Array array
1. Introduction
An array is an ordered collection of values. Each value is called an element, and each element has a position in the array, represented by a number, called an index. JavaScript arrays are untyped: array elements can be of any type, and different elements in the same array may have different types. --"The Definitive Guide to JavaScript (6th Edition)"
2. Definition
var names = new Array("Zhang San", "Li Si", "Wang Wu");
//or
var names = ["Zhang San", "Li Si", "Wang Wu"];
3. Attributes
length: Indicates the length of elements in the array.
4. Instance methods
Common methods:
1) unshift(): Insert elements at the head of the array
2) shift(): Remove and return the first element of the array
3) push(): Insert elements at the end of the array
4) pop(): Remove and return the last element of the array
4.1 concat(): Connect elements to an array. The original array will not be modified and a new array will be returned
Parameters:
①value1,value2....valueN: any number of values
Return value:
{Array} A new array, containing the original Array and the newly added elements.
Example:
var demoArray = ['a', 'b', 'c'];
var demoArray2 = demoArray.concat('e');
console.log(demoArray); // => demoArray:['a','b','c'] The original array does not change
console.log(demoArray2); // => ['a','b','c','e']
4.2 every(): Traverse the elements in sequence and determine whether each element is true
Parameters:
①function(value,index,self){}: Each element will use this function to determine whether it is true. When one is determined to be false, the traversal will end immediately.
Value: elements of array traversal
Index: element number
Self: Array itself
Return value:
{Boolean}: Returns true only if every element is true; returns false as long as one element is false.
Example:
var demoArray = [1, 2, 3];
var rs = demoArray.every(function (value, index, self) {
Return value > 0;
});
console.log(rs); // => true
4.3 filter(): Traverse the elements in sequence and return a new array containing elements that meet the conditions.
Parameters:
①function(value,index,self){}: Call this function on each element in turn and return a new array containing elements that meet the conditions.
Value: elements of array traversal
Index: element number
Self: Array itself
Return value:
{Array} A new array containing elements that meet the criteria
Example:
var demoArray = [1, 2, 3];
var rs = demoArray.filter(function (value, index, self) {
Return value > 0;
});
console.log(rs); // => [1, 2, 3]
4.4 forEach(): Traverse the elements in sequence and execute the specified function; no return value.
Parameters:
①function(value,index,self){}: Call this function for each element in turn
Value: elements of array traversal
Index: element number
Self: Array itself
Return value: None
Example:
var demoArray = [1, 2, 3];
demoArray.forEach(function (value, index, self) {
Console.log(value); // => Output in sequence: 1 2 3
});
4.5 indexOf(): Find matching elements in the array. If there is no matching element, -1 is returned. Use the "===" operator when searching, so you need to distinguish between 1 and '1'
Parameters:
①value: The value to be found in the array.
②start: The serial number position to start searching. If omitted, it will be 0.
Return value:
{Int}: Returns the serial number of the first matching value in the array. If it does not exist, returns -1
Example:
['a', 'b', 'c'].indexOf('a'); // =>0
['a', 'b', 'c'].indexOf('a', 1); // =>-1
['a', 'b', 'c'].indexOf('d'); // =>-1
[1, 2, 3].indexOf('1'); // => -1: '===' matching method used
4.6 join(): Splice all elements in the array into a string using a separator.
Parameters:
①sparator {String}: The separator between each element. If omitted, it will be separated by English commas ',' by default.
Return value:
{String}: Each element is spliced into a string with sparator as the separator.
Example:
['a', 'b', 'c'].join(); // => 'a,b,c'
['a', 'b', 'c'].join('-'); // => 'a-b-c'
4.7 lastIndexOf: Find matching elements in the reverse array. If there is no matching element, -1 is returned. Use the "===" operator when searching, so you need to distinguish between 1 and '1'
Parameters:
①value: The value to be found in the array.
②start: The serial number position to start searching. If omitted, the search will start from the last element.
Return value:
{Int}: Find the sequence number of the first matching value in the array from right to left. If it does not exist, return -1
Example:
['a', 'b', 'c'].lastIndexOf('a'); // => 0
['a', 'b', 'c'].lastIndexOf('a', 1); // => 0
['a', 'b', 'c'].lastIndexOf('d'); // => -1
[1, 2, 3].lastIndexOf('1'); // => -1: '===' matching method used
4.8 map(): Traverse and calculate each element in sequence, and return an array of calculated elements
Parameters:
①function(value,index,self){}: Each element calls this function in turn and returns the calculated element
Value: elements of array traversal
Index: element number
Self: Array itself
Return value:
{Array} A new array containing the good elements
Example:
[1, 2, 3].map(function (value, index, self) {
Return value * 2;
}); // => [2, 4, 6]
4.9 pop(): Remove and return the last element of the array
Parameters: None
Return value:
{Object} The last element of the array; if the array is empty, undefined
is returnedExample:
var demoArray = ['a', 'b', 'c'];
demoArray.pop(); // => c
demoArray.pop(); // => b
demoArray.pop(); // => a
demoArray.pop(); // => undefined
4.10 push(): Add elements to the end of the array
Parameters:
①value1,value2....valueN: Add any number of values to the end of the array
Return value:
{int} new length of array
Example:
var demoArray = ['a', 'b', 'c'];
demoArray.push('d'); // => 4, demoArray: ['a', 'b', 'c', 'd']
demoArray.push('e', 'f'); // => 6, demoArray: ['a', 'b', 'c', 'd', 'e', 'f']
console.log(demoArray); // => ['a', 'b', 'c', 'd', 'e', 'f']
4.11 reverse(): Reverse the order of array elements.
Parameters: None
Return value: None (reverse the order of elements in the original array).
Example:
var demoArray = ['a', 'b', 'c', 'd', 'e'];
demoArray.reverse();
console.log(demoArray); // => ["e", "d", "c", "b", "a"]
4.12 shift(): Remove and return the first element of the array
Parameters: None
Return value:
{Object} The first element of the array; if the array is empty, undefined is returned.
Example:
var demoArray = ['a', 'b', 'c'];
demoArray.shift(); // => a
demoArray.shift(); // => b
demoArray.shift(); // => c
demoArray.shift(); // => undefined
4.13 slice(startIndex,endIndex): Return a part of the array.
Parameters:
①startIndex: The serial number at the beginning; if it is a negative number, it means counting from the end, -1 represents the last element, -2 represents the second to last element, and so on.
②endIndex: The serial number after the element at the end. If not specified, it is the end. The intercepted element does not contain the element with the serial number here, and ends with the element before the serial number here.
Return value:
{Array} A new array containing all elements from startIndex to the previous element of endIndex.
Example:
[1, 2, 3, 4, 5, 6].slice(); // => [1, 2, 3, 4, 5, 6]
[1, 2, 3, 4, 5, 6].slice(1); // => [2, 3, 4, 5, 6]: start from sequence number 1
[1, 2, 3, 4, 5, 6].slice(0, 4); // => [1, 2, 3, 4]: intercept sequence number 0 to sequence number 3 (the one before sequence number 4) Element
[1, 2, 3, 4, 5, 6].slice(-2); // => [5, 6]: intercept the next 2 elements
4.14 sort(opt_orderFunc): Sort according to certain rules
Parameters:
①opt_orderFunc(v1,v2) {Function}: Optional sorting function. If omitted, the elements will be sorted alphabetically from small to large.
v1: The previous element when traversing.
v2: The following elements when traversing.
Sort:
Compare v1 and v2 and return a number to represent the sorting rules of v1 and v2:
Less than 0: v1 is smaller than v2, v1 is ranked in front of v2.
Equal to 0: v1 is equal to v2, v1 is ranked in front of v2.
Greater than 0: v1 is greater than v2, v1 is ranked behind v2.
Return value: None (sort operation in the original array).
Example:
[1, 3, 5, 2, 4, 11, 22].sort(); // => [1, 11, 2, 22, 3, 4, 5]: All elements here are converted to characters, The character of 11 comes before 2
[1, 3, 5, 2, 4, 11, 22].sort(function (v1, v2) {
Return v1 - v2;
}); // => [1, 2, 3, 4, 5, 11, 22]: Sort from small to large
[1, 3, 5, 2, 4, 11, 22].sort(function (v1, v2) {
Return -(v1 - v2); //If you negate it, you can convert it to From big to small
}); // => [22, 11, 5, 4, 3, 2, 1]
4.15 splice(): Insert and delete array elements
Parameters:
①start {int}: The starting sequence number to start inserting, deleting or replacing.
②deleteCount {int}: The number of elements to be deleted, counting from start.
③value1,value2 ... valueN {Object}: Optional parameter, indicating the element to be inserted, starting from start. If the ② parameter is not 0, then the deletion operation is performed first, and then the insertion operation is performed.
Return value:
{Array} Returns a new array containing the deleted elements. If the ② parameter is 0, it means that no elements are deleted and an empty array is returned.
Example:
// 1. Delete
var demoArray = ['a', 'b', 'c', 'd', 'e'];
var demoArray2 = demoArray.splice(0, 2); // Delete 2 elements starting from 0 and return an array containing the deleted elements: ['a', 'b']
console.log(demoArray2); // => ['a', 'b']
console.log(demoArray); // => ['c', 'd', 'e']
// 2. Insert
var demoArray = ['a', 'b', 'c', 'd', 'e'];
var demoArray2 = demoArray.splice(0, 0, '1', '2', '3'); // ②The parameter is 0 and an empty array is returned
console.log(demoArray2); // => [ ]
console.log(demoArray); // => ['1', '2', '3', 'a', 'b', 'c', 'd', 'e']
// 3. Delete first and then insert
var demoArray = ['a', 'b', 'c', 'd', 'e'];
// When the ② parameter is not 0, then perform the deletion operation first (delete the 4 elements starting from 0 and return an array containing the deleted elements), and then perform the insertion operation
var demoArray2 = demoArray.splice(0, 4, '1', '2', '3');
console.log(demoArray2); // => ['a', 'b', 'c', 'd']
console.log(demoArray); // => ['1', '2', '3', 'a', 'b', 'c', 'd', 'e']
4.16 toString(): Concatenate all elements in the array into a string through an English comma ','.
Parameters: None
Return value:
{String} All elements in the array are concatenated into a string through an English comma ',' and returned. The same as calling the join() method without parameters.
Example:
[1, 2, 3, 4, 5].toString(); // => '1,2,3,4,5'
['a', 'b', 'c', 'd', 'e'].toString(); // => 'a,b,c,d,e'
4.17 unshift(): Insert elements at the head of the array
Parameters:
①value1,value2....valueN: Add any number of values to the head of the array
Return value:
{int} new length of array
Example:
var demoArray = [];
demoArray.unshift('a'); // => demoArray:['a']
demoArray.unshift('b'); // => demoArray:['b', 'a']
demoArray.unshift('c'); // => demoArray:['c', 'b', 'a']
demoArray.unshift('d'); // => demoArray:['d', 'c', 'b', 'a']
demoArray.unshift('e'); // => demoArray:['e', 'd', 'c', 'b', 'a']
5. Static method
5.1 Array.isArray(): Determine whether the object is an array
Parameters:
①value {Object}: any object
Return value:
{Boolean} Returns the judgment result. When it is true, it means that the object is an array; when it is false, it means that the object is not an array
Example:
Array.isArray([]); // => true
Array.isArray(['a', 'b', 'c']); // => true
Array.isArray('a'); // => false
Array.isArray('[1, 2, 3]'); // => false
6. Practical operation
6.1 Index
Description: Each element has a position in the array, represented by a number, called an index. The index starts from 0, that is, the index of the first element is 0, the index of the second element is 1, and so on;
When getting an index that does not exist in the array, undefined is returned.
Example:
var demoArray = ['a', 'b', 'c', 'd', 'e'];
demoArray[0]; // => Get the first element: 'a'
demoArray[0] = 1; // Set the first element to 1
console.log(demoArray); // => demoArray:[1, 'b', 'c', 'd', 'e']
console.log(demoArray[9]); // => undefined: When the obtained index does not exist, return undefined
6.2 for statement
Note: You can traverse the array one by one through the for statement
Example:
var demoArray = ['a', 'b', 'c', 'd', 'e'];
for (var i = 0, length = demoArray.length; i console.log(demoArray[i]); // => Output the elements in the array one by one
}
6.3 Shallow Copy
Note: The Array type is a reference type; when array a is copied to array b, if the elements of array b are modified, array a will also be modified.
Example:
var demoArrayA = ['a', 'b', 'c', 'd', 'e'];
var demoArrayB = demoArrayA; // Assign array A to array B
demoArrayB[0] = 1; // Modify the elements of array B
console.log(demoArrayA); // => [1, 'b', 'c', 'd', 'e']: The elements of array A have also changed
6.4 Deep Copy
Description: Use the concat() method to return a new array; to prevent shallow copying, modify the elements of array b, and array a will not change.
Example:
var demoArrayA = ['a', 'b', 'c', 'd', 'e'];
var demoArrayB = demoArrayA.concat(); // Use the concat() method to return a new array
demoArrayB[0] = 1; // Modify the elements of array B
console.log(demoArrayA); // => ['a', 'b', 'c', 'd', 'e']: The elements of array A have not changed
console.log(demoArrayB); // => [ 1, 'b', 'c', 'd', 'e']: The elements of array B have changed
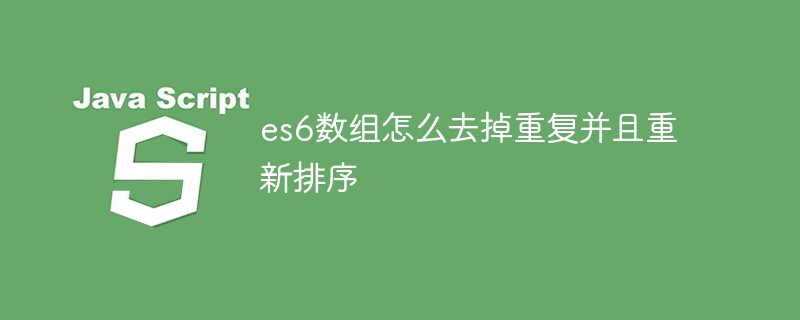
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
