皆さん、こんにちは。今日は、sheepy という新しい単体テスト ライブラリを紹介するために来ましたが、その前に単体テストの重要性について話しましょう。このライブラリは初心者向けではありません。これを使用して単体テストを行うには、少し特別な注意を払う必要があります。エンドポイントと http エラー チェック モジュールを使用した API テストのみのアサートがあります。
Github リンク: github
PyPi リンク: pypi
実稼働環境にあるすべての成熟した自尊心のあるソフトウェアには単体テストがあり、コードにすでに含まれているものが引き続き機能するかどうかを把握するため、以前に報告され修正されているバグを防ぐため、または新機能をテストするためです。これは、彼らが物事を前進させており、技術的負債が蓄積していないことを示す良い兆候です。例として Firefox ブラウザを使用してみましょう。すべてのディレクトリには、すでに報告されているバグに対する特定のテストを含む test サブディレクトリがあります。こうすることで、修正されたバグがどこからともなく再び表示されることはなく、すでに修正されたバグが表示されることが保証されます。またどこにもないお金を捨てるということです。時間の経過とともに、少ないリソースで自分よりも優れた業績を上げている競合他社に、時間、資金、効率、市場シェアを失うことになります。
何かを行うことができないと感じる人は誰でも、その何かを中傷しようとします。単体テストも例外ではありません。各ユースケースをカバーするより良い単体テストを作成するには時間がかかります。人生のすべてのことと同じように、バックエンドの皆さん、たった 1 つのチュートリアルを読んで完璧な API を作成できたとは思えませんが、フロントエンドの皆さんも同じことです。コースを受講してここに来たとは思えません。インターフェイスを完璧にします。したがって、単体テストでは何かが変わるとは考えないでください!
アサーションメソッド
+-----------------------+-------------------------------------------------------+ | Assertion Method | Description | +-----------------------+-------------------------------------------------------+ | assertEqual(a, b) | Checks if two values are equal. | | assertNotEqual(a, b) | Checks if two values are not equal. | | assertTrue(expr) | Verifies that the expression is True. | | assertFalse(expr) | Verifies that the expression is False. | | assertRaises(exc, fn) | Asserts that a function raises a specific exception. | | assertStatusCode(resp) | Verifies if the response has the expected status code.| | assertJsonResponse(resp)| Confirms the response is in JSON format. | | assertResponseContains(resp, key) | Ensures the response contains a given key. | +-----------------------+-------------------------------------------------------+
インストール
インストールは非常に簡単です。pip がインストールされた状態で選択したターミナルを開き、「pip install shepy」と入力するだけです。
使用例
from sheepy.sheeptest import SheepyTestCase class ExampleTest(SheepyTestCase): def test_success(self): self.assertTrue(True) def test_failure(self): self.assertEqual(1, 2) def test_error(self): raise Exception("Forced error") @SheepyTestCase.skip("Reason to ignore") def test_skipped(self): pass @SheepyTestCase.expectedFailure def test_expected_failure(self): self.assertEqual(1, 2)
SheepyTestCase クラスは、単体テストを作成および実行するためのいくつかの機能を提供します。これには、アサーティブネス メソッドや、テストのスキップや予期される失敗の処理などの特別な動作を構成するためのメカニズムが含まれます。
ExampleTest クラス内では、5 つのテスト メソッドが定義されています。
test_success: このテストは、assertTrue メソッドに渡された式が true かどうかをチェックします。 True 値が明示的に渡されるため、このテストは成功します。
test_failure: このテストは、assertEqual メソッドを使用して 2 つの値間の等価性をチェックします。ただし、比較される値 1 と 2 は異なるため、テストは失敗します。これは、テストで不整合を検出する必要がある、予想される失敗のケースを示しています。
test_error: このメソッドは、「強制エラー」というメッセージを含む意図的な例外を発生させます。目標は、テスト実行中に発生するエラーに対処するときのシステムの動作をテストすることです。メソッドは例外を処理せずにスローするため、結果はテストでエラーになります。
test_skipped: このテストは SheepyTestCase クラスの Skip メソッドで装飾されています。これは、テストの実行中にスキップされることを意味します。テストをスキップした理由は「無視する理由」として提供され、この正当性は最終テストレポートに表示できます。
test_expected_failure: このメソッドは ExpectedFailure デコレータを使用し、失敗が予想されることを示します。メソッド内では 1 と 2 の間の等価性チェックがあり、通常は失敗しますが、デコレータが適用されているため、フレームワークはこの失敗を予期された動作の一部とみなし、エラーとして扱われません。 「予想される失敗」として。
出力
テスト結果:
ExampleTest.test_error: FAIL - 強制エラー
ExampleTest.test_expected_failure: 期待された失敗
例Test.test_failure: FAIL - 1 != 2
ExampleTest.test_skipped: SKIPPED -
ExampleTest.test_success: OK
API テストケース
Sheepy テスト フレームワークの API テストは、簡単かつ強力になるように設計されており、テスターは GET、POST、PUT、DELETE などの一般的な HTTP メソッドを使用して API を操作できます。このフレームワークは、HttpError 例外クラスによる組み込みエラー管理を備えた、リクエストの送信と応答の処理を簡素化する専用クラス ApiRequests を提供します。
API をテストする場合、テスト クラスは SheepyTestCase を継承します。SheepyTestCase には、API の動作を検証するためのさまざまなアサーション メソッドが装備されています。これらには、HTTP ステータス コードを検証するためのassertStatusCode、応答が JSON 形式であることを確認するためのassertJsonResponse、応答本文に特定のキーが存在するかどうかを確認するためのassertResponseContains が含まれます。
For instance, the framework allows you to send a POST request to an API, verify that the status code matches the expected value, and assert that the JSON response contains the correct data. The API requests are handled through the ApiRequests class, which takes care of constructing and sending the requests, while error handling is streamlined by raising HTTP-specific errors when the server returns unexpected status codes.
By providing built-in assertions and error handling, the framework automates much of the repetitive tasks in API testing, ensuring both correctness and simplicity in writing tests. This system allows developers to focus on verifying API behavior and logic, making it an efficient tool for ensuring the reliability of API interactions.
from sheepy.sheeptest import SheepyTestCase class TestHttpBinApi(SheepyTestCase): def __init__(self): super().__init__(base_url="https://httpbin.org") def test_get_status(self): response = self.api.get("/status/200") self.assertStatusCode(response, 200) def test_get_json(self): response = self.api.get("/json") self.assertStatusCode(response, 200) self.assertJsonResponse(response) self.assertResponseContains(response, "slideshow") def test_post_data(self): payload = {"name": "SheepyTest", "framework": "unittest"} response = self.api.post("/post", json=payload) self.assertStatusCode(response, 200) self.assertJsonResponse(response) self.assertResponseContains(response, "json") self.assertEqual(response.json()["json"], payload) def test_put_data(self): payload = {"key": "value"} response = self.api.put("/put", json=payload) self.assertStatusCode(response, 200) self.assertJsonResponse(response) self.assertResponseContains(response, "json") self.assertEqual(response.json()["json"], payload) def test_delete_resource(self): response = self.api.delete("/delete") self.assertStatusCode(response, 200) self.assertJsonResponse(response)
Output example
Test Results: TestHttpBinApi.test_delete_resource: OK TestHttpBinApi.test_get_json: OK TestHttpBinApi.test_get_status: OK TestHttpBinApi.test_post_data: OK TestHttpBinApi.test_put_data: OK
Summary:
The new sheepy library is an incredible unit testing library, which has several test accession methods, including a module just for API testing, in my opinion, it is not a library for beginners, it requires basic knowledge of object-oriented programming such as methods, classes and inheritance.
以上がShepy を使用した Python での単体テストの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
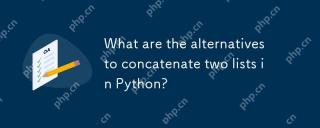
Pythonに2つのリストを接続する多くの方法があります。1。オペレーターを使用しますが、これはシンプルですが、大きなリストでは非効率的です。 2。効率的ですが、元のリストを変更する拡張メソッドを使用します。 3。=演算子を使用します。これは効率的で読み取り可能です。 4。itertools.chain関数を使用します。これはメモリ効率が高いが、追加のインポートが必要です。 5。リストの解析を使用します。これはエレガントですが、複雑すぎる場合があります。選択方法は、コードのコンテキストと要件に基づいている必要があります。
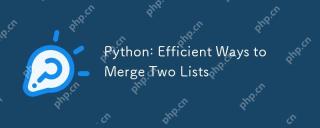
Pythonリストをマージするには多くの方法があります。1。オペレーターを使用します。オペレーターは、シンプルですが、大きなリストではメモリ効率的ではありません。 2。効率的ですが、元のリストを変更する拡張メソッドを使用します。 3. Itertools.chainを使用します。これは、大規模なデータセットに適しています。 4.使用 *オペレーター、1つのコードで小規模から中型のリストをマージします。 5. numpy.concatenateを使用します。これは、パフォーマンス要件の高い大規模なデータセットとシナリオに適しています。 6.小さなリストに適したが、非効率的な追加方法を使用します。メソッドを選択するときは、リストのサイズとアプリケーションのシナリオを考慮する必要があります。
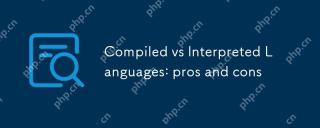
compiledlanguagesOfferspeedandsecurity、foredlanguagesprovideeaseofuseandportability.1)compiledlanguageslikec arefasterandsecurebuthavelOnderdevelopmentsplat dependency.2)
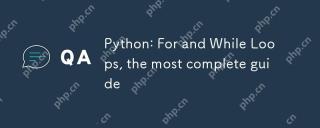
Pythonでは、forループは反復可能なオブジェクトを通過するために使用され、条件が満たされたときに操作を繰り返し実行するためにしばらくループが使用されます。 1)ループの例:リストを通過し、要素を印刷します。 2)ループの例:正しいと推測するまで、数値ゲームを推測します。マスタリングサイクルの原則と最適化手法は、コードの効率と信頼性を向上させることができます。
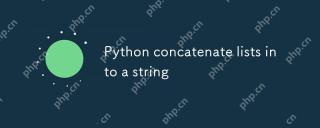
リストを文字列に連結するには、PythonのJoin()メソッドを使用して最良の選択です。 1)join()メソッドを使用して、 '' .join(my_list)などのリスト要素を文字列に連結します。 2)数字を含むリストの場合、連結する前にマップ(str、数字)を文字列に変換します。 3) '、'などの複雑なフォーマットに発電機式を使用できます。 4)混合データ型を処理するときは、MAP(STR、Mixed_List)を使用して、すべての要素を文字列に変換できるようにします。 5)大規模なリストには、 '' .join(lage_li)を使用します
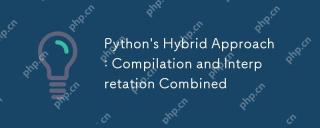
pythonusesahybridapproach、コンコイリティレーショントビテコードと解釈を組み合わせて、コードコンピレッドフォームと非依存性bytecode.2)
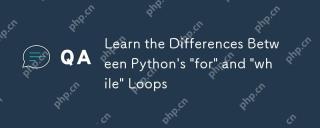
keydifferencesは、「for」と「while "loopsare:1)" for "for" loopsareideal forterating overencesonownowiterations、while2) "for" for "for" for "for" for "for" for "for" for for for for "wide" loopsarebetterunuinguntinunuinguntinisisisisisisisisisisisisisisisisisisisisisisisisisisisations.un
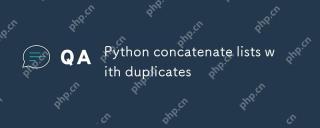
Pythonでは、さまざまな方法でリストを接続して重複要素を管理できます。1)オペレーターを使用するか、すべての重複要素を保持します。 2)セットに変換してから、リストに戻ってすべての重複要素を削除しますが、元の順序は失われます。 3)ループを使用するか、包含をリストしてセットを組み合わせて重複要素を削除し、元の順序を維持します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。
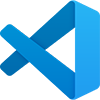
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター

メモ帳++7.3.1
使いやすく無料のコードエディター

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
