C++ は、数学的モデルを構築し、シミュレーションを実行し、パラメーターを最適化することにより、ロケット エンジンのパフォーマンスを大幅に向上させることができます。ロケット エンジンの数学的モデルを構築し、その動作を記述します。エンジンのパフォーマンスをシミュレートし、推力や比推力などの主要なパラメーターを計算します。遺伝的アルゴリズムなどの最適化アルゴリズムを使用して、主要なパラメーターを特定し、最適な値を検索します。エンジンのパフォーマンスは最適化されたパラメータに基づいて再計算され、全体的な効率が向上します。
C++ を使用したロケット エンジンのパフォーマンスの最適化
ロケット エンジニアリングにおいて、エンジンのパフォーマンスの最適化は、ロケットのペイロード容量、航続距離、全体的な効率に直接影響するため、非常に重要です。 C++ は、高性能で柔軟なプログラミング環境を提供するため、ロケット エンジンのモデリングとシミュレーションに推奨される言語の 1 つです。
ロケット エンジンのモデル化
最初のステップは、ロケット エンジンの数学的モデルを構築することです。エンジンの動作は、ニュートンの運動法則、熱力学の原理、流体力学の方程式を使用して説明できます。これらの方程式を C++ コードに変換して、ロケット エンジンの仮想モデルを作成できます。
エンジン性能のシミュレーション
次のステップは、さまざまな条件下でロケット エンジンの性能をシミュレーションすることです。これには、推力、比推力、効率などの重要なパラメータを計算するための数学的モデルの解決が含まれます。 C++ の強力な数値計算ライブラリと効率的な並列プログラミング機能により、このようなシミュレーションに最適です。
パラメータの最適化
エンジニアはシミュレーションを通じて、エンジンのパフォーマンスを最適化できる主要なパラメータを特定できます。これらのパラメータには、ノズルの形状、推進剤の組成、および燃焼室の形状が含まれる場合があります。遺伝的アルゴリズムや粒子群最適化などの C++ の最適化アルゴリズムを使用して、これらのパラメーターの最適値を検索できます。
実際的なケース
以下は、C++ を使用してロケット エンジンのパフォーマンスを最適化する実際的なケースです:
#include <iostream> #include <cmath> #include <vector> using namespace std; class RocketEngine { public: // Constructor RocketEngine(double nozzle_shape, double propellant_composition, double combustion_chamber_geometry) { this->nozzle_shape = nozzle_shape; this->propellant_composition = propellant_composition; this->combustion_chamber_geometry = combustion_chamber_geometry; } // Calculate thrust double calculate_thrust() { // Implement thrust calculation using relevant equations } // Calculate specific impulse double calculate_specific_impulse() { // Implement specific impulse calculation using relevant equations } // Calculate efficiency double calculate_efficiency() { // Implement efficiency calculation using relevant equations } // Getters and setters for parameters double get_nozzle_shape() { return nozzle_shape; } void set_nozzle_shape(double value) { nozzle_shape = value; } double get_propellant_composition() { return propellant_composition; } void set_propellant_composition(double value) { propellant_composition = value; } double get_combustion_chamber_geometry() { return combustion_chamber_geometry; } void set_combustion_chamber_geometry(double value) { combustion_chamber_geometry = value; } private: double nozzle_shape; double propellant_composition; double combustion_chamber_geometry; }; int main() { // Create a rocket engine with initial parameters RocketEngine engine(0.5, 0.7, 0.8); // Define optimization algorithm and objective function GeneticAlgorithm optimizer; double objective_function = [](RocketEngine &engine) { return engine.calculate_thrust() * engine.calculate_specific_impulse(); }; // Run optimization algorithm optimizer.optimize(engine, objective_function); // Print optimized parameters and engine performance cout << "Optimized nozzle shape: " << engine.get_nozzle_shape() << endl; cout << "Optimized propellant composition: " << engine.get_propellant_composition() << endl; cout << "Optimized combustion chamber geometry: " << engine.get_combustion_chamber_geometry() << endl; cout << "Thrust: " << engine.calculate_thrust() << endl; cout << "Specific impulse: " << engine.calculate_specific_impulse() << endl; cout << "Efficiency: " << engine.calculate_efficiency() << endl; return 0; }
この例では、パラメーターを変更できるロケット エンジン モデルを作成するために C++ が使用されています。遺伝的アルゴリズムを使用してこれらのパラメーターを最適化し、推力と比推力の積を最大化し、それによってエンジンの全体的なパフォーマンスが向上します。
以上がC++ を使用したロケット エンジンのパフォーマンスの最適化の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
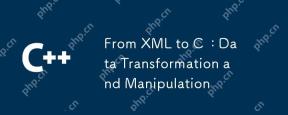
XMLからCへの変換とデータ操作の実行は、次の手順で達成できます。1)TinyXML2ライブラリを使用してXMLファイルを解析する、2)データのデータ構造にデータをマッピングし、3)データ操作のためのSTD :: VectorなどのC標準ライブラリを使用します。これらの手順を通じて、XMLから変換されたデータを処理および効率的に操作できます。
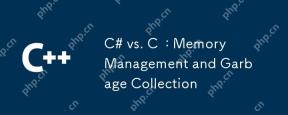
C#は自動ガベージコレクションメカニズムを使用し、Cは手動メモリ管理を使用します。 1。C#のゴミコレクターは、メモリを自動的に管理してメモリの漏れのリスクを減らしますが、パフォーマンスの劣化につながる可能性があります。 2.Cは、微細な管理を必要とするアプリケーションに適した柔軟なメモリ制御を提供しますが、メモリの漏れを避けるためには注意して処理する必要があります。

Cは、現代のプログラミングにおいて依然として重要な関連性を持っています。 1)高性能および直接的なハードウェア操作機能により、ゲーム開発、組み込みシステム、高性能コンピューティングの分野で最初の選択肢になります。 2)豊富なプログラミングパラダイムとスマートポインターやテンプレートプログラミングなどの最新の機能は、その柔軟性と効率を向上させます。学習曲線は急ですが、その強力な機能により、今日のプログラミングエコシステムでは依然として重要です。
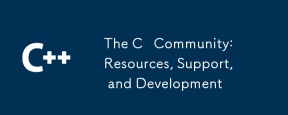
C学習者と開発者は、Stackoverflow、RedditのR/CPPコミュニティ、CourseraおよびEDXコース、Github、Professional Consulting Services、およびCPPCONのオープンソースプロジェクトからリソースとサポートを得ることができます。 1. StackOverFlowは、技術的な質問への回答を提供します。 2。RedditのR/CPPコミュニティが最新ニュースを共有しています。 3。CourseraとEDXは、正式なCコースを提供します。 4. LLVMなどのGitHubでのオープンソースプロジェクトやスキルの向上。 5。JetBrainやPerforceなどの専門的なコンサルティングサービスは、技術サポートを提供します。 6。CPPCONとその他の会議はキャリアを助けます
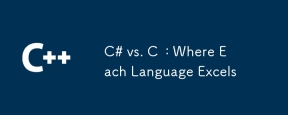
C#は、開発効率とクロスプラットフォームのサポートを必要とするプロジェクトに適していますが、Cは高性能で基礎となるコントロールを必要とするアプリケーションに適しています。 1)C#は、開発を簡素化し、ガベージコレクションとリッチクラスライブラリを提供します。これは、エンタープライズレベルのアプリケーションに適しています。 2)Cは、ゲーム開発と高性能コンピューティングに適した直接メモリ操作を許可します。
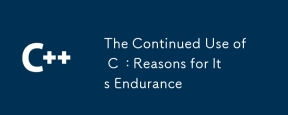
C継続的な使用の理由には、その高性能、幅広いアプリケーション、および進化する特性が含まれます。 1)高効率パフォーマンス:Cは、メモリとハードウェアを直接操作することにより、システムプログラミングと高性能コンピューティングで優れたパフォーマンスを発揮します。 2)広く使用されている:ゲーム開発、組み込みシステムなどの分野での輝き。3)連続進化:1983年のリリース以来、Cは競争力を維持するために新しい機能を追加し続けています。
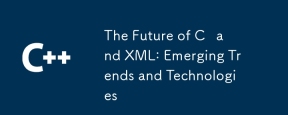
CとXMLの将来の開発動向は次のとおりです。1)Cは、プログラミングの効率とセキュリティを改善するためのC 20およびC 23の標準を通じて、モジュール、概念、CORoutinesなどの新しい機能を導入します。 2)XMLは、データ交換および構成ファイルの重要なポジションを引き続き占有しますが、JSONとYAMLの課題に直面し、XMLSchema1.1やXpath3.1の改善など、より簡潔で簡単な方向に発展します。
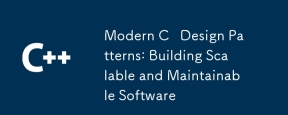
最新のCデザインモデルは、C 11以降の新機能を使用して、より柔軟で効率的なソフトウェアを構築するのに役立ちます。 1)ラムダ式とstd :: functionを使用して、オブザーバーパターンを簡素化します。 2)モバイルセマンティクスと完全な転送を通じてパフォーマンスを最適化します。 3)インテリジェントなポインターは、タイプの安全性とリソース管理を保証します。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

AtomエディタMac版ダウンロード
最も人気のあるオープンソースエディター

MinGW - Minimalist GNU for Windows
このプロジェクトは osdn.net/projects/mingw に移行中です。引き続きそこでフォローしていただけます。 MinGW: GNU Compiler Collection (GCC) のネイティブ Windows ポートであり、ネイティブ Windows アプリケーションを構築するための自由に配布可能なインポート ライブラリとヘッダー ファイルであり、C99 機能をサポートする MSVC ランタイムの拡張機能が含まれています。すべての MinGW ソフトウェアは 64 ビット Windows プラットフォームで実行できます。
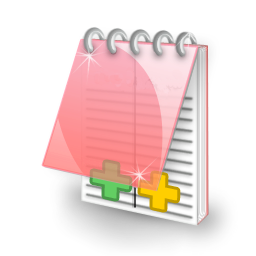
EditPlus 中国語クラック版
サイズが小さく、構文の強調表示、コード プロンプト機能はサポートされていません
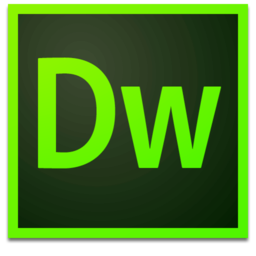
Dreamweaver Mac版
ビジュアル Web 開発ツール

メモ帳++7.3.1
使いやすく無料のコードエディター
