D3 All APIs provided by the library are under the d3 namespace. The d3 library uses semantic versioning. You can use d3.version
to view the current version information.
d3 (core part)
Selection Set
- d3.select - Selects a range of elements from the current document.
- d3.selectAll - Select multiple elements from the current document.
- selection.attr - Sets or gets the specified attribute.
- selection.classed - Add or remove the CSS class of the selected element.
- selection.style - Set or remove CSS properties. style has higher priority than attr.
- selection.property - Set or get the raw property value.
- selection.text - Sets or gets the tag body text content of the selected element.
- selection.html - Set or get the HTML content of the selected element (similar to innerHTML )
- selection.append - Creates and adds a new element to the selected element.
- selection.insert - Creates and adds a new element before the selected element.
- selection.remove - Removes selected elements from the current document object.
- selection.data - get or set data for a group of elements, while computing a relational join.
- selection.enter - Returns a placeholder object for missing elements, pointing to a portion of the bound data that is more than the selected element set.
- selection.exit - Returns the element set of excess elements, that is, the part of the selected elements that is more than the bound data. (Example 1, Example 2, Example 3 about the principle of data, enter, exit)
- selection.datum - Sets or gets the data of a single element, without association. (get or set data for individual elements, without computing a join.)
- selection.filter - Filters the selection set based on bound data.
- selection.sort - Sorts selected elements based on bound data.
- selection.order - Reorders elements in the document to match the selection set.
- selection.on - Add or remove event listeners.
- selection.transition - Starts a transition effect (returns a Transition object), which can be understood as an animation.
- selection.interrupt - Immediately stops all ongoing animations.
- selection.each - Calls the specified function for each selected set of elements.
- selection.call - Calls the specified function for the currently selected set of elements.
- selection.empty - Tests whether the selection set is empty.
- selection.node - Returns the first element in the selection.
- selection.size - Returns the number of elements in the selection set.
- selection.select - Selects the first child element of the selected elements to form a new selection set.
- selection.selectAll - Select multiple sub-elements of the selected element to form a new selection set.
-
d3.selection - Selection set object prototype (selection sets can be enhanced with
d3.selection.prototype
). - d3.event - Get the user event of the current interaction.
- d3.mouse - Get the coordinates of the mouse relative to an element.
- d3.touches - Get the touch point coordinates relative to an element.
Transition effect
- d3.transition - Starts an animated transition. Simple Tutorial
- transition.delay - Specifies the delay time for each element transition (unit: ms).
- transition.duration - Specifies the duration of each element's transition in milliseconds.
- transition.ease - Specifies the transition buffer function.
- transition.attr - Smooth transition to new attr attribute value (the starting attribute value is the current attribute).
- transition.attrTween - Smooth transition between different attr attribute values (the starting attribute value can be set in the transition function, and even the entire transition function can be customized).
- transition.style - Smooth transition to new style attribute value.
- transition.styleTween - Smooth transition between different style attribute values.
- transition.text - Sets the text content at the beginning of the transition.
- transition.tween - transitions an attribute to a new attribute value. The attribute can be a non-attr or non-style attribute, such as text.
- transition.select - Selects a child element of each current element for transition.
- transition.selectAll - Select multiple child elements of each current element for transition.
- transition.filter - Filter out some elements in the current element through data for transition.
- transition.transition - Starts a new transition after the current transition ends.
- transition.remove - Removes the current element after the transition.
- transition.empty - Returns true if the transition is empty. If there are no non-null elements in the current element, this transition is empty.
- transition.node - Returns the first element in the transition.
- transition.size - Returns the current number of elements in the transition.
- transition.each - Iterates over each element and performs the operation. When the trigger type is not specified, the operation is performed immediately. When the specified trigger type is 'start' or 'end', the operation will be performed at the beginning or end of the transition.
- transition.call - Execute a function with the current transition as this.
- d3.ease - Custom transition buffer function.
- ease - buffer function. Buffer functions can make animation effects more natural. For example, elastic buffer functions can be used to simulate the movement of elastic objects. is a special case of interpolation function.
- d3.timer - Starts a custom animation timing. The function is similar to setTimeout, but it is implemented internally using requestAnimationFrame, which is more efficient.
- d3.timer.flush - Immediately execute the current timer without delay. Can be used to deal with splash screen issues.
- d3.interpolate - Generates an interpolation function that interpolates between two parameters. The type of the difference function is automatically selected based on the type of the input argument (number, string, color, etc.).
- interpolate - interpolation function. The input parameters are between [0, 1].
- d3.interpolateNumber - Interpolates between two numbers.
- d3.interpolateRound - Interpolates between two numbers, the return value will be rounded.
- d3.interpolateString - Interpolate between two strings. Parse the numbers in the string and the corresponding numbers will be interpolated.
- d3.interpolateRgb - Interpolate between two RGB colors.
- d3.interpolateHsl - Interpolate between two HSL colors.
- d3.interpolateLab - Interpolate between two L*a*b* colors.
- d3.interpolateHcl - Interpolate between two HCL colors.
- d3.interpolateArray - Interpolates between two arrays. d3.interpolateArray( [0, 1], [1, 10, 100] )(0.5); // returns [0.5, 5.5, 100]
- d3.interpolateObject - Interpolate between two objects. d3.interpolateArray( {x: 0, y: 1}, {x: 1, y: 10, z: 100} )(0.5); // returns {x: 0.5, y: 5.5, z: 100}
- d3.interpolateTransform - Interpolate between two 2D affine transformations.
