這個想法
這個想法是為 DEA 特工創建一個簡單的平台,來管理《絕命毒師》/《風騷律師》宇宙中角色的資訊。為了讓 DEA 特工的工作變得更輕鬆,他們需要一個 API 端點,允許根據人物的姓名、出生日期、職業或他們是否是嫌疑人的事實來過濾有關人物的信息。
緝毒局試圖將毒梟關進監獄,他們正在追蹤他們以及他們所在位置周圍的人。它們將時間戳記和特定位置作為地理座標儲存在相關表中。將公開資料的端點需要允許過濾距離特定地理點特定距離內的位置條目,以及它們被指派給誰,以及記錄它們的日期時間範圍。此端點的排序應允許考慮距指定地理點的距離,升序和降序。
要了解它是如何完成的,您可以按照下面的文件在本地設定此項目並自行測試。
您可以在我的 GitHub 儲存庫中找到程式碼。
設定項目
作為先決條件,您需要在系統上安裝 Docker 和 docker-compose。
首先,前往您的專案資料夾並複製 Breaking Bad API 儲存庫:
git克隆git@github.com:drangovski/writing-bad-api.git
cd 破壞-bad-api
然後您需要建立 .env 文件,在其中放置以下變數的值:
POSTGRES_USER=heisenberg POSTGRES_PASSWORD=iamthedanger POSTGRES_DB=breakingbad DEBUG=True SECRET_KEY="<secret key>" DJANGO_ALLOWED_HOSTS=localhost 127.0.0.1 [::1] SQL_ENGINE=django.db.backends.postgresql SQL_DATABASE=breakingbad SQL_USER=heisenberg SQL_PASSWORD=iamthedanger SQL_HOST=db<br> SQL_PORT=5432 </secret>
注意:如果需要,您可以使用 env_generator.sh 檔案為您建立 .env 檔案。這也會自動產生SECRET_KEY。要運行此文件,請先使用 chmod x env_generator.sh 授予其權限,然後使用 ./env_generator.sh
運行它
一旦你有了這套,你就可以運行:
docker-compose 建置
docker-compose up
這將在 localhost:8000 啟動 Django 應用程式。要存取 API,URL 將為 localhost:8000/api。
模擬地點
為了這些項目的主題(最終,為了讓你的生活更輕鬆一點:)),你最終可以使用以下位置及其坐標:
Location | Longitude | Latitude |
---|---|---|
Los Pollos Hermanos | 35.06534619552971 | -106.64463423464572 |
Walter White House | 35.12625330483283 | -106.53566597939896 |
Saul Goodman Office | 35.12958969793146 | -106.53106126774908 |
Mike Ehrmantraut House | 35.08486667169461 | -106.64115047513016 |
Jessie Pinkman House | 35.078341181544396 | -106.62404891988452 |
Hank & Marrie House | 35.13512843853582 | -106.48159991250327 |
import requests import json url = 'http://localhost:8000/api/characters/' headers = {'Content-Type' : "application/json"} response = requests.get(url, headers=headers, verify=False) if response.status_code == 200: data = response.json() print(json.dumps(data, indent=2)) else: print("Request failed with status code:", response.status_code)
人物
檢索所有字符
檢索資料庫中所有現有的字元。
取得/api/字元/
[ { "id": 1, "name": "Walter White", "occupation": "Chemistry Professor", "date_of_birth": "1971", "suspect": false }, { "id": 2, "name": "Tuco Salamanca", "occupation": "Grandpa Keeper", "date_of_birth": "1976", "suspect": true } ]
檢索單一字符
要檢索單一角色,請將角色的 ID 傳遞給 enpoint。
GET /api/characters/{id}
創建一個新角色
您可以使用 POST 方法到 /characters/ 端點來建立新角色。
POST /api/characters/
建立參數
您需要在查詢中傳遞以下參數,才能成功建立角色:
{ "name": "string", "occupation": "string", "date_of_birth": "string", "suspect": boolean }
Parameter | Description |
---|---|
name | String value for the name of the character. |
occupation | String value for the occupation of the character. |
date_of_birth | String value for the date of brith. |
suspect | Boolean parameter. True if suspect, False if not. |
Character ordering
Ordering of the characters can be done by two fields as parameters: name and date_of_birth
GET /api/characters/?ordering={name / date_of_birth}
Parameter | Description |
---|---|
name | Order the results by the name field. |
date_of_birth | Order the results by the date_of_birth field. |
Additionally, you can add the parameter ascending with a value 1 or 0 to order the results in ascending or descending order.
GET /api/characters/?ordering={name / date_of_birth}&ascending={1 / 0}
Parameter | Description |
---|---|
&ascending=1 | Order the results in ascending order by passing 1 as a value. |
&ascending=0 | Order the results in descending order by passing 0 as a value. |
Character filtering
To filter the characters, you can use the parameters in the table below. Case insensitive.
GET /api/characters/?name={text}
Parameter | Description |
---|---|
/?name={text} | Filter the results by name. It can be any length and case insensitive. |
/?occupaton={text} | Filter the results by occupation. It can be any length and case insensitive. |
/?suspect={True / False} | Filter the results by suspect status. It can be True or False. |
Character search
You can also use the search parameter in the query to search characters and retrieve results based on the fields listed below.
GET /api/characters/?search={text}
name
occupation
date_of_birth
Update a character
To update a character, you will need to pass the {id} of a character to the URL and make a PUT method request with the parameters in the table below.
PUT /api/characters/{id}
{ "name": "Mike Ehrmantraut", "occupation": "Retired Officer", "date_of_birth": "1945", "suspect": false }
Parameter | Description |
---|---|
name | String value for the name of the character. |
occupation | String value for the occupation of the character. |
date_of_birth | String value for the date of birth. |
suspect | Boolean parameter. True if suspect, False if not. |
Delete a character
To delete a character, you will need to pass the {id} of a character to the URL and make DELETE method request.
DELETE /api/characters/{id}
Locations
Retrieve all locations
To retrieves all existing locations in the database.
GET /api/locations/
[ { "id": 1, "name": "Los Pollos Hermanos", "longitude": 35.065442792232716, "latitude": -106.6444840309555, "created": "2023-02-09T22:04:32.441106Z", "character": { "id": 2, "name": "Tuco Salamanca", "details": "http://localhost:8000/api/characters/2" } }, ]
Retrieve a single location
To retrieve a single location, pass the locations ID to the endpoint.
GET /api/locations/{id}
Create a new location
You can use the POST method to /locations/ endpoint to create a new location.
POST /api/locations/
Creation parameters
You will need to pass the following parameters in the query, to successfully create a location:
{ "name": "string", "longitude": float, "latitude": float, "character": integer }
Parameter | Description |
---|---|
name | The name of the location. |
longitude | Longitude of the location. |
latitude | Latitude of the location. |
character | This is the id of a character. It is basically ForeignKey relation to the Character model. |
Note: Upon creation of an entry, the Longitude and the Latitude will be converted to a PointField() type of field in the model and stored as a calculated geographical value under the field coordinates, in order for the location coordinates to be eligible for GeoDjango operations.
Location ordering
Ordering of the locations can be done by providing the parameters for the longitude and latitude coordinates for a single point, and a radius (in meters). This will return all of the locations stored in the database, that are in the provided radius from the provided point (coordinates).
GET /api/locations/?longitude={longitude}&latitude={latitude}&radius={radius}
Parameter | Description |
---|---|
longitude | The longitude parameter of the radius point. |
latitude | The latitude parameter of the radius point. |
radius | The radius parameter (in meters). |
Additionally, you can add the parameter ascending with values 1 or 0 to order the results in ascending or descending order.
GET /api/locations/?longitude={longitude}&latitude={latitude}&radius={radius}&ascending={1 / 0}
Parameter | Description |
---|---|
&ascending=1 | Order the results in ascending order by passing 1 as a value. |
&ascending=0 | Order the results in descending order by passing 0 as a value. |
Locaton filtering
To filter the locations, you can use the parameters in the table below. Case insensitive.
GET /api/locations/?character={text}
Parameter | Description |
---|---|
/?name={text} | Filter the results by location name. It can be any length and case insensitive. |
/?character={text} | Filter the results by character. It can be any length and case insensitive. |
/?created={timeframe} | Filter the results by when they were created. Options: today, yesterday, week, month & year. |
Note: You can combine filtering parameters with ordering parameters. Just keep in mind that if you filter by any of these fields above and want to use the ordering parameters, you will always need to pass longitude, latitude and radius altogether. Additionally, if you need to use ascending parameter for ordering, this parameter can't be passed without longitude, latitude and radius as well.
Update a location
To update a location, you will need to pass the {id} of locations to the URL and make a PUT method request with the parameters in the table below.
