


Introduction to JavaScript Design Patterns and Prototype Patterns (Object.create and prototype)_javascript skills
Prototype Mode Description
Description: Use prototype instances to copy and create new customizable objects; for new objects, you do not need to know the specific process of creating the original object;
Process: Prototype => new ProtoExam => clone to new Object;
Use relevant code:
function Prototype() {
This.name = '';
This.age = '';
This.sex = '';
}
Prototype.prototype.userInfo = function() {
Return 'Personal information, name: ' this.name ', age: ' this.age ', gender: ' this.sex '
';
}
Two or more personal information contents are now required:
var proto = new Prototype();
var person1 = Object.create(proto);
person1.name = 'Xiao Ming';
person1.sex = 'Male';
person1.age = 35;
person1.userInfo();
//
var person2 = Object.create(proto);
person2.name = 'Xiaohua';
person2.sex = 'Female';
person2.age = 33;
person2.userInfo();
Output return:
Personal information, Name: Xiao Ming, Age: 35, Gender: Male
Personal information, Name: Xiaohua, Age: 33, Gender: Female
Prototype mode is generally used when the abstract structure is complex, but the content composition is similar, the abstract content can be customized, and the newly created object only needs to be slightly modified to meet the requirements;
Object.create Instructions
1>. Definition: Create an object that can specify a prototype object and can contain optional custom properties;
2> Object.create(proto [, properties]); Optional, used to configure the properties of the new object;
1. proto: To create a prototype of a new object, it is required and can be null; this proto is only valuable if it has already been created [new] or object.prototype;
2. properties: optional, structure:
{
propField: {
value: 'val'|{}|function(){},
writable: true|false,
enumerable: true|false,
Configurable: true|false,
get:function(){return 10},
set:function(value){}
}
}
Custom attributes have the following four native attributes:
value: custom attribute value;
writable: Whether the value of this item is editable, the default is false, when it is true, obj.prodField can be assigned a value; otherwise it is read-only;
enumerable: enumerable;
confirurable: configurable;
Can also contain set, get accessor methods;
Among them, [set, get] and value and writable cannot appear at the same time;
1. Create prototype object class:
function ProtoClass(){
This.a = 'ProtoClass';
This.c = {};
This.b = function() {
}
}
Create prototype method:
ProtoClass.prototype.aMethod = function() {
//this.a;
//this.b();
Return this.a;
}
How to use
1. Create an object with ProtoClass.prototype;
var obj1 = Object.create(ProtoClass.prototype, {
foo:{value: 'obj1', writable: true}
})
obj1 has the ProtoClass prototype method aMethod;
obj1.aMethod();
//It will output undefined, the method is accessible, and the ProtoClass members are inaccessible
However, this method cannot implement the member attributes of a, b, c under ProtoClass:
2. Use instantiated ProtoClass as prototype:
var proto = new ProtoClass();
var obj2 = Object.create(proto, {
foo:{value:'obj2'}
});
The obj2 created in this way has all the member attributes a, b, c and aMethod prototype method of ProtoClass; and adds a foo read-only data attribute;
obj2.a; //ProtoClass
obj2.c: //[Object]
obj2.b(); //
obj2.aMethod(); //ProtoClass
obj2.foo; //obj2
3. Subclass inheritance:
function SubClass() {
}
SubClass.prototype = Object.create(ProtoClass.prototype ,{
foo:{value: 'subclass'}
});
SubClass.prototype.subMethod = function() {
Return this.a || this.foo;
}
This method can be inherited to the aMethod method of ProtoClass and executed;
var func = new SubClass();
func.aMethod() ;//undefined, cannot read the member attributes of ProtoClass, a, b, c
func.subMethod();//subclass
To allow SubClass to read the member attributes of ProtoClass, SubClass needs to be changed:
function SubClass()
{
ProtoClass.call(this);
}
//Other codes;
This method can obtain the member attributes and prototype methods of ProtoClass;:
var func = new SubClass();
func.aMethod() ;//ProtoClass
func.subMethod();//ProtoClass
Another method is to use an instantiated ProtoClass object as the prototype of SubClass;
var proto = new ProtoClass();
function SubClass() {
}
SubClass.prototype = Object.create(proto, {
foo:{value: 'subclass'}
});
In this way, after SubClass is instantiated, you can obtain all the properties and prototype methods of ProtoClass, and create a read-only data attribute foo;
var func = new SubClass();
func.foo; //subclass
func.a; //ProtoClass
func.b(); //
func.c; //[Object]
func.aMethod(); //ProtoClass
4. Another creation inheritance method has the same effect as Object.create using instantiated ProtoClass as prototype:
function SubClass() {
this.foo = 'subclass'; //But it can be read and written here
}
SubClass.prototype = new ProtoClass();
Object.create related instructions
Object.create is used to create a new object. When it is Object, the prototype is null, and the function is the same as new Object(); or {};
When it is a function, it has the same effect as new FunctionName;
//1 Object
var o = {}
//Equivalent to
var o2 = Object.create({});
//Both constructors are the same;
//----------------------------------------
function func() {
This.a = 'func';
}
func.prototype.method = function() {
Return this.a;
}
var newfunc = new func();
//Equivalent to [same effect]
var newfunc2 = Object.create(Object.prototype/*Function.prototype||function(){}*/, {
a: {value:'func', writable:true},
Method: {value: function() {return this.a;} }
});
But newfunc and newfunc2 have different function references in the objects that create them.
newfunc is function func() {...}, newfunc2 is function Function {Native}
Object.create(proto[, propertiesField]):
proto description, this value is required and can be null. If not set, an exception will be thrown;
If proto is non-null, it is an instantiated value, that is, a value that has been new; most objects in JavaScript have a constructor attribute, which indicates which function this object is instantiated through;
propertiesField is optional and sets the member properties or methods that the newly created object may need;
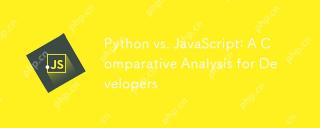
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
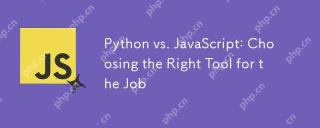
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
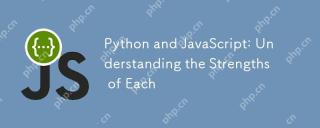
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
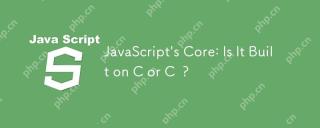
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
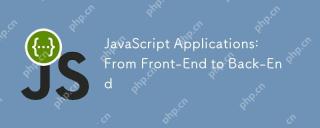
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
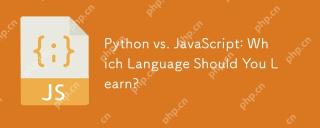
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
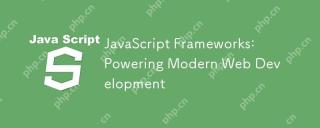
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
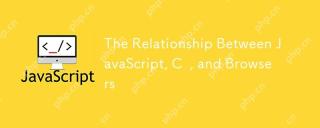
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
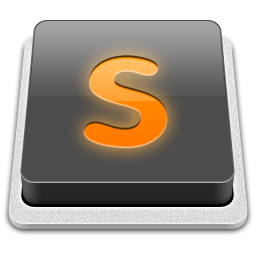
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
