HTML5 drag and drop
Drag and drop are part of the HTML5 standard.
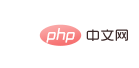
#Drag the php Chinese website icon into the rectangular box.
Drag and drop
Drag and drop is a common feature where you grab an object and then drag it to another location.
In HTML5, drag and drop is part of the standard, and any element can be dragged and dropped.
Browser support
Internet Explorer 9+, Firefox, Opera, Chrome, and Safari support dragging.
Note:Safari 5.1.2 does not support dragging.
HTML5 Drag and Drop Example
The following example is a simple drag Place instance:
Instance
<!DOCTYPE HTML> <html> <head> <meta charset="utf-8"> <title>php中文网(php.cn)</title> <style type="text/css"> #div1 {width:350px;height:70px;padding:10px;border:1px solid #aaaaaa;} </style> <script> function allowDrop(ev) { ev.preventDefault(); } function drag(ev) { ev.dataTransfer.setData("Text",ev.target.id); } function drop(ev) { ev.preventDefault(); var data=ev.dataTransfer.getData("Text"); ev.target.appendChild(document.getElementById(data)); } </script> </head> <body> <p>拖动 php中文网 图片到矩形框中:</p> <div id="div1" ondrop="drop(event)" ondragover="allowDrop(event)"></div> <br> <img id="drag1" src="https://img.php.cn/upload/course/000/000/010/58043146a1da1979.jpg" draggable="true" ondragstart="drag(event)" width="336" height="69"> </body> </html>
Run instance»
Click the "Run instance" button to view the online instance
It may look a bit complicated, but we can study different parts of the drag and drop event separately.
Set the element to be draggable
First, in order to make the element draggable, set the draggable attribute to true:
What to drag - ondragstart and setData()
Then, specify that when the element is dragged, what happens.
In the above example, the ondragstart attribute calls a function, drag(event), which specifies the data to be dragged.
dataTransfer.setData() method sets the data type and value of the dragged data:
{
ev.dataTransfer.setData("Text",ev.target.id);
}
In this example, the data type is "Text" and the value is the id of the draggable element ("drag1").
Where to put it - ondragover
The ondragover event specifies where to place the dragged data.
By default, data/elements cannot be placed into other elements. If we need to allow placement, we must prevent the default handling of the element.
This is done by calling the event.preventDefault() method of the ondragover event:
Place - ondrop
When the dragged data is placed, the drop event will occur.
In the above example, the ondrop attribute calls a function, drop(event):
function drop(ev) { ev.preventDefault(); var data=ev.dataTransfer.getData("Text"); ev.target.appendChild(document.getElementById(data)); }code explanation:
Call preventDefault() to avoid the browser's default processing of data (the default behavior of the drop event is to open it as a link)
Through dataTransfer.getData ("Text") method to obtain the dragged data. This method will return any data set to the same type in the setData() method.
The dragged data is the id of the dragged element ("drag1")
Append the dragged element to the placement element (target element) Medium
More examples
Drag and drop images back and forth
How to drag and drop images between two <div> elements.
Instance
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>php中文网(php.cn)</title> <style type="text/css"> #div1, #div2 {float:left; width:100px; height:35px; margin:10px;padding:10px;border:1px solid #aaaaaa;} </style> <script> function allowDrop(ev) { ev.preventDefault(); } function drag(ev) { ev.dataTransfer.setData("Text",ev.target.id); } function drop(ev) { ev.preventDefault(); var data=ev.dataTransfer.getData("Text"); ev.target.appendChild(document.getElementById(data)); } </script> </head> <body> <div id="div1" ondrop="drop(event)" ondragover="allowDrop(event)"> <img src="https://img.php.cn/upload/course/000/000/010/58049330dcf69494.png" draggable="true" ondragstart="drag(event)" id="drag1" width="88" height="31"></div> <div id="div2" ondrop="drop(event)" ondragover="allowDrop(event)"></div> </body> </html>
Run Instance»
Click the "Run Instance" button to view the online instance
##