By extending and customizing the PHPUnit framework, the problem that the original framework cannot meet the needs can be solved. In terms of extension, it includes custom assertions, Matcher and DataProvider; in terms of customization, it involves creating custom runners and overriding Bootstrapper. In practical applications, extended assertions can verify special characters, custom Matchers can verify list elements, and overriding Bootstrapper can increase the execution timeout limit.
Extension and customization of PHP unit testing framework
Unit testing can significantly improve code quality, but the native framework may not be able to meet the requirements All needs. Extending and customizing the framework solves this problem.
Extension methods
1. Custom assertion
PHPUnit provides assertion methods, but sometimes custom assertions are required. Create a new assertion method using the Assert
class:
class CustomAssertions extends PHPUnit_Framework_Assert { public static function assertTrueWithMessage($condition, string $message) { self::assertTrue($condition, $message); } }
2. Custom Matcher
Matcher validates whether a value meets specific conditions. Create a custom Matcher using the prophesize
library:
class CustomMatcher { public function isEven($value) { return $value % 2 == 0; } } $propecy = $prophesize(new CustomMatcher()); $propecy->isEven(6)->shouldBeTrue();
3. Implement DataProvider
DataProvider provides a custom source for test data. Use PHPUnit_Extensions_DataProvider_ArrayDataProvider
to create a custom DataProvider:
class CustomDataProvider { public static function provideData() { return [ ['foo', 'bar'], ['baz', 'qux'] ]; } } $dataProvider = new PHPUnit_Extensions_DataProvider_ArrayDataProvider(CustomDataProvider::provideData());
Customized framework
1. Create a custom runner
The runner is responsible for executing tests. Create a custom runner using PHPUnit_Framework_TestSuite_DataProvider
:
class CustomTestRunner extends PHPUnit_Framework_TestSuite_DataProvider { protected function setUp(): void { // 自定义设置 } protected function tearDown(): void { // 自定义清理 } }
2. Override Bootstrapper
Bootstrapper Set up the test running environment before the test runs. Use PHPUnit_Util_Configuration
to override Bootstrapper:
class CustomBootstrapper { public static function bootstrap() { // 自定义引导 PHPUnit_Util_Configuration::$defaultEnforceTimeLimit = 300; } } PHPUnit_Util_Configuration::$bootstrap = 'CustomBootstrapper::bootstrap';
Practical case
##Extended assertion:Verify the existence of special characters:
CustomAssertions::assertTrueWithMessage( strpos($string, "\t") !== false, "String does not contain a tab character" );
Custom Matcher:Verify that list contains elements:
$prophesize(new CustomMatcher())->contains(['foo', 'bar'])->shouldBeTrue();
Override Bootstrapper:Increase execution timeout limit to 300 seconds:
CustomBootstrapper::bootstrap();
The above is the detailed content of Extension and customization of PHP unit testing framework. For more information, please follow other related articles on the PHP Chinese website!
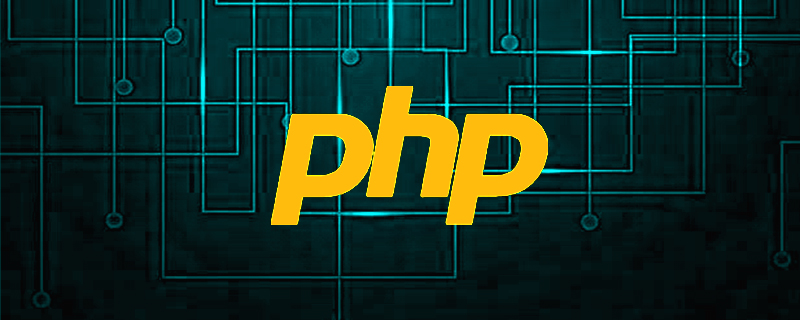
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
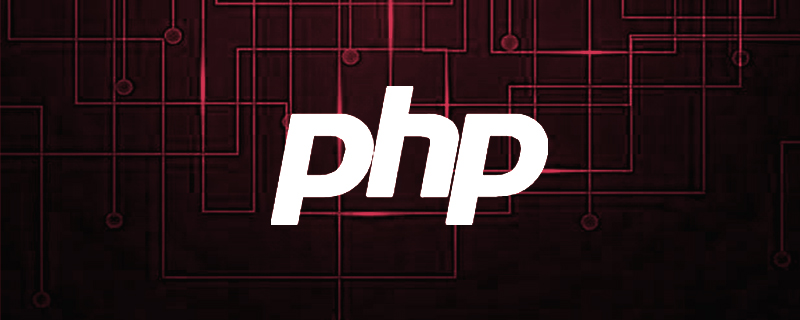
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
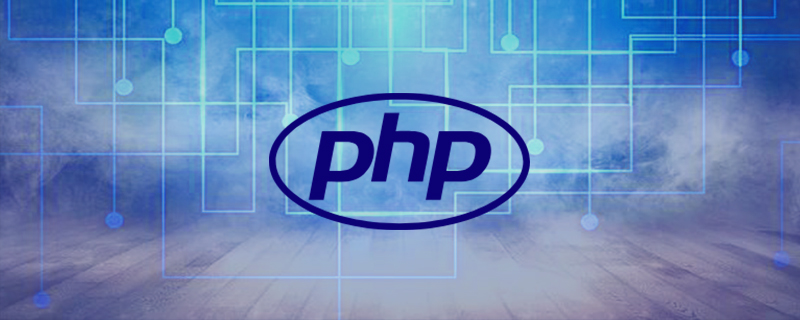
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
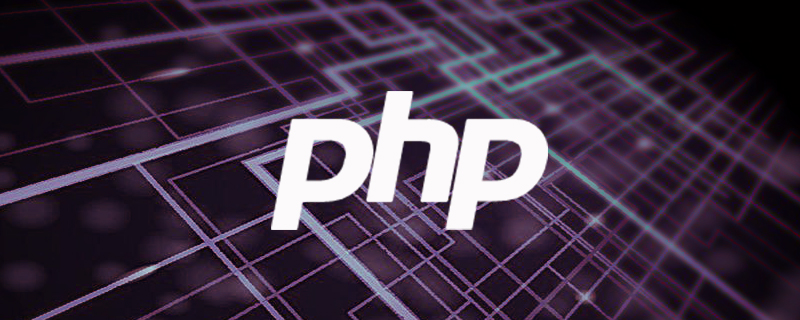
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
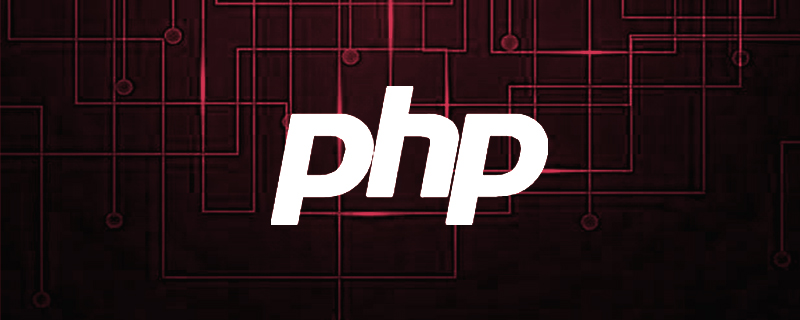
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
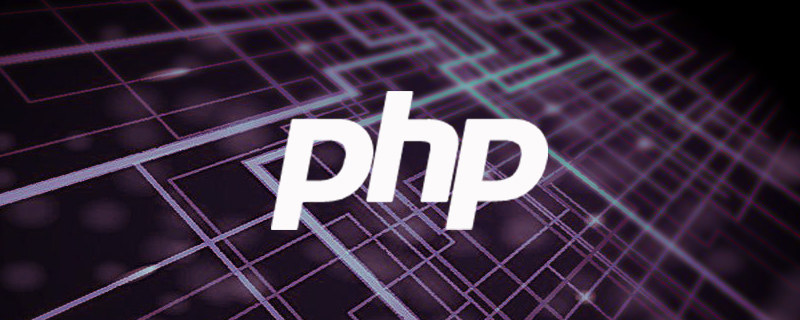
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
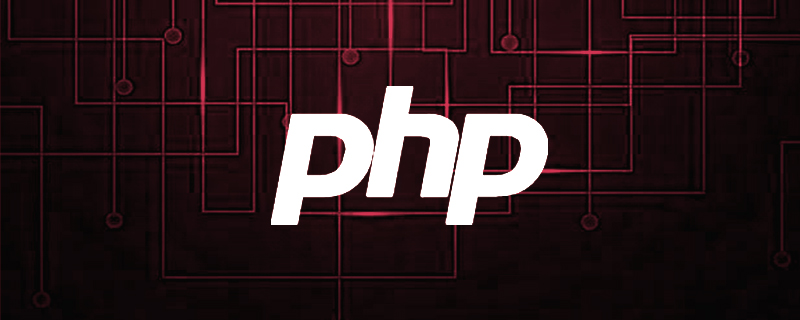
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
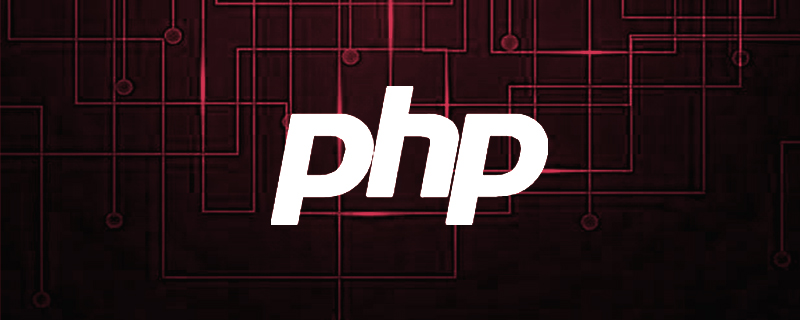
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
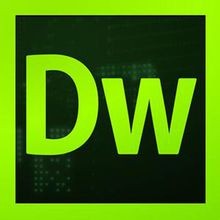
Dreamweaver CS6
Visual web development tools
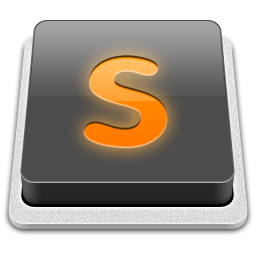
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!
