


A high-energy manual for getting started with Golang technology: resolving novice confusion
Go language beginner's manual covers the following content: Installation and configuration: download the tool chain, set environment variables, and verify the installation. Basic syntax: variable declaration, function declaration, data types, control flow, arrays and slices. Concurrent programming: Go processes, channels, synchronization primitives. Data structures: map, slice, struct. Practical case: Create a simple web server and run it in the browser.
Go Technical Introductory Manual: Step by step to resolve the confusion of novices
Introduction
Go , a modern programming language developed by Google, is loved by developers for its high performance, ease of learning, and concurrency. This manual will take you into the world of Go programming and solve the confusion that novices often encounter.
Installation and Configuration
- Download and install the Go language tool chain: https://golang.org/dl/
- Settings
GOPATH
Environment variable, pointing to the root directory of the Go workspace. - In the command line, run
go version
to verify that the installation was successful.
Basic syntax
The syntax of Go language is concise and clear:
- Variable declaration:
var name type
- Function declaration:
func funcName(params) returnType
- Data type:
int
,float
,string
,bool
- Control flow:
if
,for
,switch
- Arrays and slices:
[]type
,[length]type
Concurrent Programming
Go's largest One of the advantages is concurrent programming:
- Goroutine: lightweight thread that allows multiple tasks to be performed simultaneously.
- Channel: Pipe used to communicate between Goroutines.
-
sync
Package: Provides concurrency primitives such as mutex locks and condition variables.
Data structures
Go provides some common data structures:
-
map
: key Collection of value pairs -
slice
: Dynamically sized array -
struct
: Custom type, containing multiple fields
Practical case: Web server
Create a simple HTTP server:
package main import ( "fmt" "net/http" ) func main() { // 定义一个处理函数 handler := func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, world!") } // 创建一个 HTTP 服务器 srv := &http.Server{ Addr: ":8080", Handler: http.HandlerFunc(handler), } // 启动服务器 err := srv.ListenAndServe() if err != nil { fmt.Println(err) } }
Run this code, and then visit http:// in the browser localhost:8080
, you will see the "Hello, world!" message. This simple case demonstrates the web programming capabilities of the Go language.
The above is the detailed content of A high-energy manual for getting started with Golang technology: resolving novice confusion. For more information, please follow other related articles on the PHP Chinese website!
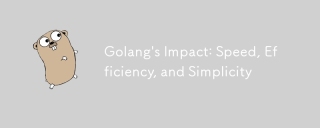
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
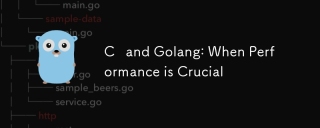
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
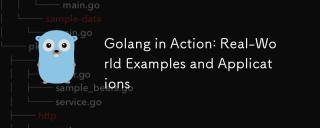
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
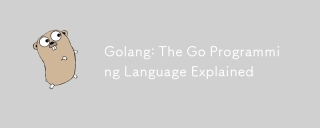
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
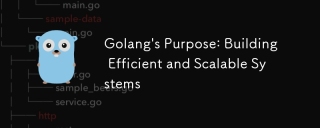
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
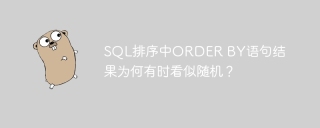
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
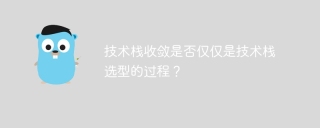
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
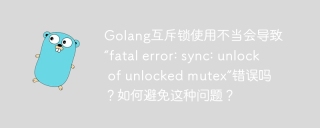
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
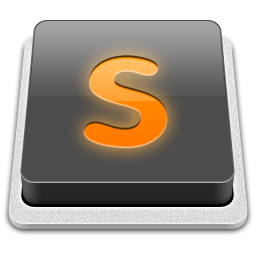
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
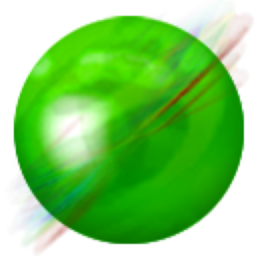
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment