Java Data Structures and Algorithms: A Practical Guide to Big Data Analysis
Big data analysis applications of data structures and algorithms in Java Master data structures (arrays, linked lists, stacks, queues, hash tables) and algorithms (sorting, search, hashing, graph theory, and union lookup) for big data Data analysis is crucial. These data structures and algorithms provide mechanisms for efficient storage, management, and processing of massive amounts of data. Practical examples demonstrate the application of these concepts, such as using hash tables to quickly find word frequencies and using graph algorithms to find relevant nodes in social networks.
Java Data Structures and Algorithms: A Practical Guide to Big Data Analysis
Introduction
Master Data Structures and Algorithms Essential for big data analysis. This article will provide a practical guide to introduce key data structures and algorithms in Java, and demonstrate their application in big data analysis through practical cases.
Data structure
- Array: Ordered collection of elements, accessed using index.
- Linked list: A linear structure composed of nodes, each node contains data and a pointer to the next node.
- Stack: Last-In-First-Out (LIFO) data structure supports fast push and pop operations.
- Queue: First-In-First-Out (FIFO) data structure supports fast enqueue and dequeue operations.
- Hash table: A fast lookup structure that uses a hash function to map keys to values.
Algorithm
- Sort: Arrange the data set in a specific order.
- Search: Find specific elements in a data collection.
- Hash: Use a hash function to generate a unique representation of the key.
- Graph theory: Algorithms that study graphs (sets of nodes and edges).
- Union lookup: Maintain a disjoint set of elements.
Practical case
Case 1: Use hash table to quickly find word frequency
import java.util.HashMap; import java.util.StringJoiner; public class WordFrequencyCounter { public static void main(String[] args) { String text = "This is an example text to count word frequencies"; // 使用哈希表存储单词及其频率 HashMap<String, Integer> frequencyMap = new HashMap<>(); // 将文本拆分为单词并将其添加到哈希表中 String[] words = text.split(" "); for (String word : words) { frequencyMap.put(word, frequencyMap.getOrDefault(word, 0) + 1); } // 从哈希表中打印每个单词及其频率 StringJoiner output = new StringJoiner("\n"); for (String word : frequencyMap.keySet()) { output.add(word + ": " + frequencyMap.get(word)); } System.out.println(output); } }
Case 2: Using graph algorithms to find relevant nodes in social networks
import java.util.*; public class SocialNetworkAnalyzer { public static void main(String[] args) { // 创建一个图来表示社交网络 Map<String, Set<String>> graph = new HashMap<>(); // 添加节点和边到图中 graph.put("Alice", new HashSet<>(Arrays.asList("Bob", "Carol"))); graph.put("Bob", new HashSet<>(Collections.singleton("Dave"))); ... // 使用广度优先搜索找到与 Alice 相关的所有节点 Queue<String> queue = new LinkedList<>(); queue.add("Alice"); Set<String> visited = new HashSet<>(); while (!queue.isEmpty()) { String current = queue.remove(); visited.add(current); for (String neighbor : graph.get(current)) { if (!visited.contains(neighbor)) { queue.add(neighbor); } } } // 打印与 Alice 相关的所有节点 System.out.println(visited); } }
Conclusion
By mastering data structures and algorithms, Java programmers can manage efficiently and analyzing big data. This article provides practical examples that demonstrate the practical application of these concepts, enabling programmers to build complex and efficient big data analytics solutions.
The above is the detailed content of Java Data Structures and Algorithms: A Practical Guide to Big Data Analysis. For more information, please follow other related articles on the PHP Chinese website!
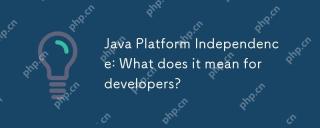
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
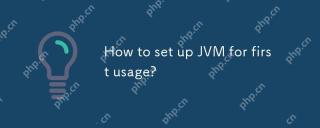
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
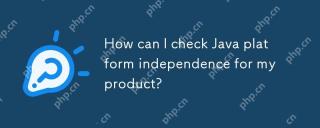
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss
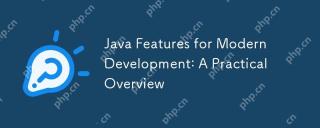
Javastandsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhancedconcurrencysupport.1)Lambdaexpressionssimplifyfunctionalprogramming,makingcodemoreconciseandreadable.2)Streamsenableefficientdataprocessingwithoperationsli
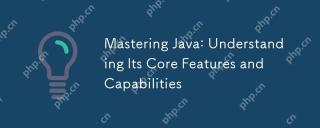
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
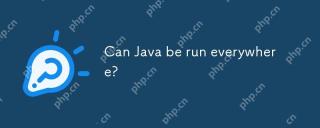
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
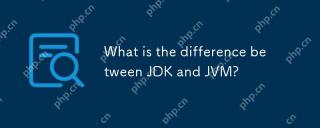
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
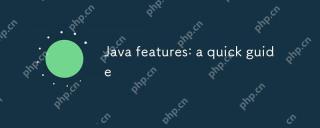
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
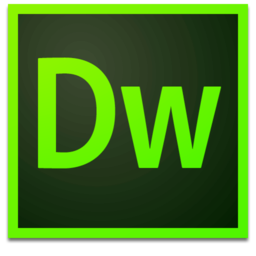
Dreamweaver Mac version
Visual web development tools
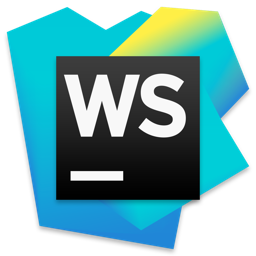
WebStorm Mac version
Useful JavaScript development tools
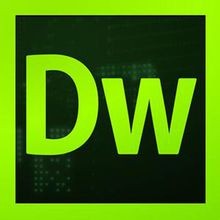
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
