publié initialement : souvikinator.xyz
Voici comment configurer des tests unitaires pour les points de terminaison de l'API.
Nous utiliserons les packages NPM suivants :
- Express pour le serveur (tout autre framework peut être utilisé)
- Blague
- Supertest
Extension VS Code utilisant :
- Blague
- Jest Runner
Installation des dépendances
npm install express
npm install -D jest supertest
Voici à quoi ressemble la structure des répertoires :
et n'oublions pas package.json
{ "name": "api-unit-testing", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "jest api.test.js", "start":"node server.js" }, "keywords": [], "author": "", "license": "ISC", "dependencies": { "express": "^4.18.1" }, "devDependencies": { "jest": "^28.1.2", "supertest": "^6.2.3" } }
Création d'un serveur express simple
Un serveur express qui accepte les requêtes POST avec un corps et un titre sur le point de terminaison /post. Si l'un ou l'autre est manquant, le statut 400 Bad Request est renvoyé.
// app.js const express = require("express"); const app = express(); app.use(express.json()); app.post("/post", (req, res) => { const { title, body } = req.body; if (!title || !body) return res.sendStatus(400).json({ error: "title and body is required" }); return res.sendStatus(200); }); module.exports = app;
const app = require("./app"); const port = 3000; app.listen(port, () => { console.log(`http://localhost:${port} ?`); });
L'application express est exportée pour être utilisée avec supertest. La même chose peut être faite avec d'autres frameworks de serveurs HTTP.
Création d'un fichier de test
// api.test.js const supertest = require("supertest"); const app = require("./app"); // express app describe("Creating post", () => { // test cases goes here });
Supertest s'occupe de faire fonctionner le serveur et de faire des requêtes pour nous, pendant que nous nous concentrons sur les tests.
L'application express est transmise à l'agent supertest et elle lie express à un port disponible aléatoire. Ensuite, nous pouvons faire une requête HTTP au point de terminaison de l'API souhaité et comparer son état de réponse avec nos attentes :
const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", });
Nous allons créer un test pour 3 cas :
- Lorsque le titre et le corps sont fournis
test("creating new post when both title and body are provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", }); // comparing response status code with expected status code expect(response.statusCode).toBe(200); });
- Quand l'un d'eux est porté disparu
test("creating new post when either of the data is not provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", }); expect(response.statusCode).toBe(400); });
- Quand ils manquent tous les deux
test("creating new post when no data is not provided", async () => { const response = await supertest.agent(app).post("/post").send(); expect(response.statusCode).toBe(400); });
et le code final devrait ressembler à :
const supertest = require("supertest"); const app = require("./app"); // express app describe("Creating post", () => { test("creating new post when both title and body are provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", }); expect(response.statusCode).toBe(200); }); test("creating new post when either of the data is not provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", }); expect(response.statusCode).toBe(400); }); test("creating new post when no data is not provided", async () => { const response = await supertest.agent(app).post("/post").send(); expect(response.statusCode).toBe(400); }); });
Test en cours
Exécutez le test à l'aide de la commande suivante :
npm run test
Si vous utilisez des extensions VS Code comme jest et jest runner, il fera le travail à votre place.
Et c'est ainsi que nous pouvons facilement tester les points de terminaison de l'API. ?
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!
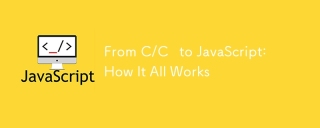
Le passage de C / C à JavaScript nécessite de s'adapter à la frappe dynamique, à la collecte des ordures et à la programmation asynchrone. 1) C / C est un langage dactylographié statiquement qui nécessite une gestion manuelle de la mémoire, tandis que JavaScript est dynamiquement typé et que la collecte des déchets est automatiquement traitée. 2) C / C doit être compilé en code machine, tandis que JavaScript est une langue interprétée. 3) JavaScript introduit des concepts tels que les fermetures, les chaînes de prototypes et la promesse, ce qui améliore la flexibilité et les capacités de programmation asynchrones.
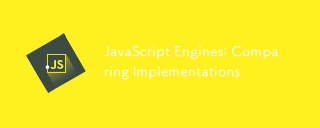
Différents moteurs JavaScript ont des effets différents lors de l'analyse et de l'exécution du code JavaScript, car les principes d'implémentation et les stratégies d'optimisation de chaque moteur diffèrent. 1. Analyse lexicale: convertir le code source en unité lexicale. 2. Analyse de la grammaire: générer un arbre de syntaxe abstrait. 3. Optimisation et compilation: générer du code machine via le compilateur JIT. 4. Exécuter: Exécutez le code machine. Le moteur V8 optimise grâce à une compilation instantanée et à une classe cachée, SpiderMonkey utilise un système d'inférence de type, résultant en différentes performances de performances sur le même code.
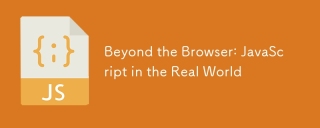
Les applications de JavaScript dans le monde réel incluent la programmation côté serveur, le développement des applications mobiles et le contrôle de l'Internet des objets: 1. La programmation côté serveur est réalisée via Node.js, adaptée au traitement de demande élevé simultané. 2. Le développement d'applications mobiles est effectué par le reactnatif et prend en charge le déploiement multiplateforme. 3. Utilisé pour le contrôle des périphériques IoT via la bibliothèque Johnny-Five, adapté à l'interaction matérielle.
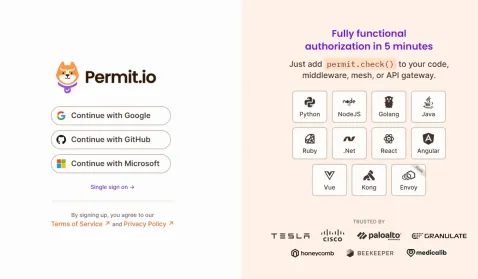
J'ai construit une application SAAS multi-locataire fonctionnelle (une application EdTech) avec votre outil technologique quotidien et vous pouvez faire de même. Premièrement, qu'est-ce qu'une application SaaS multi-locataire? Les applications saas multi-locataires vous permettent de servir plusieurs clients à partir d'un chant
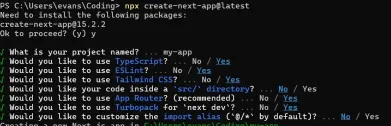
Cet article démontre l'intégration frontale avec un backend sécurisé par permis, construisant une application fonctionnelle EdTech SaaS en utilisant Next.js. Le frontend récupère les autorisations des utilisateurs pour contrôler la visibilité de l'interface utilisateur et garantit que les demandes d'API adhèrent à la base de rôles
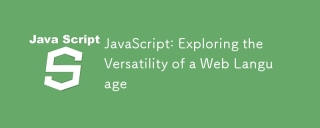
JavaScript est le langage central du développement Web moderne et est largement utilisé pour sa diversité et sa flexibilité. 1) Développement frontal: construire des pages Web dynamiques et des applications à une seule page via les opérations DOM et les cadres modernes (tels que React, Vue.js, Angular). 2) Développement côté serveur: Node.js utilise un modèle d'E / S non bloquant pour gérer une concurrence élevée et des applications en temps réel. 3) Développement des applications mobiles et de bureau: le développement de la plate-forme multiplateuse est réalisé par réact noral et électron pour améliorer l'efficacité du développement.
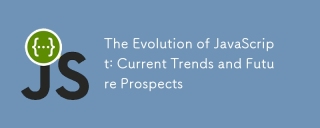
Les dernières tendances de JavaScript incluent la montée en puissance de TypeScript, la popularité des frameworks et bibliothèques modernes et l'application de WebAssembly. Les prospects futurs couvrent des systèmes de type plus puissants, le développement du JavaScript côté serveur, l'expansion de l'intelligence artificielle et de l'apprentissage automatique, et le potentiel de l'informatique IoT et Edge.
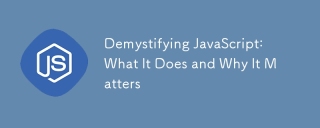
JavaScript est la pierre angulaire du développement Web moderne, et ses principales fonctions incluent la programmation axée sur les événements, la génération de contenu dynamique et la programmation asynchrone. 1) La programmation axée sur les événements permet aux pages Web de changer dynamiquement en fonction des opérations utilisateur. 2) La génération de contenu dynamique permet d'ajuster le contenu de la page en fonction des conditions. 3) La programmation asynchrone garantit que l'interface utilisateur n'est pas bloquée. JavaScript est largement utilisé dans l'interaction Web, les applications à une page et le développement côté serveur, améliorant considérablement la flexibilité de l'expérience utilisateur et du développement multiplateforme.


