


C++ Concurrent Programming: How to do thread termination and cancellation?
Thread termination and cancellation mechanisms in C include: Thread termination: std::thread::join() blocks the current thread until the target thread completes execution; std::thread::detach() detaches the target thread from thread management. Thread cancellation: std::thread::request_termination() requests the target thread to terminate execution; std::thread::get_id() obtains the target thread ID and can be used with std::terminate() to immediately terminate the target thread. In practice, request_termination() allows the thread to decide the timing of termination, and join() ensures that the worker thread completes execution before the main thread exits. Note that thread cancellation is not always respected, and terminating a thread may lead to undefined behavior.
#C Concurrent Programming: How to perform thread termination and cancellation?
In multi-threaded applications, thread termination and cancellation are critical operations when a specific thread or its task is no longer needed. C provides multiple mechanisms to implement thread termination and cancellation:
Thread termination
- ##std::thread::join()
: Block the current thread until the target thread completes execution.
- std::thread::detach()
: Detach the target thread from thread management. The thread will continue executing but can no longer be accessed through its
std::threadobject.
Thread cancellation
- ##std::thread::request_termination()
- : Request the target thread to terminate execution. The thread can decide whether to honor this request.
- : Get the ID of the target thread, which can be passed to the
std::terminate()
function. This immediately terminates the target thread.
Consider an example where two threads are created: the main thread and the worker thread. The worker thread executes an infinite loop while the main thread waits for user input and then terminates the worker thread:
#include <iostream> #include <thread> void work_thread() { while (true) { // 无限循环 } } int main() { std::thread worker(work_thread); std::cout << "按回车键终止工作线程:" << std::endl; std::cin.get(); worker.request_termination(); worker.join(); return 0; }Points
##request_termination()
- Allow worker threads to decide when to terminate themselves.
-
join()
Ensures that the worker thread completes execution before the main thread exits.
Thread cancellation is not always respected, especially if the thread performs a critical section or other uninterruptible operation.
- Terminating a thread may cause undefined behavior, so use with caution.
The above is the detailed content of C++ Concurrent Programming: How to do thread termination and cancellation?. For more information, please follow other related articles on the PHP Chinese website!
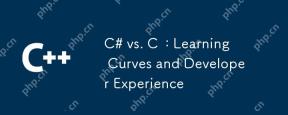
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
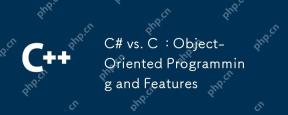
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
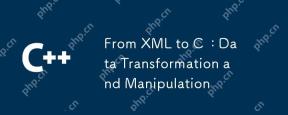
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
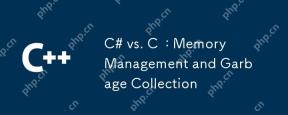
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
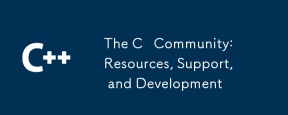
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
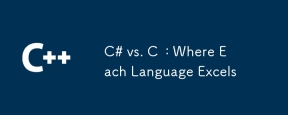
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
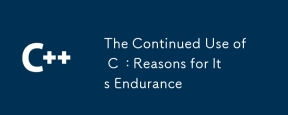
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
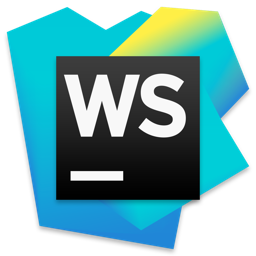
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor