TypeScript の型システムは強力ですが、エラー メッセージが難解で理解しにくい場合があります。この記事では、構築不可能な型を使用して明確で説明的なコンパイル時例外を作成するパターンを検討します。このアプローチは、有用なエラー メッセージで無効な状態を表現できないようにすることで、実行時エラーを防ぐのに役立ちます。
パターン: カスタム メッセージを含む構築不可能な型
まず、核となるパターンを分析してみましょう:
// Create a unique symbol for our type exception declare const TypeException: unique symbol; // Basic type definitions type Struct = Record<string any>; type Funct<t r> = (arg: T) => R; type Types<t> = keyof T & string; type Sanitize<t> = T extends string ? T : never; // The core pattern for type-level exceptions export type Unbox<t extends struct> = { [Type in Types<t>]: T[Type] extends Funct<any infer ret> ? (arg: Ret) => any : T[Type] extends Struct ? { [TypeException]: `Variant }> is of type <union>. Migrate logic to <none> variant to capture }> types.`; } : (value: T[Type]) => any; }; </none></union></any></t></t></t></t></t></string>
仕組み
- TypeException は、エラー メッセージの特別なキーとして機能する一意のシンボルです
- 無効な型状態に遭遇した場合、TypeException プロパティを持つオブジェクト型を返します
- この型は実行時に構築できないため、TypeScript にカスタム エラー メッセージが表示されます
- エラー メッセージには、テンプレート リテラルを使用して型情報を含めることができます
例 1: カスタム エラーによるバリアントの処理
バリアント型でこのパターンを使用する方法を示す例を次に示します。
type DataVariant = | { type: 'text'; content: string } | { type: 'number'; value: number } | { type: 'complex'; nested: { data: string } }; type VariantHandler = Unbox void; number: (value: number) => void; complex: { // This will trigger our custom error [TypeException]: `Variant <complex> is of type <union>. Migrate logic to <none> variant to capture <complex> types.` }; }>; // This will show our custom error at compile time const invalidHandler: VariantHandler = { text: (content) => console.log(content), number: (value) => console.log(value), complex: (nested) => console.log(nested) // Error: Type has unconstructable signature }; </complex></none></union></complex>
例 2: 再帰的な型検証
これは、再帰型でパターンを使用する方法を示す、より複雑な例です。
type TreeNode<t> = { value: T; children?: TreeNode<t>[]; }; type TreeHandler<t> = Unbox void; node: TreeNode<t> extends Struct ? { [TypeException]: `Cannot directly handle node type. Use leaf handler for individual values.`; } : never; }>; // Usage example - will show custom error const invalidTreeHandler: TreeHandler<string> = { leaf: (value) => console.log(value), node: (node) => console.log(node) // Error: Cannot directly handle node type }; </string></t></t></t></t>
例 3: 型状態の検証
パターンを使用して有効な型の状態遷移を強制する方法は次のとおりです。
