super is the keyword used to access super class members in Java. The specific usage is: calling the method in the super class: super.method_name() accessing the variable in the super class: super.variable_name calling the super class Constructor: super(arguments)
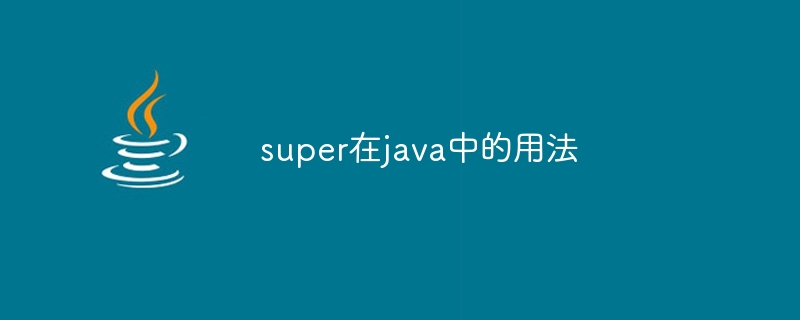
Usage of super in Java
What is super?
super is a keyword in Java used to access members of a super class. It refers to the immediate superclass of the class that calls it.
Usage of super
There are three main uses of super:
-
Access methods in the super class: super .method_name() will call the method named method_name in the superclass.
-
Accessing variables in a superclass: super.variable_name will refer to a variable named variable_name in the superclass.
-
Call the constructor of the super class: super(arguments) will call the constructor of the super class, passing the given parameters.
When to use super
Common situations where super is used include:
-
Override method: When a subclass needs to override a method in the superclass, super.method_name() can be used to call the overridden method.
-
Using variables or methods of the superclass: When a subclass needs to access variables or methods in the superclass, but they are not available in the subclass.
-
Call the constructor of the super class: When the subclass needs to initialize the state of the super class.
Example
<code class="java">class SuperClass {
int value = 10;
void printValue() {
System.out.println("SuperClass value: " + value);
}
}
class SubClass extends SuperClass {
int value = 20;
@Override
void printValue() {
super.printValue(); // 调用超类中的 printValue 方法
System.out.println("SubClass value: " + value);
}
}
public class Main {
public static void main(String[] args) {
SubClass obj = new SubClass();
obj.printValue(); // 输出:
// SuperClass value: 10
// SubClass value: 20
}
}</code>
Note:
- super can only be used in methods of subclasses .
- If the superclass does not have a method named method_name, super.method_name() will cause a compilation error.
- super cannot be used to access members in the parent interface.
- super cannot be used to access private members of the parent class.
The above is the detailed content of How to use super in java. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn