導入
Python では、アプリケーションのパフォーマンスを最適化するとき、特に同時実行または並列実行が関係する場合、スレッド と マルチプロセッシング の概念がよく議論されます。用語は重複していますが、これら 2 つのアプローチは根本的に異なります。
このブログは、スレッド と マルチプロセッシング に関する混乱を明確にし、それぞれをいつ使用するかを説明し、各概念に関連する例を提供します。
スレッド処理とマルチプロセッシング: 主な違い
例とユースケースに入る前に、主な違いを概説しましょう:
スレッド: 単一プロセス内で複数のスレッド (プロセスの小さな単位) を実行することを指します。スレッドは同じメモリ空間を共有するため、スレッドは軽量になります。ただし、Python の グローバル インタプリタ ロック (GIL) は、CPU バウンドのタスクのスレッドの真の並列処理を制限します。
マルチプロセッシング: それぞれが独自のメモリ空間を持つ複数のプロセスの実行が含まれます。プロセスはスレッドより重いですが、メモリを共有しないため、真の並列処理を実現できます。このアプローチは、コアを完全に使用する必要がある CPU バウンドのタスクに最適です。
スレッド化とは何ですか?
スレッディング は、同じプロセス内で複数のタスクを同時に実行する方法です。これらのタスクは、同じメモリ空間を共有する独立した軽量の実行単位であるスレッドによって処理されます。スレッド化は、メイン プログラムが外部リソースの待機に多くの時間を費やすファイル読み取り、ネットワーク リクエスト、データベース クエリなど、I/O バウンドの操作に有益です。
スレッドを使用する場合
- プログラムが I/O バウンドである場合 (ファイルの読み取り/書き込み、ネットワーク リクエストの実行など)。
- タスクが入力または出力操作の待機に多くの時間を費やす場合。
- 単一プロセス内で軽量の同時実行性が必要な場合。
例: 基本的なスレッド
import threading import time def print_numbers(): for i in range(5): print(i) time.sleep(1) def print_letters(): for letter in ['a', 'b', 'c', 'd', 'e']: print(letter) time.sleep(1) # Create two threads t1 = threading.Thread(target=print_numbers) t2 = threading.Thread(target=print_letters) # Start both threads t1.start() t2.start() # Wait for both threads to complete t1.join() t2.join() print("Both threads finished execution.")
上記の例では、2 つのスレッドが同時に実行されます。1 つは数値を出力し、もう 1 つは文字を出力します。 sleep() 呼び出しは I/O 操作をシミュレートし、プログラムは待機中にスレッドを切り替えることができます。
スレッドの問題: グローバル インタプリタ ロック (GIL)
Python の GIL は、複数のネイティブ スレッドが Python バイトコードを同時に実行することを防ぐメカニズムです。これにより、プロセス内で複数のスレッドがアクティブになっている場合でも、一度に 1 つのスレッドだけが実行されるようになります。
この制限により、スレッドは GIL のせいで複数のコアを完全に利用できないため、実際の並列処理を必要とする CPU バウンドのタスクにはスレッドが適さないようになります。
マルチプロセッシングとは何ですか?
マルチプロセッシング を使用すると、複数のプロセスを同時に実行できます。各プロセスは独自のメモリ空間を持ちます。プロセスはメモリを共有しないため、GIL の制限がなく、複数の CPU コアでの真の並列実行が可能になります。マルチプロセッシングは、CPU 使用率を最大化する必要がある CPU バウンドのタスクに最適です。
マルチプロセッシングを使用する場合
- プログラムが CPU に依存している場合 (負荷の高い計算やデータ処理など)。
- メモリ共有を行わない真の並列処理が必要な場合。
- 独立したタスクの複数のインスタンスを同時に実行したい場合。
例: 基本的なマルチプロセッシング
import multiprocessing import time def print_numbers(): for i in range(5): print(i) time.sleep(1) def print_letters(): for letter in ['a', 'b', 'c', 'd', 'e']: print(letter) time.sleep(1) if __name__ == "__main__": # Create two processes p1 = multiprocessing.Process(target=print_numbers) p2 = multiprocessing.Process(target=print_letters) # Start both processes p1.start() p2.start() # Wait for both processes to complete p1.join() p2.join() print("Both processes finished execution.")
この例では、2 つの別個のプロセスが同時に実行されます。スレッドとは異なり、各プロセスは独自のメモリ空間を持ち、GIL からの干渉を受けることなく独立して実行されます。
マルチプロセッシングにおけるメモリ分離
スレッド処理とマルチプロセッシングの主な違いの 1 つは、プロセスがメモリを共有しないことです。これにより、プロセス間に干渉がないことが保証されますが、プロセス間でデータを共有するには、マルチプロセッシング モジュールによって提供される Queue、Pipe、Manager オブジェクトなどの特別なメカニズムが必要になることも意味します。
スレッド処理とマルチプロセッシング: 適切なツールの選択
両方のアプローチがどのように機能するかを理解したところで、タスクのタイプに基づいてスレッド処理とマルチプロセッシングをいつ選択するべきかを詳しく見てみましょう。
Use Case | Type | Why? |
---|---|---|
Network requests, I/O-bound tasks (file read/write, DB calls) | Threading | Multiple threads can handle I/O waits concurrently. |
CPU-bound tasks (data processing, calculations) | Multiprocessing | True parallelism is possible by utilizing multiple cores. |
Task requires shared memory or lightweight concurrency | Threading | Threads share memory and are cheaper in terms of resources. |
Independent tasks needing complete isolation (e.g., separate processes) | Multiprocessing | Processes have isolated memory, making them safer for independent tasks. |
Performance Considerations
Threading Performance
Threading excels in scenarios where the program waits on external resources (disk I/O, network). Since threads can work concurrently during these wait times, threading can help boost performance.
However, due to the GIL, CPU-bound tasks do not benefit much from threading because only one thread can execute at a time.
Multiprocessing Performance
Multiprocessing allows true parallelism by running multiple processes across different CPU cores. Each process runs in its own memory space, bypassing the GIL and making it ideal for CPU-bound tasks.
However, creating processes is more resource-intensive than creating threads, and inter-process communication can slow things down if there's a lot of data sharing between processes.
A Practical Example: Threading vs. Multiprocessing for CPU-bound Tasks
Let's compare threading and multiprocessing for a CPU-bound task like calculating the sum of squares for a large list.
Threading Example for CPU-bound Task
import threading def calculate_squares(numbers): result = sum([n * n for n in numbers]) print(result) numbers = range(1, 10000000) t1 = threading.Thread(target=calculate_squares, args=(numbers,)) t2 = threading.Thread(target=calculate_squares, args=(numbers,)) t1.start() t2.start() t1.join() t2.join()
Due to the GIL, this example will not see significant performance improvements over a single-threaded version because the threads can't run simultaneously for CPU-bound operations.
Multiprocessing Example for CPU-bound Task
import multiprocessing def calculate_squares(numbers): result = sum([n * n for n in numbers]) print(result) if __name__ == "__main__": numbers = range(1, 10000000) p1 = multiprocessing.Process(target=calculate_squares, args=(numbers,)) p2 = multiprocessing.Process(target=calculate_squares, args=(numbers,)) p1.start() p2.start() p1.join() p2.join()
In the multiprocessing example, you'll notice a performance boost since both processes run in parallel across different CPU cores, fully utilizing the machine's computational resources.
Conclusion
Understanding the difference between threading and multiprocessing is crucial for writing efficient Python programs. Here’s a quick recap:
- Use threading for I/O-bound tasks where your program spends a lot of time waiting for resources.
- Use multiprocessing for CPU-bound tasks to maximize performance through parallel execution.