PUT /api/locations/{id}
{ "id": 1, "name": "Los Pollos Hermanos", "longitude": 35.065442792232716, "latitude": -106.6444840309555, "created": "2023-02-09T22:04:32.441106Z", "character": { "id": 2, "name": "Tuco Salamanca", "occupation": "Grandpa Keeper", "date_of_birth": "1975", "suspect": true } }
Parameter | Description |
---|---|
name | String value for the name of the location. |
longitude | Float value for the longitude of the location. |
latitude | Float value for the latitude of the location. |
Delete a location
To delete a location, you will need to pass the {id} of a location to the URL and make a DELETE method request.
DELETE /api/locations/{id}
以上がDjango Rest Framework を使用したハイゼンベルクの狩猟の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
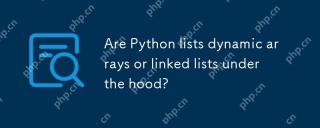
PythonListsareimplementedasdynamicarrays、notlinkedlists.1)they restorediguourmemoryblocks、それはパフォーマンスに影響を与えることに影響を与えます
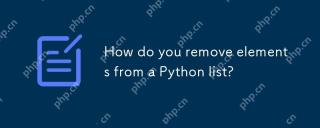
pythonoffersfourmainmethodstoremoveelements fromalist:1)removesthefirstoccurrenceofavalue、2)pop(index(index(index)removes regvess returnsaspecifiedindex、3)delstatementremoveselementselementsbyindexorseLice、および4)clear()
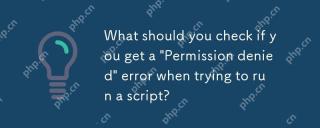
toresolvea "許可denided" errors whenrunningascript、sofflowthesesteps:1)checkandadaddadaddadadaddaddadadadaddadaddadaddadaddaddaddaddaddadaddadaddaddaddaddadaddaddaddadadaddadaddadaddadadisionsisingmod xmyscript.shtomakeitexexutable.2)
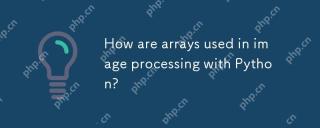
ArraySarecrucialinpythonimageprocessing asheyenable efficientmanipulation analysisofimagedata.1)画像anverttonumpyArrays、with grayscaleimagesasas2darraysandcolorimagesas.
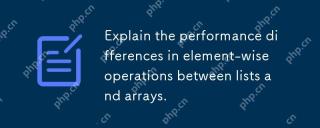
ArsareSareBetterElement-WiseOperationsduetof of ActassandoptimizedImplementations.1)ArrayshaveContigUousMoryFordiRectAccess.2)ListSareFlexibleButSlowerDueTopotentialDynamicresizizizizing.3)
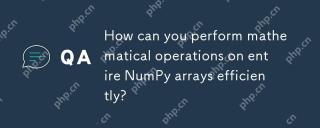
Numpyの配列全体の数学的操作は、ベクトル化された操作を通じて効率的に実装できます。 1)追加(arr 2)などの簡単な演算子を使用して、配列で操作を実行します。 2)Numpyは、基礎となるC言語ライブラリを使用して、コンピューティング速度を向上させます。 3)乗算、分割、指数などの複雑な操作を実行できます。 4)放送操作に注意して、配列の形状が互換性があることを確認します。 5)np.sum()などのnumpy関数を使用すると、パフォーマンスが大幅に向上する可能性があります。
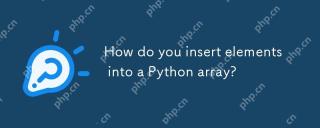
Pythonでは、要素をリストに挿入するための2つの主要な方法があります。1)挿入(インデックス、値)メソッドを使用して、指定されたインデックスに要素を挿入できますが、大きなリストの先頭に挿入することは非効率的です。 2)Append(Value)メソッドを使用して、リストの最後に要素を追加します。これは非常に効率的です。大規模なリストの場合、append()を使用するか、dequeまたはnumpy配列を使用してパフォーマンスを最適化することを検討することをお勧めします。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール
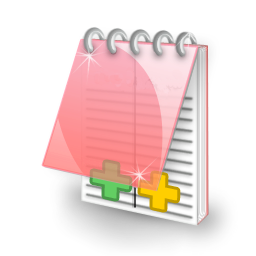
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません
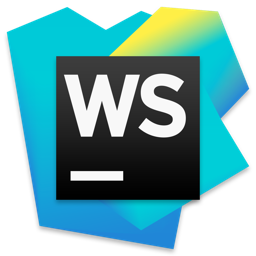
WebStorm Mac版
便利なJavaScript開発ツール

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター
