The wonderful use of design patterns in avoiding code duplication
Design patterns reduce code duplication by providing reusable solutions, thereby making code more maintainable and readable. These patterns include: Factory Pattern: Used to create objects without specifying their concrete class. Strategy pattern: Allows an algorithm or behavior to change independently of how it is used. Singleton pattern: ensures that there is only one instance of a specific class. Observer pattern: allows objects to subscribe to events and be notified of them when they occur. Decoration mode: Dynamically extend the functionality of an object.
The wonderful use of design patterns in avoiding code duplication
Design patterns are reusable solutions to common software design problems. They can help keep code maintainable and readable by reducing code duplication.
Common design patterns to avoid code duplication
- Factory pattern: Used to create objects without specifying their concrete classes. This helps couple your code, allowing you to easily change the logic of creating objects.
- Strategy Mode: Allows an algorithm or behavior to change independently of how it is used. It allows you to create flexible applications by combining different policy objects.
- Singleton pattern: Ensures that there is only one instance of a specific class. This helps avoid the overhead of creating multiple identical objects.
- Observer Pattern: Allows objects to subscribe to events and then notify them when an event occurs. It is used to create loose coupling between objects.
- Decoration mode: Dynamicly extend the functionality of the object. It allows adding new functionality without modifying the original class.
Practical Case: Factory Pattern
Consider creating an application for creating various shapes. Without using design mode, you would need to write separate code for each shape.
public class Square { public void draw() { // ... } } public class Circle { public void draw() { // ... } } public class Rectangle { public void draw() { // ... } }
Using the factory pattern, you can separate the creation logic from the created object:
public interface Shape { void draw(); } public class ShapeFactory { public static Shape createShape(String type) { switch (type) { case "square": return new Square(); case "circle": return new Circle(); case "rectangle": return new Rectangle(); } return null; } }
Now you can do this by simply calling ShapeFactory.createShape("square")
to easily create different types of shape objects.
The above is the detailed content of The wonderful use of design patterns in avoiding code duplication. For more information, please follow other related articles on the PHP Chinese website!
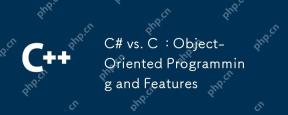
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
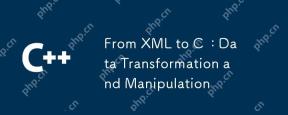
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
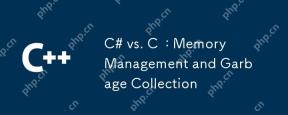
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
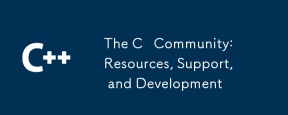
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
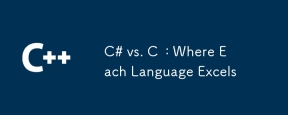
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
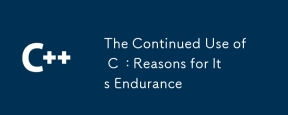
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
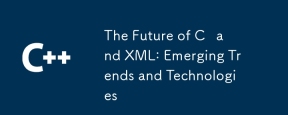
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
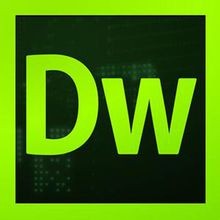
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
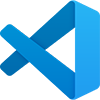
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft