導入
Wikipedia を検索し、見つかった情報に基づいて質問に答えることができる AI エージェントを作成します。この ReAct (Reason and Act) エージェントは、Google Generative AI API を使用してクエリを処理し、応答を生成します。私たちのエージェントは次のことができるようになります:
- 関連情報については Wikipedia を検索してください。
- Wikipedia ページから特定のセクションを抽出します。
- 収集した情報について理由を考え、答えを組み立てます。
[2] ReAct Agentとは何ですか?
ReAct Agent は、Reflection-Action サイクルに従う特定のタイプのエージェントです。利用可能な情報と実行可能なアクションに基づいて現在のタスクを反映し、どのアクションを実行するか、またはタスクを完了するかどうかを決定します。
[3] エージェントの計画
3.1 必要なツール
- Node.js
- HTTP リクエスト用の Axios ライブラリ
- Google Generative AI API (gemini-1.5-flash)
- ウィキペディア API
3.2 エージェントの構造
ReAct Agent には 3 つの主な状態があります:
- 考えたこと(反省)
- アクション (実行)
- ANSWER(応答)
[4] エージェントの導入
各状態を強調表示しながら、ReAct Agent を段階的に構築してみましょう。
4.1 初期設定
まず、プロジェクトをセットアップし、依存関係をインストールします。
mkdir react-agent-project cd react-agent-project npm init -y npm install axios dotenv @google/generative-ai
プロジェクトのルートに .env ファイルを作成します:
GOOGLE_AI_API_KEY=your_api_key_here
4.2 Tools.js ファイルの作成
次の内容を含む Tools.js を作成します:
const axios = require("axios"); class Tools { static async wikipedia(q) { try { const response = await axios.get("https://en.wikipedia.org/w/api.php", { params: { action: "query", list: "search", srsearch: q, srwhat: "text", format: "json", srlimit: 4, }, }); const results = await Promise.all( response.data.query.search.map(async (searchResult) => { const sectionResponse = await axios.get( "https://en.wikipedia.org/w/api.php", { params: { action: "parse", pageid: searchResult.pageid, prop: "sections", format: "json", }, }, ); const sections = Object.values( sectionResponse.data.parse.sections, ).map((section) => `${section.index}, ${section.line}`); return { pageTitle: searchResult.title, snippet: searchResult.snippet, pageId: searchResult.pageid, sections: sections, }; }), ); return results .map( (result) => `Snippet: ${result.snippet}\nPageId: ${result.pageId}\nSections: ${JSON.stringify(result.sections)}`, ) .join("\n\n"); } catch (error) { console.error("Error fetching from Wikipedia:", error); return "Error fetching data from Wikipedia"; } } static async wikipedia_with_pageId(pageId, sectionId) { if (sectionId) { const response = await axios.get("https://en.wikipedia.org/w/api.php", { params: { action: "parse", format: "json", pageid: parseInt(pageId), prop: "wikitext", section: parseInt(sectionId), disabletoc: 1, }, }); return Object.values(response.data.parse?.wikitext ?? {})[0]?.substring( 0, 25000, ); } else { const response = await axios.get("https://en.wikipedia.org/w/api.php", { params: { action: "query", pageids: parseInt(pageId), prop: "extracts", exintro: true, explaintext: true, format: "json", }, }); return Object.values(response.data?.query.pages)[0]?.extract; } } } module.exports = Tools;
4.3 ReactAgent.js ファイルの作成
次の内容で ReactAgent.js を作成します:
require("dotenv").config(); const { GoogleGenerativeAI } = require("@google/generative-ai"); const Tools = require("./Tools"); const genAI = new GoogleGenerativeAI(process.env.GOOGLE_AI_API_KEY); class ReActAgent { constructor(query, functions) { this.query = query; this.functions = new Set(functions); this.state = "THOUGHT"; this._history = []; this.model = genAI.getGenerativeModel({ model: "gemini-1.5-flash", temperature: 2, }); } get history() { return this._history; } pushHistory(value) { this._history.push(`\n ${value}`); } async run() { this.pushHistory(`**Task: ${this.query} **`); try { return await this.step(); } catch (e) { if (e.message.includes("exhausted")) { return "Sorry, I'm exhausted, I can't process your request anymore. >>>>>>>", finalAnswer); return finalAnswer; } } module.exports = ReActAgent;
4.4 エージェント(index.js)の実行
次の内容でindex.jsを作成します:
const ReActAgent = require("./ReactAgent.js"); async function main() { const query = "What does England border with?"; const functions = [ [ "wikipedia", "params: query", "Semantic Search Wikipedia API for snippets, pageIds and sectionIds >> \n ex: Date brazil has been colonized? \n Brazil was colonized at 1500, pageId, sections : []", ], [ "wikipedia_with_pageId", "params : pageId, sectionId", "Search Wikipedia API for data using a pageId and a sectionIndex as params. \n ex: 1500, 1234 \n Section information about blablalbal", ], ]; const agent = new ReActAgent(query, functions); try { const result = await agent.run(); console.log("THE AGENT RETURN THE FOLLOWING >>>", result); } catch (e) { console.log("FAILED TO RUN T.T", e); } } main().catch(console.error);
[5] Wikipedia 部分の仕組み
Wikipedia との対話は 2 つの主な手順で行われます:
-
初期検索 (wikipedia 機能):
- Wikipedia 検索 API にリクエストを送信します。
- クエリに対して最大 4 つの関連結果を返します。
- 結果ごとに、ページのセクションを取得します。
-
詳細検索 (wikipedia_with_pageId 関数):
- ページ ID とセクション ID を使用して、特定のコンテンツを取得します。
- 要求されたセクションのテキストを返します。
このプロセスにより、エージェントはまずクエリに関連するトピックの概要を把握し、次に必要に応じて特定のセクションをさらに深く掘り下げることができます。
[6] 実行フロー例
- ユーザーが質問します。
- エージェントは THOUGHT 状態に入り、質問について考えます。
- Wikipedia を検索することを決定し、ACTION 状態に入ります。
- wikipedia 関数を実行し、結果を取得します。
- THOUGHT 状態に戻り、結果を反映します。
- さらに詳細な情報や別のアプローチを検索することを決定する場合があります。
- 必要に応じて思考と行動のサイクルを繰り返します。
- 十分な情報がある場合、ANSWER 状態に入ります。
- 収集されたすべての情報に基づいて最終的な回答を生成します。
- ウィキペディアに収集するデータがない場合は常に無限ループに入ります。タイマーで修正します =P
[7] 最終的な考慮事項
- モジュール構造により、新しいツールや API を簡単に追加できます。
- 無限ループや過剰なリソースの使用を避けるために、エラー処理と時間/反復制限を実装することが重要です。
- 使用温度: 99999 (笑)
以上がNodeJS を使用して ReAct Agent を最初から作成する (wikipedia 検索)の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
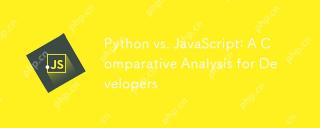
PythonとJavaScriptの主な違いは、タイプシステムとアプリケーションシナリオです。 1。Pythonは、科学的コンピューティングとデータ分析に適した動的タイプを使用します。 2。JavaScriptは弱いタイプを採用し、フロントエンドとフルスタックの開発で広く使用されています。この2つは、非同期プログラミングとパフォーマンスの最適化に独自の利点があり、選択する際にプロジェクトの要件に従って決定する必要があります。
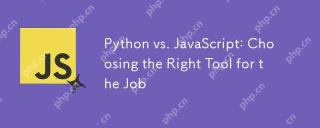
PythonまたはJavaScriptを選択するかどうかは、プロジェクトの種類によって異なります。1)データサイエンスおよび自動化タスクのPythonを選択します。 2)フロントエンドとフルスタック開発のためにJavaScriptを選択します。 Pythonは、データ処理と自動化における強力なライブラリに好まれていますが、JavaScriptはWebインタラクションとフルスタック開発の利点に不可欠です。
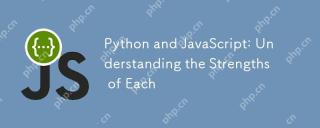
PythonとJavaScriptにはそれぞれ独自の利点があり、選択はプロジェクトのニーズと個人的な好みに依存します。 1. Pythonは、データサイエンスやバックエンド開発に適した簡潔な構文を備えた学習が簡単ですが、実行速度が遅くなっています。 2。JavaScriptはフロントエンド開発のいたるところにあり、強力な非同期プログラミング機能を備えています。 node.jsはフルスタックの開発に適していますが、構文は複雑でエラーが発生しやすい場合があります。
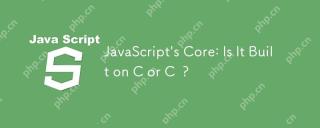
javascriptisnotbuiltoncorc;それは、解釈されていることを解釈しました。
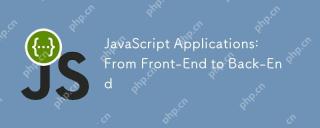
JavaScriptは、フロントエンドおよびバックエンド開発に使用できます。フロントエンドは、DOM操作を介してユーザーエクスペリエンスを強化し、バックエンドはnode.jsを介してサーバータスクを処理することを処理します。 1.フロントエンドの例:Webページテキストのコンテンツを変更します。 2。バックエンドの例:node.jsサーバーを作成します。
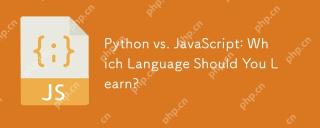
PythonまたはJavaScriptの選択は、キャリア開発、学習曲線、エコシステムに基づいている必要があります。1)キャリア開発:Pythonはデータサイエンスとバックエンド開発に適していますが、JavaScriptはフロントエンドおよびフルスタック開発に適しています。 2)学習曲線:Python構文は簡潔で初心者に適しています。 JavaScriptの構文は柔軟です。 3)エコシステム:Pythonには豊富な科学コンピューティングライブラリがあり、JavaScriptには強力なフロントエンドフレームワークがあります。
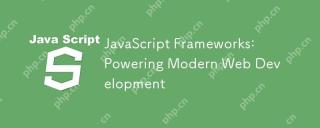
JavaScriptフレームワークのパワーは、開発を簡素化し、ユーザーエクスペリエンスとアプリケーションのパフォーマンスを向上させることにあります。フレームワークを選択するときは、次のことを検討してください。1。プロジェクトのサイズと複雑さ、2。チームエクスペリエンス、3。エコシステムとコミュニティサポート。
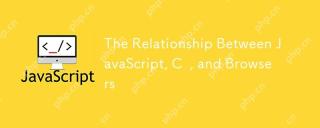
はじめに私はあなたがそれを奇妙に思うかもしれないことを知っています、JavaScript、C、およびブラウザは正確に何をしなければなりませんか?彼らは無関係であるように見えますが、実際、彼らは現代のウェブ開発において非常に重要な役割を果たしています。今日は、これら3つの間の密接なつながりについて説明します。この記事を通して、JavaScriptがブラウザでどのように実行されるか、ブラウザエンジンでのCの役割、およびそれらが協力してWebページのレンダリングと相互作用を駆動する方法を学びます。私たちは皆、JavaScriptとブラウザの関係を知っています。 JavaScriptは、フロントエンド開発のコア言語です。ブラウザで直接実行され、Webページが鮮明で興味深いものになります。なぜJavascrを疑問に思ったことがありますか


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
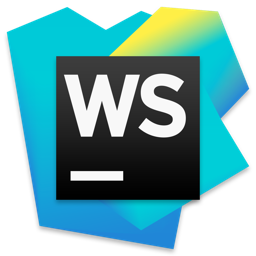
WebStorm Mac版
便利なJavaScript開発ツール

MinGW - Minimalist GNU for Windows
このプロジェクトは osdn.net/projects/mingw に移行中です。引き続きそこでフォローしていただけます。 MinGW: GNU Compiler Collection (GCC) のネイティブ Windows ポートであり、ネイティブ Windows アプリケーションを構築するための自由に配布可能なインポート ライブラリとヘッダー ファイルであり、C99 機能をサポートする MSVC ランタイムの拡張機能が含まれています。すべての MinGW ソフトウェアは 64 ビット Windows プラットフォームで実行できます。
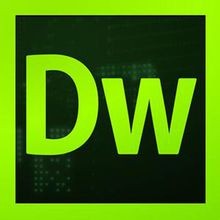
ドリームウィーバー CS6
ビジュアル Web 開発ツール
