テンプレート メソッド パターンはアルゴリズムのスケルトンを定義し、特定のステップはサブクラスによって実装されるため、サブクラスは全体の構造を変更せずに特定のステップをカスタマイズできます。このパターンは次の目的で使用されます。 1. アルゴリズムのスケルトンを定義します。 2. アルゴリズムの特定の動作をサブクラスに延期します。 3. アルゴリズム全体の構造を変更せずに、サブクラスがアルゴリズムの特定のステップをカスタマイズできるようにします。
PHP のテンプレート メソッド パターン
はじめに
テンプレート メソッド パターンは、アルゴリズムの骨格を定義する設計パターンであり、特定のステップはサブクラスによって実装されます。これにより、サブクラスはアルゴリズムの全体的な構造を変更することなく、特定のステップをカスタマイズできます。
UML 図
+----------------+ | AbstractClass | +----------------+ | + templateMethod() | +----------------+ +----------------+ | ConcreteClass1 | +----------------+ | + concreteMethod1() | +----------------+ +----------------+ | ConcreteClass2 | +----------------+ | + concreteMethod2() | +----------------+
コード例
AbstractClass.php
abstract class AbstractClass { public function templateMethod() { $this->step1(); $this->step2(); $this->hookMethod(); } protected abstract function step1(); protected abstract function step2(); protected function hookMethod() {} }
ConcreteClass1.php
class ConcreteClass1 extends AbstractClass { protected function step1() { echo "ConcreteClass1: Step 1<br>"; } protected function step2() { echo "ConcreteClass1: Step 2<br>"; } }
ConcreteClass2.php
class ConcreteClass2 extends AbstractClass { protected function step1() { echo "ConcreteClass2: Step 1<br>"; } protected function step2() { echo "ConcreteClass2: Step 2<br>"; } protected function hookMethod() { echo "ConcreteClass2: Hook Method<br>"; } }
実際的なケース
生徒がいると仮定します。システムを管理するには、「学生リスト」ページと「学生詳細」ページの 2 つのページを作成する必要があります。 2 つのページは同じレイアウトを使用していますが、内容が異なります。
StudentManager.php
class StudentManager { public function showStudentList() { $students = // 获取学生数据 $view = new StudentListView(); $view->setStudents($students); $view->render(); } public function showStudentDetail($id) { $student = // 获取学生数据 $view = new StudentDetailView(); $view->setStudent($student); $view->render(); } }
StudentListView.php
class StudentListView extends AbstractView { private $students; public function setStudents($students) { $this->students = $students; } public function render() { $this->showHeader(); $this->showStudents(); $this->showFooter(); } protected function showStudents() { echo "<h1 id="学生列表">学生列表</h1>"; echo "<ul>"; foreach ($this->students as $student) { echo "<li>" . $student->getName() . "</li>"; } echo "</ul>"; } }
StudentDetailView.php
class StudentDetailView extends AbstractView { private $student; public function setStudent($student) { $this->student = $student; } public function render() { $this->showHeader(); $this->showStudent(); $this->showFooter(); } protected function showStudent() { echo "<h1 id="学生详情">学生详情</h1>"; echo "<p>姓名:" . $this->student->getName() . "</p>"; echo "<p>年龄:" . $this->student->getAge() . "</p>"; } }
以上がPHPでテンプレートメソッドパターンを使用するにはどうすればよいですか?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
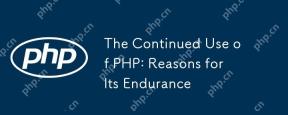
まだ人気があるのは、使いやすさ、柔軟性、強力なエコシステムです。 1)使いやすさとシンプルな構文により、初心者にとって最初の選択肢になります。 2)Web開発、HTTP要求とデータベースとの優れた相互作用と密接に統合されています。 3)巨大なエコシステムは、豊富なツールとライブラリを提供します。 4)アクティブなコミュニティとオープンソースの性質は、それらを新しいニーズとテクノロジーの傾向に適応させます。
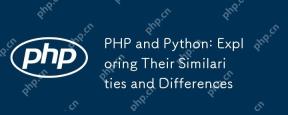
PHPとPythonはどちらも、Web開発、データ処理、自動化タスクで広く使用されている高レベルのプログラミング言語です。 1.PHPは、ダイナミックウェブサイトとコンテンツ管理システムの構築によく使用されますが、PythonはWebフレームワークとデータサイエンスの構築に使用されることがよくあります。 2.PHPはエコーを使用してコンテンツを出力し、Pythonは印刷を使用します。 3.両方ともオブジェクト指向プログラミングをサポートしますが、構文とキーワードは異なります。 4。PHPは弱いタイプの変換をサポートしますが、Pythonはより厳しくなります。 5. PHPパフォーマンスの最適化には、Opcacheおよび非同期プログラミングの使用が含まれますが、PythonはCprofileおよび非同期プログラミングを使用します。
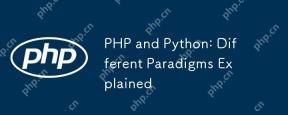
PHPは主に手順プログラミングですが、オブジェクト指向プログラミング(OOP)もサポートしています。 Pythonは、OOP、機能、手続き上のプログラミングなど、さまざまなパラダイムをサポートしています。 PHPはWeb開発に適しており、Pythonはデータ分析や機械学習などのさまざまなアプリケーションに適しています。
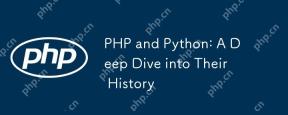
PHPは1994年に発信され、Rasmuslerdorfによって開発されました。もともとはウェブサイトの訪問者を追跡するために使用され、サーバー側のスクリプト言語に徐々に進化し、Web開発で広く使用されていました。 Pythonは、1980年代後半にGuidovan Rossumによって開発され、1991年に最初にリリースされました。コードの読みやすさとシンプルさを強調し、科学的コンピューティング、データ分析、その他の分野に適しています。
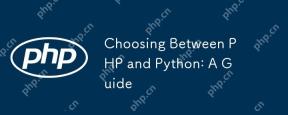
PHPはWeb開発と迅速なプロトタイピングに適しており、Pythonはデータサイエンスと機械学習に適しています。 1.PHPは、単純な構文と迅速な開発に適した動的なWeb開発に使用されます。 2。Pythonには簡潔な構文があり、複数のフィールドに適しており、強力なライブラリエコシステムがあります。
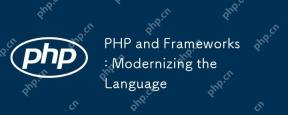
PHPは、多数のWebサイトとアプリケーションをサポートし、フレームワークを通じて開発ニーズに適応するため、近代化プロセスで依然として重要です。 1.PHP7はパフォーマンスを向上させ、新機能を紹介します。 2。Laravel、Symfony、Codeigniterなどの最新のフレームワークは、開発を簡素化し、コードの品質を向上させます。 3.パフォーマンスの最適化とベストプラクティスは、アプリケーションの効率をさらに改善します。
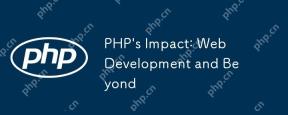
phphassiblasifly-impactedwebdevevermentandsbeyondit.1)itpowersmajorplatformslikewordpratsandexcelsindatabase interactions.2)php'sadaptableability allowsitale forlargeapplicationsusingframeworkslikelavel.3)
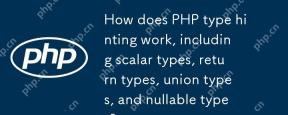
PHPタイプは、コードの品質と読みやすさを向上させるためのプロンプトがあります。 1)スカラータイプのヒント:php7.0であるため、基本データ型は、int、floatなどの関数パラメーターで指定できます。 3)ユニオンタイプのプロンプト:PHP8.0であるため、関数パラメーターまたは戻り値で複数のタイプを指定することができます。 4)Nullable Typeプロンプト:null値を含めることができ、null値を返す可能性のある機能を処理できます。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

SecLists
SecLists は、セキュリティ テスターの究極の相棒です。これは、セキュリティ評価中に頻繁に使用されるさまざまな種類のリストを 1 か所にまとめたものです。 SecLists は、セキュリティ テスターが必要とする可能性のあるすべてのリストを便利に提供することで、セキュリティ テストをより効率的かつ生産的にするのに役立ちます。リストの種類には、ユーザー名、パスワード、URL、ファジング ペイロード、機密データ パターン、Web シェルなどが含まれます。テスターはこのリポジトリを新しいテスト マシンにプルするだけで、必要なあらゆる種類のリストにアクセスできるようになります。