type LoadingState<t> = { idle: null; loading: null; error: Error; success: T; }; type StateHandler<t> = Unbox void; loading: () => void; error: (error: Error) => void; success: (data: T) => void; // Prevent direct access to state object state: LoadingState<t> extends Struct ? { [TypeException]: `Cannot access state directly. Use individual handlers for each state.`; } : never; }>; // This will trigger our custom error const invalidStateHandler: StateHandler<string> = { idle: () => {}, loading: () => {}, error: (e) => console.error(e), success: (data) => console.log(data), state: (state) => {} // Error: Cannot access state directly }; </string></t></t></t>
このパターンを使用する場合
このパターンは、次の場合に特に役立ちます。
- コンパイル時に特定の型の組み合わせを防ぐ必要があります
- 型違反について明確で説明的なエラー メッセージを提供したいと考えています
- 特定の操作を制限する必要がある複雑なタイプの階層を構築しています
- 役立つエラー メッセージを表示して、開発者を正しい使用パターンに導く必要があります
技術的な詳細
パターンが内部的にどのように機能するかを詳しく見てみましょう:
// Create a unique symbol for our type exception declare const TypeException: unique symbol; // Basic type definitions type Struct = Record<string any>; type Funct<t r> = (arg: T) => R; type Types<t> = keyof T & string; type Sanitize<t> = T extends string ? T : never; // The core pattern for type-level exceptions export type Unbox<t extends struct> = { [Type in Types<t>]: T[Type] extends Funct<any infer ret> ? (arg: Ret) => any : T[Type] extends Struct ? { [TypeException]: `Variant }> is of type <union>. Migrate logic to <none> variant to capture }> types.`; } : (value: T[Type]) => any; }; </none></union></any></t></t></t></t></t></string>
従来のアプローチに勝る利点
- エラー メッセージをクリア: TypeScript のデフォルトの型エラーの代わりに、何が問題になったのかを正確に説明するカスタム メッセージが表示されます
- コンパイル時の安全性: すべてのエラーは実行時ではなく開発中に捕捉されます
- 自己文書化: エラー メッセージには、問題の解決方法に関する指示が含まれる場合があります
- タイプ セーフ: 開発者エクスペリエンスを向上させながら、完全なタイプ セーフを維持します
- 実行時コストゼロ: すべてのチェックは実行時のオーバーヘッドなしでコンパイル時に行われます
結論
カスタム エラー メッセージで構築不可能な型を使用することは、自己文書化型制約を作成するための強力なパターンです。 TypeScript の型システムを活用してコンパイル時に明確なガイダンスを提供し、開発者が実行時の問題になる前に問題を見つけて修正できるようにします。
このパターンは、特定の組み合わせが無効である必要がある複雑な型システムを構築する場合に特に役立ちます。無効な状態を表現できないようにし、明確なエラー メッセージを提供することで、より保守しやすく開発者にとって使いやすい TypeScript コードを作成できます。
以上が構築不可能な型を使用した TypeScript での豊富なコンパイル時例外の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
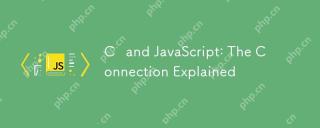
CおよびJavaScriptは、WebAssemblyを介して相互運用性を実現します。 1)CコードはWebAssemblyモジュールにコンパイルされ、JavaScript環境に導入され、コンピューティングパワーが強化されます。 2)ゲーム開発では、Cは物理エンジンとグラフィックスレンダリングを処理し、JavaScriptはゲームロジックとユーザーインターフェイスを担当します。
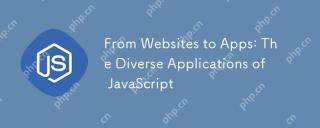
JavaScriptは、Webサイト、モバイルアプリケーション、デスクトップアプリケーション、サーバー側のプログラミングで広く使用されています。 1)Webサイト開発では、JavaScriptはHTMLおよびCSSと一緒にDOMを運用して、JQueryやReactなどのフレームワークをサポートします。 2)ReactNativeおよびIonicを通じて、JavaScriptはクロスプラットフォームモバイルアプリケーションを開発するために使用されます。 3)電子フレームワークにより、JavaScriptはデスクトップアプリケーションを構築できます。 4)node.jsを使用すると、JavaScriptがサーバー側で実行され、高い並行リクエストをサポートします。
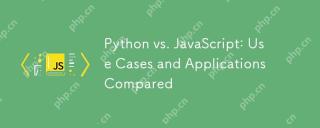
Pythonはデータサイエンスと自動化により適していますが、JavaScriptはフロントエンドとフルスタックの開発により適しています。 1. Pythonは、データ処理とモデリングのためにNumpyやPandasなどのライブラリを使用して、データサイエンスと機械学習でうまく機能します。 2。Pythonは、自動化とスクリプトにおいて簡潔で効率的です。 3. JavaScriptはフロントエンド開発に不可欠であり、動的なWebページと単一ページアプリケーションの構築に使用されます。 4. JavaScriptは、node.jsを通じてバックエンド開発において役割を果たし、フルスタック開発をサポートします。
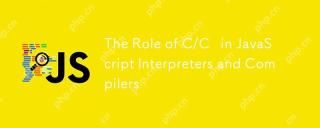
CとCは、主に通訳者とJITコンパイラを実装するために使用されるJavaScriptエンジンで重要な役割を果たします。 1)cは、JavaScriptソースコードを解析し、抽象的な構文ツリーを生成するために使用されます。 2)Cは、Bytecodeの生成と実行を担当します。 3)Cは、JITコンパイラを実装し、実行時にホットスポットコードを最適化およびコンパイルし、JavaScriptの実行効率を大幅に改善します。
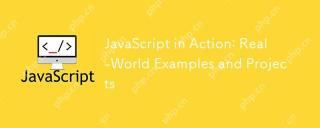
現実世界でのJavaScriptのアプリケーションには、フロントエンドとバックエンドの開発が含まれます。 1)DOM操作とイベント処理を含むTODOリストアプリケーションを構築して、フロントエンドアプリケーションを表示します。 2)node.jsを介してRestfulapiを構築し、バックエンドアプリケーションをデモンストレーションします。
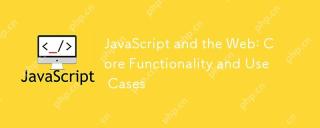
Web開発におけるJavaScriptの主な用途には、クライアントの相互作用、フォーム検証、非同期通信が含まれます。 1)DOM操作による動的なコンテンツの更新とユーザーインタラクション。 2)ユーザーエクスペリエンスを改善するためにデータを提出する前に、クライアントの検証が実行されます。 3)サーバーとのリフレッシュレス通信は、AJAXテクノロジーを通じて達成されます。
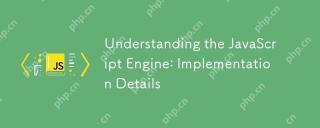
JavaScriptエンジンが内部的にどのように機能するかを理解することは、開発者にとってより効率的なコードの作成とパフォーマンスのボトルネックと最適化戦略の理解に役立つためです。 1)エンジンのワークフローには、3つの段階が含まれます。解析、コンパイル、実行。 2)実行プロセス中、エンジンはインラインキャッシュや非表示クラスなどの動的最適化を実行します。 3)ベストプラクティスには、グローバル変数の避け、ループの最適化、constとletsの使用、閉鎖の過度の使用の回避が含まれます。

Pythonは、スムーズな学習曲線と簡潔な構文を備えた初心者により適しています。 JavaScriptは、急な学習曲線と柔軟な構文を備えたフロントエンド開発に適しています。 1。Python構文は直感的で、データサイエンスやバックエンド開発に適しています。 2。JavaScriptは柔軟で、フロントエンドおよびサーバー側のプログラミングで広く使用されています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

SublimeText3 中国語版
中国語版、とても使いやすい

MinGW - Minimalist GNU for Windows
このプロジェクトは osdn.net/projects/mingw に移行中です。引き続きそこでフォローしていただけます。 MinGW: GNU Compiler Collection (GCC) のネイティブ Windows ポートであり、ネイティブ Windows アプリケーションを構築するための自由に配布可能なインポート ライブラリとヘッダー ファイルであり、C99 機能をサポートする MSVC ランタイムの拡張機能が含まれています。すべての MinGW ソフトウェアは 64 ビット Windows プラットフォームで実行できます。

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール
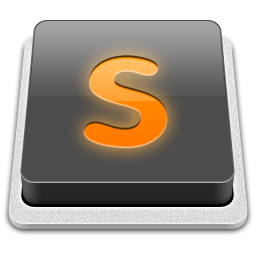
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)
