Revealing common problems in the application of Java design patterns
Common problems with the application of design patterns in Java include: overuse, not understanding the intent, confusing patterns and anti-patterns, and over-design. Practical examples show how the Strategy pattern makes algorithms client-independent, allowing algorithm selection at runtime.
Revealing common problems in the application of Java design patterns
In the design and development of Java applications, design patterns are a A powerful tool for solving common problems and improving code reusability. However, there are some common pitfalls in applying design patterns that can lead to code complexity or maintenance issues.
1. Overuse of design patterns
The most common mistake is the overuse of design patterns. Design patterns are a tool and should be used with caution. Misuse of design patterns can lead to code that is redundant, difficult to maintain, and violates SOLID principles.
2. Not understanding the intent of the pattern
A common mistake developers make when applying design patterns is that they don’t really understand the intent of the pattern or the circumstances in which it applies. . This can lead to misuse or abuse of the pattern, thereby defeating its intended effect.
3. Confusing patterns and anti-patterns
Design patterns and anti-patterns are easily confused. Design patterns are good solutions to specific problems, while anti-patterns are common pitfalls that should be avoided. It is crucial to understand the difference between the two to avoid making mistakes.
4. Over-design
Another common problem is over-design. Developers may rely too much on design patterns even when they don't have to. Over-engineering can lead to unnecessary complexity and hard-to-understand code.
Practical Case: Strategy Pattern Application
Strategy pattern is a design pattern used to define a family of algorithms so that the algorithm can be independent of the client using it And change. Let's look at a practical case of using the strategy pattern:
interface SortingStrategy { int[] sort(int[] numbers); } class BubbleSortStrategy implements SortingStrategy { @Override public int[] sort(int[] numbers) { // Bubble sort implementation... return numbers; } } class SelectionSortStrategy implements SortingStrategy { @Override public int[] sort(int[] numbers) { // Selection sort implementation... return numbers; } } class SortingContext { private SortingStrategy strategy; public SortingContext(SortingStrategy strategy) { this.strategy = strategy; } public int[] sort(int[] numbers) { return strategy.sort(numbers); } } public class Main { public static void main(String[] args) { int[] numbers = { 5, 3, 1, 2, 4 }; SortingContext context = new SortingContext(new BubbleSortStrategy()); numbers = context.sort(numbers); // Change strategy to selection sort context = new SortingContext(new SelectionSortStrategy()); numbers = context.sort(numbers); for (int number : numbers) { System.out.println(number); } } }
In this example, the SortingStrategy
interface defines a set of sorting algorithms. BubbleSortStrategy
and SelectionSortStrategy
implement these algorithms. SortingContext
class uses the strategy pattern, allowing the sorting algorithm to be selected at runtime as needed.
The above is the detailed content of Revealing common problems in the application of Java design patterns. For more information, please follow other related articles on the PHP Chinese website!
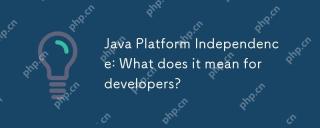
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
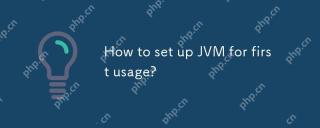
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
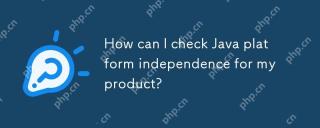
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss
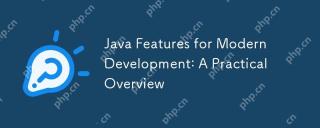
Javastandsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhancedconcurrencysupport.1)Lambdaexpressionssimplifyfunctionalprogramming,makingcodemoreconciseandreadable.2)Streamsenableefficientdataprocessingwithoperationsli
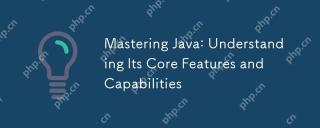
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
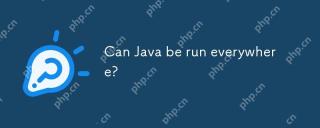
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
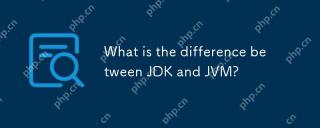
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
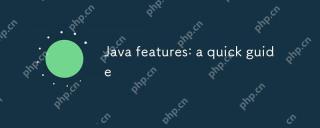
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
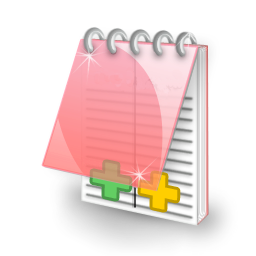
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
