1. Factory pattern: Separate object creation and business logic, and create objects of specified types through factory classes. 2. Observer pattern: Allows subject objects to notify observer objects of their state changes, achieving loose coupling and observer pattern.
PHP design pattern practical case analysis
Foreword
Design pattern is the solution Examples of proven solutions to common software design problems. They help create reusable, maintainable, and extensible code. In this article, we’ll explore some of the most commonly used design patterns in PHP and provide practical examples.
Factory Pattern
The best way to create objects is to separate the instantiation process from the business logic. The factory pattern uses a central factory class to decide which type of object to create.
Practical case: Creating a shape factory
interface Shape { public function draw(); } class Square implements Shape { public function draw() { echo "Drawing a square.\n"; } } class Circle implements Shape { public function draw() { echo "Drawing a circle.\n"; } } class ShapeFactory { public static function createShape(string $type): Shape { switch ($type) { case "square": return new Square(); case "circle": return new Circle(); default: throw new Exception("Invalid shape type."); } } } // Usage $factory = new ShapeFactory(); $square = $factory->createShape("square"); $square->draw(); // 输出:Drawing a square.
Observer pattern
The observer pattern allows an object (theme) Notify other objects (observers) about changes in their state.
Practical Case: Creating a Blog System
interface Observer { public function update(Subject $subject); } class Subject { protected $observers = []; public function attach(Observer $observer) { $this->observers[] = $observer; } public function detach(Observer $observer) { $key = array_search($observer, $this->observers); if ($key !== false) { unset($this->observers[$key]); } } public function notify() { foreach ($this->observers as $observer) { $observer->update($this); } } } class Post extends Subject { private $title; private $body; // ... Post related methods public function publish() { $this->notify(); } } class EmailObserver implements Observer { public function update(Subject $subject) { // Send an email notification for the new post. } } class PushObserver implements Observer { public function update(Subject $subject) { // Send a push notification for the new post. } } // Usage $post = new Post(); $observer1 = new EmailObserver(); $observer2 = new PushObserver(); $post->attach($observer1); $post->attach($observer2); $post->publish(); // Sends email and push notifications for the new post.
Summary
We explored factory and observer design through practical examples Patterns, explains how design patterns can improve code reusability, maintainability, and scalability.
The above is the detailed content of PHP design pattern practical case analysis. For more information, please follow other related articles on the PHP Chinese website!
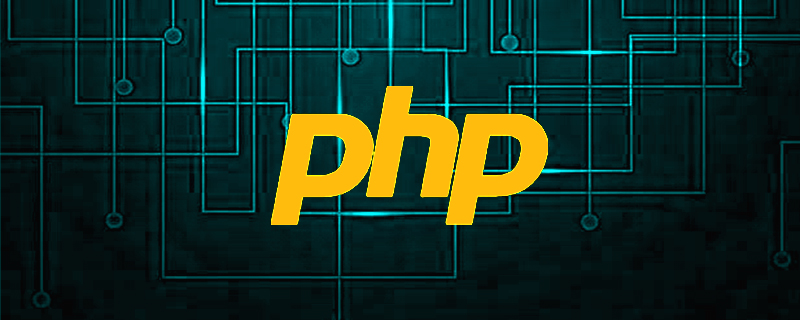
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
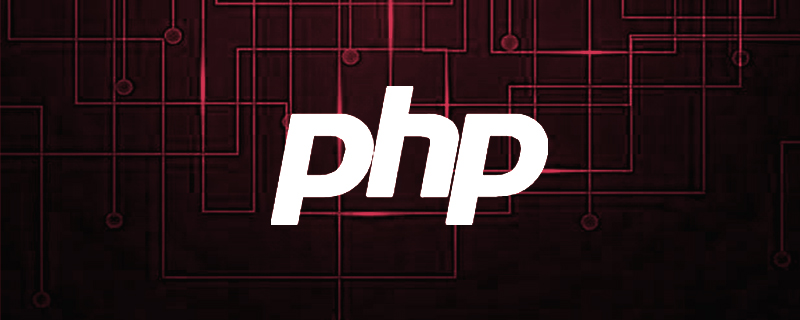
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
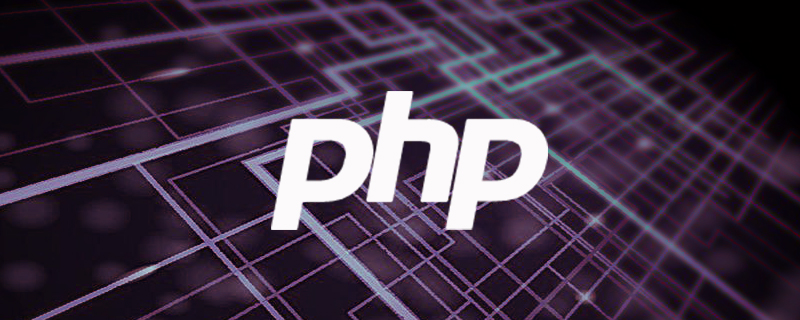
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
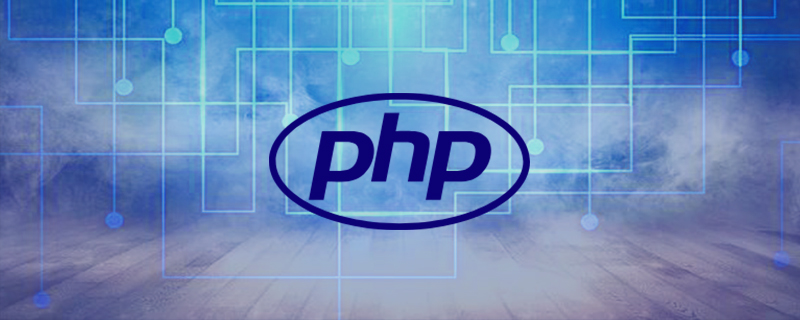
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
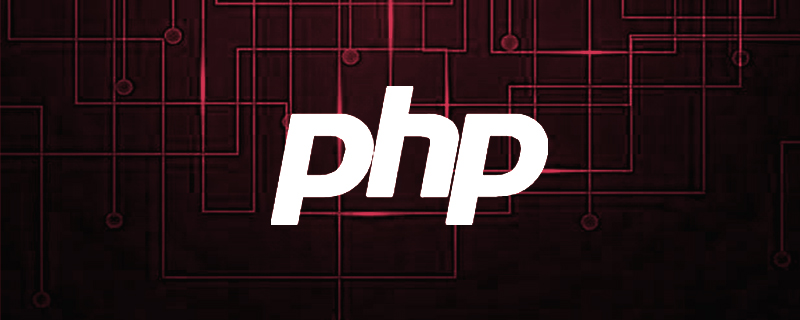
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
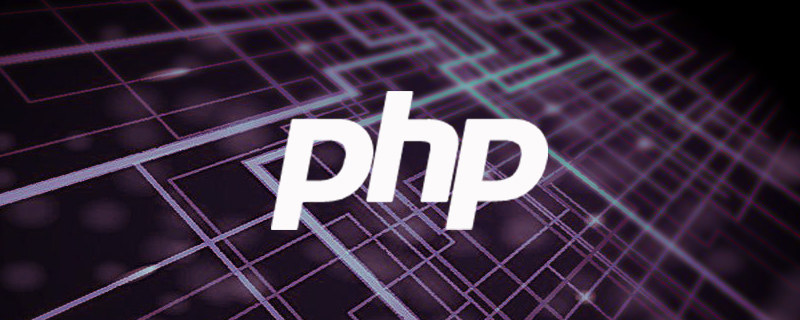
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
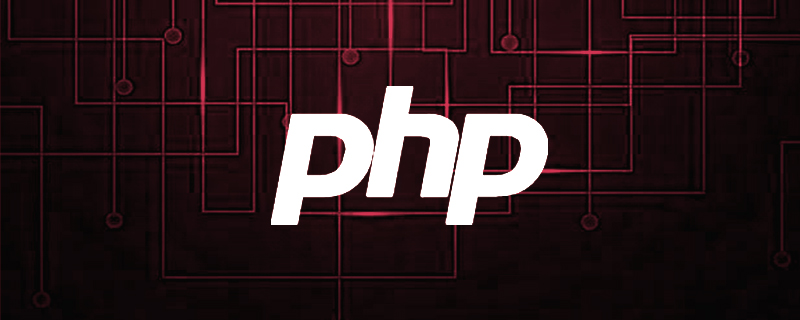
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
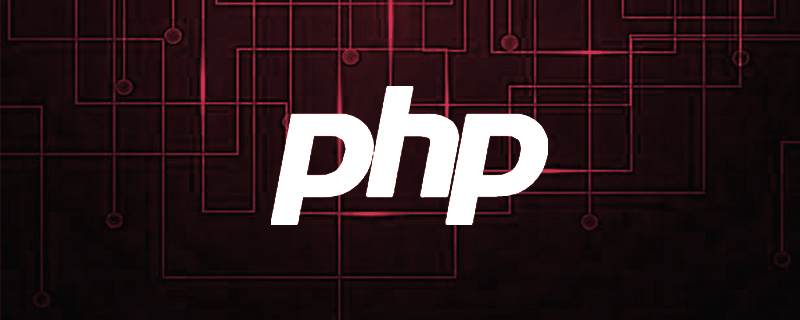
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
