1. Return the Jquery object instance through the method
Use var someDiv = $(‘#someDiv’).hide(); instead of var someDiv = $(‘#someDiv’); someDiv.hide();
2. Use find to find
Use $('#someDiv').find('p.someClass').hide(); instead of $('#someDiv p.someClass').hide(); because you don’t have to trigger Jquery’s Sizzle engine. At the same time, when writing selectors, try to keep your selectors simple and optimize the rightmost selector
3. Don’t abuse $(this)
Use $('#someAnchor').click(function() { alert( this.id ); }); instead of $('#someAnchor').click(function() {alert($(this). attr('id'));});
4. The abbreviation of ready
Use $(function() { }); instead of $(document).ready(function() {});
5. Make your code safe
Method 1 (using noConflict)
var j = jQuery.noConflict();
j(‘#someDiv’).hide();
// The line below will reference some other library's $ function.
$(‘someDiv’).style.display = ‘none’;
Method 2 (pass in parameter Jquery)
(function($) {
// Within this function, $ will always refer to jQuery
})(jQuery);
Method 3 (via ready method)
jQuery(document).ready(function($) {
// $ refers to jQuery
});
6. Simplify the code
Use each instead of for, use arrays to save temporary variables, and use document fragments. This is actually the same thing you need to pay attention to when writing native Javascript.
7. How to use Ajax
Jquery provides useful ajax methods such as get getJSON post ajax
8. Access native properties and methods
For example, the method of obtaining element id is
// OPTION 1 – Use jQuery
var id = $(‘#someAnchor’).attr(‘id’);
// OPTION 2 – Access the DOM element
var id = $(‘#someAnchor’)[0].id;
// OPTION 3 – Use jQuery’s get method
var id = $(‘#someAnchor’).get(0).id;
// OPTION 3b – Don't pass an index to get
anchorsArray = $(‘.someAnchors’).get();
var thirdId = anchorsArray[2].id;
9. Use PHP to check Ajax requests
By using xhr.setRequestHeader(“X-Requested-With”, “XMLHttpRequest”); The server side such as PHP can pass
function isXhr() {
return $_SERVER['HTTP_X_REQUESTED_WITH'] === 'XMLHttpRequest';
}
To check if it is an Ajax request, it may be used in some situations where Javascript is disabled
10.The relationship between Jquery and $
At the bottom of the Jquery code, you can see the following code
window.jQuery = window.$ = jQuery; $ is actually a shortcut of Jquery
11. Conditional loading Jquery
<script>!window.jQuery && document.write(‘<script src=”js/jquery-1.4.2.min.js”></script>')
If CDN does not download to Jquery, read from local
12.Jquery Filters
<script><br /> $('p:first').data('info', 'value'); // populates $'s data object to have something to work with<br /> $.extend(<br /> jQuery.expr[":"], {<br /> block: function(elem) {<br /> return $(elem).css(“display”) === “block”;<br /> },<br /> hasData : function(elem) {<br /> return !$.isEmptyObject( $(elem).data() );<br /> }<br /> }<br /> );<br /> $(“p:hasData”).text(“has data”); // grabs paras that have data attached<br /> $(“p:block”).text(“are block level”); // grabs only paragraphs that have a display of “block”<br /> </script>
Note: $.expr[":"] is equivalent to $.expr.filters
13.hover method
$(‘#someElement’).hover(function() {
//You can use the toggle method here to achieve the effect of sliding over and sliding out
});
14. Pass in the attribute object
When creating an element, Jquery1.4 can pass in an attribute object
$('', {
id : ‘someId’,
className : ‘someClass’,
href : ‘somePath.html’
});
Even properties or events specified by Jquery such as text, click
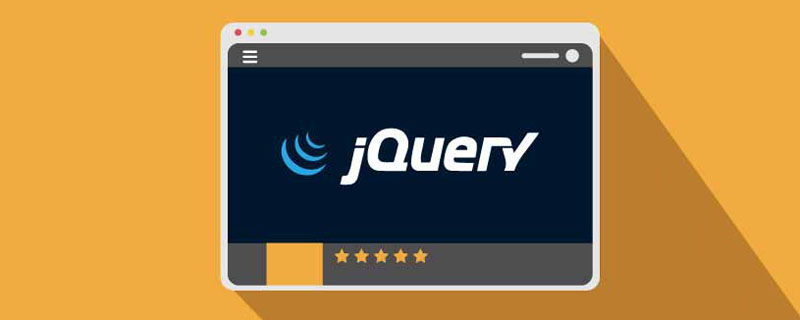
实现方法:1、用“$("img").delay(毫秒数).fadeOut()”语句,delay()设置延迟秒数;2、用“setTimeout(function(){ $("img").hide(); },毫秒值);”语句,通过定时器来延迟。
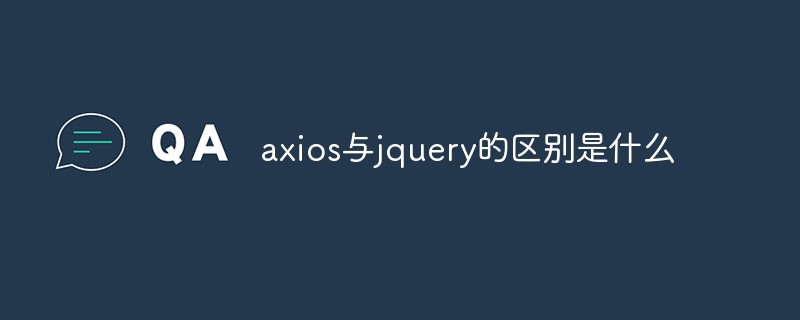
区别:1、axios是一个异步请求框架,用于封装底层的XMLHttpRequest,而jquery是一个JavaScript库,只是顺便封装了dom操作;2、axios是基于承诺对象的,可以用承诺对象中的方法,而jquery不基于承诺对象。
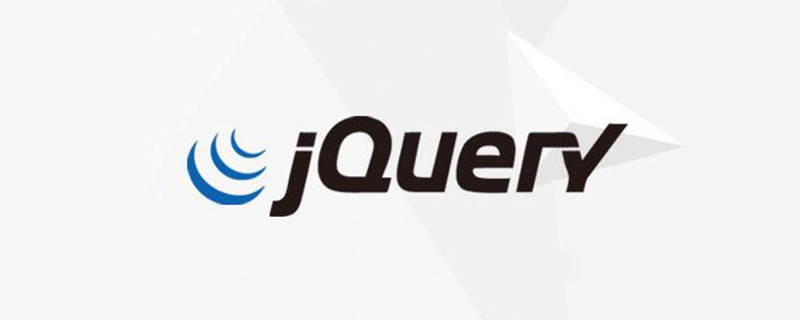
修改方法:1、用css()设置新样式,语法“$(元素).css("min-height","新值")”;2、用attr(),通过设置style属性来添加新样式,语法“$(元素).attr("style","min-height:新值")”。
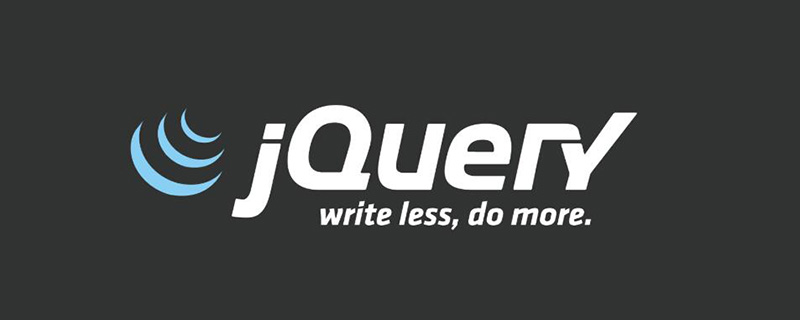
增加元素的方法:1、用append(),语法“$("body").append(新元素)”,可向body内部的末尾处增加元素;2、用prepend(),语法“$("body").prepend(新元素)”,可向body内部的开始处增加元素。
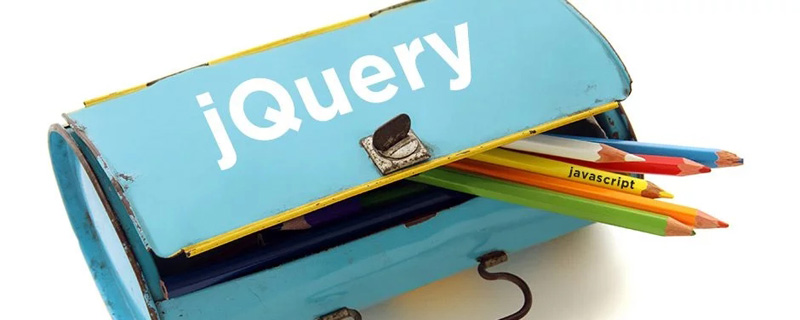
删除方法:1、用empty(),语法“$("div").empty();”,可删除所有子节点和内容;2、用children()和remove(),语法“$("div").children().remove();”,只删除子元素,不删除内容。
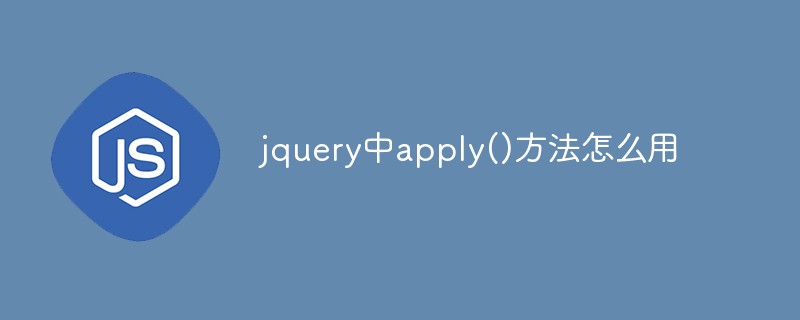
在jquery中,apply()方法用于改变this指向,使用另一个对象替换当前对象,是应用某一对象的一个方法,语法为“apply(thisobj,[argarray])”;参数argarray表示的是以数组的形式进行传递。
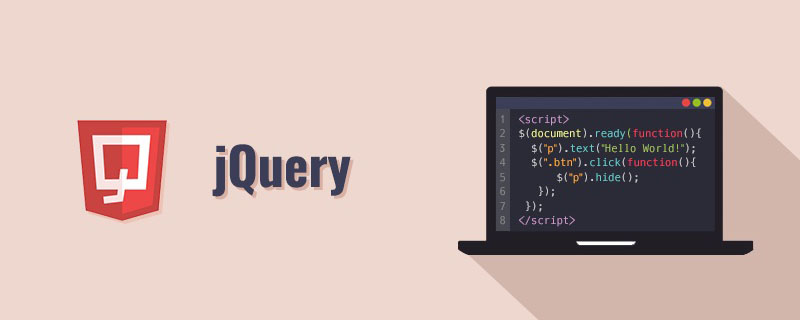
去掉方法:1、用“$(selector).removeAttr("readonly")”语句删除readonly属性;2、用“$(selector).attr("readonly",false)”将readonly属性的值设置为false。
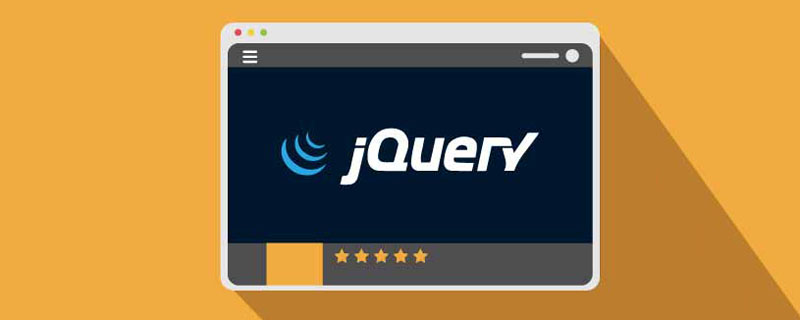
on()方法有4个参数:1、第一个参数不可省略,规定要从被选元素添加的一个或多个事件或命名空间;2、第二个参数可省略,规定元素的事件处理程序;3、第三个参数可省略,规定传递到函数的额外数据;4、第四个参数可省略,规定当事件发生时运行的函数。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
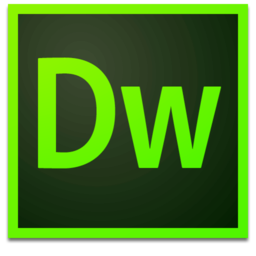
Dreamweaver Mac version
Visual web development tools
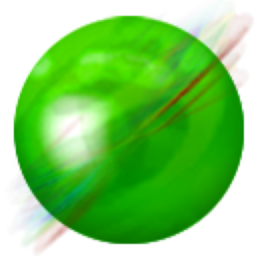
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