Outils d'IA chauds

Undresser.AI Undress
Application basée sur l'IA pour créer des photos de nu réalistes

AI Clothes Remover
Outil d'IA en ligne pour supprimer les vêtements des photos.

Undress AI Tool
Images de déshabillage gratuites

Clothoff.io
Dissolvant de vêtements AI

AI Hentai Generator
Générez AI Hentai gratuitement.

Article chaud

Outils chauds

Listes Sec
SecLists est le compagnon ultime du testeur de sécurité. Il s'agit d'une collection de différents types de listes fréquemment utilisées lors des évaluations de sécurité, le tout en un seul endroit. SecLists contribue à rendre les tests de sécurité plus efficaces et productifs en fournissant facilement toutes les listes dont un testeur de sécurité pourrait avoir besoin. Les types de listes incluent les noms d'utilisateur, les mots de passe, les URL, les charges utiles floues, les modèles de données sensibles, les shells Web, etc. Le testeur peut simplement extraire ce référentiel sur une nouvelle machine de test et il aura accès à tous les types de listes dont il a besoin.
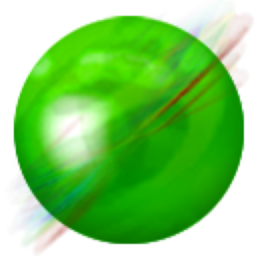
ZendStudio 13.5.1 Mac
Puissant environnement de développement intégré PHP

Télécharger la version Mac de l'éditeur Atom
L'éditeur open source le plus populaire

PhpStorm version Mac
Le dernier (2018.2.1) outil de développement intégré PHP professionnel
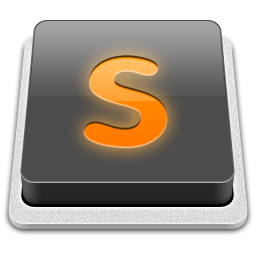
SublimeText3 version Mac
Logiciel d'édition de code au niveau de Dieu (SublimeText3)