Golang best practices and use cases in machine learning
Best practices for using the Go language effectively in machine learning include leveraging parallelism, garbage collection, type systems, and modular design. Use cases include image recognition, natural language processing, and machine learning model training, allowing developers to use the benefits of Go to create high-performing and easy-to-maintain applications.
Golang Best Practices and Use Cases in Machine Learning
The Go language is popular for its parallel processing capabilities, garbage collection mechanism, and fast compilation times. Welcome to the field of machine learning. Here are the best practices and use cases for using the Go language effectively in machine learning.
Best Practices
- Use Parallelism: Go’s coroutines can easily implement parallel computing, thereby increasing the speed of training and prediction of machine learning models.
- Take advantage of garbage collection: Go’s garbage collector automatically manages memory to ensure that applications are efficient and free of memory leaks.
- Use the type system: Go’s type system ensures code reliability, reduces errors, and improves maintainability.
- Modular design: Break the code into reusable modules for easy maintenance and expansion.
- Pay attention to performance: In machine learning applications, performance is crucial. Use Go's profiling tools to identify and optimize bottlenecks.
Use Case
Image Recognition:
package main import ( "fmt" "image" "image/color" "log" "github.com/golang/freetype/truetype" "golang.org/x/image/font" "golang.org/x/image/font/gofont/gomedium" "golang.org/x/image/math/fixed" ) func main() { // 加载图像 img, err := image.Open("image.jpg") if err != nil { log.Fatal(err) } // 创建画布 newImg := image.NewRGBA(img.Bounds()) // 加载字体 fontBytes, err := gomedium.TTF() if err != nil { log.Fatal(err) } fontFace, err := truetype.Parse(fontBytes) if err != nil { log.Fatal(err) } // 创建绘制上下文 c := font.Drawer{ Dst: newImg, Src: image.Black, Face: fontFace, Dot: fixed.I(2), } // 在图像上添加文本 c.DrawString("Machine Learning with Go", fixed.I(50), fixed.I(50)) // 保存新图像 if err := image.Encode(image.PNG, newImg, "new_image.png"); err != nil { log.Fatal(err) } fmt.Println("Image successfully processed.") }
Natural Language Processing:
package main import ( "fmt" "log" "github.com/gonum/nlp" ) func main() { // 创建 NLP 文档 doc, err := nlp.NewDocument("This is an example document.") if err != nil { log.Fatal(err) } // 分析文档中的名词短语 nounPhrases := doc.NounPhrases() for _, phrase := range nounPhrases { fmt.Println(phrase) } // 分析文档中的谓语短语 verbPhrases := doc.VerbPhrases() for _, phrase := range verbPhrases { fmt.Println(phrase) } }
Machine Learning Model Training:
package main import ( "fmt" "log" "github.com/tensorflow/tensorflow/core/protos/saved_model_pb2" "github.com/tensorflow/tensorflow/tensorflow/go" "github.com/tensorflow/tensorflow/tensorflow/go/op" ) func main() { // 加载预训练模型 model, err := tensorflow.LoadSavedModel("saved_model", nil) if err != nil { log.Fatal(err) } // 创建输入数据 inputData := []float32{0.1, 0.2, 0.3} // 创建输入张量 inputTensor := op.NewTensor(inputData) // 设置输出张量 outputTensor := model.Operation("output").Output(0) // 执行预测 outputs, err := model.Session.Run(map[tensorflow.Output]*tensorflow.Tensor{inputTensor: inputTensor}, []tensorflow.Output{outputTensor}, nil) if err != nil { log.Fatal(err) } // 获取预测结果 prediction := outputs[0].Value() fmt.Println(prediction) }
By following these best practices and use cases, developers can leverage the power of Go to create high-performance, maintainable, and scalable applications in machine learning projects program.
The above is the detailed content of Golang best practices and use cases in machine learning. For more information, please follow other related articles on the PHP Chinese website!
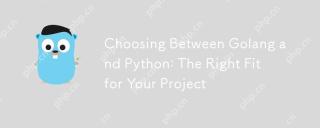
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
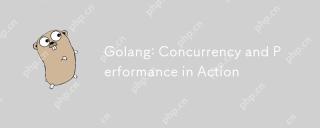
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
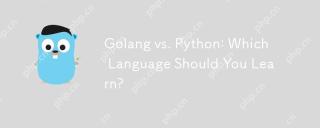
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
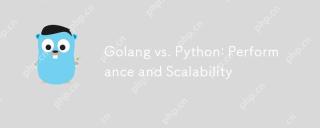
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
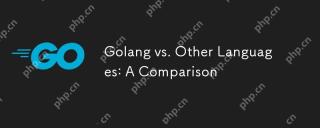
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
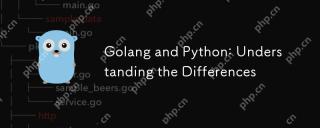
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
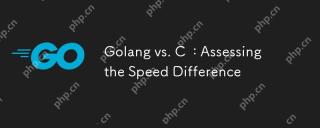
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
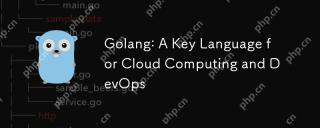
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
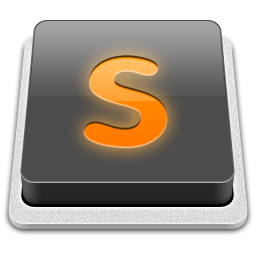
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.