Before reading this book, I would like to thank the Taobao technical team for translating this JavaScript core, and thank Flanagan for writing this book. Thank you for your selfless sharing, and I only dedicate this note to your hard work.
1: JavaScript language core
After this chapter, we will mainly focus on the basics of javascript. In Chapter 2, we explain JavaScript comments, semicolons and unicode character sets; Chapter 3 will be more interesting, mainly explaining JavaScript variables and assignments
Here are some example codes illustrating the key points of the first two chapters.
The most common way to write expressions in JavaScript is an operator like the following code
//Operator serves as an operator and generates a new value
//The most common arithmetic operators
3 2 // =>5 Addition
3-2 // =>Subtraction
3*2 // =>Multiplication
// => Point[1].x -point[0].x //=>Complex operations can also work as usual
"3" "2" // => 32. Addition operations can be completed, and string concatenation can also be completed.
//Javascript defines some arithmetic operators as shorthand forms
var count = 0; //Define a variable
Count ; //Increase by 1
count--; Count = 2; // Self -increase 2 and "Count = Count 2;" The writing method is the same
Count *= 3 // Self -ride 3. "Count = Count *3;" Writing
//=> 6: The variable name itself is also an expression
//Equality relational operator is used to determine whether two values are equal
//The result of the inequality, greater than, and less than operators is true or false
var x=2,y=3; x == y; x! = y; x y; x >= y; "two"=="three"; //false The two strings are not equal
"two" > "three"; //true "tw" has a greater index in the alphabet than "th"
false == (x>y); //ture false =false;
//Logical operators are the combination or negation of Boolean values
(x == 2)&&(y==3); //=>true Both comparisons are true.&& is "AND"
(x > 3) || (y false Neither comparison is true. || means "or"
(x == y); //=>true! Indicates negation
If the "phrase" in JavaScript is an expression, then the entire sentence is called a statement, which will be explained in detail in Chapter 5.
In the above code, the lines ending with a semicolon are all statements. Roughly speaking, the expression only calculates a value (or the value it contains we don't care about) but they change the running state of the program. In the above, we have seen variable declaration statements and assignment statements. Another type of statement is the "control structure", such as conditional judgments and loops. After introducing the functions, we give relevant sample codes.
A function is a JavaScript code segment with a name and parameters that can be defined once and used multiple times. Chapter 8 will explain functions in formal detail. Like objects and arrays, functions are mentioned in many places in this book. Here are some simple example codes.
//The function is a javascript code segment with treatment parameters, which can be dispatched multiple times
function plus1(x) { //A function named plus1 is defined with parameter x
Return x 1; //Return a value that is 1 greater than the value passed in.
// The code block of the function is the part wrapped in curly braces
plus1(y) //
VAR SQUARE = FUNCTION (X) {// The function is a value that can be assigned to the variable
Return x*x; // Calculate the value of the function
}; // Send the end of the assignment statement
square(plus1(y));
//When a function assigns a value to an object's property, we call it
//"Method", all JavaScript objects contain methods
Var a = []; // Create an empty array
a.push(1,2,3); a.reverse(); // document.write(a)
//We can define the method of the child, the "this" keyword is the definition method
A reference to the object. The example here is the array containing the position information of the two points mentioned above.
POINTS.DIST = Function () {// Define the distance between two points to calculate the distance between the two points
Var p1 = this [0]; // Reference to the current array through this keyword
var p2 =this[1]; //And get the first two elements of the called array
Var a = p2.x- p1.y; // X's distance on the coordinate shaft
var b =p2.y - p1.y; //Distance on the y coordinate axis
return Math.sqrt(a * a "we call it" b * b); //Pythagorean Theorem
}; //Math.sqrt() calculates the square root
Points.dist() // => Find the distance between two points
Now, give some examples of control statements. The example function body here contains the most common javascript control statements
//The javascript statements here use this syntax to include conditional judgments and loops
//Using syntax similar to java c and other languages
function abs(x) { //Find the absolute value function
If (x >= 0) { //if
Return x; //If true, execute this code
} Else {// false executes
return -x;
}
}
function factprial(n) { //Calculate factorial
var product = 1; //Assign the value to product as 1
While (n & gt; 1) {// () value expression executes {} content
Product *= n; //product = abbreviation for product * n
n--; // n = n-1 writing
// End of loop
return product; //Return product
}
factprial(4) // =>24 1*4*3*2 document.write(factprial(4))
function factorial2(n) { //Another way to write a loop
var i, product = 1; //
for (i = 2; i Product *= i; // The cycle body, when there is only one code in the circular body, omit {}
return product; //Calculate and return a good factorial
}
factorial2(5) //document.write(factorial2(5)) =>120: 1*2*3*4*5
JavaScript is an object-oriented programming language, but it is very different from traditional page objects. Chapter 9 will explain the object-oriented nature of JavaScript in detail. This chapter will have a lot of sample code, which is the best part of this book. The longest chapter.