Knowing when to use which approach can lead to significant performance improvements and efficient use of resources.
以上がPython のスレッドとマルチプロセッシングを理解する: 包括的なガイドの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
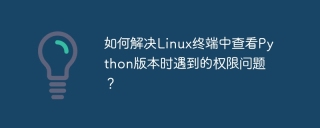
LinuxターミナルでPythonバージョンを表示する際の許可の問題の解決策PythonターミナルでPythonバージョンを表示しようとするとき、Pythonを入力してください...
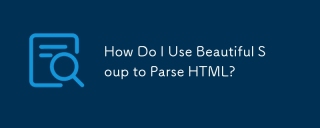
この記事では、Pythonライブラリである美しいスープを使用してHTMLを解析する方法について説明します。 find()、find_all()、select()、およびget_text()などの一般的な方法は、データ抽出、多様なHTML構造とエラーの処理、および代替案(SEL
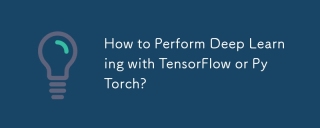
この記事では、深い学習のためにTensorflowとPytorchを比較しています。 関連する手順、データの準備、モデルの構築、トレーニング、評価、展開について詳しく説明しています。 特に計算グラップに関して、フレームワーク間の重要な違い

PythonのPandasライブラリを使用する場合、異なる構造を持つ2つのデータフレーム間で列全体をコピーする方法は一般的な問題です。 2つのデータがあるとします...
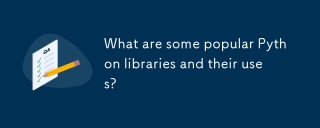
この記事では、numpy、pandas、matplotlib、scikit-learn、tensorflow、django、flask、and requestsなどの人気のあるPythonライブラリについて説明し、科学的コンピューティング、データ分析、視覚化、機械学習、Web開発、Hの使用について説明します。
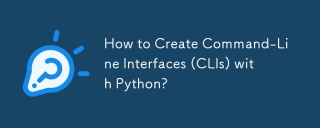
この記事では、コマンドラインインターフェイス(CLI)の構築に関するPython開発者をガイドします。 Typer、Click、Argparseなどのライブラリを使用して、入力/出力の処理を強調し、CLIの使いやすさを改善するためのユーザーフレンドリーな設計パターンを促進することを詳述しています。
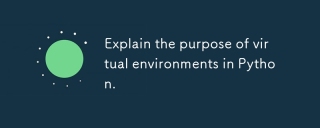
この記事では、Pythonにおける仮想環境の役割について説明し、プロジェクトの依存関係の管理と競合の回避に焦点を当てています。プロジェクト管理の改善と依存関係の問題を減らすための作成、アクティベーション、およびメリットを詳しく説明しています。
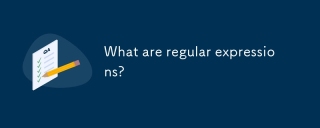
正規表現は、プログラミングにおけるパターンマッチングとテキスト操作のための強力なツールであり、さまざまなアプリケーションにわたるテキスト処理の効率を高めます。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

SublimeText3 英語版
推奨: Win バージョン、コードプロンプトをサポート!

SublimeText3 中国語版
中国語版、とても使いやすい
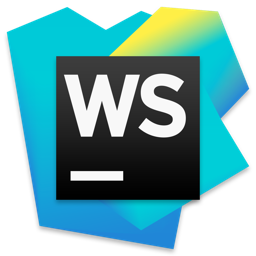
WebStorm Mac版
便利なJavaScript開発ツール
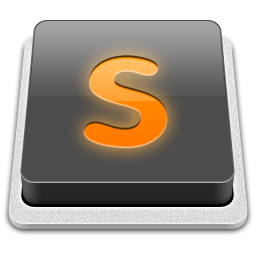
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

ホットトピック



