child_process implements multi-process in Node.js_node.js
var http = require('http');
function fib (n) {
If (n Return 1;
} else {
Return fib(n - 2) fib(n - 1);
}
}
var server = http.createServer(function (req, res) {
var num = parseInt(req.url.substring(1), 10);
res.writeHead(200);
res.end(fib(num) "n");
});
server.listen(8000);
The above example provides a Fibonacci sequence calculation service. Since this calculation is quite time-consuming and is single-threaded, when there are multiple requests at the same time, only one can be processed. This can be solved by child_process.fork() This question
Here is an example from the official website, through which you can better understand the function of fork()
var cp = require('child_process');
var n = cp.fork(__dirname '/sub.js');
n.on('message', function(m) {
console.log('PARENT got message:', m);
});
n.send({ hello: 'world' });
The result of executing the above code snippet:
PARENT got message: { foo: 'bar' }
CHILD got message: { hello: 'world' }
The content of sub.js is as follows:
process.on('message', function(m) {
console.log('CHILD got message:', m);
});
process.send({ foo: 'bar' });
In the child process, the process object has a send() method, and it will publish a message object every time it receives a message
What’s a little confusing is: the message sent by child.send() is received by the process.on() method, and the message sent by the process.send() method is received by the child.on() method
Referring to this example, we can improve the first service that provides Fibonacci data so that each request has a separate new process to handle
var http = require('http');
var cp = require('child_process');
var server = http.createServer(function(req, res) {
var child = cp.fork(__dirname '/fibonacci-calc.js');//Each request generates a new child process
child.on('message', function(m) {
res.end(m.result 'n');
});
var input = parseInt(req.url.substring(1));
child.send({input : input});
});
server.listen(8000);
fibonacci-calc.js
function fib(n) {
If (n Return 1;
} else {
Return fib(n - 2) fib(n - 1);
}
}
process.on('message', function(m) {
Process.send({result: fib(m.input)});
});
After starting the service, visit http://localhost:8080/9 to calculate the value of the Fibonacci sequence of 9
The above is the entire content of this article, I hope you all like it.
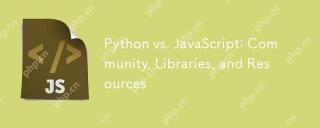
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
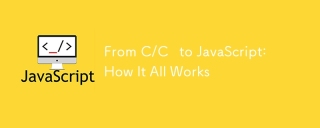
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
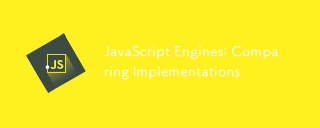
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
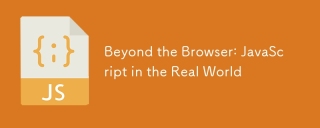
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
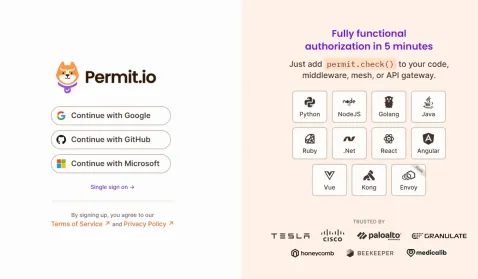
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
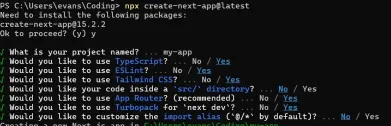
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
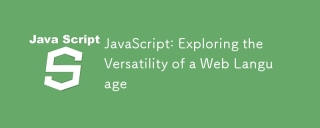
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
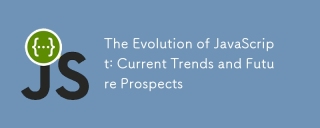
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
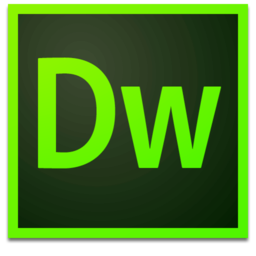
Dreamweaver Mac version
Visual web development tools