Here is a simple example, this code shows how to define a class in javascript to represent points in the geometry of a 2D face. The object instantiated by this class has a method named r(), which is used to calculate the distance from the change point to the origin.
//Define a constructor to initialize a new point object
function Point(x, y) { //Constructors generally start with a capital letter
This.x = x; //The keyword this refers to the initialized instance
this.y = y; //Storage function parameters as attributes of the object
}
//Use the new keyword and constructor to create an instance
var p = new Point(1, 1); //Point 1,1,
in plane geometry //Assign value through constructor prototype object
// Come to define the Point object
Point.prototype.r = function() {
return Math.sqrt( //Return the square root of x squared y squared
This.x * this.x //this refers to the object that dispatches this method
This.y * this.y);
};
//Point instance object p (and all point instance objects) inherits the method r()
p.r() // => 1.4142135623730951 /document.write(p.r())
Chapter 9 is the essence of the first part . The subsequent chapters have been sporadic extensions and will lead us to the end of our exploration of JavaScript.
Chapter 10 mainly talks about text matching patterns using regular expressions.
Chapter 11 is a subset and superset of the core JavaScript language .
Before entering the content of client-side javascript, in Chapter 12 we only need to introduce two javascript running environments outside the web.
2. Client javascript
There are many cross-references in the core parts of the JavaScript language, and the knowledge hierarchy is not clear. Content layout in client-side JavaScript has changed a lot. By following this chapter, you can use JavaScript in your web browser. (But if you want to learn javascript by reading this book, you can't just focus on the second part) Chapter 13 is the first chapter of the second part. This chapter introduces how to make javascript run in a web browser. Chapter 14 explains web browser scripting technology and covers an important global function of client-side JavaScript.
For example:
function moveon() {
//Ask a question through the dialog box
var answer = confirm("Are you ready?");
//Click OK and the browser will load a new page
If (answer) window.location = "http://www.baidu.com";
}
//Execute this function after 1 minute (60000 milliseconds)
setTimeout(moveon,300);
Chapter 15 will describe how JavaScript manipulates the display of HTML style definition content . The content of Chapter 15 will be more pragmatic, manipulating the content of HTML documents through scripts, and it will show how to select specific Web page elements, how to set attributes for html elements, how to modify the content of elements, and how to add new nodes to the document
The following example function shows how to find and modify basic article content
// Super debugging information in the area tree of a specified information in the document
//If this element does not exist on the document, create one
function debug(msg) {
//Find the debugging part of the document by looking at the id attribute of the html element
var log = document.getElementById("debuglog");
//If the element does not exist, create one
if (!log) {
log = document.createElement("div"); //Create a new div element
log.id = "debuglog"; //Assign a value to the id of each element
log.innerHTML = "
Debug Log
"; //Customize initial contentdocument.body.appendChild(log); //Add it to the end of the document
}
//Include the message in
and add it to the log<br> var pre = document.createElement("pre"); //Create pre element<br> var text = document.createElement(msg); //Include msg on a text node <br> pre.appendChild(text); //Text is added to pre<br> log.appendChild(pre); //pre is added to log<br> }<br>
In Chapter 16, we will talk about how to use javascript to operate elements . This usually uses the style and class attributes of the element
function hide(e, reflow) { //Manipulate elements and hide elements through jvascript e
if (reflow) { //If the second parameter is true
e.style.display = "none" //Hide this element and the space it occupies is also sold
} else {
e.style.visibility = "hidden"; //Hide e and retain the space it occupies
}
}
function highlight(e) { //Highlight e by setting css
If (!e.className) e.className = "highcss";
Else
e.className = "highcss";
}
You can control the content and CSS style of elements through JavaScript, and you can also define document behavior through event handlers. Event handlers are a JavaScript function registered in the browser center. When a specific event occurs The browser can then call this function.
Usually the event types we are concerned about are mouse clicks and keyboard key events (smartphones are various touch events). In other words, when the browser completes loading the document, when the user changes the size of the window or when the user enters data into the form, a human event will be triggered.
Chapter 17 will describe in detail how to define and register time handlers, and how the browser calls them when the event occurs.
The simplest way to customize the event handler is to bind a callback to the HTML attribute prefixed with on. When writing some simple program tests, the most practical way is to bind a callback to the "onclick" handler. . Assuming that the above debug() and hide() functions are saved to the debug.js and hide.js files, then a simple html test file can be used to specify an event handler for the onclick attribute. As follows

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
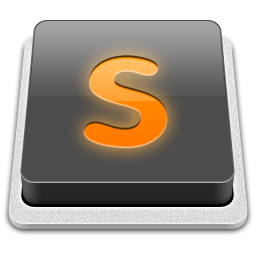
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
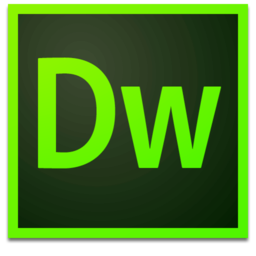
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool