#-*- coding: utf-8 -*- import datetime #给定日期向后N天的日期 def dateadd_day(days): d1 = datetime.datetime.now() d3 = d1 + datetime.timedelta(days) return d3 #昨天 def getYesterday(): today = datetime.date.today() oneday = datetime.timedelta(days=1) yesterday = today - oneday return yesterday #今天 def getToday(): return datetime.date.today() #获取给定参数的前几天的日期,返回一个list def getDaysBefore(num): today = datetime.date.today() oneday = datetime.timedelta(days=1) li = [] for i in range(0, num): #今天减一天,一天一天减 today = today - oneday #把日期转换成字符串 li.append(datetostr(today)) return li #将字符串转换成datetime类型 def strtodatetime(datestr, format): return datetime.datetime.strptime(datestr, format) #时间转换成字符串,格式为2015-02-02 def datetostr(date): return str(date)[0:10] #时间转换成字符串,格式为2015-02-02 def datetostr_secod(date): return str(date)[0:19] #两个日期相隔多少天,例:2015-2-04和2015-3-1 def datediff(beginDate, endDate): format = "%Y-%m-%d" bd = strtodatetime(beginDate, format) ed = strtodatetime(endDate, format) oneday = datetime.timedelta(days=1) count = 0 while bd != ed: ed = ed - oneday count += 1 return count #两个日期之间相差的秒 def datediff_seconds(beginDate, endDate): format = "%Y-%m-%d %H:%M:%S" if " " not in beginDate or ':' not in beginDate: bformat = "%Y-%m-%d" else: bformat = format if " " not in endDate or ':' not in endDate: eformat = "%Y-%m-%d" else: eformat = format starttime = strtodatetime(beginDate, bformat) endtime = strtodatetime(endDate, eformat) ret = endtime - starttime return ret.days * 86400 + ret.seconds #获取两个时间段的所有时间,返回list def getDays(beginDate, endDate): format = "%Y-%m-%d" begin = strtodatetime(beginDate, format) oneday = datetime.timedelta(days=1) num = datediff(beginDate, endDate) + 1 li = [] for i in range(0, num): li.append(datetostr(begin)) begin = begin + oneday return li #获取当前年份 是一个字符串 def getYear(date=datetime.date.today()): return str(date)[0:4] #获取当前月份 是一个字符串 def getMonth(date=datetime.date.today()): return str(date)[5:7] #获取当前天 是一个字符串 def getDay(date=datetime.date.today()): return str(date)[8:10] #获取当前小时 是一个字符串 def getHour(date=datetime.datetime.now()): return str(date)[11:13] #获取当前分钟 是一个字符串 def getMinute(date=datetime.datetime.now()): return str(date)[14:16] #获取当前秒 是一个字符串 def getSecond(date=datetime.datetime.now()): return str(date)[17:19] def getNow(): return datetime.datetime.now() print dateadd_day(10) #2015-02-14 16:41:13.275000 print getYesterday() #2015-02-03 print getToday() #2015-02-04 print getDaysBefore(3) #['2015-02-03', '2015-02-02', '2015-02-01'] print datediff('2015-2-01', '2015-10-05') #246 print datediff_seconds('2015-02-04', '2015-02-05') #86400 print datediff_seconds('2015-02-04 22:00:00', '2015-02-05') #7200 print getDays('2015-2-03', '2015-2-05') #['2015-02-03', '2015-02-04', '2015-02-05'] print datetostr_secod(getNow()) #2015-02-04 16:46:47 print str(getYear(dateadd_day(-50))) + '-'\ + getMonth() + '-'\ + getDay() + ' '\ + getHour() + ':'\ + getMinute() + ':'\ + getSecond() #2014-02-04 16:59:04 print getNow() #2015-02-04 16:46:47.454000
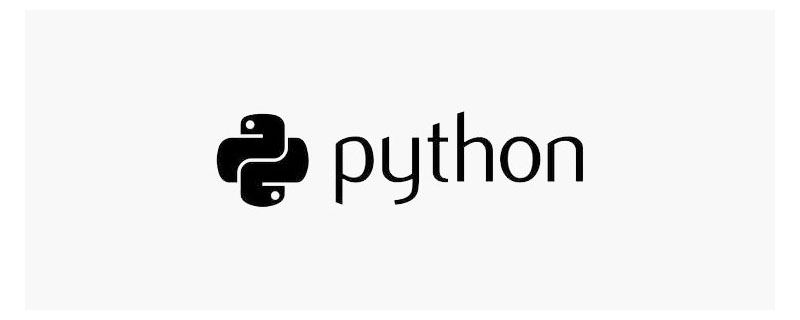
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
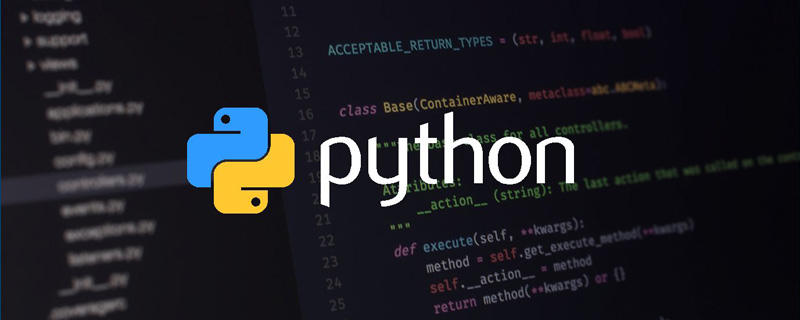
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
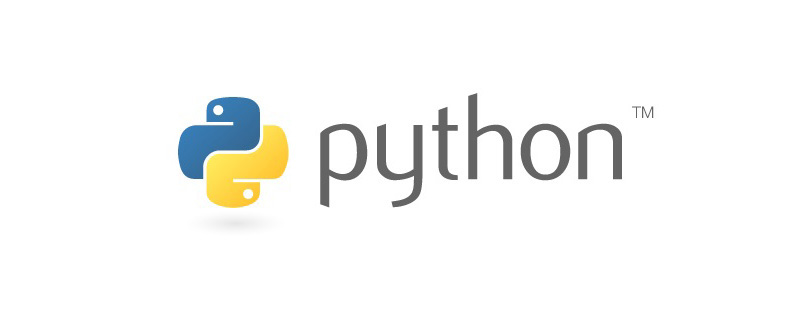
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
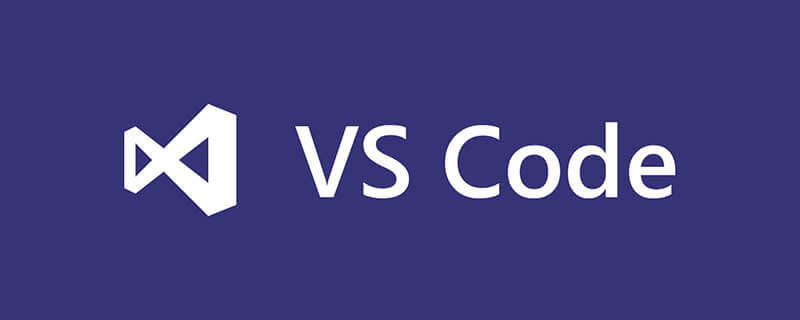
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
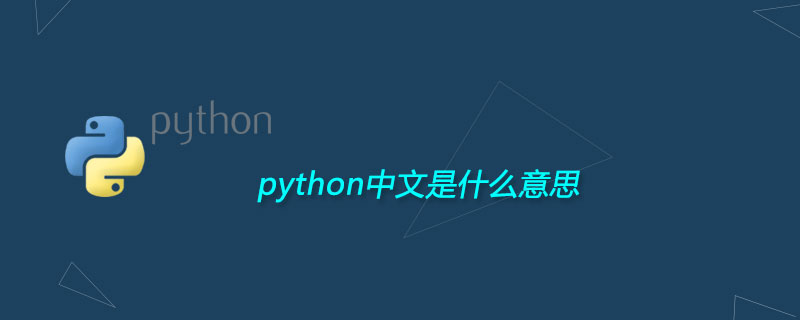
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。
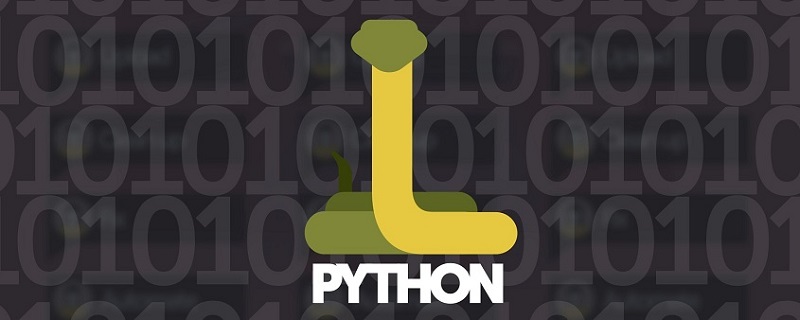
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
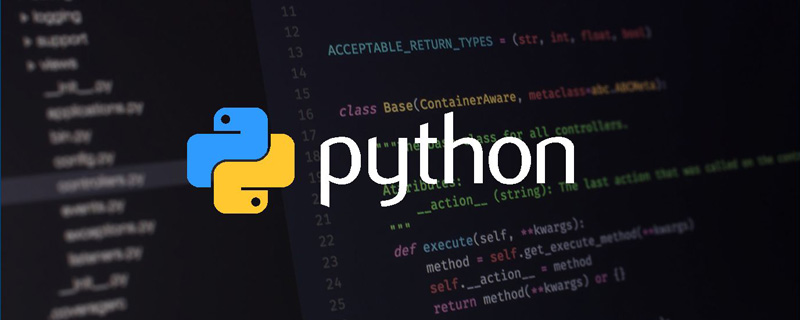
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
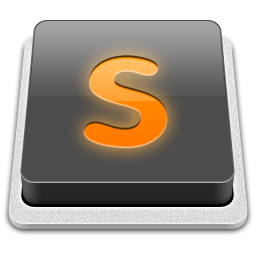
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