- d3.interpolateZoom - Smoothly scale the pan between two points.Example
- d3.interpolators - Add a custom interpolation function.
Data Operation (Working with Arrays)
- d3.ascending - Ascending sort function.
- d3.descending - Descending sort function.
- d3.min - Get the minimum value in the array.
- d3.max - Get the maximum value in the array.
- d3.extent - Get the range of the array (minimum and maximum values).
- d3.sum - Get the sum of the numbers in the array.
- d3.mean - Gets the arithmetic mean of the numbers in an array.
- d3.median - Gets the median of the numbers in the array (equivalent to a 0.5-quantile value).
- d3.quantile - Get a quantile of a sorted array.
- d3.bisect - Get the insertion position of a number in the sorted array through bisection (same as d3.bisectRight).
- d3.bisectRight - Gets the insertion position of a number in the sorted array (equal values go to the right).
- d3.bisectLeft - Gets the insertion position of a number in the sorted array (equal values go to the left).
- d3.bisector - Customize a bisection function.
- d3.shuffle - Shuffle, randomly arrange the elements in the array.
- d3.permute - Arrange the elements in the array in the specified order.
- d3.zip - An array that combines multiple arrays into one array. The i-th element of the new array is an array composed of the i-th elements in the original arrays.
- d3.transpose - Matrix transposition, implemented through d3.zip.
- d3.pairs - Returns an array of pairs of adjacent elements, d3.pairs([1, 2, 3, 4]); // returns [ [1, 2], [2, 3] , [3, 4] ].
- d3.keys - Returns an array composed of keys of an associative array (hash table, json, object object).
- d3.values - Returns an array composed of the values of the associative array.
- d3.entries - Returns an array composed of key-value entities of the associative array, d3.entries({ foo: 42 }); // returns [{key: "foo", value: 42 }].
- d3.merge - Concatenate multiple arrays into one, similar to the native method concat. d3.merge([ [1], [2, 3] ]); // returns [1, 2, 3].
- d3.range - Get a sequence. d3.range([start, ]stop[, step])
- d3.nest - Gets a nest object that organizes arrays into hierarchical structures. Example: http://bl.ocks.org/phoebebright/raw/3176159/
- nest.key - Adds a level to the nest hierarchy.
- nest.sortKeys - Sort the current nest hierarchy by key.
- nest.sortValues - Sort leaf nest levels by value.
- nest.rollup - Set the function to modify the leaf node value.
- nest.map - performs nest operation and returns an associative array (json).
- nest.entries - performs nest operations and returns a key-value array. If nest.map returns a result similar to { foo: 42 }, then nest.entries returns a result similar to [{ key: "foo", value: 42}].
- d3.map - Converts JavaScript object into hash, shielding the problem of inconsistency with hash caused by the prototype chain function of object.
- map.has - map returns true if it has a certain key.
- map.get - Returns the value corresponding to a key in the map.
- map.set - Set the value corresponding to a key in the map.
- map.remove - delete a key in the map.
- map.keys - Returns an array of all keys in the map.
- map.values - Returns an array composed of all values in map.
- map.entries - Returns an array composed of all entries (key-value pairs) in the map. Similar to { foo: 42 } converted into [{key: "foo", value: 42 }]
- map.forEach - Execute a function for each entry in the map.
- d3.set - Converts JavaScript array into set, shielding the problem of inconsistency with set caused by the object prototype chain function of array. The value in set is the result of converting each value in array into a string. The values in the set are deduplicated.
- set.has - Returns whether the set contains a certain value.
- set.add - Add a value.
- set.remove - delete a value.
- set.values - Returns an array composed of the values in the set. The values in the set are deduplicated.
- set.forEach - Execute a function for each value in the set.
Math
- d3.random.normal - Generate a random number using the normal distribution.
- d3.random.logNormal - Generate a random number using the lognormal distribution.
- d3.random.irwinHall - Generate a random number using the Irwin–Hall distribution (a simple, feasible and easy-to-program normal distribution implementation method).
- d3.transform - Convert the svg transform format into the standard 2D transformation matrix string format.
Loading External Resources
- d3.xhr - Initiates an XMLHttpRequest request to obtain resources.
- xhr.header - Set request header.
- xhr.mimeType - Set Accept request header and override response MIME type.
- xhr.response - Set the response return value conversion function. Such as function(request) { return JSON.parse(request.responseText); }
- xhr.get - Initiates a GET request.
- xhr.post - Initiates a POST request.
- xhr.send - Initiates a request with the specified method and data.
- xhr.abort - Abort the current request.
- xhr.on - Add event listeners for requests such as "beforesend", "progress", "load" or "error".
- d3.text - Requests a text file.
- d3.json - Requests a JSON.
- d3.html - Requests an html text fragment.
- d3.xml - Requests an XML text fragment.
- d3.csv - Requests a CSV (comma-separated values) file.
- d3.tsv - Requests a TSV (tab-separated values, tab-separated values) file.
String Formatting
- d3.format - Convert numbers into strings in the specified format. The converted formats are very rich and very intelligent.
- d3.formatPrefix - Gets a [SI prefix] object with the specified value and precision. This function can be used to automatically determine the magnitude of data, such as K (thousands), M (millions), etc. Example: var prefix = d3.formatPrefix(1.21e9); console.log(prefix.symbol); // “G”; console.log(prefix.scale(1.21e9)); // 1.21
- d3.requote - Escapes a string into a format that can be used in regular expressions. Such as d3.requote(‘$’); // return “$”
- d3.round - Set the number of decimal places to round a certain number. Similar to toFixed(), but the return format is number. Such as d3.round(1.23); // return 1; d3.round(1.23, 1); // return 1.2; d3.round(1.25, 1); // return 1.3
CSV format (d3.csv)
- d3.csv - Get a CSV (comma-separated values, colon separated values) file.
- d3.csv.parse - Convert the CSV file string into an array of objects. The key of the object is determined by the first line. For example: [{"Year": "1997", "Length": "2.34"}, {"Year": "2000", "Length": "2.38"}]
- d3.csv.parseRows - Converts a CSV file string into an array of arrays. For example: [ ["Year", "Length"],["1997", "2.34"],["2000", "2.38"] ]
- d3.csv.format - Converts an array of objects into a CSV file string, which is the reverse operation of d3.csv.parse.
- d3.csv.formatRows - Converts an array of arrays into a CSV file string, which is the reverse operation of d3.csv.parseRows.
- d3.tsv - Get a TSV (tab-separated values, tab-separated values) file.
- d3.tsv.parse - Similar to d3.csv.parse.
- d3.tsv.parseRows - Similar to d3.csv.parseRows.
- d3.tsv.format - Similar to d3.csv.format.
- d3.tsv.formatRows - Similar to d3.csv.formatRows.
- d3.dsv - Create a file processing object similar to d3.csv, with customizable delimiters and mime types. For example: var dsv = d3.dsv(“|”, “text/plain”);
Color
- d3.rgb - Specify a color and create an RGB color object. Supports input in multiple color formats.
- rgb.brighter - Enhance the brightness of the color, the range of change is determined by the parameters.
- rgb.darker - Reduces the brightness of the color, the range of change is determined by the parameters.
- rgb.hsl - Convert RGB color objects into HSL color objects.
- rgb.toString - Convert RGB color to string format.
- d3.hsl - Creates an HSL color object. Supports input in multiple color formats.
- hsl.brighter - Enhance the brightness of the color, the change range is determined by the parameters.
- hsl.darker - Reduces the brightness of the color, the range of change is determined by the parameters.
- hsl.rgb - Convert HSL color object to RGB color object.
- hsl.toString - Convert HSL color to string format.
- d3.lab - Creates a Lab color object. Supports input in multiple color formats.
- lab.brighter - Enhance the brightness of the color, the change range is determined by the parameters.
- lab.darker - Reduces the brightness of the color, the range of change is determined by the parameters.
- lab.rgb - Convert Lab color object to RGB color object.
- lab.toString - Lab color converted to string format.
- d3.hcl - Creates an HCL color object. Supports input in multiple color formats.
- hcl.brighter - Enhance the brightness of the color, the range of change is determined by the parameters.
- hcl.darker - Reduces the brightness of the color, the range of change is determined by the parameters.
- hcl.rgb - Convert HCL color object to RGB color object.
- hcl.toString - Convert HCL color to string format.
Namespace
- d3.ns.prefix - Get or extend a known XML namespace.
- d3.ns.qualify - Verify whether the namespace prefix exists, such as "xlink:href" in which xlink is a known namespace.
Internals
- d3.functor - Functionalization. Convert a non-function variable into a function that returns only the value of the variable. If you enter a function, the original function is returned; if you enter a value, a function is returned, which only returns the original value.
- d3.rebind - Bind the methods of one object to another object.
- d3.dispatch - Create a custom event.
- dispatch.on - Add or remove an event listener. Multiple listeners can be added to an event.
- dispatch.type - trigger event. Where 'type' is the name of the event to be triggered.
d3.scale(Scales)
Quantitative
- d3.scale.linear - Creates a linear quantitative transformation. (It is recommended to refer to the source code for a deeper understanding of various transformations.)
- linear - takes a domain value and returns a domain value.
- linear.invert - Inverse transformation, input domain value returns domain value.
- linear.domain - get or set domain.
- linear.range - get or set value range.
- linear.rangeRound - Sets the range and rounds the result.
- linear.interpolate - The interpolation function of get or set transformation, such as replacing the default linear interpolation function with the rounded linear interpolation function d3_interpolateRound.
- linear.clamp - Sets whether the value range is closed. The default is not closed. When the range is closed, if the interpolation result is outside the range, the boundary value of the range will be taken. For example, if the value range is [1, 2], the calculation result of the interpolation function is 3. If it is not closed, the final result is 3; if it is closed, the final result is 2.
- linear.nice - Expand the scope of the domain to make the domain more regular. For example, [0.20147987687960267, 0.996679553296417] becomes [0.2, 1].
- linear.ticks - Take representative values from the domain. Usually used to select coordinate axis scales.
- linear.tickFormat - Gets the format conversion function, usually used for format conversion of coordinate axis scales. For example: var x = d3.scale.linear().domain([-1, 1]); console.log(x.ticks(5).map(x.tickFormat(5, “%”))); / / ["-100%", "-50%", " 0%", " 50%", " 100%"]
- linear.copy - Copies a transformation from an existing transformation.
- d3.scale.sqrt - Creates a quantitative transformation that takes square roots.
- d3.scale.pow - Creates an exponential transformation. (Please refer to the comments of the linear corresponding function)
- pow - Inputs a domain value and returns a domain value.
- pow.invert - Inverse transformation, the input domain value returns the domain value.
- pow.domain - get or set domain.
- pow.range - get or set value range.
- pow.rangeRound - Sets the range and rounds the result.
- pow.interpolate - Interpolation function for get or set transformation.
- pow.clamp - Sets whether the value range is closed, not closed by default.
- pow.nice - Expand the scope of the domain to make the domain more regular.
- pow.ticks - Take representative values from the domain. Usually used to select coordinate axis scales.
- pow.tickFormat - Get the format conversion function, usually used for format conversion of coordinate axis scale.
- pow.exponent - get or set the power of the exponent. The default is a power of 1.
- pow.copy - Copies a transform from an existing transform.
- d3.scale.log - Creates a logarithmic transformation. (Please refer to the comments of the linear corresponding function)
- log - Inputs a domain value and returns a domain value.
- log.invert - Inverse transformation, the input domain value returns the domain value.
- log.domain - get or set domain.
- log.range - get or set value range.
- log.rangeRound - Sets the range and rounds the result.
- log.interpolate - Interpolation function for get or set transformation.
- log.clamp - Set whether the value range is closed or not. The default is not closed.
- log.nice - Expand the scope of the domain to make the domain more regular.
- log.ticks - Take representative values from the domain. Usually used to select coordinate axis scales.
- log.tickFormat - Get the format conversion function, usually used for format conversion of coordinate axis scale.
- log.copy - Copies a transformation from an existing transformation.
- d3.scale.quantize - Creates a quantize linear transformation with a definition domain of a numerical interval and a value range of several discrete values.
- quantize - Enter a numerical value and return a discrete value. For example: var q = d3.scale.quantize().domain([0, 1]).range(['a', 'b', 'c']); //q(0.3) === 'a ', q(0.4) === 'b', q(0.6) === 'b', q(0.7) ==='c;
- quantize.invertExtent - Returns the range of a discrete value. // The result of q.invertExtent(‘a’) is [0, 0.3333333333333333]
- quantize.domain - The domain of the get or set transformation.
- quantize.range - get or set the value range of the transformation.
- quantize.copy - Copies a transform from an existing transform.
- d3.scale.threshold - Construct a threshold linear transformation. The domain is a numerical sequence of separated values, and the range is discrete values. The difference between it and quantize is that the value range specified by quantize is an interval, and then the interval is divided into multiple small intervals to correspond to each discrete value. threshold specifies the boundary separation value between each cell. Example: var t = d3.scale.threshold().domain([0, 1]).range(['a', 'b', 'c']); t(-1) === 'a' ; t(0) === 'b'; t(0.5) === 'b'; t(1) === 'c'; t(1000) === 'c'; t.invertExtent(' a'); //returns [undefined, 0] t.invertExtent('b'); //returns [0, 1] t.invertExtent('c'); //returns [1, undefined]
- threshold - Enter a numerical value and return a discrete value.
- threshold.invertExtent - Enter a discrete value and return a numerical value.
- threshold.domain - The domain of the get or set transformation.
- threshold.range - The value range of the get or set transformation.
- threshold.copy - Copies a transform from an existing transform.
- d3.scale.quantile - Constructs a quantile linear transformation. The usage method is completely similar to quantize. The difference is that quantile separates intervals based on the median, and quantize separates intervals based on the arithmetic mean. example
- quantile - Enter a numerical value and return a discrete value.
- quantile.invertExtent - Input a discrete value and return a numerical value.
- quantile.domain - The domain of the get or set transformation.
- quantile.range - get or set the value range of the transformation.
- quantile.quantiles - Get separated values of quantile transformation. Example: var q = d3.scale.quantile().domain([0, 1]).range(['a', 'b', 'c']); q.quantiles() returns [0.33333333333333326, 0.6666666666666665]
- quantile.copy - Copies a transform from an existing transform.
- d3.scale.identity - Constructs an identity linear transformation. A special linear linear transformation. The definition domain of this transformation is the same as the value domain. It is only used in some axis or brush modules inside d3.
- identity - identity linear transformation function. Returns the input value.
- identity.invert - Same as the identity function, returns the input value.
- identity.domain - The domain of the get or set transformation.
- identity.range - The value range of the get or set transformation.
- identity.ticks - Take representative values from the domain. Usually used to select coordinate axis scales.
- identity.tickFormat - Get the format conversion function, usually used for format conversion of coordinate axis scale.
- identity.copy - Copies a transform from an existing transform.
Ordinal transformation (Ordinal)
- d3.scale.ordinal - Constructs an ordinal transformation object. The input domain and output domain of the ordinal transformation are both discrete. The input domain of quantitative transformation is continuous, which is the biggest difference between the two.
- ordinal - Inputs a discrete value and returns a discrete value. Input values that are not in the current domain are automatically added to the domain.
- ordinal.domain - The domain of the get or set transformation.
- ordinal.range - get or set the value range of the transformation.
- ordinal.rangePoints - Use several discrete points to divide a continuous interval. See the legend in the link for details.
- ordinal.rangeBands - Split a continuous range with several discrete intervals. See the legend in the link for details.
- ordinal.rangeRoundBands - Use several discrete intervals to divide a continuous interval. The interval boundaries and widths will be rounded. See the legend in the link for details.
- ordinal.rangeBand - Gets the width of a discrete interval.
- ordinal.rangeExtent - Get the minimum and maximum value of the output domain.
- ordinal.copy - Copies a transform from an existing transform.
- d3.scale.category10 - Construct an ordinal transform with 10 colors.
- d3.scale.category20 - Construct an ordinal transform with 20 colors.
- d3.scale.category20b - Build an ordinal transform with another 20 colors.
- d3.scale.category20c - Construct an ordinal transform with another 20 colors.
d3.svg (SVG)
Shapes
- d3.svg.line - Creates a line segment generator.
- line - Generate a polyline in the line chart.
- line.x - Sets or gets the x axis accessor.
- line.y - sets or gets the y axis accessor
- line.interpolate - Sets or gets the interpolation mode.
- line.tension - Gets or sets the cardinal spline tension accessor.
- line.defined - defines whether a line exists at a certain point.
- d3.svg.line.radial - Creates a radial line generator.
- line - Generates piecewise linear curves for use in latitude line/radar line charts.
- line.radius - Gets or sets the radius accessor.
- line.angle - Gets or sets the angle accessor.
- line.defined - Sets or gets the line definition accessor.
- d3.svg.area - Creates a new area generator.
- area - generates a linear area for area charts.
- area.x - accessor to get or set the coordinates of x.
- area.x0 - accessor to get or set x0 coordinates (baseline).
- area.x1 - accessor to get or set x1 coordinates (backline).
- area.y - accessor to get or set y coordinates.
- area.y0 - accessor to get or set y0 coordinates (baseline).
- area.y1 - accessor to get or set y1 coordinates (backline).
- area.interpolate - Gets or sets the interpolation mode.
- area.tension - Gets or sets the tension accessor (the cardinal spline tension).
- area.defined - determines whether to get or define the area definition accessor.
- d3.svg.area.radial - Creates a new area generator.
- area - Generates piecewise linear areas for use in latitude/radar charts.
- area.radius - Gets or sets the radius accessor.
- area.innerRadius - Gets or sets the inner radius (baseline) accessor.
- area.outerRadius - Gets or sets the outer radius (backline) accessor.
- area.angle - Gets or sets the angle accessor.
- area.startAngle - Gets or sets the internal angle (baseline) accessor.
- area.endAngle - Gets or sets the external angle (backline) accessor.
- area.defined - determines whether to get or define the area definition accessor.
- d3.svg.arc - Creates an arc generator.
- arc - Generates a linear arc for use in pie or donut charts.
- arc.innerRadius - Gets or sets the inner radius accessor.
- arc.outerRadius - Gets or sets the outer radius accessor.
- arc.startAngle - Gets or sets the starting angle accessor.
- arc.endAngle - Gets or sets the end angle accessor.
- arc.centroid - Calculate the centroid point of the arc.
- d3.svg.symbol - Create a symbol generator.
- symbol - generates the specified symbol for use in hash maps.
- symbol.type - Gets or sets the symbol type accessor.
- symbol.size - Get or set the symbol size (in square pixels) accessor.
- d3.svg.symbolTypes - Array of supported symbol types.
- d3.svg.chord - Creates a new chord generator.
- chord - Generates a quadratic Bezier curve connecting two arcs, for use in chord diagrams.
- chord.radius - Gets or sets the arc radius accessor.
- chord.startAngle - Gets or sets the arc starting angle accessor.
- chord.endAngle - Gets or sets the arc end angle accessor.
- chord.source - Gets or sets the source radian accessor.
- chord.target - Gets or sets the target radian accessor.
- d3.svg.diagonal - Creates a new diagonal generator.
- diagonal - Generates a 2D Bezier connector for node connection graphs.
- diagonal.source - Gets or sets the source accessor.
- diagonal.target - Gets or sets the target point accessor.
- diagonal.projection - Gets or sets an optional point transformer.
- d3.svg.diagonal.radial - Creates a new slash generator.
- diagonal - Creates a 2D Bezier connector for node connection graphs.
Axes
- d3.svg.axis - Creates an axis generator.
- axis - officially generates axis in the page.
- axis.scale - get or set the scale transformation of the coordinate axis, which sets the conversion rules for numerical values and pixel positions.
- axis.orient - get or set the coordinate axis scale direction.
- axis.ticks - Controls how axis ticks are generated.
- axis.tickValues - Set specific axis values.
- axis.tickSize - Specifies the pixel length of the tick marks on the axis.
- axis.innerTickSize - get or set the pixel length of the coordinate axis minor tick mark.
- axis.outerTickSize - get or set the pixel length of the coordinate axis' large tick mark.
- axis.tickPadding - Specifies the pixel distance between axis ticks and tick text.
- axis.tickFormat - Set the format of tick text.
Controls
- d3.svg.brush - Click and drag to select a 2D area.
- brush - formally binds a brush to a certain area on the page.
- brush.x - get or set the x transformation of the brush, used for horizontal dragging.
- brush.y - get or set the y transformation of the brush, used for vertical dragging.
- brush.extent - get or set the brush selection range (extent).
- brush.clear - Set the brush selection range (extent) to empty.
- brush.empty - Determines whether the brush selection range (extent) is empty.
- brush.on - event listener for get or set brush. Three types of events can be monitored: brushstart, brush, brushend.
- brush.event - Trigger the listening event through the program and use it after setting the extent through the program.
d3.time (Time)
Time Formatting
- d3.time.format - Create a local time format converter based on a certain time format.
- format - Convert a date object into a string in a specific time format.
- format.parse - Convert a string in a specific time format into a date object.
- d3.time.format.utc - Creates a Coordinated Universal Time (UTC) format converter based on a certain time format.
- d3.time.format.iso - Creates an ISO Universal Time (ISO 8601 UTC) format converter based on a certain time format.
Time Scales
- d3.time.scale - Creates a linear time transformation whose domain is a numerical interval and its range is a time interval. Commonly used for creating time axis. For details, please refer to d3.scale.linear.
- scale - The input is a numerical value and the return is a time.
- scale.invert - Inverse transformation, input time and return numerical value.
- scale.domain - The domain of the get or set transformation.
- scale.nice - Expand the scope of the domain to make the domain more regular.
- scale.range - get or set the value range of the transformation.
- scale.rangeRound - Sets the range and rounds the result.
- scale.interpolate - The interpolation function of get or set transformation, such as replacing the default linear interpolation function with an exponential interpolation function.
- scale.clamp - Set whether the value range is closed or not. The default is not closed. When the range is closed, if the interpolation result is outside the range, the boundary value of the range will be taken. See linear.clamp for details.
- scale.ticks - Take representative values from the domain. Usually used to select coordinate axis scales.
- scale.tickFormat - Get the format conversion function, usually used for format conversion of coordinate axis scale.
- scale.copy - Copies a transform from an existing time transform.
Time Intervals
- d3.time.interval - Returns a time interval for local time.
- interval - The effect is the same as interval.floor method.
- interval.range - Returns the date within the specified interval.
- interval.floor - Round down to the nearest interval value.
- interval.round - Round up or down to the nearest interval value.
- interval.ceil - Round up to the nearest interval value.
- interval.offset - Returns the date offset for the specified time interval.
- interval.utc - Returns the corresponding UTC time interval.
- d3.time.day - Returns the specified time based on the start of the day (the default start is 12:00am).
- d3.time.days - Returns all days based on the specified time interval and interval conditions, the effect is the same as day.range.
- d3.time.dayOfYear - Calculate the number of days in the year for the specified time.
- d3.time.hour - Returns the specified time based on the start of the hour (e.g., 1:00 AM).
- d3.time.hours - Returns all hours based on the specified time interval and interval conditions, the effect is the same as hour.range.
- d3.time.minute - Returns the specified time based on the start of the minute (e.g., 1:02 AM).
- d3.time.minutes - Returns all minutes based on the specified time interval and interval conditions, the effect is the same as minute.range.
- d3.time.month - Returns the specified time based on the start of the month (e.g., February 1, 12:00 AM).
- d3.time.months - Returns all times based on months for the specified time interval and interval conditions, the effect is the same as month.range.
- d3.time.second - Returns the specified time starting in seconds (e.g., 1:02:03 AM).
- d3.time.seconds - Returns all times based on seconds for the specified time interval and interval conditions. The effect is the same as second.range.
- d3.time.sunday - Returns the specified time based on the start of Sunday (e.g., February 5, 12:00 AM).
- d3.time.sundays - Returns all times based on sunday for the specified time interval and interval conditions. The effect is the same as sunday.range.
- d3.time.sundayOfYear - Calculate the number of weeks in the year for the specified time with sunday as the base point.
- d3.time.monday - every Monday (e.g., February 5, 12:00 AM).
- d3.time.mondays - alias for monday.range.
- d3.time.mondayOfYear - computes the monday-based week number.
- d3.time.tuesday - every Tuesday (e.g., February 5, 12:00 AM).
- d3.time.tuesdays - alias for tuesday.range.
- d3.time.tuesdayOfYear - computes the tuesday-based week number.
- d3.time.wednesday - every Wednesday (e.g., February 5, 12:00 AM).
- d3.time.wednesdays - alias for wednesday.range.
- d3.time.wednesdayOfYear - computes the wednesday-based week number.
- d3.time.thursday - every Thursday (e.g., February 5, 12:00 AM).
- d3.time.thursdays - alias for thursday.range.
- d3.time.thursdayOfYear - computes the thursday-based week number.
- d3.time.friday - every Friday (e.g., February 5, 12:00 AM).
- d3.time.fridays - alias for friday.range.
- d3.time.fridayOfYear - computes the friday-based week number.
- d3.time.saturday - every Saturday (e.g., February 5, 12:00 AM).
- d3.time.saturdays - alias for saturday.range.
- d3.time.saturdayOfYear - computes the saturday-based week number.
- d3.time.week - alias for sunday.
- d3.time.weeks - alias for sunday.range.
- d3.time.weekOfYear - alias for sundayOfYear.
- d3.time.year - 返回指定时间基于年起始的时间(e.g., January 1, 12:00 AM).
- d3.time.years - 返回指定时间区间和间隔条件的所有时间,效果同year.range.
构图(d3.layout)
Bundle
- d3.layout.bundle - construct a new default bundle layout.
- bundle - apply Holten's hierarchical bundling algorithm to edges.
弦图(Chord)
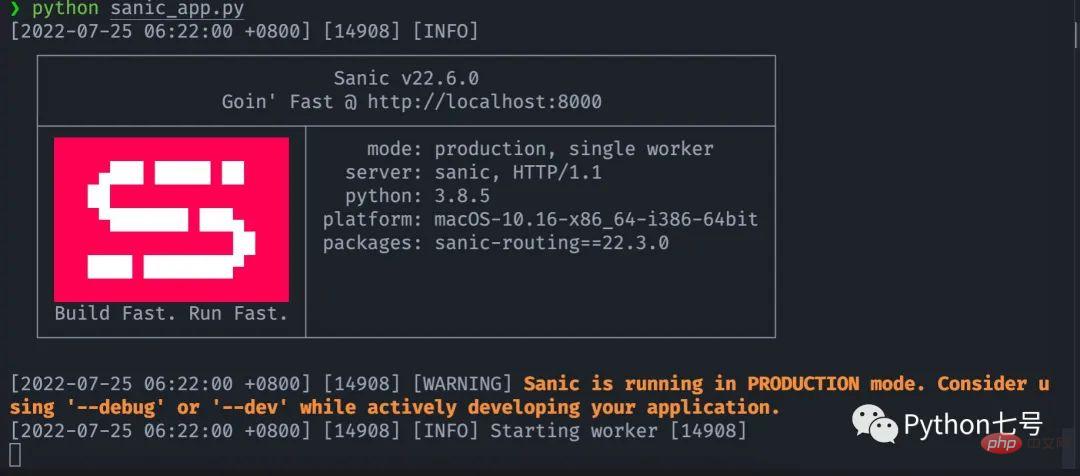
提到API开发,你可能会想到DjangoRESTFramework,Flask,FastAPI,没错,它们完全可以用来编写API,不过,今天分享的这个框架可以让你更快把现有的函数转化为API,它就是Sanic。Sanic简介Sanic[1],是Python3.7+Web服务器和Web框架,旨在提高性能。它允许使用Python3.5中添加的async/await语法,这可以有效避免阻塞从而达到提升响应速度的目的。Sanic致力于提供一种简单且快速,集创建和启动于一体的方法
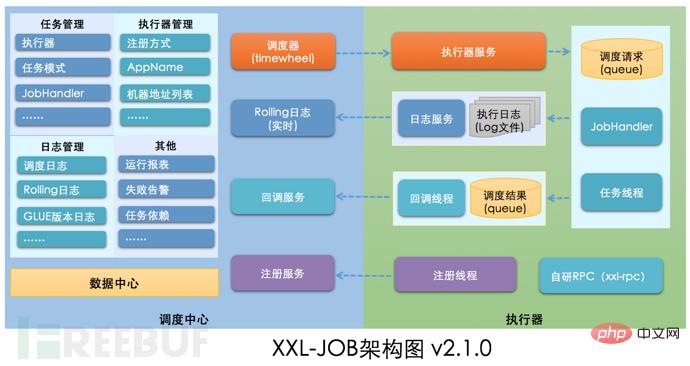
XXL-JOB描述XXL-JOB是一个轻量级分布式任务调度平台,其核心设计目标是开发迅速、学习简单、轻量级、易扩展。现已开放源代码并接入多家公司线上产品线,开箱即用。一、漏洞详情此次漏洞核心问题是GLUE模式。XXL-JOB通过“GLUE模式”支持多语言以及脚本任务,该模式任务特点如下:●多语言支持:支持Java、Shell、Python、NodeJS、PHP、PowerShell……等类型。●WebIDE:任务以源码方式维护在调度中心,支持通过WebIDE在线开发、维护。●动态生效:用户在线通
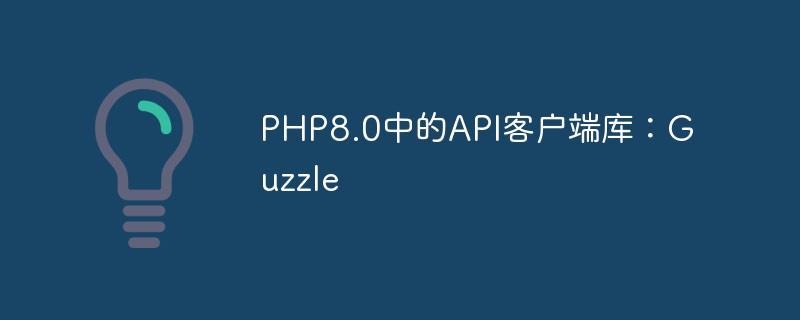
随着网络技术的发展,Web应用程序和API应用程序越来越普遍。为了访问这些应用程序,需要使用API客户端库。在PHP中,Guzzle是一个广受欢迎的API客户端库,它提供了许多功能,使得在PHP中访问Web服务和API变得更加容易。Guzzle库的主要目标是提供一个简单而又强大的HTTP客户端,它可以处理任何形式的HTTP请求和响应,并且支持并发请求处理。在
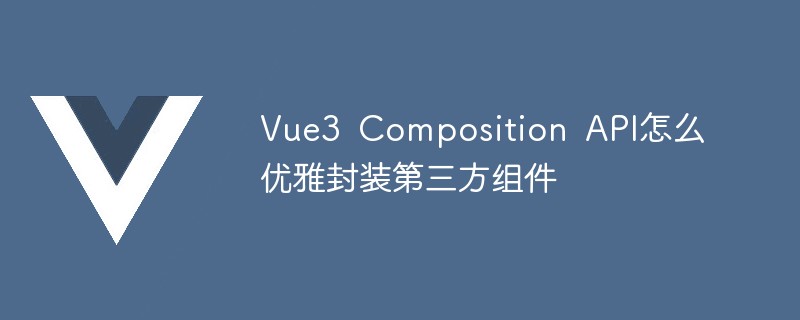
前言对于第三方组件,如何在保持第三方组件原有功能(属性props、事件events、插槽slots、方法methods)的基础上,优雅地进行功能的扩展了?以ElementPlus的el-input为例:很有可能你以前是这样玩的,封装一个MyInput组件,把要使用的属性props、事件events和插槽slots、方法methods根据自己的需要再写一遍://MyInput.vueimport{computed}from'vue'constprops=define
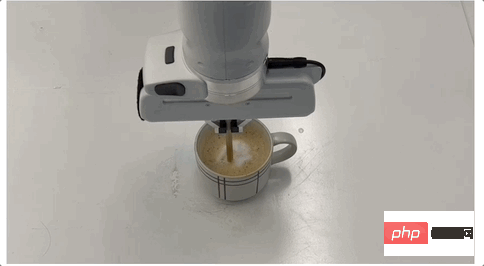
机器人也能干咖啡师的活了!比如让它把奶泡和咖啡搅拌均匀,效果是这样的:然后上点难度,做杯拿铁,再用搅拌棒做个图案,也是轻松拿下:这些是在已被ICLR 2023接收为Spotlight的一项研究基础上做到的,他们推出了提出流体操控新基准FluidLab以及多材料可微物理引擎FluidEngine。研究团队成员分别来自CMU、达特茅斯学院、哥伦比亚大学、MIT、MIT-IBM Watson AI Lab、马萨诸塞大学阿默斯特分校。在FluidLab的加持下,未来机器人处理更多复杂场景下的流体工作也都
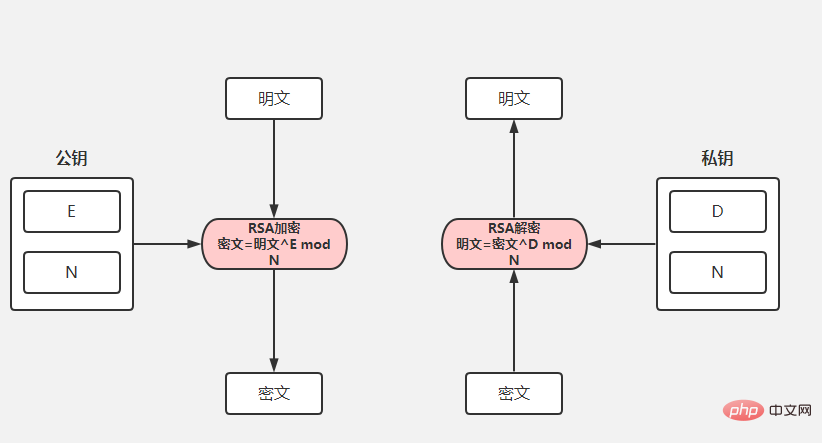
SpringBoot的API加密对接在项目中,为了保证数据的安全,我们常常会对传递的数据进行加密。常用的加密算法包括对称加密(AES)和非对称加密(RSA),博主选取码云上最简单的API加密项目进行下面的讲解。下面请出我们的最亮的项目rsa-encrypt-body-spring-boot项目介绍该项目使用RSA加密方式对API接口返回的数据加密,让API数据更加安全。别人无法对提供的数据进行破解。SpringBoot接口加密,可以对返回值、参数值通过注解的方式自动加解密。什么是RSA加密首先我
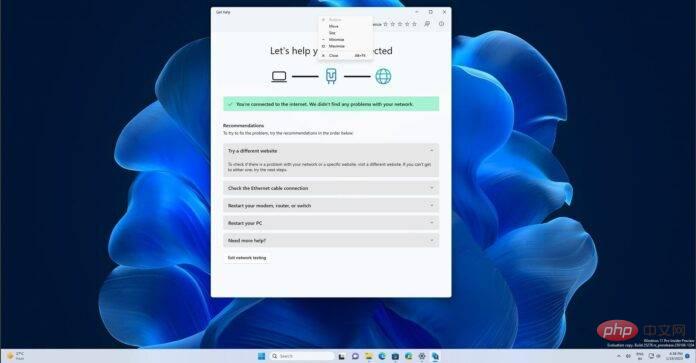
当您的WindowsPC出现网络问题时,问题出在哪里并不总是很明显。很容易想象您的ISP有问题。然而,Windows笔记本电脑上的网络并不总是顺畅的,Windows11中的许多东西可能会突然导致Wi-Fi网络中断。随机消失的Wi-Fi网络是Windows笔记本电脑上报告最多的问题之一。网络问题的原因各不相同,也可能因Microsoft的驱动程序或Windows而发生。Windows是大多数情况下的问题,建议使用内置的网络故障排除程序。在Windows11
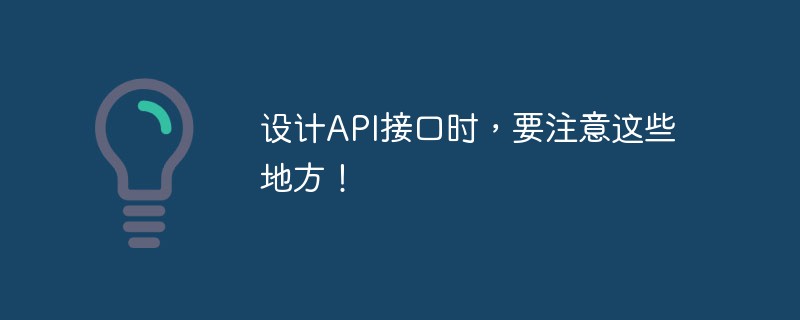
本篇文章给大家带来了关于API的相关知识,其中主要介绍了设计API需要注意哪些地方?怎么设计一个优雅的API接口,感兴趣的朋友,下面一起来看一下吧,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
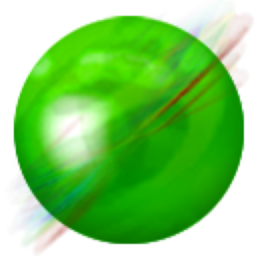
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
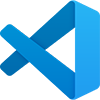
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
