Java プログラミング言語はさまざまな例外処理ケースを提供しており、同時変更例外もその 1 つです。同時変更例外は、プログラム内のスレッドが、現在のプロセス中に編集する権限を持たないオブジェクトを変更しようとしたときに発生します。つまり、別のプロセスによって現在使用されているオブジェクトを編集しようとすると、ConcurrentModificationException が表示されます。
無料ソフトウェア開発コースを始めましょう
Web 開発、プログラミング言語、ソフトウェア テスト、その他
非常に単純な例は、スレッドによって処理中のコレクションです。処理中のコレクションは変更できないため、ConcurrentModificationException がスローされます。
構文:
以下は、例外の最も単純な構文です。 ConcurrentModificationException は Java lang RunTimeException の一部であり、それを拡張します。
public class Concurrent Modification Exception extends Runtime Exception
Java で ConcurrentModificationException はどのように動作しますか?
Java でオブジェクトを作成するときに、必要に応じていくつかの制限と権限を設定することは理解できます。ただし、リストがスレッド内にあるときに、値を変更したり、リストを修正しようとしたりすることがあります。そのとき、オブジェクトは別のスレッドで使用されており、編集または修正できないため、ConcurrentModificationException が表示されます。
この例外は、オブジェクトが同時に変更されていることを必ずしも意味するわけではないことを知っておくことも重要です。単一スレッドの場合、メソッド呼び出しが発生すると、この例外がスローされる可能性があります。これは何らかの形でオブジェクト コントラクトに違反します。
コンストラクター:
ConcurrentModificationException の非常に基本的なコンストラクターは、ConcurrentModificationException () のように単純です。単純なコンストラクターの他に、必要に応じて使用できる次のコントリビューターがあります:
- ConcurrentModificationException ( String textmessage): 単純なメッセージを含むコンストラクター。
- ConcurrentModificationException ( String textmessage, Throwable textcause): メッセージと詳細な原因を含むコンストラクター。
- ConcurrentModificationException ( Throwable textcause): 原因を持つコンストラクター。ここで、原因は null になる可能性があります。これは、原因が不明であることを意味します。
Java ConcurrentModificationException の例
同時変更例外とは何か、そしてそれがどのように機能するかを理解したので、実装に移りましょう。ここで、例を挙げて例外を理解します。たとえば、配列リストがあり、何らかの操作とともにそれを変更しようとすると、例外が発生します。例のコードは次のとおりです:
例 #1
コード:
import java.awt.List; import java.util.*; public class ConcurrentModificationException { public static void main ( String[] args) { ArrayList<integer> Numberlist = new ArrayList<integer> () ; Numberlist.add ( 1) ; Numberlist.add ( 2) ; Numberlist.add ( 3) ; Numberlist.add ( 4) ; Numberlist.add ( 5) ; Iterator<integer> it = Numberlist.iterator () ; while ( it.hasNext () ) { Integer numbervalue = it.next () ; System.out.println ( "List Value:" + numbervalue) ; if ( numbervalue .equals ( 3) ) Numberlist.remove ( numbervalue) ; } } }</integer></integer></integer>
コードの説明: いくつかのインポート ファイルから始まり、次にクラスの減速と main メソッドです。配列リストを初期化し、数値を加算し続ける関数を追加します。このように、処理中になります。次に追加するたびに、リスト値を出力します。ただし、if ループがあり、3 に等しい数値を探し、3 が見つかるとその数値を削除しようとします。ここで例外が発生し、プログラムが終了します。リストにこれ以上追加されることはありません。
上記のコードをエラーなしで実行すると、コードで例外が発生します。その例外は ConcurrentModificationException.main (ConcurrentModificationException.java:14) にあります。この例外の理由は明らかで、リスト Numberlist を変更しようとしたためです。適切な出力については、以下の添付のスクリーンショットを参照してください:
ご覧のとおり、プログラムは意図したとおりに実行され、print ステートメントは値 3 に一致するまで正常に実行されました。プログラム フローは値 3 で中断されました。これは、次のような if ループがあったためです。値が 3 の場合は削除します。ただし、イテレータはすでに進行中であるため、3 を削除しようとすると失敗し、例外 ConcurrentModificationException がスローされます。
例 #2
2 番目の例では、同時変更例外を回避するプログラムの実行を試みます。同じコードで、処理中に配列リストを変更しようとすると、ConcurrentModificationException がキャッチされ、プログラムが終了します。 2 番目の例のコードは次のとおりです:
コード:
import java.util.Iterator; import java.util.ArrayList; public class ConcurrentModificationException { public static void main ( String args[]) { ArrayList<string> arraylist = new ArrayList<string> ( ) ; arraylist.add ( "One") ; arraylist.add ( "Two") ; arraylist.add ( "Three") ; arraylist.add ( "Four") ; try { System.out.println ( "ArrayList: ") ; Iterator<string> iteratornew = arraylist.iterator () ; while ( iteratornew.hasNext ( ) ) { System.out.print ( iteratornew.next () + ", "); } System.out.println ( "\n\n Trying to add an element in between iteration:" + arraylist.add ( "Five") ) ; System.out.println ( "\n Updated ArrayList: \n") ; iteratornew = arraylist.iterator () ; while ( iteratornew.hasNext ( ) ) { System.out.print ( iteratornew.next () + ", "); } } catch ( Exception e) { System.out.println ( e) ; } } }</string></string></string>
Code Explanation: Similar to the earlier program, we have the required files and methods. We are simply adding a few elements to the array list, and it is iterating; we add another element, which is Five. We will print the updated list upon the latest additions, which will have our newly added element. We have implemented the try-catch block to catch the exception. If any exception occurs, it will print the exception.
Output:
As you can see in the output, the first array list is plain, with few elements. Then in the next line, we attempt to add an element while in iteration, the element is “Five”. It is successfully added, and it prints true for the sentence. And in the next line, we print an updated list, which has the Five-element. We have updated the list after the process is ending; if we try to add the element while in the process, it will throw the ConcurrentModificationException, and the program will cease.
How to Avoid ConcurrentModificationException in Java?
Avoiding Concurrent Modification Exception is possible on a different level of threads. To avoid a Concurrent Exception with a single thread environment, you can remove the object from the iterator and modify it, use remove ().
And in the case of a multi-thread environment, you have few choices like converting the list in the process into an array and then working on the array. Then you can also put a synchronized block over a list and lock it. But locking the list is not recommended as it may limit the advantages of multi-threading programming. Using a sublist to modify a list will result in nothing. Out of all the ways to avoid, the most preferred way is to wait until the execution is finished and then edit or modify the list or the object.
Conclusion
When a process is using one resource and another process attempts to edit or amend it, the exception ConcurrentModificationException will be thrown. The simplest method to avoid is to modify the list after iteration. Many methods are listed to avoid the exception but advised to implement them with small programs.
以上がJava ConcurrentModificationExceptionの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
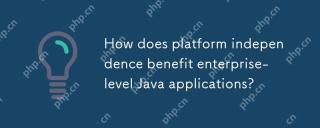
Javaは、プラットフォームの独立性により、エンタープライズレベルのアプリケーションで広く使用されています。 1)プラットフォームの独立性は、Java Virtual Machine(JVM)を介して実装されているため、Javaをサポートする任意のプラットフォームでコードを実行できます。 2)クロスプラットフォームの展開と開発プロセスを簡素化し、柔軟性とスケーラビリティを高めます。 3)ただし、パフォーマンスの違いとサードパーティライブラリの互換性に注意を払い、純粋なJavaコードやクロスプラットフォームテストの使用などのベストプラクティスを採用する必要があります。
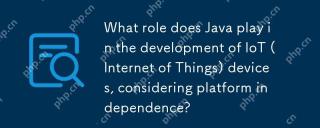
javaplaysasificanificantduetduetoitsplatformindepence.1)itallowscodetobewrittendunonvariousdevices.2)java'secosystemprovidesutionforiot.3)そのセキュリティフィートルセンハンス系
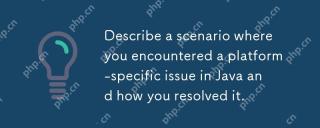
TheSolution to HandlefilepathsaCrosswindossandlinuxinjavaistousepaths.get()fromthejava.nio.filepackage.1)usesystem.getProperty( "user.dir")およびhearterativepathtoconstructurctthefilepath.2)
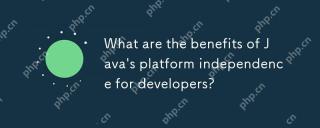
java'splatformentepenceissificAntiveSifcuseDeverowsDevelowSowRitecodeOdeonceantoniTONAnyPlatformwsajvm.これは「writeonce、runanywhere」(wora)adportoffers:1)クロスプラットフォームの複雑性、deploymentacrossdiferentososwithusisues; 2)re
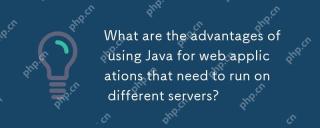
Javaは、クロスサーバーWebアプリケーションの開発に適しています。 1)Javaの「Write and、Run Averywhere」哲学は、JVMをサポートするあらゆるプラットフォームでコードを実行します。 2)Javaには、開発プロセスを簡素化するために、SpringやHibernateなどのツールを含む豊富なエコシステムがあります。 3)Javaは、パフォーマンスとセキュリティにおいて優れたパフォーマンスを発揮し、効率的なメモリ管理と強力なセキュリティ保証を提供します。
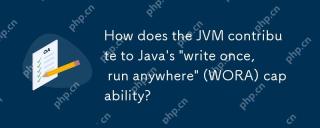
JVMは、バイトコード解釈、プラットフォームに依存しないAPI、動的クラスの負荷を介してJavaのWORA機能を実装します。 2。標準API抽象オペレーティングシステムの違い。 3.クラスは、実行時に動的にロードされ、一貫性を確保します。
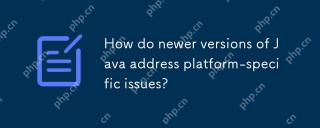
Javaの最新バージョンは、JVMの最適化、標準的なライブラリの改善、サードパーティライブラリサポートを通じて、プラットフォーム固有の問題を効果的に解決します。 1)Java11のZGCなどのJVM最適化により、ガベージコレクションのパフォーマンスが向上します。 2)Java9のモジュールシステムなどの標準的なライブラリの改善は、プラットフォーム関連の問題を削減します。 3)サードパーティライブラリは、OpenCVなどのプラットフォーム最適化バージョンを提供します。
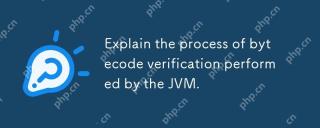
JVMのバイトコード検証プロセスには、4つの重要な手順が含まれます。1)クラスファイル形式が仕様に準拠しているかどうかを確認し、2)バイトコード命令の有効性と正確性を確認し、3)データフロー分析を実行してタイプの安全性を確保し、検証の完全性とパフォーマンスのバランスをとる。これらの手順を通じて、JVMは、安全で正しいバイトコードのみが実行されることを保証し、それによりプログラムの完全性とセキュリティを保護します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SublimeText3 中国語版
中国語版、とても使いやすい

メモ帳++7.3.1
使いやすく無料のコードエディター

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

PhpStorm Mac バージョン
最新(2018.2.1)のプロフェッショナル向けPHP統合開発ツール

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。