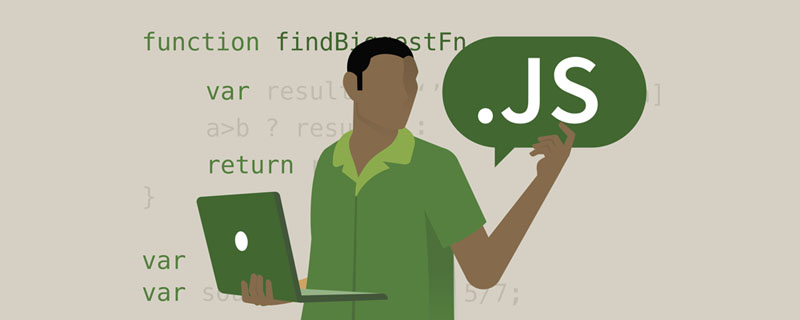
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
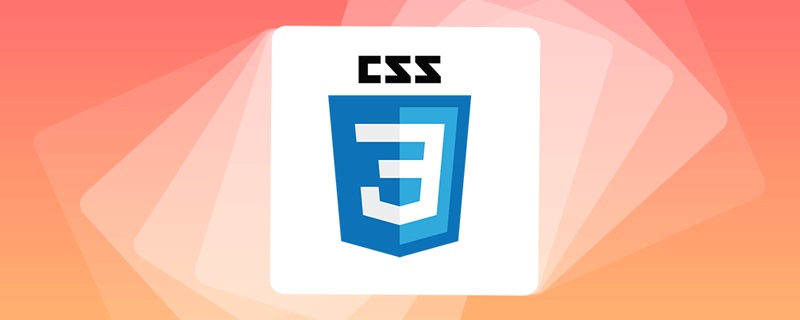
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
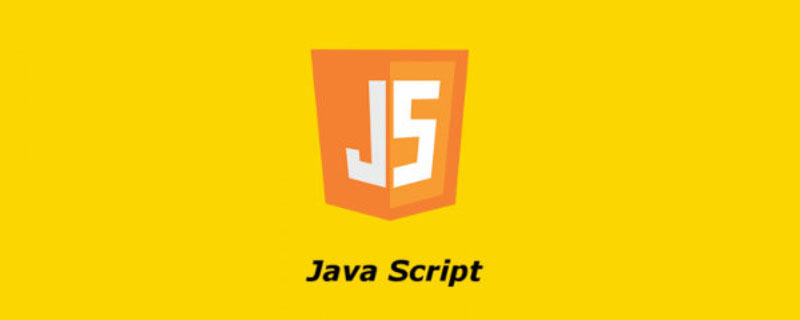
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
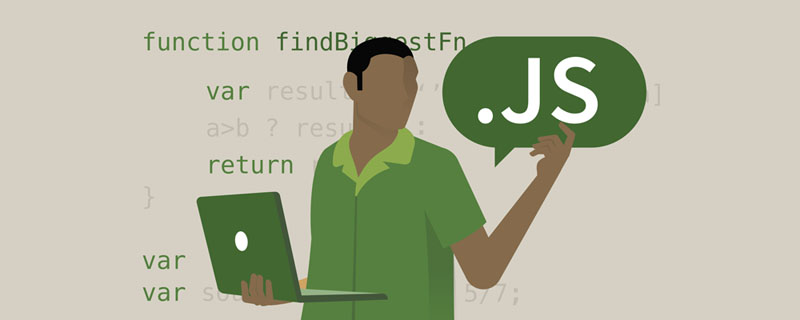
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
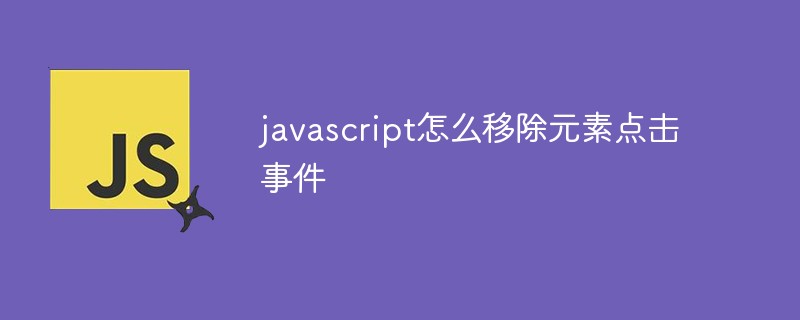
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
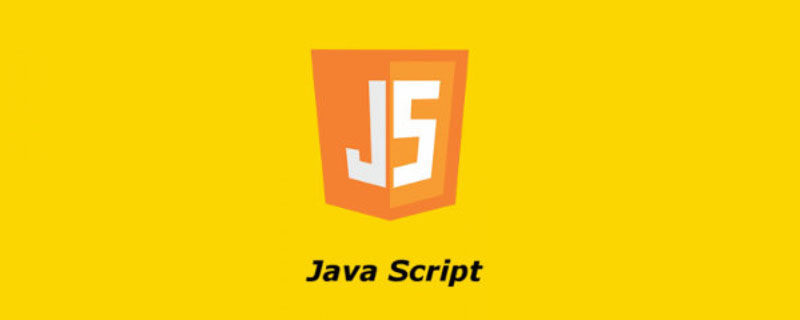
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
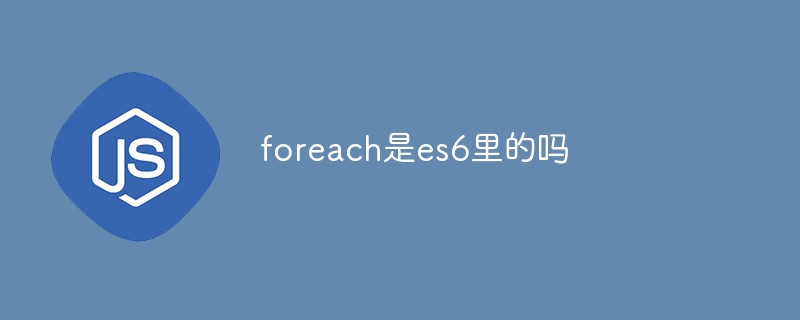
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
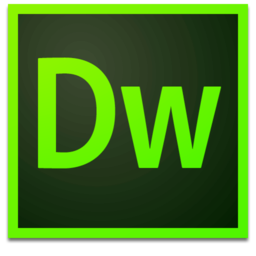
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
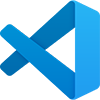
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
