I haven’t posted a post for a long time. Today I suddenly analyzed the implementation principle of the extend method in JQuery. The purpose is to improve my understanding of JQuery, and also want to understand how JavaScript masters write JS. Please correct me if there are any deficiencies. Thanks!
The following is the source code of JQuery.extend method:
jQuery.extend = jQuery.fn.extend = function() {
var options, name, src, copy, copyIsArray, clone,
target = arguments[0] || {}, // target object
i = 1,
length = arguments.length,
deep = false;
// Handle deep copy situation (the first parameter is boolean type and true)
If ( typeof target === "boolean" ) {
deep = target;
target = arguments[1] || {};
// Skip the first parameter (whether to deep copy or not) and the second parameter (target object)
i = 2;
}
// If the target is not an object or function, initialize to an empty object
If ( typeof target !== "object" && !jQuery.isFunction(target) ) {
Target = {};
}
// If only one parameter is specified, jQuery itself is used as the target object
If ( length === i ) {
target = this;
--i;
}
for ( ; i < length; i ) {
// Only deal with non-null/undefined values
If ( (options = arguments[ i ]) != null ) {
// Extend the base object
for ( name in options ) {
src = target[name];
copy = options[ name ];
// Prevent never-ending loop
If ( target === copy ) {
Continue;
// If the object contains an array or other objects, use recursion to copy
If ( deep && copy && ( jQuery.isPlainObject(copy) || (copyIsArray = jQuery.isArray(copy)) ) ) {
// Processing arrays
If (copyIsArray) {
copyIsArray = false;
// If the array does not exist in the target object, create an empty array;
clone = src && jQuery.isArray(src) ? src : [];
clone = src && jQuery.isPlainObject(src) ? src : {};
// Never change the original object, only make a copy
target[ name ] = jQuery.extend( deep, clone, copy );
// Do not copy UNDEFINED value
target[name] = copy;
}
// Return the modified object
Return target;
};
From the above analysis, we can see that the extend function supports deep copy. So what is deep copy in JS?
My understanding is as follows: If an object contains a reference object (such as an array or object), then when copying the object, it is not simply a copy of the address of the reference object, but the content of the reference object is copied and saved as a separate object. (As shown below).
From the picture above, you can see that two student objects share a friend object. The operations of one party on the friend object are also visible to the other party. For example: if you change your friend's last name to "zhangsan", then the other object can also see it.
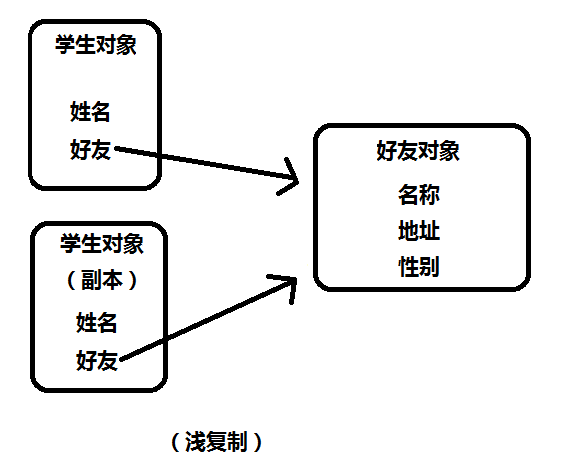
As can be seen from the picture above, both student objects have their own friend objects, and the modification of one is completely transparent to the other (without any impact). The above is my understanding of deep copying. If there is anything wrong, please don’t laugh. Thank you.
So how does the JQuery.extend method implement shallow copy and deep copy?
How to use JQuery.extend:
1. JQuery.extend (source object)
Expand the source object to the jQuery object, that is, copy the properties and methods of the source object to jQuery. Use jQuery as the target object, the source code is as follows:
Copy code
The code is as follows:
// If only one parameter is specified, use jQuery itself as the target object
if ( length === i ) {
target = this;
--i;
}
【Example 1】: Extend the method of person object to jQuery object.
Copy code
ShowName : function(name){
alert("Name: " name);
}
};
jQuery.extend(person); // Extend the person object to the jQuery($) object
jQuery.showName("admin"); // Name: admin
$.showName("admin"); // Name: amdin
alert("Sex: " $.sex); // Sex: male
[Example 2] Verify that using this form of extend method is shallow copy.
Copy code
ShowName : function(name){
alert("Name: " name);
}
};
jQuery.extend(person); // Extend the person object to the jQuery($) object
alert($.language); // java, c, sql
$.language.push('PL/SQL'); // Modify the expanded object
alert(person.language); // java, c, sql, PL/SQL
person.language.pop();
alert($.language); // java, c, sql
From the above example, we can find that any modification of the language array by either the expanded object ($) or the source object (person) will affect the other party. This is shallow copy
2. JQuery.extend (target object, source object)
Copy the properties and methods of the source object to the target object, using shallow copy.
[Example] Create person and student objects respectively, and then extend the person's attributes and methods to the student object through the jQuery.extend method.
var person = {
Language: ['java', 'c ', 'sql'],
ShowName : function(name){
alert("Name: " name);
}
};
var student = {
ShowNum : function(num){
alert("Num: " num);
}
};
jQuery.extend(student, person); // Extend the person object to the specified student object
student.showName("admin");
alert(student.language);
3. JQuery.extend (boolean, source object)
The boolean parameter in this method indicates whether to use deep copy. If it is true, deep copy will be used.
[Example] Extend the person object to the jQuery object
var person = {
Language: ['java', 'c ', 'sql'],
ShowName : function(name){
alert("Name: " name);
}
};
jQuery.extend(true, person); // Extend the person object to the jQuery object
alert($.language); // java, c, sql
$.language.push('PL/SQL'); // Modify the expanded object
alert(person.language); // java, c, sql
person.language.pop();
From the above example, we can see that modifications to $.language will not affect the language attribute in person. This is deep copy
4. JQuery.extend (boolean, target object, source object)
Determines whether to use deep copy to extend the source object onto the target object. As follows:
[Example] Create person and student objects respectively, and then extend the person's attributes and methods to the student object through the jQuery.extend method.
var person = {
ShowName : function(name){
alert("Name: " name);
}
};
var student = {
Language: ["java", "c ", "javascript"],
ShowNum : function(num){
alert("Num: " num);
}
};
var target = jQuery.extend(person, student);
alert(target.language); // java, c, javascript
target.language.push("PL/SQL");
alert(student.language); // java, c, javascript, PL/SQL
student.language.pop();
alert(target.language); // java, c, javascript
var target2 = jQuery.extend(true, person, student);
alert(target2.language); // java, c, javascript
target2.language.push("PL/SQL");
alert(student.language); // java, c, javascript
student.language.pop();
alert(target2.language); // java, c, javascript, PL/SQL
The above is my understanding of the extend method. Please correct me if there is anything incorrect. Thank you very much!