


A brief discussion on the stringify function, toJosn function and parse function in JSON_json
JSON.stringify function (JavaScript)
Syntax: JSON.stringify(value [, replacer] [, space])
Convert a JavaScript value to a JavaScript Object Notation (Json) string.
value
Required. The JavaScript value to convert (usually an object or array).
replacer
Optional. The function or array used to convert the result.
If replacer is a function, JSON.stringify will call the function, passing in the key and value of each member. Use the return value instead of the original value. If this function returns undefined, the member is excluded. The key of the root object is an empty string: "".
If replacer is an array, only members with key values in the array are converted. Members are converted in the same order as the keys in the array. When the value argument is also an array, the replacer array is ignored.
space
Optional. Adds indentation, spaces, and newlines to the return value JSON text to make it easier to read.
If space is omitted, the return value text will be generated without any extra spaces.
If space is a number, the return value text is indented by the specified number of spaces at each level. If space is greater than 10, the text is indented by 10 spaces.
If space is a non-empty string (such as " "), the return value text is indented at each level by characters in the string.
If space is a string longer than 10 characters, the first 10 characters are used.
If value has a toJSON method, the JSON.stringify function will use the return value of that method. If the return value of the toJSON method is undefined, the member is not converted. This enables the object to determine its own JSON representation.
Values that do not have a JSON representation, such as undefined, will not be converted. In the object, these values are discarded. In the array, these values are replaced with null.
Execution order
During the serialization process, if the value parameter corresponds to the toJSON method, JSON.stringify will first call the toJSON method. If the method does not exist, the original value is used. Next, if the replacer parameter is supplied, that value (either the original value or the toJSON return value) is replaced with the return value of the replacer parameter. Finally, spaces are added to the value based on the optional space parameter to produce the final JSON text.
This example uses JSON.stringify to convert a contact object to JSON text. Define the memberfilter array to convert only the surname and phone members. Omit the firstname member.
var contact = new Object();
contact.firstname = "Jesper";
contact.surname = "Aaberg";
contact.phone = ["555-0100", "555-0120"];
var memberfilter = new Array();
memberfilter[0] = "surname";
memberfilter[1] = "phone";
var jsonText = JSON.stringify(contact, memberfilter, "t");
document.write(jsonText);
// Output:
// { "surname": "Aaberg", "phone": [ "555-0100", "555-0120" ] }
toJSON method (Date) (JavaScript)
Syntax: objectname.toJSON()
objectname
Required. Object that needs to be JSON serialized.
The toJSON method is a built-in member of the Date JavaScript object. It returns an ISO-formatted date string in the UTC time zone (represented by the suffix Z).
The following example uses the toJSON method to serialize uppercase string member values. The toJSON method is called when calling JSON.stringify.
JavaScript
var contact = new Object();
contact.firstname = "Jesper";
contact.surname = "Aaberg";
contact.phone = ["555-0100", "555-0120"];
contact.toJSON = function(key)
{
var replacement = new Object();
for (var val in this)
{
If (typeof (this[val]) === 'string')
replacement[val] = this[val].toUpperCase();
else
replacement[val] = this[val]
}
Return replacement;
};
var jsonText = JSON.stringify(contact);
/* The value of jsonText is:
'{"firstname":"JESPER","surname":"AABERG","phone":["555-0100","555-0120"]}'
*/
JSON.parse function (JavaScript)
Convert JavaScript Object Notation (JSON) string to object
Syntax: JSON.parse(text [, reviver])
text
Required. A valid JSON string.
reviver
Optional. A function that converts the result. This function will be called for each member of the object. If a member contains nested objects, the nested objects are converted before the parent object. For each member, the following happens:
If reviver returns a valid value, the member value is replaced with the converted value.
If the reviver returns the same value it received, the member value is not modified.
If reviver returns null or undefined, the member is removed.
The following example uses JSON.parse to convert a JSON string into an object.
var jsontext = '{"firstname":"Jesper","surname":"Aaberg","phone":["555-0100","555-0120"]}';
var contact = JSON.parse(jsontext);
document.write(contact.surname ", " contact.firstname);
// Output: Aaberg, Jesper
The following example demonstrates how to use JSON.stringify to convert an array into a JSON string, and then use JSON.parse to convert the string back into an array.
var arr = ["a", "b", "c"];
var str = JSON.stringify(arr);
document.write(str);
document.write ("
");
var newArr = JSON.parse(str);
while (newArr.length > 0) {
Document.write(newArr.pop() "
");
}
// Output:
// ["a","b","c"]
// c
// b
// a
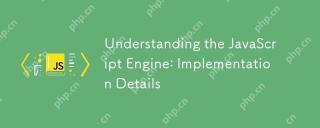
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
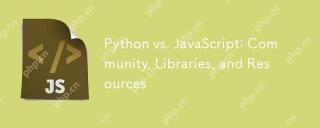
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
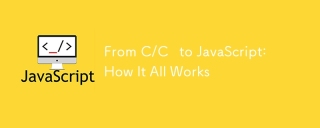
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
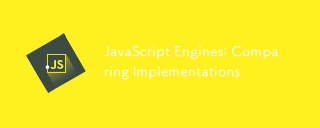
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
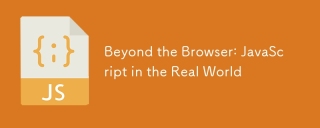
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
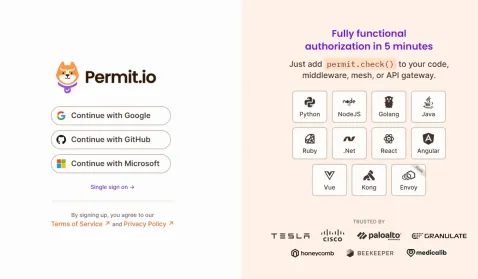
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
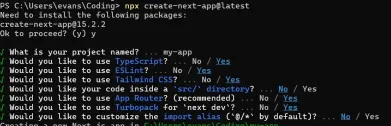
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
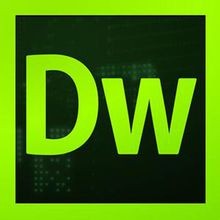
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
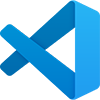
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft