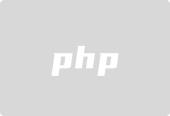
Web パフォーマンスの最適化は、高速でシームレスなユーザー エクスペリエンスを提供するために重要です。これを実現する効果的な方法の 1 つは、CSS、JavaScript、および HTML ファイルを縮小して結合することです。今日は、縮小と結合の意味、重要な理由、実践例を使用してそれらを実装する方法について説明します
縮小化
縮小化とは、コードの機能を変更せずにコードから不要な文字を削除するプロセスです。これには以下が含まれます:
-
空白の削除: スペース、タブ、改行。
-
コメントの削除: 開発者向けの機能しないテキスト。
-
変数名の短縮: 変数と関数の短縮名を使用します。
縮小化の例
元のコード
CSS ファイル (styles.css)
/* Main Styles */
body {
background-color: #f0f0f0; /* Light gray background */
font-family: Arial, sans-serif;
}
/* Header Styles */
header {
background-color: #333; /* Dark background for header */
color: #fff;
padding: 10px;
}
header h1 {
margin: 0;
}
JavaScript ファイル (script.js)
// Function to change background color
function changeBackgroundColor(color) {
document.body.style.backgroundColor = color;
}
// Function to log message
function logMessage(message) {
console.log(message);
}
縮小されたコード
縮小 CSS (styles.min.css)
cssbody{background-color:#f0f0f0;font-family:Arial,sans-serif}header{background-color:#333;color:#fff;padding:10px}header h1{margin:0}
縮小された JavaScript (script.min.js)
javascript
function changeBackgroundColor(a){document.body.style.backgroundColor=a}function logMessage(a){console.log(a)}
説明:
-
CSS: 空白とコメントが削除されます。プロパティ名と値は可能な限り短縮されています。
-
JavaScript: コメントと不要な空白は削除されます。変数名は短縮されます。
なぜそれを行うのか:
-
ファイル サイズを削減します: ファイルが小さいほど、ダウンロードするデータが少なくなり、読み込み時間が短縮されます。
-
パフォーマンスの向上: ファイル転送の高速化により、ページの読み込み時間が短縮され、ユーザー エクスペリエンスが向上します。
-
帯域幅使用量の削減: ファイルが小さいほど、転送されるデータ量が減り、帯域幅が節約され、コストが削減される可能性があります。
いつ行うべきか:
-
デプロイ前: 運用環境にデプロイする前に、ビルド プロセスの一部としてファイルを縮小します。これにより、ユーザーに提供されるコードのパフォーマンスが確実に最適化されます。
-
リリースごと: 継続的インテグレーション/継続的デプロイメント (CI/CD) パイプラインに縮小化を組み込んで、リリースごとにファイルを自動的に縮小します。
ファイルを結合する
ファイルの結合とは、複数の CSS または JavaScript ファイルを 1 つのファイルに結合することを指します。例:
-
CSS ファイルの結合: 複数の CSS ファイルを用意する代わりに、それらを 1 つに結合します。
-
JavaScript ファイルの結合: 同様に、複数の JavaScript ファイルが 1 つに結合されます。
ファイルの結合例
元のファイル
CSS ファイル
- リセット.css
- タイポグラフィ.css
- レイアウト.css
JavaScript ファイル
- utils.js
- main.js
- analytics.js
結合されたファイル
結合 CSS (styles.css)
css/* Reset styles */
body, h1, h2, h3, p { margin: 0; padding: 0; }
/* Typography styles */
body { font-family: Arial, sans-serif; }
h1 { font-size: 2em; }
/* Layout styles */
.container { width: 100%; max-width: 1200px; margin: 0 auto; }
結合された JavaScript (scripts.js)
javascript// Utility functions
function changeBackgroundColor(color) { document.body.style.backgroundColor = color; }
function logMessage(message) { console.log(message); }
// Main application logic
function initApp() { console.log('App initialized'); }
window.onload = initApp;
// Analytics
function trackEvent(event) { console.log('Event tracked:', event); }
Explanation:
-
CSS: Multiple CSS files are merged into a single file, preserving their order and combining styles.
-
JavaScript: Multiple JavaScript files are merged into a single file, keeping functions and logic organized.
Why Do It:
-
Reduce HTTP Requests: Each file requires a separate HTTP request. Combining files reduces the number of requests the browser needs to make, which can significantly improve load times.
-
Improve Page Load Speed: Fewer HTTP requests mean less overhead and faster loading, as browsers can handle fewer connections and process fewer files.
-
Simplify Management: Fewer files can simplify your file structure and make it easier to manage dependencies.
When To Do It:
-
During the Build Process: Like minification, combining files should be part of your build process, usually handled by task runners or build tools (e.g., Webpack, Gulp, or Parcel).
-
In Production: Combine files before deploying to production to ensure that users receive the optimized versions.
Tools and Techniques
-
Minification Tools: Tools like UglifyJS, Terser (for JavaScript), and CSSNano (for CSS) are commonly used for minification.
-
Build Tools: Task runners like Gulp or Webpack can automate both minification and file combining.
-
CDNs: Many Content Delivery Networks (CDNs) offer built-in minification and combination features.
By minifying and combinSure! Let's walk through some practical examples of minifying and combining CSS and JavaScript files.
Why This Matters
-
Minification: Reduces the size of individual files, which decreases the amount of data the browser needs to download.
-
Combining: Reduces the number of HTTP requests, which decreases load time and improves performance.
Tools for Combining and Minifying:
-
Gulp: A task runner that can automate minification and combining.
-
Webpack: A module bundler that can combine and minify files as part of its build process.
-
Online Tools: Websites like CSS Minifier and JSCompress can also be used for minification.
By following these practices, you optimize the performance of your web application, leading to a faster and smoother user experience.ing CSS and JavaScript files, you streamline the delivery of your web assets, leading to faster load times and a better overall user experience.
以上がCSS、JavaScript を縮小するとはどういう意味ですか?なぜ、いつ行うのか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。