Prérequis
Avant de commencer, assurez-vous d'avoir une compréhension de base de Next.js et de React.
1. Création de la route API backend
Nous allons créer une route API Next.js qui interagit avec notre API de géolocalisation.
Créez un nouveau fichier à l'adresse : src/app/api/geolocation/route.ts
import { NextResponse } from "next/server"; import axios from "axios"; type IPGeolocation = { ip: string; version?: string; city?: string; region?: string; region_code?: string; country_code?: string; country_code_iso3?: string; country_fifa_code?: string; country_fips_code?: string; country_name?: string; country_capital?: string; country_tld?: string; country_emoji?: string; continent_code?: string; in_eu: boolean; land_locked: boolean; postal?: string; latitude?: number; longitude?: number; timezone?: string; utc_offset?: string; country_calling_code?: string; currency?: string; currency_name?: string; languages?: string; country_area?: number; asn?: string; // Append ?fields=asn to the URL isp?: string; // Append ?fields=isp to the URL } type IPGeolocationError = { code: string; error: string; } export async function GET() { // Retrieve IP address using the getClientIp function // For testing purposes, we'll use a fixed IP address // const clientIp = getClientIp(req.headers); const clientIp = "84.17.50.173"; if (!clientIp) { return NextResponse.json( { error: "Unable to determine IP address" }, { status: 400 } ); } const key = process.env.IPFLARE_API_KEY; if (!key) { return NextResponse.json( { error: "IPFlare API key is not set" }, { status: 500 } ); } try { const response = await axios.get<ipgeolocation ipgeolocationerror>( `https://api.ipflare.io/${clientIp}`, { headers: { "X-API-Key": key, }, } ); if ("error" in response.data) { return NextResponse.json({ error: response.data.error }, { status: 400 }); } return NextResponse.json(response.data); } catch { return NextResponse.json( { error: "Internal Server Error" }, { status: 500 } ); } } </ipgeolocation>
2. Obtention de votre clé API
Nous allons utiliser un service de géolocalisation gratuit appelé IP Flare. Visitez la page Clés API : accédez à la page Clés API.
Visite : www.ipflare.io
À partir de la page Clés API, nous pouvons obtenir notre clé API et utiliser la copie rapide pour la stocker en tant que variable d'environnement dans notre fichier .env. Nous l'utiliserons pour authentifier nos demandes.
3. Création du composant frontend
J'ai créé ce composant tout-en-un qui inclut le fournisseur et le sélecteur de devises. J'utilise shadcn/ui et quelques SVG de drapeaux que j'ai trouvés en ligne.
Vous devrez envelopper l'application dans le fichier
Maintenant, n'importe où dans l'application où nous voulons accéder à la devise, nous pouvons utiliser le hook const { monnaie } = useCurrency();.
Pour intégrer cela à Stripe, lorsque vous créez le paiement, il vous suffit d'envoyer la devise et de vous assurer que vous avez ajouté des tarifs multidevises à vos produits Stripe.
"use client"; import { useRouter } from "next/navigation"; import { createContext, type FC, type ReactNode, useContext, useEffect, useMemo, useState, } from "react"; import axios from "axios"; // 1) Import axios import { Flag } from "~/components/flag"; import { Select, SelectContent, SelectGroup, SelectItem, SelectTrigger, SelectValue, } from "~/components/ui/select"; import { cn } from "~/lib/utils"; import { type Currency } from "~/server/schemas/currency"; // -- [1] Create a local type for the data returned by /api/geolocation. type GeolocationData = { country_code?: string; continent_code?: string; currency?: string; }; type CurrencyContext = { currency: Currency; setCurrency: (currency: Currency) => void; }; const CurrencyContext = createContext<currencycontext null>(null); export function useCurrency() { const context = useContext(CurrencyContext); if (!context) { throw new Error("useCurrency must be used within a CurrencyProvider."); } return context; } export const CurrencyProvider: FC = ({ children }) => { const router = useRouter(); // -- [2] Local state for geolocation data const [location, setLocation] = useState<geolocationdata null>(null); const [isLoading, setIsLoading] = useState<boolean>(true); // -- [3] Fetch location once when the component mounts useEffect(() => { const fetchLocation = async () => { setIsLoading(true); try { const response = await axios.get("/api/geolocation"); setLocation(response.data); } catch (error) { console.error(error); } finally { setIsLoading(false); } }; void fetchLocation(); }, []); // -- [4] Extract currency from location if present (fallback to "usd") const geoCurrency = location?.currency; const getInitialCurrency = (): Currency => { if (typeof window !== "undefined") { const cookie = document.cookie .split("; ") .find((row) => row.startsWith("currency=")); if (cookie) { const value = cookie.split("=")[1]; if (value === "usd" || value === "eur" || value === "gbp") { return value; } } } return "usd"; }; const [currency, setCurrencyState] = useState<currency>(getInitialCurrency); useEffect(() => { if (!isLoading && geoCurrency !== undefined) { const validatedCurrency = validateCurrency(geoCurrency, location); if (validatedCurrency) { setCurrency(validatedCurrency); } } // eslint-disable-next-line react-hooks/exhaustive-deps }, [isLoading, location, geoCurrency]); // -- [5] Update currency & store cookie; no more tRPC invalidation const setCurrency = (newCurrency: Currency) => { setCurrencyState(newCurrency); if (typeof window !== "undefined") { document.cookie = `currency=${newCurrency}; path=/; max-age=${ 60 * 60 * 24 * 365 }`; // Expires in 1 year } // Removed tRPC invalidate since we are no longer using tRPC router.refresh(); }; const contextValue = useMemo<currencycontext>( () => ({ currency, setCurrency, }), [currency], ); return ( <currencycontext.provider value="{contextValue}"> {children} </currencycontext.provider> ); }; export const CurrencySelect = ({ className }: { className?: string }) => { const { currency, setCurrency } = useCurrency(); return ( <select value="{currency}" onvaluechange="{setCurrency}"> <selecttrigger classname='{cn("w-[250px]",'> <selectvalue placeholder="Select a currency"></selectvalue> </selecttrigger> <selectcontent> <selectgroup classname="text-sm"> <selectitem value="usd"> <div classname="flex items-center gap-3"> <flag code="US" classname="h-4 w-4 rounded"></flag> <span>$ USD</span> </div> </selectitem> <selectitem value="eur"> <div classname="flex items-center gap-3"> <flag code="EU" classname="h-4 w-4 rounded"></flag> <span>€ EUR</span> </div> </selectitem> <selectitem value="gbp"> <div classname="flex items-center gap-3"> <flag code="GB" classname="h-4 w-4 rounded"></flag> <span>£ GBP</span> </div> </selectitem> </selectgroup> </selectcontent> </select> ); }; // -- [6] Use our new GeolocationData type in place of RouterOutputs const validateCurrency = ( currency: string, location?: GeolocationData | null, ): Currency | null => { if (currency === "usd" || currency === "eur" || currency === "gbp") { return currency; } if (!location) { return null; } if (location.country_code === "GB") { return "gbp"; } // Check if they are in the EU if (location.continent_code === "EU") { return "eur"; } // North America if (location.continent_code === "NA") { return "usd"; } return null; }; </currencycontext></currency></boolean></geolocationdata></currencycontext>
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!
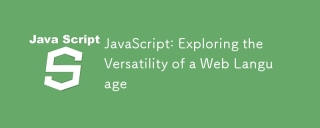
JavaScript est le langage central du développement Web moderne et est largement utilisé pour sa diversité et sa flexibilité. 1) Développement frontal: construire des pages Web dynamiques et des applications à une seule page via les opérations DOM et les cadres modernes (tels que React, Vue.js, Angular). 2) Développement côté serveur: Node.js utilise un modèle d'E / S non bloquant pour gérer une concurrence élevée et des applications en temps réel. 3) Développement des applications mobiles et de bureau: le développement de la plate-forme multiplateuse est réalisé par réact noral et électron pour améliorer l'efficacité du développement.
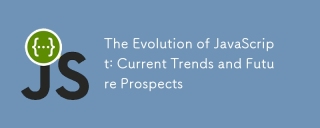
Les dernières tendances de JavaScript incluent la montée en puissance de TypeScript, la popularité des frameworks et bibliothèques modernes et l'application de WebAssembly. Les prospects futurs couvrent des systèmes de type plus puissants, le développement du JavaScript côté serveur, l'expansion de l'intelligence artificielle et de l'apprentissage automatique, et le potentiel de l'informatique IoT et Edge.
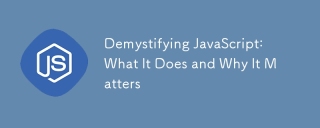
JavaScript est la pierre angulaire du développement Web moderne, et ses principales fonctions incluent la programmation axée sur les événements, la génération de contenu dynamique et la programmation asynchrone. 1) La programmation axée sur les événements permet aux pages Web de changer dynamiquement en fonction des opérations utilisateur. 2) La génération de contenu dynamique permet d'ajuster le contenu de la page en fonction des conditions. 3) La programmation asynchrone garantit que l'interface utilisateur n'est pas bloquée. JavaScript est largement utilisé dans l'interaction Web, les applications à une page et le développement côté serveur, améliorant considérablement la flexibilité de l'expérience utilisateur et du développement multiplateforme.
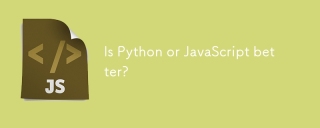
Python est plus adapté à la science des données et à l'apprentissage automatique, tandis que JavaScript est plus adapté au développement frontal et complet. 1. Python est connu pour sa syntaxe concise et son écosystème de bibliothèque riche, et convient à l'analyse des données et au développement Web. 2. JavaScript est le cœur du développement frontal. Node.js prend en charge la programmation côté serveur et convient au développement complet.
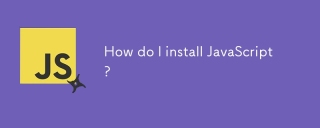
JavaScript ne nécessite pas d'installation car il est déjà intégré à des navigateurs modernes. Vous n'avez besoin que d'un éditeur de texte et d'un navigateur pour commencer. 1) Dans l'environnement du navigateur, exécutez-le en intégrant le fichier HTML via des balises. 2) Dans l'environnement Node.js, après avoir téléchargé et installé Node.js, exécutez le fichier JavaScript via la ligne de commande.
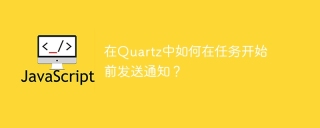
Comment envoyer à l'avance des notifications de tâches en quartz lors de l'utilisation du minuteur de quartz pour planifier une tâche, le temps d'exécution de la tâche est défini par l'expression CRON. Maintenant...
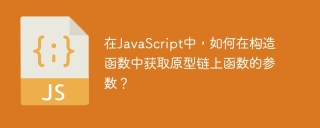
Comment obtenir les paramètres des fonctions sur les chaînes prototypes en JavaScript dans la programmation JavaScript, la compréhension et la manipulation des paramètres de fonction sur les chaînes prototypes est une tâche commune et importante ...
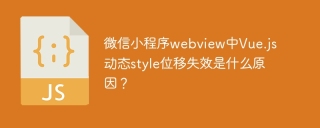
Analyse de la raison pour laquelle la défaillance du déplacement de style dynamique de l'utilisation de Vue.js dans la vue Web de l'applet WeChat utilise Vue.js ...


Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

AI Hentai Generator
Générez AI Hentai gratuitement.

Article chaud

Outils chauds

mPDF
mPDF est une bibliothèque PHP qui peut générer des fichiers PDF à partir de HTML encodé en UTF-8. L'auteur original, Ian Back, a écrit mPDF pour générer des fichiers PDF « à la volée » depuis son site Web et gérer différentes langues. Il est plus lent et produit des fichiers plus volumineux lors de l'utilisation de polices Unicode que les scripts originaux comme HTML2FPDF, mais prend en charge les styles CSS, etc. et présente de nombreuses améliorations. Prend en charge presque toutes les langues, y compris RTL (arabe et hébreu) et CJK (chinois, japonais et coréen). Prend en charge les éléments imbriqués au niveau du bloc (tels que P, DIV),

SublimeText3 Linux nouvelle version
Dernière version de SublimeText3 Linux
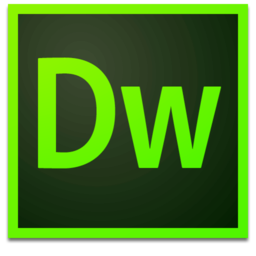
Dreamweaver Mac
Outils de développement Web visuel

SublimeText3 version anglaise
Recommandé : version Win, prend en charge les invites de code !

DVWA
Damn Vulnerable Web App (DVWA) est une application Web PHP/MySQL très vulnérable. Ses principaux objectifs sont d'aider les professionnels de la sécurité à tester leurs compétences et leurs outils dans un environnement juridique, d'aider les développeurs Web à mieux comprendre le processus de sécurisation des applications Web et d'aider les enseignants/étudiants à enseigner/apprendre dans un environnement de classe. Application Web sécurité. L'objectif de DVWA est de mettre en pratique certaines des vulnérabilités Web les plus courantes via une interface simple et directe, avec différents degrés de difficulté. Veuillez noter que ce logiciel