List<printhellotype>(); foreach (var type in types) { // Capture a copy of the current 'type' value using a new variable var newType = type; // Initialize the lambda expression with the new variable 'newType' var sayHello = new PrintHelloType(greeting => SayGreetingToType(newType, greeting)); helloMethods.Add(sayHello); } // Call the delegates with the greeting "Hi" foreach (var helloMethod in helloMethods) { Console.WriteLine(helloMethod("Hi")); } } public string SayGreetingToType(Type type, string greetingText) { return greetingText + " " + type.Name; } }</printhellotype></printhellotype></code>
この変更により、各ラムダ式が型の独自のコピーを持つことが保証され、正しい値で SayGreetingToType を呼び出すことができるようになります。型引数。
以上がForeach ループ内のラムダ式がループ変数の最後の値をキャプチャするのはなぜですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
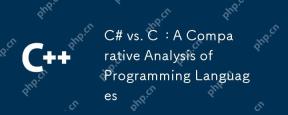
C#とCの主な違いは、構文、メモリ管理、パフォーマンスです。1)C#構文は最新であり、LambdaとLinqをサポートし、CはC機能を保持し、テンプレートをサポートします。 2)C#はメモリを自動的に管理し、Cは手動で管理する必要があります。 3)CパフォーマンスはC#よりも優れていますが、C#パフォーマンスも最適化されています。
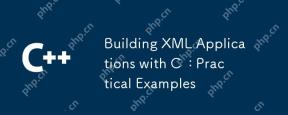
tinyxml、pugixml、またはlibxml2ライブラリを使用して、CでXMLデータを処理できます。1)XMLファイルを解析する:DOMまたはSAXメソッドを使用し、DOMは小さなファイルに適しており、SAXは大きなファイルに適しています。 2)XMLファイルを生成:データ構造をXML形式に変換し、ファイルに書き込みます。これらの手順を通じて、XMLデータを効果的に管理および操作できます。
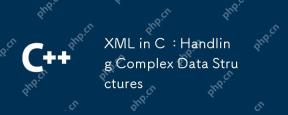
CのXMLデータ構造を使用すると、TinyXMLまたはPUGIXMLライブラリを使用できます。 1)PUGIXMLライブラリを使用して、XMLファイルを解析して生成します。 2)本情報などの複雑なネストされたXML要素を処理します。 3)XML処理コードを最適化し、効率的なライブラリとストリーミング解析を使用することをお勧めします。これらの手順を通じて、XMLデータを効率的に処理できます。
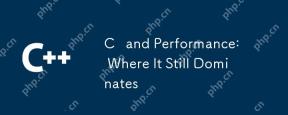
Cは、低レベルのメモリ管理と効率的な実行機能により、ゲーム開発、金融取引システム、組み込みシステムに不可欠であるため、パフォーマンスの最適化を支配しています。具体的には、次のように現れます。1)ゲーム開発では、Cの低レベルのメモリ管理と効率的な実行機能により、ゲームエンジン開発に適した言語になります。 2)金融取引システムでは、Cのパフォーマンスの利点は、非常に低いレイテンシと高スループットを保証します。 3)組み込みシステムでは、Cの低レベルのメモリ管理と効率的な実行機能により、リソースに制約のある環境で非常に人気があります。
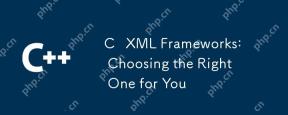
C XMLフレームワークの選択は、プロジェクトの要件に基づいている必要があります。 1)TinyXMLは、リソースに制約のある環境に適しています。2)PUGIXMLは高性能要件に適しています。
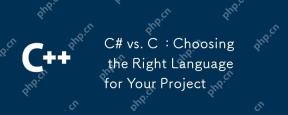
C#は、開発効率とタイプの安全性を必要とするプロジェクトに適していますが、Cは高性能とハードウェア制御を必要とするプロジェクトに適しています。 1)C#は、エンタープライズアプリケーションやWindows開発に適したGarbage CollectionとLINQを提供します。 2)Cは、その高性能と根本的な制御で知られており、ゲームやシステムのプログラミングで広く使用されています。
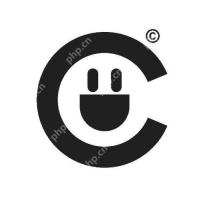
Cコードの最適化は、次の戦略を通じて実現できます。1。最適化のためにメモリを手動で管理する。 2。コンパイラ最適化ルールに準拠したコードを書きます。 3.適切なアルゴリズムとデータ構造を選択します。 4.インライン関数を使用して、コールオーバーヘッドを削減します。 5.コンパイル時に最適化するために、テンプレートメタプログラムを適用します。 6.不要なコピーを避け、移動セマンティクスと参照パラメーターを使用します。 7. constを正しく使用して、コンパイラの最適化を支援します。 8。std :: vectorなどの適切なデータ構造を選択します。
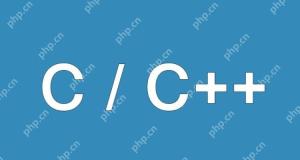
Cの揮発性キーワードは、変数の値がコード制御の外側に変更され、したがって最適化できないことをコンパイラに通知するために使用されます。 1)センサー状態などのハードウェアまたは割り込みサービスプログラムによって変更される可能性のある変数の読み取りによく使用されます。 2)揮発性は、マルチスレッドの安全性を保証することはできず、Mutexロックまたは原子操作を使用する必要があります。 3)揮発性を使用すると、パフォーマンスがわずかに減少する可能性がありますが、プログラムの正確性を確保します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。
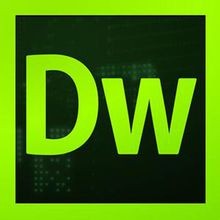
ドリームウィーバー CS6
ビジュアル Web 開発ツール

メモ帳++7.3.1
使いやすく無料のコードエディター

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。
