I was browsing some Alibaba front-end interview questions recently, and one of them involves the use of the replace() method in JavaScript. Here is the original question:
“Tell me what the following functions do? What should be filled in the blank space?”
// define (function (window) { function fn(str) { this.str = str; } fn.prototype.format = function () { var arg = ______; return this.str.replace(_______, function (a, b) { return arg[b] || ''; }); } window.fn = fn; })(window); // use (function(){ var t = new fn('<p><a href="{0}">{1}</a><span>{2}</span></p>'); console.log( t.format('http://www.alibaba.com', 'Alibaba', 'Welcome') ); })();
The following is the analysis process (it feels like it is better to number it, personally I think it is more organized)
1. Because this question also involves other knowledge points, such as anonymous functions, prototypes, etc., but it is not the focus of this discussion.
2. According to the question, we know that the source code of this question is similar to writing a template engine. Replace placeholders such as '{1}' in the template with the parameters passed to it. So arg should be arguments. but! ! ! Since arg is not an array, but an array-like object (if you don’t understand, you can google it yourself (u_u)), so we need to do some conversions,
3. The right side of the equal sign is the first empty answer. Having said so much, then the second space is the focus of our discussion~~~~~~We all know that the second space is to find the placeholder through regular expressions and convert it according to the numbers in the placeholder. Replace it with the string in the arg array. To be honest, it is rarely encountered that the second parameter of the replace method is a function. Generally, this is the case when we encounter it. Look at the following code:
var pattern=/8(.*)8/;
var str='This is a 8baidu8';
document.write(str.replace(pattern,'$1'));
4. Since there are relatively few situations where the second parameter of replace is a function, let’s focus on the situation where the second parameter is a function.
First of all, this is the syntax of the replace function: stringobject.replace(regexp/substr,replacement)
Where regexp/substr is required. A regexp object that specifies the string or pattern to be replaced. (Note that if the value is a string, it is retrieved as a literal literal pattern rather than first being converted to a regexp object.) replacement required. A string value. Specifies functions for replacing text or generating replacement text. Finally, a new string is returned, which is obtained after replacing the first match or all matches of regexp with replacement.
5. ECMAScript stipulates that the parameter replacement of the replace() method can be a function instead of a string. In this case, the function is called for each match and the string it returns is used as the replacement text. The first parameter represents the matched character, the second parameter represents the minimum index position of the matched character (RegExp.index), and the third parameter represents the matched string (RegExp.input).
6. So the second space can be written like this: /{(d )}/g, and the complete sentence when placed in the statement is:
return this.str.replace(/{(d )}/g, function (a, b) {
Return arg[b] || '';
});
When performing the first match, {0} is replaced by arg[0]
When performing the first match, {1} is replaced by arg[1]
When performing the first match, {2} is replaced by arg[2]
7. The above is the explanation of the second parameter of the js string method replace() as a function (if there are any imperfections, please add them by yourself). Of course, this interview question has also been solved~~~~~
The above is the entire content of this article, I hope you all like it.
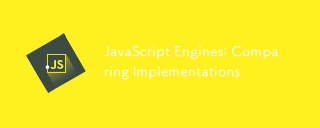
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
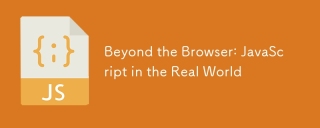
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
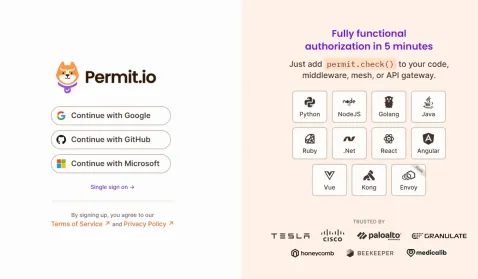
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
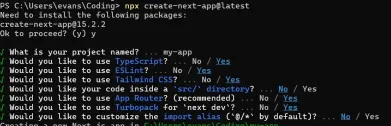
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
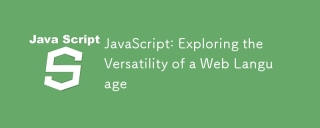
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
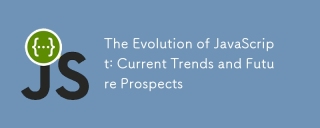
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
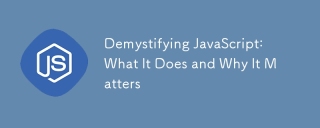
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
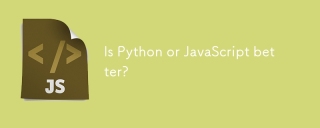
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
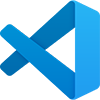
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
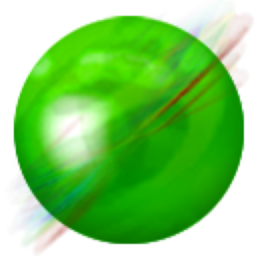
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
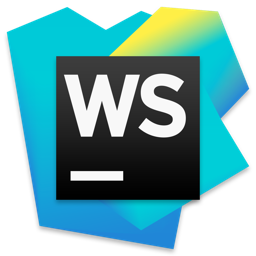
WebStorm Mac version
Useful JavaScript development tools