Java では、Scanner は、文字列および int、double などのさまざまなプリミティブ型の入力を取得するために使用されるクラスです。スキャナー クラスは、パッケージ java にあります。これは Object クラスを拡張し、Closeable インターフェイスと Iterator インターフェイスを実装します。入力は空白区切り文字を使用してクラスに分割されます。 Java の Scanner クラスの宣言、構文、動作、その他のいくつかの側面については、次のセクションで説明します。
無料ソフトウェア開発コースを始めましょう
Web 開発、プログラミング言語、ソフトウェア テスト、その他
Java スキャナー クラス宣言
Java Scanner クラスは以下のように宣言されています。
public final class Scanner extends Object implements Iterator<String>
構文:
Scanner クラスは、Java プログラムの以下の構文で使用できます。
Scanner sc = new Scanner(System.in);
ここで、sc はスキャナ オブジェクトであり、System.in は Java に入力がこれであることを伝えます。
Java スキャナ クラスはどのように機能しますか?
次に、Scanner クラスがどのように機能するかを見てみましょう。
- クラス java.util.Scanner またはパッケージ全体 java.util.
をインポートします。
import java.util.*;
import java.util.Scanner;
- 構文に示すようにスキャナ オブジェクトを作成します。
Scanner s = new Scanner(System.in);
- 型 int、char、または string を宣言します。
int n = sc.nextInt();
コンストラクター
Java のスキャナー クラスにはさまざまなコンストラクターがあります。それらは次のとおりです:
-
Scanner(File src): 言及されたファイルから値を生成する新しいスキャナーが構築されます。
-
Scanner(File src, String charsetName): 前述のファイルからスキャンされた値を生成する新しいスキャナーが構築されます。
-
Scanner(InputStream src): 前述の入力ストリームから値を生成する新しいスキャナーが構築されます。
-
Scanner(InputStream src, String charsetName): 前述の入力ストリームから値を生成する新しいスキャナーが構築されます。
-
Scanner(Path src): 前述のファイルから値を生成する新しいスキャナーが構築されます。
-
Scanner(Path src, String charsetName): 前述のファイルから値を生成する新しいスキャナーが構築されます。
-
Scanner(Readable src): 言及されたソースから値を生成する新しいスキャナーが構築されます。
-
Scanner(ReadableByteChannel src): 言及されたチャネルから値を生成する新しいスキャナーが構築されます。
-
Scanner(ReadableByteChannel src, String charsetName): 言及されたチャネルから値を生成する新しいスキャナーが構築されます。
-
Scanner(String src): 指定された文字列から値を生成する新しいスキャナーが構築されます。
Java スキャナ クラスのメソッド
次に、さまざまな機能を実行するメソッドを示します。
-
close(): Scanner gets closed on calling this method.
-
findInLine(Pattern p): Next occurrence of the mentioned pattern p will be attempted to find out, ignoring the delimiters.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
delimiter(): Pattern that is currently used by the scanner to match delimiters will be returned.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
findWithinHorizon(Pattern p, int horizon): Tries to identify the mentioned pattern’s next occurrence.
-
hasNext(): If the scanner has another token in the input, true will be returned.
-
findWithinHorizon(String p, int horizon): Tries to identify the next occurrence of the mentioned pattern made from the string p, ignoring delimiters.
-
hasNext(Pattern p): If the next token matches the mentioned pattern p, true will be returned.
-
hasNext(String p): If the next token matches the mentioned pattern p made from the string p, true will be returned.
-
next(Pattern p): Next token will be returned, which matches the mentioned pattern p.
-
next(String p): Next token will be returned, which matches the mentioned pattern p made from the string p.
-
nextBigDecimal(): Input’s next token will be scanned as a BigDecimal.
-
nextBigInteger(): Input’s next token will be scanned as a BigInteger.
-
nextBigInteger(int rad): Input’s next token will be scanned as a BigInteger.
-
nextBoolean(): Input’s next token will be scanned as a Boolean, and it will be returned.
-
nextByte(): Input’s next token will be scanned as a byte.
-
nextByte(int rad): Input’s next token will be scanned as a byte.
-
nextDouble(): Input’s next token will be scanned as a double.
-
nextFloat(): Input’s next token will be scanned as a float.
-
nextInt(): Input’s next token will be scanned as an integer.
-
nextInt(int rad): Input’s next token will be scanned as an integer.
-
nextLong(int rad): Input’s next token will be scanned as a long.
-
nextLong(): Input’s next token will be scanned as a long.
-
nextShort(int rad): Input’s next token will be scanned as a short.
-
nextShort(): Input’s next token will be scanned as a short.
-
radix(): The default radix of the scanner will be returned.
-
useDelimiter(Pattern p): Delimiting pattern of the scanner will be set to the mentioned pattern.
-
useDelimiter(String p): Delimiting pattern of the scanner will be set to the mentioned pattern made from the string p.
-
useRadix(int rad): The scanner’s default radix will be set to the mentioned radix.
-
useLocale(Locale loc): The locale of the scanner will be set to the mentioned locale.
Examples of Java Scanner Class
Now, let us see some sample programs of the Scanner class in Java.
Example #1
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter the username");
String obj = sc.nextLine();
System.out.println("The name you entered is: " + obj);
}
}
Output:
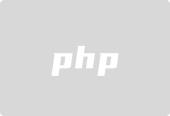
On executing the program, the user will be asked to enter a name. As the input is a string, the nextLine() method will be used. Once it is entered, a line will be printed displaying the name user given as input.
Example #2
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter age");
int obj = sc.nextInt();
System.out.println("The age you entered is: " + obj);
}
}
Output:
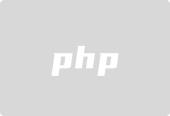
On executing the program, the user will be asked to enter the age. As the input is a number, the nextInt() method will be used. Once it is entered, a line will be printed displaying the age user given as input.
以上がJava スキャナ クラスの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。