JavaScript の中核は ECMAScript です。他の言語と同様に、ECMAScript 文字列は不変です。つまり、その値は変更できません。
次のコードを考えてみましょう:
var str = "hello ";
str = "world";実際、このコードによってバックグラウンドで実行される手順は次のとおりです。
1. 「hello」文字列を格納する文字。
2.「world」を格納する文字列を作成します。
3. 接続結果を保存する文字列を作成します。
4. str の現在の内容を結果にコピーします。
5. 「world」を結果にコピーします。
6. 結果を指すように str を更新します。
ステップ 2 ~ 6 は文字列の連結が完了するたびに実行されるため、この操作はリソースを非常に消費します。このプロセスが何百回、あるいは何千回も繰り返されると、パフォーマンスの問題が発生する可能性があります。解決策は、Array オブジェクトを使用して文字列を保存し、join() メソッド (パラメータは空の文字列) を使用して最終的な文字列を作成することです。前のコードを次のコードに置き換えることを想像してください:
var arr = new Array();
arr[0] = "hello ";
arr[1] = "world";
var str = arr.join("");
このように、結合操作は join() メソッドが呼び出されたときにのみ発生するため、配列にどれだけ多くの文字列が導入されても問題にはなりません。この時点で実行する手順は次のとおりです:
1. 結果を保存する文字列を作成します
2. 各文字列を結果内の適切な場所にコピーします
この解決策は優れていますが、より良い方法があります。問題は、このコードが意図した内容を正確に反映していないことです。理解しやすくするために、この機能を StringBuffer クラスでラップできます。
function StringBuffer () {
this._strings_ = new Array();
}
StringBuffer.prototype.append = function(str) {
this._strings_.push(str);
};
StringBuffer.prototype.toString = function() {
return this._strings_.join("");
};
この中で最初に注意すべきことコードは文字列です。 属性はプライベート属性であることを意味します。これには、append() メソッドと toString() メソッドという 2 つのメソッドしかありません。 append() メソッドにはパラメータがあり、このパラメータを文字列配列に追加します。 toString() メソッドは配列の join メソッドを呼び出し、実際の連結された文字列を返します。 StringBuffer オブジェクトを使用して文字列のセットを連結するには、次のコードを使用できます。
varbuffer = new StringBuffer ();
buffer.append("hello ");
buffer.append("world");
var result =buffer.toString ();
上記の実装に基づいて、実行時間を比較してみましょう。つまり、「 " を使用して文字列とカプセル化されたツールを 1 つずつ接続します。次のコードを使用して、StringBuffer オブジェクトと従来の文字列連結メソッドのパフォーマンスをテストできます。
var d1 = new Date();
var str = "";
for (var i=0; i < 10000; i ) {
str = "テキスト";
}
var d2 = 新しい日付();
console.log("プラスとの連結: "
(d2.getTime() - d1.getTime()) " ミリ秒");
varbuffer = new StringBuffer();
d1 = new Date();
for (var i=0; i buffer.append("text") ;
}
var result =buffer.toString();
d2 = new Date();
console.log("StringBuffer との連結: "
(d2.getTime() - d1.getTime()) " ミリ秒");
This code performs two tests for string concatenation, the first using the plus sign and the second using the StringBuffer class. Each operation concatenates 10,000 strings. The date values d1 and d2 are used to determine how long it takes to complete the operation. Please note that when creating a Date object without parameters, the current date and time are assigned to the object. To calculate how long the join operation took, subtract the millisecond representation of the date (using the return value of the getTime() method). This is a common way to measure JavaScript performance. The results of this test can help you compare the efficiency of using the StringBuffer class versus using the plus sign.
The results of the above example are as follows:
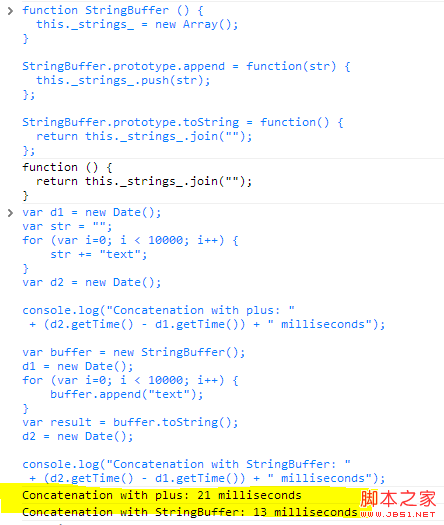
Then some people may say that the String object in JavaScript also encapsulates a concat() method. We will also use the concat() method below to do the same thing. Enter the following code in the consoel:
var d1 = new Date();
var str = "";
for (var i=0; i < 10000; i ) {
str.concat("text");
}
var d2 = new Date();
console.log("Concatenation with plus: "
(d2.getTime() - d1.getTime()) " milliseconds");
We can see that it is done 10000 times The time it takes to concatenate characters is:
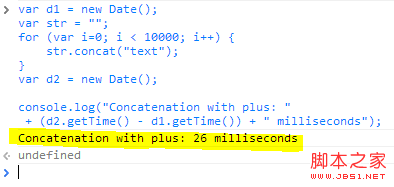
It can be concluded that when it comes to a certain number of string connections, we can improve performance by encapsulating a StringBuffer object (function) similar to Java in Javascript to perform operations.