


Exception handling in C++ technology: How to use exception classes to encapsulate exception information?
C The exception handling mechanism allows to encapsulate exception information, derive from std::exception through the exception class and use throw to throw exceptions. This class provides the what() method to obtain error messages, which can be used to handle specific exceptions in catch blocks to improve the clarity and efficiency of error handling.
Use exception class to encapsulate exception information in C
Introduction
Exception handling It is a built-in mechanism in C for handling errors and exceptions. By throwing an exception, the program can notify the caller that a problem has occurred. Exception classes allow us to encapsulate additional information about the exception, such as error code, description, or stack trace.
Syntax for using exception classes
When defining an exception class in C, it is usually derived from std::exception
:
class MyException : public std::exception { public: // 构造函数 MyException(const std::string& error_message) : _error_message(error_message) {} // 获取错误消息 const char* what() const noexcept override { return _error_message.c_str(); } private: std::string _error_message; };
Throwing exceptions
To throw our own exceptions, we can use throw
Keywords:
try { // 可能会抛出异常的代码 } catch(MyException& e) { // 处理异常 std::cout << "异常消息:" << e.what() << std::endl; }
Actual combat Case
Suppose we have a function that opens a file. If the file does not exist, it will throw MyException
exception:
void open_file(const std::string& filename) { std::ifstream file(filename); if (!file) { throw MyException("文件 '" + filename + "' 不存在。"); } }
In the main function, we can call the open_file
function and handle the potential exception:
int main() { try { open_file("example.txt"); // 如果没有异常抛出,则继续执行 } catch(MyException& e) { std::cout << "错误:" << e.what() << std::endl; } return 0; }
Summary
By using exception classes, we can encapsulate additional information about exceptions, making error handling clearer and more efficient. Exception classes enable us to provide error details in different parts of the application and capture and handle various error conditions.
The above is the detailed content of Exception handling in C++ technology: How to use exception classes to encapsulate exception information?. For more information, please follow other related articles on the PHP Chinese website!
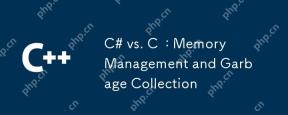
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
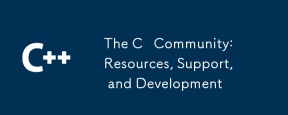
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
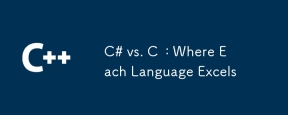
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
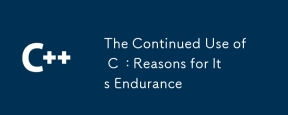
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
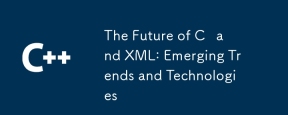
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
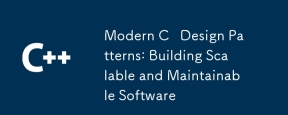
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
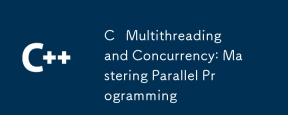
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
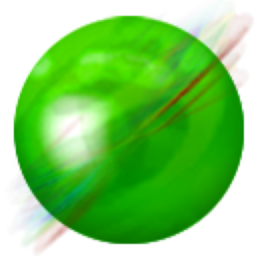
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
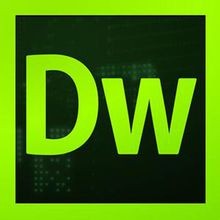
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version