A brief discussion on JavaScript Date and time objects_Basic knowledge
Date date and time object
1. Introduction
Date object is an object that operates on date and time. The Date object can only operate on date and time through methods.
2. Constructor
2.1 new Date(): Return the current local date and time
Parameters: None
Return value:
{Date} returns a Date object representing the local date and time.
Example:
var dt = new Date();
console.log(dt); // => Returns a Date object representing the local date and time
2.2 new Date(milliseconds): Convert milliseconds to Date object
Parameters:
①milliseconds {int}: Number of milliseconds; indicating the number of milliseconds starting from '1970/01/01 00:00:00' as the starting point.
Note: The current time zone must be added to the starting point. The time zone of Beijing time is East 8th District. The actual starting time is: '1970/01/01 08:00:00'
Return value:
{Date} returns a superimposed Date object.
Example:
var dt = new Date(1000 * 60 * 1); // Number of milliseconds to advance 1 minute
console.log(dt); // => {Date}:1970/01/01 08:01:00
dt = new Date(-1000 * 60 * 1); // Number of milliseconds to go back 1 minute
console.log(dt); // => {Date}:1970/01/01 07:59:00
2.3 new Date(dateStr): Convert string to Date object
Parameters:
①dateStr {string}: A string that can be converted into a Date object (time can be omitted); there are two main formats of strings:
1) yyyy/MM/dd HH:mm:ss (recommended): If the time is omitted, the time of the returned Date object is 00:00:00.
2) yyyy-MM-dd HH:mm:ss: If the time is omitted, the time of the returned Date object is 08:00:00 (plus the local time zone). If the time is not omitted, this string will fail to be converted in IE!
Return value:
{Date} returns a converted Date object.
Example:
var dt = new Date('2014/12/25'); // yyyy/MM/dd
console.log(dt); // => {Date}:2014/12/25 00:00:00
dt = new Date('2014/12/25 12:00:00'); // yyyy/MM/dd HH:mm:ss
console.log(dt); // => {Date}:2014/12/25 12:00:00
dt = new Date('2014-12-25'); // yyyy-MM-dd
console.log(dt); // => {Date}:2014-12-25 08:00:00 (Added the time zone of East 8th District)
dt = new Date('2014-12-25 12:00:00'); // yyyy-MM-dd HH:mm:ss (Note: This conversion method will report an error in IE!)
console.log(dt); // => {Date}:2014-12-25 12:00:00
2.4 new Date(year, month, opt_day, opt_hours, opt_minutes, opt_seconds, opt_milliseconds): Convert year, month, day, hours, minutes and seconds into Date objects
Parameters:
①year {int}: year; 4 digits. Such as: 1999, 2014
②month {int}: month; 2 digits. Calculation starts from 0, 0 represents January and 11 represents December.
③opt_day {int} Optional: number; 2 digits; counting from 1, 1 means No. 1.
④opt_hours {int} Optional: hours; 2 digits; value 0~23.
⑤opt_minutes {int} Optional: minutes; 2 digits; value 0~59.
⑥opt_seconds {int} Optional: seconds; 2 unnumbered; value 0~59.
⑦opt_milliseconds {int} Optional: milliseconds; value 0~999.
Return value:
{Date} returns a converted Date object.
Example:
var dt = new Date(2014, 11); // December 2014 (the month number entered here is 11)
console.log(dt); // => {Date}:2014/12/01 00:00:00
dt = new Date(2014, 11, 25); // December 25, 2014
console.log(dt); // => {Date}:2014/12/25 00:00:00
dt = new Date(2014, 11, 25, 15, 30, 40); // December 25, 2014 15:30:40
console.log(dt); // => {Date}:2014/12/25 15:30:40
dt = new Date(2014, 12, 25); // December 25, 2014 (the month number entered here is 12, which means the 13th month and jumps to January of the second year)
console.log(dt); // => {Date}:2015/01/25
3. Attributes
None; Date objects can only operate on date and time through methods.
4. Instance methods
The instance methods of Date objects are mainly divided into two forms: local time and UTC time. The same method generally operates on these two time formats (the method name with UTC is the operation of UTC time). Here we mainly introduce the operation of local time.
4.1 get method
4.1.1 getFullYear(): Returns the year value of the Date object; 4-digit year.
4.1.2 getMonth(): Returns the month value of the Date object. Starts from 0, so real month = return value 1 .
4.1.3 getDate(): Returns the date value in the month of the Date object; the value range is 1~31.
4.1.4 getHours(): Returns the hour value of the Date object.
4.1.5 getMinutes(): Returns the minute value of the Date object.
4.1.6 getSeconds(): Returns the seconds value of the Date object.
4.1.7 getMilliseconds(): Returns the millisecond value of the Date object.
4.1.8 getDay(): Returns the day of the week value of the Date object; 0 is Sunday, 1 is Monday, 2 is Tuesday, and so on
4.1.9 getTime(): Returns the millisecond value between the Date object and '1970/01/01 00:00:00' (the time zone of Beijing time is East 8th District, the starting time is actually: '1970/01 /01 08:00:00').
Example:
dt.getFullYear(); // => 2014: Year
dt.getMonth(); // => 11: month; actually December (month starts from 0)
dt.getDate(); // => 25: day
dt.getHours(); // => 15: Hours
dt.getMinutes(); // => 30: Minutes
dt.getSeconds(); // => 40: seconds
dt.getMilliseconds(); // => 333: milliseconds
dt.getDay(); // => 4: Day of the week value
dt.getTime(); // => 1419492640333: Returns the millisecond value between the Date object and '1970/01/01 00:00:00' (the time zone of Beijing time is East 8th District, the starting time is actually:' 1970/01/01 08:00:00')
4.2 set method
4.2.1 setFullYear(year, opt_month, opt_date): Set the year value of the Date object; 4-digit year.
4.2.2 setMonth(month, opt_date): Set the month value of the Date object. 0 represents January and 11 represents December.
4.2.3 setDate(date): Set the date value in the month of the Date object; the value range is 1~31.
4.2.4 setHours(hour, opt_min, opt_sec, opt_msec): Set the hour value of the Date object.
4.2.5 setMinutes(min, opt_sec, opt_msec): Set the minute value of the Date object.
4.2.6 setSeconds(sec, opt_msec): Set the seconds value of the Date object.
4.2.7 setMilliseconds(msec): Set the millisecond value of the Date object.
Example:
var dt = new Date();
dt.setFullYear(2014); // => 2014: Year
dt.setMonth(11); // => 11: month; actually December (month starts from 0)
dt.setDate(25); // => 25: day
dt.setHours(15); // => 15: Hours
dt.setMinutes(30); // => 30: Minutes
dt.setSeconds(40); // => 40: seconds
dt.setMilliseconds(333); // => 333: milliseconds
console.log(dt); // => December 25, 2014 15:30:40 333 milliseconds
4.3 Other methods
4.3.1 toString(): Convert Date into a 'year, month, day, hour, minute, and second' string
4.3.2 toLocaleString(): Convert Date into a local format string of 'year, month, day, hour, minute and second'
4.3.3 toDateString(): Convert Date into a 'year, month, day' string
4.3.4 toLocaleDateString(): Convert Date into a local format string of 'year, month and day'
4.3.5 toTimeString(): Convert Date into a string of 'hours, minutes and seconds'
4.3.6 toLocaleTimeString(): Convert Date into a local format string of 'hours, minutes and seconds'
4.3.7 valueOf(): Same as getTime(), returns the millisecond value between the Date object and '1970/01/01 00:00:00' (the time zone of Beijing time is East 8th District, the starting time is actually is: '1970/01/01 08:00:00')
Example:
var dt = new Date();
console.log(dt.toString()); // => Tue Dec 23 2014 22:56:11 GMT 0800 (China Standard Time): Convert Date into a 'year, month, day, hour, minute, and second' string
console.log(dt.toLocaleString()); // => December 23, 2014 10:56:11 pm: Convert Date into a local format string of 'year, month, day, hour, minute, and second'
console.log(dt.toDateString()); // => Tue Dec 23 2014: Convert Date to a 'year, month and day' string
console.log(dt.toLocaleDateString()); // => December 23, 2014: Convert Date into a local format string of 'year, month and day'
console.log(dt.toTimeString()); // => 22:56:11 GMT 0800 (China Standard Time): Convert Date into an 'hours, minutes and seconds' string
console.log(dt.toLocaleTimeString()); // => 10:56:11 PM: Convert Date to a local format string of 'hours, minutes and seconds'
console.log(dt.valueOf()); // => Returns the millisecond value between the Date object and '1970/01/01 00:00:00' (the time zone of Beijing time is East 8th District, the starting time is actually is: '1970/01/01 08:00:00')
5. Static method
5.1 Date.now()
Description: Returns the millisecond value between the Date object of the current date and time and '1970/01/01 00:00:00' (the time zone of Beijing time is East 8th District, the starting time is actually: '1970/01/01 08 :00:00')
Parameters: None
Return value:
{int}: The number of milliseconds between the current time and the starting time.
Example:
console.log(Date.now()); // => 1419431519276
5.2 Date.parse(dateStr)
Description: Convert the string to a Date object, and then return the millisecond value between this Date object and '1970/01/01 00:00:00' (the time zone of Beijing time is East 8th District, the starting time is actually: '1970 /01/01 08:00:00')
Parameters:
①dateStr {string}: A string that can be converted into a Date object (time can be omitted); there are two main formats of strings:
1) yyyy/MM/dd HH:mm:ss (recommended): If the time is omitted, the time of the returned Date object is 00:00:00.
2) yyyy-MM-dd HH:mm:ss: If the time is omitted, the time of the returned Date object is 08:00:00 (plus the local time zone). If the time is not omitted, this string returns NaN (not a number) in IE!
Return value:
{int} Returns the number of milliseconds between the converted Date object and the starting time.
Example:
console.log(Date.parse('2014/12/25 12:00:00')); // => 1419480000000
console.log(Date.parse('2014-12-25 12:00:00')); // => 1419480000000 (Note: This conversion method returns NaN in IE!)
6. Practical operation
6.1 Convert C#’s DateTime type to Js’s Date object
Note: The format of C#'s DateTime type returned to the front desk through Json serialization is: "/Date(1419492640000)/". The number in the middle represents the number of milliseconds between the DateTime value and the starting time.
Example:
Backend code: simple ashx
public void ProcessRequest (HttpContext context) {
System.Web.Script.Serialization.JavaScriptSerializer js = new System.Web.Script.Serialization.JavaScriptSerializer();
DateTime dt = DateTime.Parse("2014-12-25 15:30:40");
String rs = js.Serialize(dt); // Serialize into Json
Context.Response.ContentType = "text/plain";
context.Response.Write(rs);
}
Front-end code:
var dateTimeJsonStr = '/Date(1419492640000)/'; // Json format for C# DateTime type conversion
var msecStr = dateTimeJsonStr.toString().replace(//Date(([-]?d ))//gi, "$1"); // => '1419492640000': Get the millisecond string var msesInt = Number.parseInt(msecStr); // Convert millisecond string to numerical value
var dt = new Date(msesInt); //Initialize Date object
console.log(dt.toLocaleString()); // => December 25, 2014 3:30:40 pm
6.2 Get Countdown
Description: Calculate how many days, hours and minutes the current time is from the destination time.
Example:
/**
* Return to countdown
* @param dt {Date}: destination Date object
* @return {Strin}: Return countdown: X days X hours X minutes
*/
function getDownTime(dt) {
// 1. Get countdown
var intervalMsec = dt - Date.now(); // Subtract the current time from the destination time and get the number of milliseconds difference between the two
var intervalSec = intervalMsec / 1000; // Convert to seconds
var day = parseInt(intervalSec / 3600 / 24); // Number of days
var hour = parseInt((intervalSec - day * 24 * 3600) / 3600); // Hour
var min = parseInt((intervalSec - day * 24 * 3600 - hour * 3600) / 60); // minutes
// 2. If the difference in milliseconds is less than 0, it means that the destination time is less than the current time. The values taken at this time are all negative: -X days - hours - minutes. When displaying, only the negative days in front of it are displayed.
If (intervalMsec hour = 0 - hour;
min = 0 - min;
}
// 3. Concatenate strings and return
var rs = day 'day' hour 'hour' min 'minute';
Return rs;
}
// Current time: 2014/12/28 13:26
console.log(getDownTime(new Date('2015/06/01'))); // => 154 days 10:33
console.log(getDownTime(new Date('2014/01/01'))); // => -361 days 13:26
6.3 Compare the sizes of two Date objects
Note: You can compare the two with the number of milliseconds of the starting time to distinguish the size.
Example:
var dt1 = new Date('2015/12/01');
var dt2 = new Date('2015/12/25');
console.log(dt1 > dt2); // => false
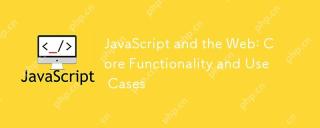
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
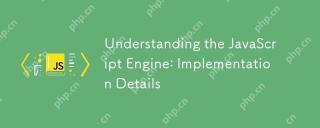
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
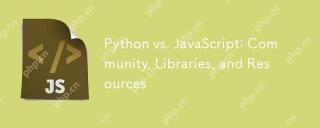
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
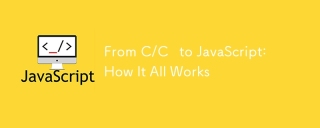
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
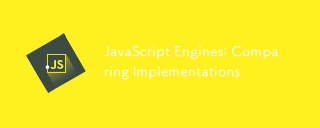
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
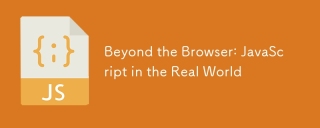
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
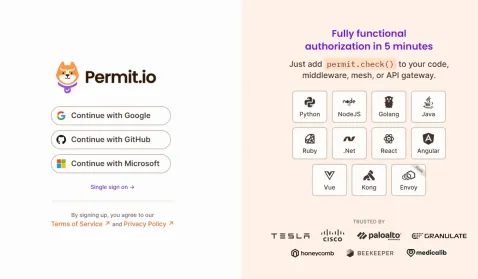
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
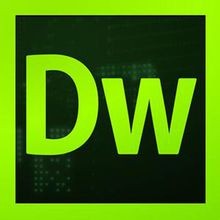
Dreamweaver CS6
Visual web development tools
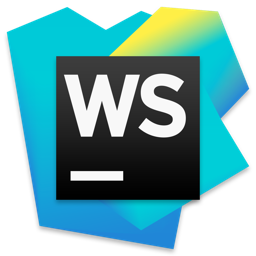
WebStorm Mac version
Useful JavaScript development tools
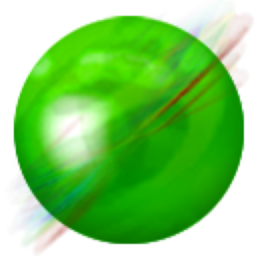
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor