It is not uncommon for articles to discuss jQuery and javascript performance. However, I plan to teach you how to improve your JSLite and JavaScript code based on some speed tips and suggestions that others have put together for jQuery. Good code will bring speed improvements. Fast rendering and responsiveness means a better user experience.
First of all, keep in mind that JSLite is javascript. This means we should adopt the same coding conventions, style guides and best practices.
First of all, if you are a javascript newbie and have never used jQuery, I suggest you read the syntax introduction of the official document first. This is a high-quality javascript tutorial, which means that you have been using jQuery for a while. .
When you are ready to use JSLite, I strongly recommend that you follow these guidelines:
Cache variables
DOM traversal is expensive, so try to cache reused elements.
// Oops
h = $('#element').height();
$('#element').css('height',h-20);
// Suggestion
$element = $('#element');
h = $element.height();
$element.css('height',h-20);
Avoid global variables
JSLite is the same as JavaScript. Generally speaking, it is best to ensure that your variables are within function scope.
// Oops
$element = $('#element');
h = $element.height();
$element.css('height',h-20);
// Suggestion
var $element = $('#element');
var h = $element.height();
$element.css('height',h-20);
Use Hungarian nomenclature
Add $ prefix before variables to easily identify JSLite objects.
// Oops
var first = $('#first');
var second = $('#second');
var value = $first.val();
// Suggestion - Prefix JSLite objects with $
var $first = $('#first');
var $second = $('#second'),
var value = $first.val();
Use var chain (single var mode)
Consolidate multiple var statements into one statement. I recommend putting unassigned variables at the end.
var $first = $('#first'),
$second = $('#second'),
Value = $first.val(),
k = 3,
Cookiestring = 'SOMECOOKIESPLEASE',
i,
j,
MyArray = {};
Please use on
In the new version of JSLite, the shorter on("click") is used to replace functions like click(). In previous versions on() was bind() . on() is the preferred method of attaching event handlers. However, for consistency's sake, you can simply use the on() method all together.
// Oops
$first.click(function(){
$first.css('border','1px solid red');
$first.css('color','blue');
});
$first.hover(function(){
$first.css('border','1px solid red');
})
// Suggestion
$first.on('click',function(){
$first.css('border','1px solid red');
$first.css('color','blue');
})
$first.on('hover',function(){
$first.css('border','1px solid red');
})
Streamlined javascript
In general, it’s best to combine functions whenever possible.
// Oops
$first.click(function(){
$first.css('border','1px solid red');
$first.css('color','blue');
});
// Suggestion
$first.on('click',function(){
$first.css({
'border':'1px solid red',
'color':'blue'
});
});
Chain operation
It is very easy to implement chained operations of methods in JSLite. Take advantage of this below.
// Oops
$second.html(value);
$second.on('click',function(){
alert('hello everybody');
});
$second.fadeIn('slow');
$second.animate({height:'120px'},500);
// Suggestion
$second.html(value);
$second.on('click',function(){
alert('hello everybody');
}).fadeIn('slow').animate({height:'120px'},500);
Maintain code readability
Along with streamlining the code and using chaining, the code may become difficult to read. Adding pinches and line breaks can work wonders.
// Oops
$second.html(value);
$second.on('click',function(){
alert('hello everybody');
}).fadeIn('slow').animate({height:'120px'},500);
// Suggestion
$second.html(value);
$second
.on('click',function(){ alert('hello everybody');})
.fadeIn('slow')
.animate({height:'120px'},500);
Select short-circuit evaluation
Short-circuit evaluation is an expression that is evaluated from left to right, using the && (logical AND) or || (logical OR) operators.
// Oops
function initVar($myVar) {
If(!$myVar) {
$myVar = $('#selector');
}
}
// Suggestion
function initVar($myVar) {
$myVar = $myVar || $('#selector');
}
Select shortcut
One way to streamline your code is to take advantage of coding shortcuts.
// Oops
if(collection.length > 0){..}
// Suggestion
if(collection.length){..}
Separating elements during heavy operations
If you plan to do a lot of operations on DOM elements (setting multiple attributes or css styles in succession), it is recommended to separate the elements first and then add them.
// Oops
var
$container = $("#container"),
$containerLi = $("#container li"),
$element = null;
$element = $containerLi.first();
//... Many complex operations
// better
var
$container = $("#container"),
$containerLi = $container.find("li"),
$element = null;
$element = $containerLi.first().detach();
//... Many complex operations
$container.append($element);
Memory skills
You may be inexperienced with using methods in JSLite, be sure to check out the documentation, there may be a better or faster way to use it.
// Oops
$('#id').data(key,value);
// Suggestion (efficient)
$.data('#id',key,value);
Parent element cached using subquery
As mentioned earlier, DOM traversal is an expensive operation. A typical approach is to cache parent elements and reuse these cached elements when selecting child elements.
// Oops
var
$container = $('#container'),
$containerLi = $('#container li'),
$containerLiSpan = $('#container li span');
// Suggestion (efficient)
var
$container = $('#container '),
$containerLi = $container.find('li'),
$containerLiSpan= $containerLi.find('span');
Avoid universal selectors
Putting the universal selector into a descendant selector has terrible performance.
// Oops
$('.container > *');
// Suggestion
$('.container').children();
Avoid implicit universal selectors
Universal selectors are sometimes implicit and difficult to find.
// Oops
$('.someclass :radio');
// Suggestion
$('.someclass input:radio');
Optimization selector
For example, the Id selector should be unique, so there is no need to add additional selectors.
// Oops
$('div#myid');
$('div#footer a.myLink');
// Suggestion
$('#myid');
$('#footer .myLink');
Avoid multiple ID selectors
I emphasize here that the ID selector should be unique, there is no need to add additional selectors, and there is no need for multiple descendant ID selectors.
// Oops
$('#outer #inner');
// Suggestion
$('#inner');
Stick to the latest version
New versions are usually better: more lightweight, more efficient, with more methods, and more comprehensive coverage of jQuery methods. Obviously, you need to consider the compatibility of the code you want to support. For example, does the project run on good support for HTML5/CSS3
Combine JSLite and javascript native code when necessary
As mentioned above, JSLite is javascript, which means that anything you can do with JSLite can also be done with native code. Native code may not be as readable and maintainable as JSLite, and the code may be longer. But it also means more efficient (usually the closer to the underlying code the less readable the higher the performance, for example: assembly, which of course requires more powerful people). Keep in mind that no framework can be smaller, lighter, and more efficient than native code (note: the test link is no longer valid, you can search for the test code online).
Final advice
Finally, I record this article with the purpose of improving the performance of JSLite and some other good suggestions. If you want to delve deeper into this topic you will find a lot of fun. Remember, JSLite is not required, just an option. Think about why you want to use it. DOM manipulation? ajax? stencil? css animation? Or a selector? Heavy jQuery developer?
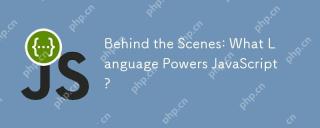
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
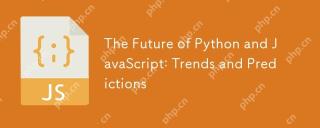
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
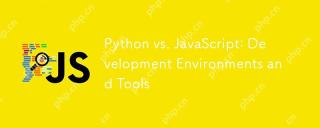
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
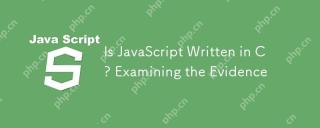
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
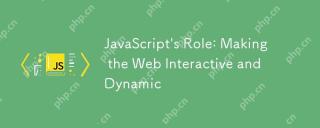
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
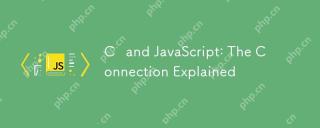
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
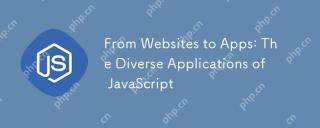
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
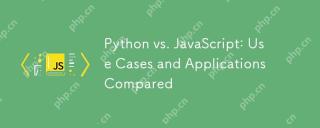
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
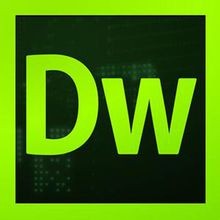
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
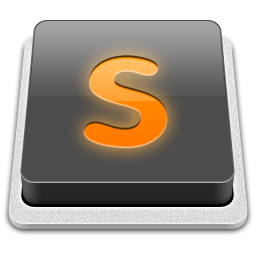
SublimeText3 Mac version
God-level code editing software (SublimeText3)
